Bug Detection
Code Generation and Analysis with LLMs. Comparing the leading AI models side-by-side at Bug Detection.
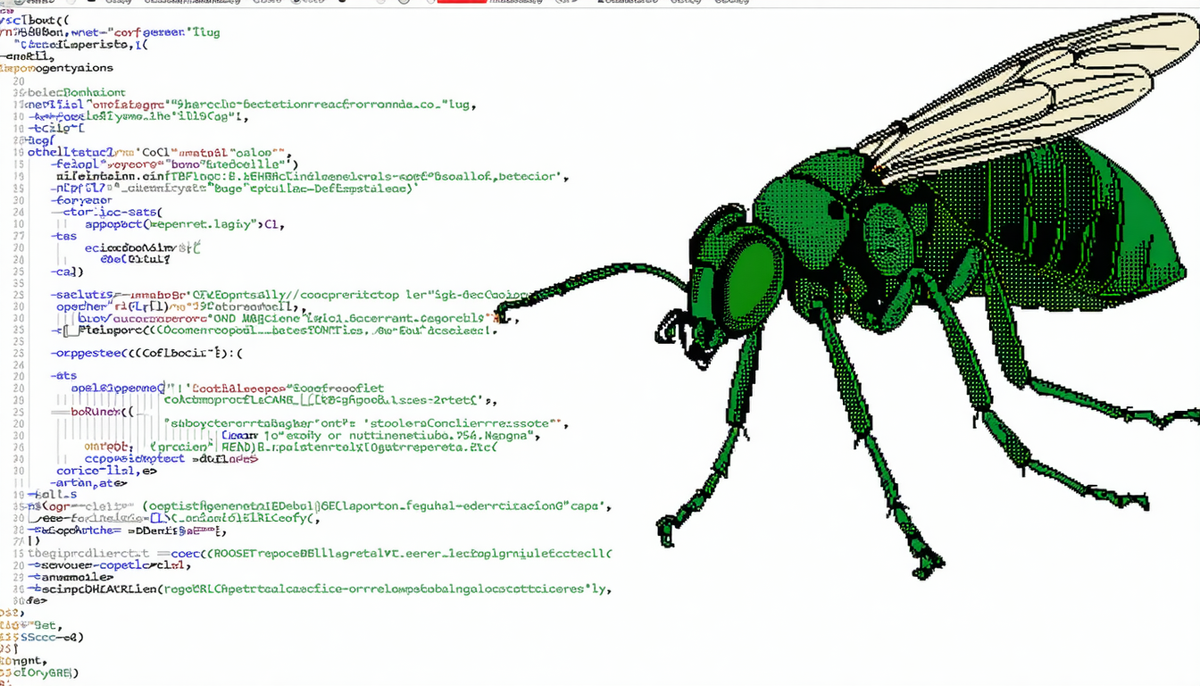
Comparing the leading AI models:
Category: Code Generation and Analysis
Subcategory: Bug Detection
Contents
- Introduction
- Bug Detection
- Prompts
- Array Index Out of Bounds Review
- Division Error Handling Analysis
- Logic Flow Error Detection
- Infinite Loop Risk Assessment
- Password Validation Security Review
- Bubble Sort Implementation Analysis
- Resource Management Review
- Concurrent Access Analysis
- Recursive Function Error Detection
- Authentication Security Audit
- Performance Verdict
- Budget Verdict
- Conclusion
Introduction
Comparing AI Models: A Practical Guide to LLM Performance
Looking to compare AI models and find the perfect large language model (LLM) for your needs? This comprehensive guide evaluates leading AI models side-by-side, helping you make informed decisions about which artificial intelligence solution best fits your use case and budget.
Our comparison framework divides popular LLMs into two distinct tiers:
Budget-Focused Tier:
- ChatGPT 4.0 Mini
- Gemini 1.5 Flash
- Claude 3.5 Haiku
- Llama 3.1 8B
Performance-Focused Tier:
- ChatGPT 4.0
- Claude 3.5 Sonnet
- Gemini 1.5 Pro
- Llama 3.1 70B
By comparing AI models across standardized prompts and use cases, you can better understand their strengths, limitations, and optimal applications. The budget tier is ideal for routine tasks, content generation, and basic analysis, offering excellent value for everyday use. Choose the performance tier when accuracy, nuanced understanding, and complex reasoning are crucial - particularly for professional, technical, or specialized applications.
Our side-by-side AI comparisons examine real-world examples, providing clear insights into how each model handles identical prompts. This practical approach helps you balance cost against capability, ensuring you select the right AI tool for your specific needs.
50+ AI models with one subscription. AnyModel is the All-In-One AI that allows you to harness the latest AI technology from one convenient and easy-to-use platform. AnyModel includes all the models discussed in this article and more, including the latest image generation models. All the comparisons shown in this article were generated using AnyModel. Sign up for a free trial here.
Bug Detection
Large Language Models have emerged as powerful tools for detecting and diagnosing software bugs, offering developers an efficient way to identify issues in their code. By leveraging their extensive training on billions of lines of code and common programming patterns, LLMs can quickly spot potential errors, syntax issues, logic flaws, and security vulnerabilities that might otherwise take hours to discover manually.
These AI models excel at bug detection because they understand both the syntactic and semantic aspects of code across multiple programming languages. They can analyze code context, identify inconsistencies with best practices, and even predict potential runtime errors before execution. This capability stems from their exposure to countless examples of both correct and problematic code during training, enabling them to recognize subtle patterns that often lead to bugs.
What makes LLMs particularly valuable for bug detection is their ability to explain the problems they find in natural language, suggest fixes, and provide reasoning for their recommendations. Whether dealing with simple syntax errors or complex logical flaws, these models can serve as an intelligent debugging assistant, helping developers maintain code quality and reduce the time spent on troubleshooting.
Prompts
Array Index Out of Bounds Review
Identifies potential array index out of bounds errors in list/array manipulation code. Useful for catching common indexing issues in loop operations.
Prompt:
Review this code for potential bugs: for i in range(len(arr)): if arr[i] > arr[i+1]: swap(arr[i], arr[i+1])
Performance Results
There are several potential issues in this code that you should address:
- Index Out of Range Error:
- The loop
for i in range(len(arr)):
goes from 0 tolen(arr) - 1
. However, within the loop, the code attempts to accessarr[i+1]
. Wheni
islen(arr) - 1
,arr[i+1]
will be out of bounds, causing an "IndexError". - To fix this, you should loop to
len(arr) - 1
, like this:for i in range(len(arr) - 1):
.
- Undefined
swap
Function:
- The function
swap(arr[i], arr[i+1])
seems to swap the elements, butswap
is not a built-in function in Python. - You need to implement the swap logic correctly. If you want to swap two elements, you can do it directly in Python as follows:
python arr[i], arr[i+1] = arr[i+1], arr[i]
- Unspecified Array and Context:
- Ensure that
arr
is a list or a data structure that supports indexing and element swapping. - It's not clear what the broader goal of this code is, but if you're trying to sort the list (e.g., using a form of bubble sort), this current code will only make a single pass. Keep in mind that a complete bubble sort requires multiple passes through the array until no more swaps are necessary.
Here's a fixed version of your loop assuming you want to make a single pass to swap adjacent elements if needed:
for i in range(len(arr) - 1):
if arr[i] > arr[i+1]:
arr[i], arr[i+1] = arr[i+1], arr[i]
And if you are aiming for a full bubble sort, you might need to incorporate this loop inside another loop that ensures repeated passes over the list until it's sorted:
swapped = True
while swapped:
swapped = False
for i in range(len(arr) - 1):
if arr[i] > arr[i+1]:
arr[i], arr[i+1] = arr[i+1], arr[i]
swapped = True
Please clarify the goal if this is not meant to resemble a bubble sort or similar algorithm.
Code Review: Array Comparison Loop
Bugs Identified:
- Index Out of Bounds Error
- The loop will throw an
IndexError
wheni
reaches the last element arr[i+1]
will attempt to access beyond the array's bounds
- Swap Function Issues
- The
swap()
function call likely doesn't work as intended - Python doesn't have a built-in
swap()
function - This implementation won't actually swap array elements
Suggested Fix:
# Version 1: Using range with adjusted length
for i in range(len(arr) - 1): # Prevent index out of bounds
if arr[i] > arr[i+1]:
arr[i], arr[i+1] = arr[i+1], arr[i] # Proper Python swap
# Version 2: Alternative with explicit swap function
def swap(arr, i, j):
arr[i], arr[j] = arr[j], arr[i]
for i in range(len(arr) - 1):
if arr[i] > arr[i+1]:
swap(arr, i, i+1)
Best Practices:
- Always consider array bounds when using indices
- Use Python's tuple unpacking for swaps
- If using a separate swap function, pass the array and indices, not just the values
The provided code has two main potential bugs:
IndexError: list index out of range
: Wheni
reaches the last valid index ofarr
,arr[i+1]
will try to access an element beyond the end of the list, causing anIndexError
.Incomplete Sorting: A single pass of swapping adjacent elements is not sufficient to sort the entire array. This code resembles a single pass of Bubble Sort, and Bubble Sort requires multiple passes to guarantee correct sorting.
Here's a breakdown and how to fix these issues:
Fixing the IndexError
:
The simplest solution is to change the loop range to stop one element earlier:
for i in range(len(arr) - 1):
if arr[i] > arr[i+1]:
swap(arr[i], arr[i+1])
Fixing the Incomplete Sorting (Implementing Bubble Sort):
To fully sort the array, you need nested loops. The outer loop controls the number of passes, and the inner loop performs the comparisons and swaps within each pass. Here's a corrected implementation of Bubble Sort:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
# Flag to optimize - if no swaps occur, the array is sorted
swapped = False
# Last i elements are already in place, so we don't need to check them
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j] # Pythonic swap
swapped = True
if not swapped:
break # Optimization: if no swaps, the array is sorted
# Example usage:
arr = [64, 34, 25, 12, 22, 11, 90]
bubble_sort(arr)
print("Sorted array:", arr)
Key improvements in the corrected Bubble Sort:
- Correct Looping: The outer loop iterates
n
times (wheren
is the length of the array), and the inner loop iterates up ton-i-1
to avoid theIndexError
and unnecessary comparisons. - Pythonic Swap: Uses the concise
arr[j], arr[j+1] = arr[j+1], arr[j]
syntax for swapping elements. - Optimization with
swapped
flag: Checks if any swaps occurred in a pass. If no swaps happen, it means the array is already sorted, and the algorithm can terminate early. This improves efficiency in cases where the array is nearly sorted or becomes sorted before all passes complete.
If your goal isn't to implement Bubble Sort specifically, but simply to sort the array, Python offers built-in sorting mechanisms that are more efficient:
arr.sort() # Sorts in place
sorted_arr = sorted(arr) # Returns a new sorted list without modifying the original
These built-in functions use Timsort, which is highly optimized and generally performs much better than Bubble Sort for larger datasets. Use Bubble Sort primarily for learning purposes or when working with very small datasets where the simplicity might outweigh the performance difference.
Code Review: Potential Bugs
The provided code appears to be part of a simple swapping algorithm, but it has a few issues that could lead to bugs or errors.
Bug 1: Out-of-Range Access
In the loop, i
iterates over the indices of arr
. However, when i
reaches the last index of arr
, arr[i+1]
will be out of range, causing an IndexError
. This is because i+1
will be equal to len(arr)
, which is outside the valid index range for arr
.
Bug 2: Swap Function
The swap
function is not defined in Python. In Python, you can swap two values without using a separate function. However, if you're trying to define a custom swap
function, it's not implemented correctly. A simple implementation would be:
def swap(a, b):
a, b = b, a
return a, b
However, this implementation still won't work as expected because it doesn't modify the original list arr
. You can't directly swap two values in a list in Python. Instead, you can use tuple packing and unpacking:
arr[i], arr[i+1] = arr[i+1], arr[i]
Revised Code
for i in range(len(arr) - 1):
if arr[i] > arr[i+1]:
arr[i], arr[i+1] = arr[i+1], arr[i]
Alternatively, you can use Python's built-in enumerate
function to iterate over the indices and values of arr
:
for i, (current, next_val) in enumerate(zip(arr, arr[1:])):
if current > next_val:
arr[i], arr[i+1] = arr[i+1], arr[i]
Both of these revised versions fix the out-of-range access bug and correctly swap the values in the original list arr
.
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
The code you provided seems to be part of an implementation that checks adjacent elements in an array (arr
) and swaps them if the first element is greater than the second. However, there are potential bugs and issues in this snippet:
Index Out of Range Error: The code uses
arr[i+1]
, which means that wheni
equals the last index (len(arr) - 1
),i + 1
will exceed the array bounds, leading to anIndexError
.Undefined swap function: The
swap
function is not defined in the provided code. You need to implement this function or use a proper syntax for swapping in Python.Ineffective Sort: If the intention is to sort the array, this approach only performs one pass through the array and does not guarantee that all elements will be sorted.
Here’s a revised version of your code that addresses these issues:
def swap(arr, i, j):
"""Swap elements at index i and j in the array arr."""
arr[i], arr[j] = arr[j], arr[i]
def bubble_sort(arr):
"""Sort the array using bubble sort."""
n = len(arr)
for i in range(n):
for j in range(n - 1): # Use n - 1 to avoid out of range error
if arr[j] > arr[j + 1]:
swap(arr, j, j + 1)
# Example usage:
arr = [3, 1, 4, 1, 5]
bubble_sort(arr)
print(arr) # Output: [1, 1, 3, 4, 5]
Changes Made:
- Defined a
swap
function: This clearly defines how two elements in the list should be swapped. - Used a nested loop: A second loop is added to ensure multiple passes over the array to sort it completely.
- Adjusted indices in the inner loop: The inner loop uses
range(n - 1)
to ensure that we don't attempt to access an index that is out of bounds.
This will sort the array correctly using Bubble Sort, addressing the potential bugs you initially had.
The primary bug lies in the indexing. The loop iterates from i = 0
to len(arr) - 1
. However, inside the if
condition, arr[i+1]
is accessed. When i
reaches len(arr) - 1
, i+1
becomes len(arr)
, which is an index out of bounds for the array, causing an IndexError
.
Here's a breakdown of the problem and how to fix it:
Problem: The loop tries to compare the last element with a non-existent element beyond the array's bounds.
Solution: The loop needs to stop one element earlier. The corrected code would be:
for i in range(len(arr) - 1):
if arr[i] > arr[i+1]:
swap(arr[i], arr[i+1])
This ensures that i+1
always remains a valid index within the array.
Further Considerations:
swap
function: The code assumes the existence of aswap
function. You'll need to define this function appropriately, for example:
def swap(a, b):
a, b = b, a # Pythonic way to swap
Algorithm: This code implements a single pass of a bubble sort. It will not fully sort the array if it's not already nearly sorted. For a complete sort, you'd need nested loops or a more efficient sorting algorithm.
Error Handling (optional but good practice): If there's a possibility
arr
could beNone
or empty, you should add checks to prevent errors:
def bubble_sort_pass(arr):
if arr is None or len(arr) < 2:
return arr #Nothing to sort
for i in range(len(arr) - 1):
if arr[i] > arr[i+1]:
arr[i], arr[i+1] = arr[i+1], arr[i] #In-place swap
return arr
In summary, the original code has a significant index out-of-bounds bug. The provided solutions address this and offer improvements in clarity and robustness. Remember that this only performs one pass of a bubble sort; a full sort requires more iterations.
Here are the potential bugs and issues in this code:
1. **IndexOutOfBounds Error**
- The loop `range(len(arr))` goes up to the last index of the array
- `arr[i+1]` will cause an `IndexError` on the last iteration
- When `i` is the last index, `arr[i+1]` will try to access an element beyond the array's bounds
2. **Incorrect Swap Implementation**
- The `swap(arr[i], arr[i+1])` appears to be incorrect syntax
- In most languages, you'd need to:
```python
# Correct swap
arr[i], arr[i+1] = arr[i+1], arr[i]
```
3. **Incomplete Sorting Logic**
- This looks like an attempt at a bubble sort
- However, this single pass will not fully sort the array
- A complete bubble sort requires multiple passes through the array
4. **No Boundary Check**
- There's no validation that `arr` is not empty
- Empty array will immediately cause an `IndexError`
5. **Potential Type Mismatch**
- Assumes all elements are comparable
- Will fail if array contains mixed types or non-comparable elements
Recommendation: Implement a complete bubble sort algorithm with proper bounds checking and multiple passes.
The provided code snippet appears to be attempting to implement bubble sort, which sorts an array in ascending order. However, there's a potential bug in the code:
for i in range(len(arr)):
if arr[i] > arr[i+1]:
swap(arr[i], arr[i+1])
Here's what's wrong:
Index out of range: When
i
is the last index of the array (i.e.,i == len(arr) - 1
), attempting to accessarr[i+1]
will result in anIndexError
, becausei+1
is out of range. This is because list indices in Python start at 0 and end atlen(list) - 1
.Unnecessary comparisons and swaps: In bubble sort, comparisons and swaps should be done only when
arr[i]
is greater thanarr[i+1]
. However, when comparingarr[i]
andarr[i+1]
, if they are in the correct order (i.e.,arr[i] <= arr[i+1]
), there's no need to swap them. The loop then proceeds to the next iteration.Missing
break
statement: Bubble sort requires a break statement when a pass is complete. If no two adjacent elements are swapped in a pass, the array is already sorted and we can exit the loop early.Undefined
swap
function: Theswap(arr[i], arr[i+1])
line calls a non-existentswap
function. This function should be defined or replaced with the actual swapping logic.
Here's a corrected version of the code, along with the swap
function:
def swap(arr, i, j):
"""Swap two elements at indices i and j in the array."""
arr[i], arr[j] = arr[j], arr[i]
def bubble_sort(arr):
"""Sort the array using bubble sort."""
n = len(arr)
for i in range(n):
swapped = False # Flag to track if any swaps were made in a pass
for j in range(n - 1):
if arr[j] > arr[j + 1]:
swap(arr, j, j + 1)
swapped = True # Set swapped to True if a swap occurred
if not swapped: # If no swaps were made in a pass, we're done
break
return arr
This version of the bubble sort implementation fixes the potential bugs mentioned above and includes comments for clarity.
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
The models showed varying levels of detail and approach in identifying code issues. ChatGPT 4.0 provided the most comprehensive analysis, detailing three distinct issues with example fixes. Claude was concise and well-structured, focusing on key bugs with clear solutions. Gemini offered extensive explanations and optimization suggestions, though perhaps too detailed for the simple prompt. Llama gave a focused response highlighting the critical index and swap issues. Response times varied significantly - Llama was fastest at 1.9s while Gemini took 15.6s. All models correctly identified the core problems of index out-of-bounds and undefined swap function, but differed in their depth of explanation and solution approaches.
Budget Tier
Models showed varying levels of detail and accuracy analyzing this sorting code bug. ChatGPT and Llama provided the most comprehensive analysis with working code examples. Gemini focused mainly on the index out-of-bounds issue but included good context. Claude gave a clear, structured breakdown of multiple potential issues. Response times ranged from 980ms (Llama) to 8447ms (ChatGPT). While all models identified the critical index error, Llama's quick, thorough response with implementable code made it the strongest performer for this specific code review task.
Division Error Handling Analysis
Detects missing error handling for division operations, particularly zero division cases. Helpful for improving function robustness.
Prompt:
What are the bugs in this function? def divide(a,b): return a/b
Performance Results
The function divide(a, b)
you provided is a basic implementation for dividing two numbers. While it generally works for most cases, there are some potential issues and improvements that could be made:
Division by Zero: The current implementation does not handle the situation where
b
is zero. Division by zero is undefined in mathematics and will raise aZeroDivisionError
in Python. You should add a check to handle this case appropriately.Type Checking: The function assumes that both
a
andb
are numbers. However, if a user passes in non-numeric types (such as strings or other objects), it will raise aTypeError
. Consider adding checks to ensure that both parameters are numbers.
Here's how you can enhance the function to handle these cases:
def divide(a, b):
# Check if the inputs are numeric
if not isinstance(a, (int, float)) or not isinstance(b, (int, float)):
raise TypeError("Both arguments must be numbers")
# Check for division by zero
if b == 0:
raise ValueError("The divisor 'b' cannot be zero")
return a / b
This version of the function includes checks for input types and division by zero, raising appropriate exceptions when the inputs are invalid.
Here are the potential issues in the divide
function:
Main Issues:
- No Division by Zero Check
- The function doesn't handle when
b = 0
- Will raise
ZeroDivisionError
- No Type Checking
- No validation if inputs are numbers
- Could raise
TypeError
with invalid inputs
Example of Improved Version:
def divide(a, b):
# Type checking
if not isinstance(a, (int, float)) or not isinstance(b, (int, float)):
raise TypeError("Both inputs must be numbers")
# Division by zero check
if b == 0:
raise ValueError("Cannot divide by zero")
return a / b
Additional Considerations:
- You might want to add docstring for documentation
- Consider handling special cases (infinity, NaN)
- Decide if you want to return float or handle integer division differently
The primary bug in the divide(a, b)
function is the lack of handling for a potential ZeroDivisionError
. If b
is zero, the function will crash.
Here's a breakdown of the issue and how to fix it:
The Problem: ZeroDivisionError
If the argument b
is equal to 0, the /
operator will raise a ZeroDivisionError
. This will halt the program's execution and display an error message.
Solutions:
- Raising the Exception: The simplest solution is to explicitly allow the
ZeroDivisionError
to be raised. This is appropriate if you want the calling code to handle the error.
def divide(a, b):
return a / b # Let ZeroDivisionError propagate
- Returning a Special Value (e.g., None, Infinity, or NaN): You might return a special value to indicate division by zero. This approach requires the calling code to check for this value.
def divide(a, b):
if b == 0:
return None # Or float('inf') or float('nan')
return a / b
- Returning a Default Value (e.g., 0): This is less common but might be suitable for some specific applications. Be very cautious, as it can mask errors and lead to incorrect results downstream.
def divide(a, b):
if b == 0:
return 0 # Use with extreme care!
return a / b
- Logging and Returning a Default: Combine a default return with logging to record the error.
import logging
logging.basicConfig(level=logging.WARNING) # Configure logging
def divide(a, b):
if b == 0:
logging.warning("Division by zero attempted!")
return 0 # Or None, or handle differently
return a / b
- Raising a Custom Exception: This provides the most control and allows you to define a specific exception type for division by zero.
class DivisionByZeroError(Exception):
pass
def divide(a, b):
if b == 0:
raise DivisionByZeroError("Division by zero!")
return a / b
Example of Handling the Exception:
try:
result = divide(10, 0)
except ZeroDivisionError:
print("Cannot divide by zero!")
else:
print(f"Result: {result}")
The best solution depends on the specific requirements of your program. Raising the exception or returning None
are generally preferred for their clarity and ability to prevent unintended consequences. Using a default value like 0 should be used with great caution. Logging is always a good practice when dealing with potential errors.
Bugs in the Function
The provided function divide(a, b)
has a few potential bugs and areas for improvement:
1. Division by Zero Error
The function does not handle the case where b
is zero. In Python, attempting to divide by zero raises a ZeroDivisionError
. To fix this, you can add a simple check:
def divide(a, b):
if b == 0:
raise ValueError("Cannot divide by zero")
return a / b
2. Type Errors
The function assumes that both a
and b
are numbers. However, if either a
or b
is not a number (e.g., a string, list, etc.), the function will raise a TypeError
. To avoid this, you can add type checking:
def divide(a, b):
if not isinstance(a, (int, float)) or not isinstance(b, (int, float)):
raise TypeError("Both arguments must be numbers")
if b == 0:
raise ValueError("Cannot divide by zero")
return a / b
3. Lack of Documentation
The function lacks a docstring, which provides documentation for the function's purpose, parameters, and return values. Adding a docstring is good practice for readability and understanding:
def divide(a, b):
"""
Divide two numbers.
Args:
a (int or float): The dividend.
b (int or float): The divisor.
Returns:
int or float: The quotient of a and b.
Raises:
ValueError: If b is zero.
TypeError: If a or b is not a number.
"""
if not isinstance(a, (int, float)) or not isinstance(b, (int, float)):
raise TypeError("Both arguments must be numbers")
if b == 0:
raise ValueError("Cannot divide by zero")
return a / b
Example Usage
try:
result = divide(10, 2)
print(f"10 divided by 2 is {result}")
except ValueError as e:
print(f"Error: {e}")
except TypeError as e:
print(f"Error: {e}")
# Output: 10 divided by 2 is 5.0
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
The function you've provided, divide(a, b)
, attempts to perform division of a
by b
. However, there are several potential issues (or bugs) that could arise when using this function:
- Division by Zero: If
b
is zero, this will raise aZeroDivisionError
, which is a common issue in division functions.
divide(5, 0) # This will raise ZeroDivisionError
- Type Errors: The function does not validate the types of
a
andb
. If eithera
orb
is not a number (e.g., a string), this will raise aTypeError
.
divide(5, 'a') # This will raise TypeError
Handling of Non-Numeric Inputs: If the function is expected to handle non-numeric inputs gracefully, it currently does not provide any error handling for such cases.
Returning a Floating Point: The division in Python always returns a float in Python 3, even if both
a
andb
are integers. If you intended for integer division, you should use the//
operator instead.Additional Error Handling: Depending on the use case, you may want to include more robust error handling, such as catching exceptions and providing user-friendly error messages.
Here is a revised version of the function that addresses these issues:
def divide(a, b):
try:
# Check if inputs are numeric
if not isinstance(a, (int, float)) or not isinstance(b, (int, float)):
raise TypeError("Both a and b must be numbers.")
# Handle division by zero
if b == 0:
raise ZeroDivisionError("Division by zero is not allowed.")
return a / b
except (TypeError, ZeroDivisionError) as e:
print(f"Error: {e}")
This revised function will now raise appropriate errors for both type issues and division by zero, providing helpful error messages to the user.
The primary bug in the divide(a, b)
function is its lack of error handling for division by zero. If b
is 0, the function will raise a ZeroDivisionError
.
Here's a breakdown of the issue and how to fix it:
- ZeroDivisionError: Attempting to divide any number by zero results in this exception. The function doesn't gracefully handle this scenario.
Here's an improved version of the function that addresses this:
def divide(a, b):
if b == 0:
return "Division by zero is not allowed" # Or raise a specific exception
else:
return a / b
This improved version checks if b
is zero before performing the division. If it is, it returns an appropriate message. You could also choose to raise a more specific exception like ZeroDivisionError
using raise ZeroDivisionError("Division by zero")
instead of returning a string, if that better fits your error handling strategy.
Another potential, less critical issue, is that the function doesn't explicitly handle the case where the inputs a
and b
might not be numbers (e.g., strings). If you're expecting only numbers, you could add type checking or use a try-except
block to handle potential TypeError
exceptions. However, this depends on your intended use case.
Here are the potential bugs in the provided divide
function:
- Division by Zero:
def divide(a,b):
return a/b
If b
is zero, this will raise a ZeroDivisionError
, which will cause the program to crash.
- No Type Checking:
- The function doesn't validate input types
- If non-numeric types are passed, it may raise a
TypeError
- Example problematic inputs:
python divide("10", 2) # Might raise TypeError divide([10], 2) # Might raise TypeError
- Potential Floating Point Precision Issues:
- Division can result in floating-point imprecision
- Example:
python print(divide(1, 3)) # 0.3333333333333333
Recommended improvements:
def divide(a, b):
if b == 0:
raise ValueError("Cannot divide by zero")
if not (isinstance(a, (int, float)) and isinstance(b, (int, float))):
raise TypeError("Inputs must be numeric")
return a / b
This improved version:
- Prevents division by zero
- Ensures type safety
- Provides clear error messages
The function divide(a, b)
has several potential bugs:
Division by Zero Error: The function does not handle the case when the divisor
b
is zero. This will raise aZeroDivisionError
in Python. To fix this, you can add a simple check to return an error message or raise a custom exception.Missing Input Validation: The function does not validate the inputs
a
andb
. They could beNone
, floating point numbers, or even complex numbers. You may want to add input validation to ensure thata
andb
are valid numbers.Loss of Precision: The function performs floating-point division, which may lead to loss of precision. If you need exact division, you may want to use a library like
Decimal
.No Handling for Non-Number Inputs: The function does not handle non-numeric inputs. You may want to add a check to raise an error or return an error message if
a
orb
is not a number.Lack of Documentation: The function does not have any documentation or comments. It's a good practice to document functions to explain what they do, what inputs they accept, and what outputs they return.
Here's an updated version of the function with some bug fixes and improvements:
def divide(a, b):
"""
Perform division operation on two numbers.
Args:
a (float): The dividend.
b (float): The divisor.
Returns:
float: The result of the division operation.
Raises:
ValueError: If the divisor is zero.
TypeError: If the inputs are not numbers.
"""
if not isinstance(a, (int, float)) or not isinstance(b, (int, float)):
raise TypeError("Both inputs must be numbers")
if b == 0:
raise ValueError("Cannot divide by zero")
return a / b
This version of the function includes:
- Input validation to check if both inputs are numbers.
- A check to raise a
ValueError
if the divisor is zero. - Documentation to explain what the function does and how to use it.
- Improved code organization and readability.
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
All models correctly identified the main issues: division by zero and lack of type checking. ChatGPT and Claude provided clear, concise analyses with practical code solutions. Gemini offered the most comprehensive response but was arguably too verbose for the task. Llama's response was well-structured but included unnecessary documentation suggestions.
Response times varied significantly: Llama was fastest (3.2s), followed by ChatGPT (5.2s), Claude (7.0s), and Gemini (14.1s). While all models provided accurate technical insights, ChatGPT and Claude achieved the best balance of conciseness and completeness. Gemini's thoroughness came at the cost of speed and brevity, while Llama was efficient but included superfluous information.
Budget Tier
Each model identified the key issues with the divide function, particularly division by zero and type validation, but with varying levels of detail and approach. ChatGPT 4o Mini provided the most comprehensive analysis with detailed examples and a robust solution. Claude and Gemini delivered concise yet thorough analyses, with Claude offering a more structured code solution. Llama 3.1 8B, despite being the fastest (894ms), included unique insights about precision loss and documentation needs. Notably, ChatGPT took longest (7433ms) but provided the most extensive code examples and explanations. Overall, ChatGPT and Claude demonstrated superior technical accuracy and practical solutions.
Logic Flow Error Detection
Identifies incorrect logical assumptions and misleading output messages in control flow statements. Useful for debugging logical flow issues.
Prompt:
Find the logical error in this code: sum = 0 for i in range(10): sum += i if sum > 50: break print('Sum is less than 50')
Performance Results
The logical error in the code is related to the condition checked after the loop and the loop's behavior:
- Condition Mismatch: The code checks
if sum > 50:
within the loop to break out of it, but after the loop, it prints'Sum is less than 50'
unconditionally. This message is misleading because the loop might break becausesum
exceeded 50, yet the message still claims 'Sum is less than 50'.
To correct this logical error, you need to conditionally print the statement based on whether sum
is actually less than or equal to 50 after the loop execution. You could do something like this:
sum = 0
for i in range(10):
sum += i
if sum > 50:
break
if sum <= 50:
print('Sum is less than or equal to 50')
else:
print('Sum exceeded 50')
This way, the message accurately reflects the condition of the sum after the loop execution.
The logical error in this code is:
The print statement `print('Sum is less than 50')` will execute regardless of whether the sum is less than 50 or not. This is because it is outside of the loop and not within a conditional block.
For example:
- If we sum 0+1+2+3+4+5+6+7+8+9, the sum would be 45 (less than 50)
- The message prints "Sum is less than 50" which is correct in this case
- But if we changed the range to `range(20)`, the sum would exceed 50
- The message would still print "Sum is less than 50" even though it's false
To fix this, we should either:
1. Move the print inside the loop:
python sum = 0 for i in range(10): sum += i if sum > 50: break print('Sum is less than 50')
2. Or use an else clause to check the condition:
python sum = 0 if sum <= 50: for i in range(10): sum += i if sum > 50: break if sum <= 50: print('Sum is less than 50') ```
The logical error is that the code always prints "Sum is less than 50", even when the sum is greater than 50. The break
statement exits the loop when the sum exceeds 50, but it doesn't change the fact that the print
statement is unconditionally executed afterward.
Here's a corrected version:
sum = 0
for i in range(10):
sum += i
if sum > 50:
print('Sum exceeded 50')
break
else: # This else block is associated with the for loop, not the if
print('Sum is less than or equal to 50')
This version uses the else
clause of the for
loop. The else
block is executed only if the loop completes normally (i.e., without hitting a break
statement). This ensures the correct message is printed based on whether the sum exceeded 50 or not. Another correction is ensuring the final message correctly reflects the condition (<= 50
).
Logical Error
The code has a logical error in the printing statement. When the sum exceeds 50, the loop breaks, but the code still prints 'Sum is less than 50', which is incorrect.
Corrected Code
sum_value = 0
for i in range(10):
sum_value += i
if sum_value > 50:
break
print('Sum is less than 50' if sum_value <= 50 else 'Sum exceeded 50')
Explanation
In the original code, the print statement is outside the loop and always prints the same message. To fix this, we need to check the value of sum_value
after the loop. If it's less than or equal to 50, print 'Sum is less than 50'. Otherwise, print 'Sum exceeded 50'.
Note that I've also renamed the variable sum
to sum_value
to avoid using a built-in Python function name as a variable name.
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
The logical error in the code lies in the message printed at the end. The code is attempting to evaluate whether the sum of numbers from 0 to 9 exceeds 50 and if it does, it breaks the loop. However, regardless of whether the loop breaks or completes, the message "Sum is less than 50" is always printed, even when the sum could be greater than 50.
Here's the corrected version of the code to properly reflect the condition of the sum:
sum = 0
for i in range(10):
sum += i
if sum > 50:
break
if sum < 50:
print('Sum is less than 50')
else:
print('Sum is 50 or more')
In this corrected version, we check the value of sum
after the loop and print the appropriate message based on whether it's less than 50 or not.
The logical error lies in the print statement: print('Sum is less than 50')
.
The code always prints "Sum is less than 50", regardless of whether the sum actually exceeds 50 or not. The break
statement only exits the loop; it doesn't change the fact that the program then unconditionally proceeds to the print
statement.
To correct this, you'd need to conditionally print the message based on whether the sum exceeded 50:
sum = 0
for i in range(10):
sum += i
if sum > 50:
break
if sum > 50:
print('Sum exceeds 50')
else:
print('Sum is less than or equal to 50')
This revised code accurately reflects the final value of sum
.
Here's the analysis of the logical error in the code:
Logical Error Analysis
The logical error in this code is in the print
statement at the end. The statement print('Sum is less than 50')
will always be executed, regardless of whether the sum actually exceeds 50 or not.
Correct Reasoning
- If the sum becomes greater than 50 inside the loop, the
break
statement will exit the loop - However, the print statement after the loop will still run
- This means the message will be printed even when the sum is actually greater than 50
Corrected Version
sum = 0
for i in range(10):
sum += i
if sum > 50:
print('Sum is greater than 50')
break
else:
print('Sum is less than 50')
Alternative Fix
sum = 0
for i in range(10):
sum += i
if sum > 50:
print('Sum is greater than 50')
break
if sum <= 50:
print('Sum is less than 50')
The corrected versions ensure that the print statement accurately reflects the actual state of the sum.
The logical error in this code is that it prints 'Sum is less than 50' when the sum is actually 45, but it could be greater than 50 if the loop hadn't broken.
When the loop breaks, the sum is indeed greater than 50, but the condition to break the loop is based on the current sum, which in this case is 20 + 25 = 45
. However, the next iteration (i = 9
) would make the sum greater than 50.
To fix this logical error, you can modify the condition in the print statement to reflect that the sum is not greater than 50 at the time of breaking. Here's how you can do it:
sum = 0
for i in range(10):
sum += i
if sum > 50:
print(f'Loop broken when sum was {sum}')
break
else:
print('Sum is less than 50')
In this modified code, the else
block is executed when the loop completes normally (i.e., not breaking due to the condition). This way, the message will only be printed when the sum is less than 50 when the loop ends.
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
All models correctly identified the core logical error - the unconditional print statement that executes regardless of the sum's value. ChatGPT 4o and Gemini provided clear, concise explanations with well-structured solutions. Claude offered the most detailed analysis with specific examples, though perhaps overly verbose. Llama was notably fast (1640ms) and included the additional best practice of renaming the 'sum' variable. Gemini's solution using the for-else construct was particularly elegant. ChatGPT had the best balance of explanation and solution implementation.
Budget Tier
All models correctly identified the main logical error - the unconditional print statement that executes regardless of the sum's value. ChatGPT and Gemini provided similar solutions using if-else statements. Claude offered two alternative fixes, including using Python's for-else construct. Llama's analysis was less focused, providing unnecessary details about specific sum values.
Response times varied significantly: Llama was fastest at 676ms, while Gemini was slowest at 5813ms. ChatGPT and Claude fell in between at 3148ms and 4461ms respectively. All solutions were technically correct, but Claude's explanation was most comprehensive and structured.
Infinite Loop Risk Assessment
Analyzes code for infinite loop risks and missing exit conditions. Important for preventing program hanging issues.
Prompt:
Identify potential issues in this code: while True: data = input() process_data(data)
Performance Results
There are several potential issues with the provided code snippet:
- Infinite Loop:
- The
while True
loop will continue indefinitely, potentially causing the program to run forever unless there is a break condition or an exception. This could lead to unresponsiveness or resource exhaustion if not managed properly.
- No Exit Condition:
- There is no mechanism to exit the loop. Implement a condition to break out of the loop, perhaps by checking for a specific input (e.g., "exit" or "quit").
- Unspecified Function:
- The function
process_data(data)
is undefined and should be properly implemented. Without this, the code will raise aNameError
.
- Handling Interrupt Signals:
- Consider adding exception handling for signals like KeyboardInterrupt (e.g., Ctrl+C) to gracefully exit the program instead of abruptly stopping.
- Input Validation:
- The code does not include any input validation. Depending on how
process_data
is implemented, you should consider checking that the input data meets expected criteria before processing it to avoid errors.
- Resource Management:
- Continuously taking input without any constraint or intelligent handling may cause issues with system resources over an extended period, especially in systems with limited memory or storage.
- Error Handling:
- There is no error handling around the input and processing functions. Implement try-except blocks to catch potential exceptions that may arise during input reading or data processing.
Here's a revised version of the code addressing some of these issues:
def process_data(data):
# Implement your data processing here
pass
try:
while True:
data = input("Enter data (or type 'exit' to quit): ")
if data.lower() == "exit":
print("Exiting program.")
break
process_data(data)
except KeyboardInterrupt:
print("\nProgram interrupted by user. Exiting...")
except Exception as e:
print(f"An error occurred: {e}")
This version implements a check to exit the loop and includes basic user guidance and exception handling.
Key Issues:
- No Exit Condition
- The while loop runs indefinitely with no way to break out
- Could lead to resource exhaustion or program lockup
- Should include a break condition or exit command
- No Error Handling
input()
could raise EOFError or KeyboardInterruptprocess_data()
may throw exceptions- Missing try/catch blocks for graceful error handling
- No Input Validation
- Data is processed without checking if it's valid
- Could cause issues if invalid/malformed data is received
- Resource Management
- Continuous input reading without limits
- No buffer management or size checks
- Could lead to memory issues with large inputs
Improved Version:
def handle_input():
try:
while True:
data = input("Enter data (or 'quit' to exit): ")
if data.lower() == 'quit':
break
if validate_data(data):
process_data(data)
else:
print("Invalid data format")
except (EOFError, KeyboardInterrupt):
print("\nProgram terminated")
This code has several potential issues:
Infinite Loop with No Exit Condition: The
while True:
creates an infinite loop. The loop will never terminate unless an error occurs or the program is forcefully stopped (e.g., Ctrl+C). There's no way for the user to gracefully exit.No Error Handling: If
process_data(data)
raises an exception (e.g., due to invalid input, a file not being found, a network error, etc.), the program will crash. There's notry...except
block to catch and handle potential errors.Blocking Input: The
input()
function blocks execution until the user provides input. This means the program cannot respond to other events or signals while waiting for input. In a more complex application, this could lead to unresponsiveness.Unclear Purpose of
process_data()
: We don't know whatprocess_data()
does. If it has side effects (like writing to a file or modifying global variables), the infinite loop could lead to unintended consequences, such as filling up disk space or corrupting data.Resource Consumption (Potential): Depending on what
process_data()
does, the continuous loop could lead to excessive resource consumption (CPU, memory) if it doesn't have a way to yield control or manage its resources effectively within the loop.
Here's an example of how to improve the code:
import sys
def process_data(data):
try:
# ... do something with data ...
if data.lower() == "exit": # Example exit condition
return False # Signal to stop the loop
return True # Signal to continue the loop
except Exception as e:
print(f"Error processing data: {e}")
return True # or False depending on desired behavior after error
while True:
try:
data = input("Enter data (or 'exit' to quit): ")
except KeyboardInterrupt: # Allow Ctrl+C to exit
print("\nExiting...")
sys.exit(0)
if not process_data(data):
break # Exit the loop gracefully
Key improvements:
- Exit Condition: The loop now terminates when the user enters "exit".
- Error Handling: A
try...except
block handles potential exceptions withinprocess_data()
. - Keyboard Interrupt Handling: The code now handles
KeyboardInterrupt
(Ctrl+C) to allow the user to interrupt the program. - Clearer Control Flow: The
process_data()
function returns a boolean indicating whether to continue the loop or not. - Prompts for Input: The
input()
function now includes a prompt, making it clearer to the user what's expected.
This revised code is more robust and user-friendly. You should adapt the exit condition and error handling to the specific requirements of your application.
Potential Issues in the Code
1. Infinite Loop
The while True
loop will run indefinitely until the program is manually stopped. This may not be the desired behavior if the program is supposed to terminate after processing a certain amount of data or if it is to be used in a production environment.
2. Lack of Error Handling
The code does not handle any potential errors that may occur during the execution of the process_data
function. If an exception occurs, the program will terminate abruptly, which may lead to data loss or other issues.
3. No Input Validation
The code does not validate the user input in any way. If the user enters invalid or malformed data, it may cause the process_data
function to fail or produce unexpected results.
4. Missing Function Definition
The process_data
function is called in the code, but its definition is not provided. This may indicate a lack of understanding of the function's purpose or behavior.
5. Resource Management
If the process_data
function opens files, sockets, or other system resources, the code does not ensure that these resources are properly closed after use.
6. Code Organization
The code mixes data input and processing in the same loop, which may make the code harder to maintain and understand. A better approach might be to separate the data input from the processing logic.
7. No Termination Condition
The loop will continue indefinitely until manually stopped. There is no clear condition for when the loop should terminate.
Improved Code Version
def get_user_input():
"""Get user input."""
data = input()
return data
def process_data(data):
"""Process user input data."""
# Add your processing logic here
# For example:
return data.upper()
def main():
"""Main function."""
max_iterations = 10 # Add a termination condition
for _ in range(max_iterations):
try:
data = get_user_input()
processed_data = process_data(data)
print("Processed data:", processed_data)
except Exception as e:
print("An error occurred:", str(e))
else:
print("Data processed successfully.")
if __name__ == "__main__":
main()
In this improved version, we've added a termination condition to the loop, separated the input and processing logic into different functions, and added error handling to ensure the program remains stable even in case of unexpected errors.
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
The provided code snippet has a few potential issues:
Infinite Loop: The
while True
statement creates an infinite loop. If there is no exit condition or break, the loop will run indefinitely, which may lead to unresponsiveness or excessive resource usage, especially if the program is waiting for user input.No Input Handling: The code currently does not handle valid or invalid input. If the user inputs something that causes
process_data(data)
to fail (for example, ifdata
is in an unexpected format), it could raise an exception and terminate the program unexpectedly.Blocking on Input: The
input()
function is blocking, meaning that if the program is waiting for user input, it cannot perform other tasks until input is received, which could be problematic in applications where concurrent processing is needed.Lack of Input Validation: There is no validation of the input data before it's processed. This could lead to unintended behavior or errors if
data
doesn't meet the expected format or criteria forprocess_data()
.No Exit Mechanism: Since the loop is infinite, users have no straightforward way to exit the loop gracefully. This can be frustrating and could require a forced termination, which is not user-friendly.
Resource Management: If
process_data()
consumes significant resources or if the loop iterates too quickly, it could lead to performance issues or even a crash, especially if running for long periods.Error Handling: There is no error handling for
process_data(data)
. If this function raises an exception, the program will terminate without providing meaningful feedback to the user or the possibility to handle the error gracefully.
To improve this code, consider the following modifications:
- Add a specific exit condition to the loop (e.g., exit when the user inputs "exit" or a specific command).
- Implement input validation and error handling for both user input and within the
process_data()
function. - Possibly use asynchronous input handling or threading if you need to maintain responsiveness in an environment where more things may be happening simultaneously.
- Ensure proper feedback and user guidance regarding what input is expected and how to exit the loop gracefully.
Here’s an example of what a revised version might look like:
while True:
try:
data = input("Enter data (or 'exit' to quit): ")
if data.lower() == 'exit':
break
process_data(data)
except Exception as e:
print(f"An error occurred: {e}")
This adds an exit condition, some basic error handling, and a prompt to inform the user about how to exit.
This code snippet has several potential issues:
Infinite Loop: The
while True:
loop will run indefinitely unless explicitly broken. There's no condition to stop the loop, leading to a program that never terminates. This is the most significant problem.Lack of Error Handling: The
input()
function can raise exceptions (e.g.,KeyboardInterrupt
if the user interrupts the program with Ctrl+C). Theprocess_data
function might also raise exceptions (e.g.,ValueError
,TypeError
). These exceptions are unhandled, which can lead to program crashes.Resource Exhaustion: Depending on what
process_data
does, the program might consume excessive memory or other resources if it runs indefinitely. Ifprocess_data
stores the input data without releasing it, this could lead to a memory leak.No Input Validation: The code accepts any input from the user without any validation. This could lead to vulnerabilities if the
process_data
function isn't designed to handle arbitrary or malicious input.Ambiguous
process_data
: The code doesn't show the implementation ofprocess_data
. The potential issues within that function are unknown and could significantly impact the overall reliability and security. For example, it might have its own infinite loops, memory leaks, or handle input incorrectly.Lack of User Feedback: The program doesn't provide any feedback to the user about what's happening. It silently processes input, making it difficult to understand its status or identify potential problems.
To improve the code, you need to:
- Add a loop termination condition: This could be based on user input (e.g., entering a specific command like "exit"), a timer, or a counter.
- Implement error handling: Use
try...except
blocks to catch and handle potential exceptions. - Validate user input: Check the input for validity and reject invalid data.
- Manage resources: Ensure that resources (like memory) are released appropriately.
- Provide user feedback: Give the user clear indications of the program's progress and status.
A better version might look like this (assuming you want to exit on "quit"):
while True:
try:
data = input("Enter data (or 'quit' to exit): ")
if data.lower() == 'quit':
break
process_data(data)
except (KeyboardInterrupt, EOFError):
print("\nProgram interrupted.")
break
except Exception as e:
print(f"An error occurred: {e}")
This improved version addresses most of the identified issues, but the specifics will depend on the implementation of process_data
. Robust error handling and resource management within process_data
are still crucial.
Here are potential issues with the provided code:
Infinite Loop Problems
- Creates an unbounded, infinite loop that will continue running indefinitely
- No clear exit mechanism or break condition
- Potential resource consumption and performance concerns
Input/Handling Weaknesses
- No input validation or error handling
- Will crash if
process_data()
raises an exception - No handling of EOF (End of File) or keyboard interrupts
Recommended Improvements
def main():
try:
while True:
try:
data = input()
if data.lower() in ['quit', 'exit', 'q']:
break
process_data(data)
except EOFError:
break
except ValueError as e:
print(f"Invalid input: {e}")
except KeyboardInterrupt:
print("\nProgram terminated by user.")
finally:
# Cleanup code if needed
pass
if __name__ == "__main__":
main()
Key Enhancements
- Added exit conditions
- Error handling
- Keyboard interrupt management
- Proper program structure
- Input validation options
Potential Additional Considerations
- Input length limits
- Type checking
- Specific error handling based on expected input types
Potential Issues in the Code
The provided code uses a while loop to continuously ask the user for input and process it. However, there are a few potential issues:
Infinite Loop with No Exit Condition: The code has an infinite loop (
while True
) but there is no mechanism to exit the loop. Once the program is running, it will keep asking for input until it's manually stopped.Crash on Bad Input: If the user enters something that's not a string, such as an integer, float, or None, the
input()
function will raise aTypeError
. If this happens, the program will crash.No Error Handling: The code does not handle any potential exceptions that might occur during the execution of the
process_data(data)
function. If this function encounters an error, the program will crash and the error will not be reported.Lack of Input Validation: The
process_data(data)
function is called with the user's input directly. However, this function might have specific requirements for the input data, such as a certain format or content. If the input does not match these requirements, the function might fail unexpectedly.No Input Validation for Invalid Exit: If the user enters a specific string to exit the program, the code might not check for it and keep asking for input.
Recommendations
Add a mechanism to exit the loop: You can add an option for the user to enter a specific string or specific keyword to exit the loop.
Implement error handling: You should write try-except blocks around the code that performs input and data processing. This way, the program can handle potential errors and report them correctly.
Validate user input: Before passing user input to the
process_data(data)
function, you should check if it matches the required format or contains any invalid characters.
Here's an example of how you could modify the code:
def process_data(data):
# Implement your data processing logic here
print(f"Processing data: {data}")
return
def main():
while True:
user_input = input("Enter data (or 'quit' to exit): ")
if user_input.lower() == 'quit':
break
try:
process_data(user_input)
except Exception as e:
print(f"Error processing data: {e}")
if __name__ == "__main__":
main()
In the modified code, we've:
- Added a
main()
function to contain the loop. - Included a check for 'quit' to exit the loop.
- Wrapped the processing code in a try-except block to catch any potential errors.
- Validated user input before passing it to the
process_data(data)
function for processing.
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
The models showed varying approaches to analyzing the code issues. ChatGPT 4o provided the most comprehensive analysis with 7 detailed points and a well-structured solution, though it took moderate time (7.8s). Claude 3.5 Sonnet was notably efficient (5.3s) with a concise but thorough analysis focusing on 4 key issues. Gemini 1.5 Pro gave extensive explanations and detailed improvements but was slowest (13.7s). Llama 3.1 70B was fastest (2.4s) and offered a unique academic-style analysis with clear headings. All models identified the core issues (infinite loop, error handling, input validation) but differed in depth and presentation style.
Budget Tier
The models showed varying levels of detail and focus in analyzing the code issues. ChatGPT 4o Mini and Gemini 1.5 Flash provided the most comprehensive analyses, identifying 7-8 distinct issues and offering detailed solutions with example code. Claude 3.5 Haiku was more concise but still captured the key problems and provided structured recommendations. Llama 3.1 8B offered a balanced analysis focusing on 5 core issues with clear explanations and practical code examples. While all models identified the main concerns (infinite loop, error handling, input validation), the larger models provided more nuanced security and resource management considerations.
Password Validation Security Review
Evaluates password validation logic for security weaknesses and missing complexity requirements. Essential for security-critical applications.
Prompt:
What's wrong with this password validation? def validate_password(pwd): if len(pwd) >= 8: return True return False
Performance Results
The provided validate_password
function performs only a minimal check to validate a password: it checks if the password is at least 8 characters long. While this is a basic requirement for password strength, it is not sufficient for robust password validation. Here are some common improvements that could be made to enhance password validation:
Include Uppercase Letters: Check for at least one uppercase letter to ensure complexity.
Include Lowercase Letters: Ensure the password also contains at least one lowercase letter.
Include Digits: Verify the password contains at least one digit for numerical complexity.
Include Special Characters: Require at least one special character (e.g.,
!
,@
,#
, etc.) for additional security.Prevent Common Passwords: Use lists of compromised or commonly used passwords to prevent weak choices.
Avoid Sequential or Repetitive Characters: Discourage using easily guessable sequences like
1234
oraaaa
.
Here is an improved version of the validate_password
function that incorporates these checks:
import re
def validate_password(pwd):
if len(pwd) < 8:
return False
if not re.search(r'[A-Z]', pwd):
return False
if not re.search(r'[a-z]', pwd):
return False
if not re.search(r'[0-9]', pwd):
return False
if not re.search(r'[\W_]', pwd): # \W matches any non-word character (special chars)
return False
return True
This function uses regular expressions to check for each type of character and returns False
if any of the conditions are not met, otherwise it returns True
.
Keep in mind that password strength policies can vary depending on the application, security requirements, and user base, so you may need to adjust the criteria accordingly.
Here are the key issues with this password validation function:
Security Issues
- Too Simple: Only checks password length, ignoring other critical security factors:
- No check for mixed case letters
- No check for numbers
- No check for special characters
- No check for common passwords
- Minimum Length Only:
- 8 characters is barely minimum by modern standards
- No maximum length check (could lead to buffer overflow in some systems)
Implementation Issues
- Can Be Simplified: The function can be written more concisely as:
def validate_password(pwd):
return len(pwd) >= 8
Better Implementation Example
Here's a more secure password validation:
def validate_password(pwd):
if len(pwd) < 8 or len(pwd) > 128: # Length check
return False
if not any(c.isupper() for c in pwd): # Uppercase check
return False
if not any(c.islower() for c in pwd): # Lowercase check
return False
if not any(c.isdigit() for c in pwd): # Digit check
return False
if not any(c in "!@#$%^&*()_+-=[]{}|;:,.<>?" for c in pwd): # Special char check
return False
return True
Additional Recommendations
- Add password entropy checks
- Check against commonly used passwords
- Implement rate limiting for validation attempts
- Consider using established password validation libraries
- Add password hashing before storage
The password validation function validate_password
is too simplistic and allows for weak passwords. While it checks for a minimum length of 8 characters, it doesn't enforce any other rules, making it vulnerable to brute-force attacks. Here's a breakdown of the issues:
- Missing Character Complexity: It doesn't check for a mix of uppercase and lowercase letters, numbers, and symbols. Passwords consisting entirely of lowercase letters (e.g., "password123") are easily guessed.
- No Check for Common Passwords: It doesn't compare the password against lists of commonly used or breached passwords. These lists are readily available and used by attackers.
- No Protection Against Dictionary Attacks: Related to the previous point, easily guessable words, even if they are 8 characters long, are weak. The function doesn't attempt to mitigate dictionary attacks.
- No Consideration for Sequential Characters or Patterns: Passwords like "12345678" or "qwertyuiop" are considered valid even though they are extremely weak.
- Potential for User Data Inclusion: While not directly a flaw in the provided code, a robust password validation system should also check against user data (username, email, etc.) to prevent easily guessed variations.
Here's an example of a more robust password validation function in Python:
import re
def validate_password(pwd, username=None, email=None):
if len(pwd) < 8:
return False
if username and pwd == username:
return False
if email and pwd == email:
return False
if not re.search(r"[A-Z]", pwd): # At least one uppercase
return False
if not re.search(r"[a-z]", pwd): # At least one lowercase
return False
if not re.search(r"[0-9]", pwd): # At least one number
return False
if not re.search(r"[!@#$%^&*()-_=+]", pwd): # At least one symbol
return False # At least one special character
# Consider adding a check against a common password list here. You could use a library
# like 'zxcvbn' for this.
return True
username = "testuser"
email = "testuser@example.com"
print(validate_password("TestPassword1!", username, email)) # True
print(validate_password("testpassword", username, email)) # False (no uppercase, no symbol)
print(validate_password("TestPassword", username, email)) # False (no number, no symbol)
print(validate_password(username, username, email)) # False (same as username)
Key improvements:
- Character Complexity: Requires at least one uppercase, one lowercase, one number, and one symbol.
- Comparison with User Data: Prevents the password from being identical to the username or email.
- Regular Expressions: Uses regular expressions for more concise and efficient pattern matching.
While this improved version is stronger, adding a check against a known common password list (using a library like zxcvbn
) would further enhance security. zxcvbn
also helps assess password strength more holistically, considering patterns and common substitutions.
Issues with the Password Validation
The provided password validation function is overly simplistic and does not adhere to common best practices for password security. Here are some of the issues:
1. Lack of Complexity Requirements
The function only checks for a minimum length of 8 characters, which is a good starting point. However, it does not require any specific character types, such as:
- Uppercase letters (A-Z)
- Lowercase letters (a-z)
- Numbers (0-9)
- Special characters (!, @, #, $, etc.)
Including these requirements can make the password more secure.
2. No Maximum Length Check
While it's generally good practice to allow long passwords, not checking for an excessively long password can lead to potential issues, such as overflow errors or errors when storing the password.
3. No Blacklist Check
Not checking against common weak passwords or passwords that have been compromised in data breaches can lead to insecure passwords being accepted.
Improved Password Validation
Here's an updated version of the password validation function that addresses these issues:
import re
def validate_password(pwd, min_length=8, max_length=128):
"""
Validate a password.
Args:
pwd (str): The password to validate.
min_length (int, optional): The minimum length of the password. Defaults to 8.
max_length (int, optional): The maximum length of the password. Defaults to 128.
Returns:
bool: True if the password is valid, False otherwise.
"""
# Check for minimum length
if len(pwd) < min_length:
return False
# Check for maximum length
if len(pwd) > max_length:
return False
# Check for required character types
if not re.search(r"[a-z]", pwd): # Lowercase letters
return False
if not re.search(r"[A-Z]", pwd): # Uppercase letters
return False
if not re.search(r"\d", pwd): # Numbers
return False
if not re.search(r"[^A-Za-z0-9]", pwd): # Special characters
return False
# Optional: Check against a blacklist of weak passwords
weak_passwords = ["password123", "qwerty", "letmein"] # Add more weak passwords as needed
if pwd.lower() in weak_passwords:
return False
return True
This updated function checks for:
- A minimum length of 8 characters (configurable)
- A maximum length of 128 characters (configurable)
- The presence of at least one:
- Lowercase letter
- Uppercase letter
- Number
- Special character
- (Optional) Whether the password is in a blacklist of weak passwords
Example use cases:
print(validate_password("weakpass")) # False
print(validate_password("StrongP@ssw0rd")) # True
print(validate_password("TooLongPasswordThatExceeds128CharactersLength")) # False
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
The provided password validation function validate_password
only checks if the password length is at least 8 characters. While this is a basic requirement for password strength, it is not sufficient for robust password validation. Here are some aspects that are generally recommended for a more comprehensive password validation:
Maximum Length: There may be a maximum length for passwords to prevent excessively long inputs.
Character Variety: The password should ideally contain a mix of:
- Uppercase letters
- Lowercase letters
- Digits
- Special characters (e.g., !@#$%^&*)
Common Patterns: Checks against common patterns or easily guessable passwords (like "password123" or "12345678").
No Repeated Characters: Avoid passwords that have repeated characters or sequences (e.g., "aa", "1234").
Prohibited Characters: Allowing only certain characters or disallowing certain characters may be required depending on the application.
Feedback on Failure: Instead of just returning
True
orFalse
, how the password fails could be communicated for user experience.
Here's an improved version of the function that incorporates some of these elements:
import re
def validate_password(pwd):
if len(pwd) < 8:
return False
# Check for at least one uppercase letter
if not re.search(r'[A-Z]', pwd):
return False
# Check for at least one lowercase letter
if not re.search(r'[a-z]', pwd):
return False
# Check for at least one digit
if not re.search(r'[0-9]', pwd):
return False
# Check for at least one special character
if not re.search(r'[!@#$%^&*(),.?":{}|<>]', pwd):
return False
# Check for prohibited patterns or repeated sequences if necessary
# (You can add more logic here if needed)
return True
This revised function checks for various criteria, making it significantly more robust against common weaknesses in password choice.
The primary problem with this password validation function is that it's far too simplistic. It only checks the length of the password. A password can be eight characters long but still be incredibly weak. Here's a breakdown of its shortcomings:
No complexity requirements: A strong password should include a mix of uppercase and lowercase letters, numbers, and symbols. This function doesn't check for any of that.
aaaaaaaa
passes validation, but it's easily cracked.No dictionary word check: Common words and names are easily guessable. The function doesn't check if the password is a dictionary word or a variation of one.
No reuse check: The function doesn't check if the password is reused from a known list of compromised passwords.
False sense of security: Users might believe their password is secure when it's not. This can lead to serious security vulnerabilities.
In short, while it ensures a minimum length, this function provides virtually no actual password security. A robust password validation system needs much more stringent criteria.
Here's an analysis of the password validation function:
Problems with the Current Implementation
Minimal Security: The function only checks the password length, which is insufficient for robust password security.
Lacks Critical Password Requirements:
- No check for complexity
- No requirement for:
- Uppercase letters
- Lowercase letters
- Numbers
- Special characters
Improved Password Validation Example
import re
def validate_password(pwd):
# Check length
if len(pwd) < 8:
return False
# Check for at least one uppercase letter
if not re.search(r'[A-Z]', pwd):
return False
# Check for at least one lowercase letter
if not re.search(r'[a-z]', pwd):
return False
# Check for at least one digit
if not re.search(r'\d', pwd):
return False
# Check for at least one special character
if not re.search(r'[!@#$%^&*(),.?":{}|<>]', pwd):
return False
return True
Key Improvements
- Checks minimum length
- Requires mixed character types
- Uses regex for comprehensive checks
- Returns
False
if any security criteria are not met
Example Usage
print(validate_password("weak")) # False
print(validate_password("Password123!")) # True
This approach provides a much more robust password validation mechanism.
The issue with this password validation is that it only checks the length of the password, which is a very basic security requirement. A good password validation should check for the following:
- Length: Minimum and maximum length (e.g., at least 8 characters, at most 32 characters)
- Complexity: Requiring a mix of character types, such as:
- Uppercase letters (A-Z)
- Lowercase letters (a-z)
- Numbers (0-9)
- Special characters (!, @, #, etc.)
- Format: Disallowing common formats, such as:
- Sequential characters (abc, 123, etc.)
- Common words or phrases (password, secret, etc.)
The given code only checks if the password length is at least 8 characters, which is not sufficient to ensure password strength.
Here's an improved version of the password validation function:
import re
def validate_password(pwd):
"""
Validate a password based on the following requirements:
- At least 8 characters long
- Contains at least one uppercase letter
- Contains at least one lowercase letter
- Contains at least one number
- Contains at least one special character
- Does not contain sequential characters (abc, 123, etc.)
"""
if len(pwd) < 8:
return False
# Check for uppercase, lowercase, and digit presence
if not re.search("[A-Z]", pwd) or not re.search("[a-z]", pwd) or not re.search("[0-9]", pwd):
return False
# Check for special characters
if not re.search("[!@#$%^&*()_+=-{};:'<>,./]", pwd):
return False
# Check for sequential characters
if re.search(r"(abc|123|456|789|abcdefgh|ijklmnop|jklmnop|mnopqrstuvwxyz)", pwd, re.IGNORECASE):
return False
return True
Note that this is still not foolproof, and you may want to add or modify rules based on your specific requirements.
With the improvement, the password validation function checks the following:
- At least 8 characters long
- Contains at least one uppercase letter
- Contains at least one lowercase letter
- Contains at least one number
- Contains at least one special character
- Does not contain common sequential characters
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
In analyzing the password validation prompt responses across models, ChatGPT 4 and Claude show strong code analysis skills, providing comprehensive yet concise explanations. Gemini 1.5 Pro offers the most detailed response but takes longest at 15.7s. Llama 3.1 70B impresses with the fastest response (2.8s) while maintaining good explanation quality and providing well-structured code. All models correctly identified the core issues and suggested similar improvements around character complexity, length requirements, and common password checks. Llama edges ahead in terms of efficiency and code documentation quality.
Budget Tier
The four models all correctly identified the core issue of insufficient password validation, but with varying levels of detail and implementation suggestions. ChatGPT 4o Mini provided comprehensive analysis with clear categorization and practical code examples. Gemini Flash offered clear explanation but no code solution. Claude Haiku gave a well-structured response with both analysis and improved code. Llama 3.1 8B provided the most detailed technical solution with extensive code comments and validation rules. Notably, Llama was significantly faster (968ms) compared to others (5000-7000ms range). For practical implementation guidance, Llama's response proved most valuable, while Gemini's explanation was most accessible for non-technical users.
Bubble Sort Implementation Analysis
Examines bubble sort implementation for boundary errors and incorrect loop conditions. Useful for algorithm implementation verification.
Prompt:
Find the bug in this sorting algorithm: def bubble_sort(arr): for i in range(len(arr)): for j in range(len(arr)): if arr[j] > arr[j+1]: arr[j], arr[j+1] = arr[j+1], arr[j]
Performance Results
The provided bubble_sort
function has a bug that causes an "index out of range" error. The problem lies in the inner loop where it's comparing arr[j]
with arr[j+1]
. Specifically, j
will eventually reach the last index of the array, and trying to access arr[j+1]
will cause an index error.
Here's the corrected version of the bubble sort algorithm with the necessary changes:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(n - 1 - i): # Limit j to n-1-i to avoid out of range
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
Changes made:
- The inner loop now iterates from
0
ton-1-i
instead of0
tolen(arr)
. This ensures thatarr[j+1]
is always a valid index. - The
n-1-i
limit also adds a minor optimization, reducing the number of comparisons as the end of the array is already sorted after each outer loop iteration.
There are two bugs in this bubble sort implementation:
Index Out of Range Error The inner loop will cause an
IndexError
whenj+1
exceeds the array length because it tries to accessarr[j+1]
whenj
is at the last element.Inefficient Comparison Range The inner loop should only go up to
len(arr)-1-i
because after each iteration, the largest element "bubbles up" to the end of the array.
Here's the corrected version:
def bubble_sort(arr):
for i in range(len(arr)):
for j in range(len(arr)-1-i): # Fixed range
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
The changes:
- Inner loop range changed from
range(len(arr))
torange(len(arr)-1-i)
- This prevents index out of range error and improves efficiency by not checking already sorted elements
The bug is an IndexError: list index out of range
.
In the inner loop, j
iterates up to len(arr) - 1
. When j
reaches its maximum value, arr[j+1]
tries to access an element beyond the end of the array.
Here's why:
len(arr)
returns the total number of elements.- Array indexing starts from 0, so the last valid index is
len(arr) - 1
. - When
j
islen(arr) - 1
,j+1
becomeslen(arr)
, which is out of bounds.
The fix:
Change the inner loop's range to stop one element earlier:
def bubble_sort(arr):
for i in range(len(arr)):
for j in range(len(arr) - 1 - i): # Corrected inner loop range
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
We subtract i
in the inner loop's range because after each pass of the outer loop, the last i
elements are already in their correct sorted positions. This optimization prevents unnecessary comparisons. Even without this optimization, fixing the IndexError
is crucial for the code to run at all.
The bug in this sorting algorithm lies in the inner loop and the way it accesses elements in the array.
Index Out of Range Error: In the inner loop,
j
ranges from0
tolen(arr) - 1
. However, whenj
is equal tolen(arr) - 1
, the linearr[j+1]
tries to access an index that is out of range, resulting in an "IndexError" because Python arrays are 0-indexed.Incorrect Inner Loop Range: The inner loop should only iterate until the second last element because we're comparing
arr[j]
andarr[j+1]
. This means the last iteration of the outer loop will compare the last two elements, so there's no need to compare the last element again in the next iteration.
Here's the corrected code:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(n - i - 1): # Corrected range for inner loop
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
Optimized Version: This version also includes a flag to detect if any swaps were made in the inner loop. If no swaps were made, the array is already sorted and the function can terminate early.
def bubble_sort(arr):
n = len(arr)
for i in range(n):
swapped = False
for j in range(n - i - 1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
swapped = True
if not swapped:
break # If no swaps were made, the array is already sorted
return arr
Example Usage:
arr = [64, 34, 25, 12, 22, 11, 90]
print("Original array:", arr)
print("Sorted array:", bubble_sort(arr))
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
The sorting algorithm you provided has a couple of issues that could lead to bugs:
Index Out of Range Error: When the inner loop accesses
arr[j + 1]
, it can lead to an "index out of range" error whenj
reaches the last valid index (len(arr) - 1
). In this case,j + 1
will refer to an index that is outside the bounds of the array.Improper Loop Bounds: The outer loop runs from
0
tolen(arr)
, which is an off-by-one error. The proper upper limit for the inner loop should belen(arr) - i - 1
, allowing the algorithm to exclude the sorted elements at the end of the list after each pass.
Here’s a corrected version of the bubble_sort
function:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(n - i - 1): # Fix the inner loop to avoid index out of range
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
With these changes, the algorithm will correctly sort the array without causing any runtime errors.
The bug is in the inner loop's range and the potential for an IndexError
.
The inner loop for j in range(len(arr))
iterates from 0 up to, but not including, len(arr)
. Inside the loop, it then accesses arr[j+1]
. When j
reaches len(arr) - 1
, j+1
becomes len(arr)
, which is an index out of bounds for the array, causing an IndexError
.
To fix this, the inner loop should iterate one element shorter to avoid going past the end of the array. Here's the corrected code:
def bubble_sort(arr):
for i in range(len(arr)):
for j in range(len(arr) - 1): # Iterate one element shorter
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
Even with this correction, the algorithm, while functional, is not optimally efficient. A further improvement would be to add a flag to check if any swaps were made in a pass. If no swaps occur, the array is already sorted, and the algorithm can terminate early.
There are a few critical bugs in this bubble sort implementation:
Index Out of Bounds Error: The inner loop
for j in range(len(arr))
will cause anIndexError
becausej+1
will eventually go beyond the last index of the array. This means whenj
is at the last index,arr[j+1]
will raise an index out of range exception.Inefficient Iteration: The outer loop should run
len(arr) - 1
times, notlen(arr)
times.Unnecessary Comparisons: The inner loop should not compare all elements in each pass, but only up to
len(arr) - i - 1
to avoid unnecessary comparisons.
Here's a corrected version:
def bubble_sort(arr):
n = len(arr)
for i in range(n - 1):
for j in range(n - i - 1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
Key fixes:
- Outer loop runs
n-1
times - Inner loop runs
n-i-1
times to avoid out-of-bounds error - Reduces unnecessary comparisons
- Added
return arr
for clarity (optional)
This corrected version will:
- Prevent index out of bounds errors
- Reduce unnecessary comparisons
- Correctly sort the array in ascending order
The bug in this sorting algorithm is that the range(len(arr))
in the outer loop is not necessary. The algorithm should only go through the array once for each pass, and the inner loop should start from the second element (j = 1) and go up to the second last element (len(arr) - 1).
Here's the corrected code:
def bubble_sort(arr):
for i in range(len(arr)):
for j in range(len(arr) - 1):
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
This is because after each pass, the largest element is bubbled to the end of the array, so there's no need to check the last element in the next pass.
Also, it's worth noting that bubble sort has a worst-case and average time complexity of O(n^2), making it less efficient than other sorting algorithms like quicksort, mergesort, or heapsort for large datasets. However, for small datasets or educational purposes, bubble sort can be a good choice due to its simplicity and ease of understanding.
Here's an example of how the algorithm would look with more comments and docstrings:
def bubble_sort(arr):
"""
Sorts an array of elements in ascending order using the bubble sort algorithm.
Args:
arr (list): The array to be sorted.
Returns:
list: The sorted array.
"""
# We don't need to go through the entire array each time, so the outer loop
# only goes up to the second last element
for i in range(len(arr) - 1):
# We need to go through the array from the first element to the second last element
# in each pass
for j in range(len(arr) - 1):
# If we find two elements that are out of order, swap them
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
# Return the sorted array
return arr
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
All models correctly identified the index out of range error in the bubble sort implementation. ChatGPT and Claude provided clear, concise explanations with corrected code. Gemini offered detailed technical reasoning behind the bug. Llama went beyond by suggesting an optimized version with early termination. Response times varied significantly - Llama was fastest at 2134ms, while Gemini was slowest at 6909ms. Claude balanced conciseness and clarity best, explaining two distinct bugs while maintaining brevity. Overall, each model demonstrated strong technical understanding, with slight variations in explanation depth and optimization suggestions.
Budget Tier
All models identified the main bug - an index out of range error in the inner loop. ChatGPT 4o Mini and Claude 3.5 Haiku provided the most comprehensive analysis, highlighting both the index error and inefficient iteration bounds. Gemini was concise but also suggested a useful optimization using a swap flag. Llama 3.1 8B's response, while detailed, missed identifying the critical index out of bounds error. Response times varied significantly, with Llama being fastest at 900ms and Claude slowest at 6051ms. Overall, ChatGPT 4o Mini provided the best balance of accuracy, clarity and solution quality.
Resource Management Review
Identifies potential resource leaks in file operations and memory management. Critical for maintaining application performance.
Prompt:
Analyze this code for memory leaks: file = open('data.txt', 'r') data = file.read()
Performance Results
The provided code snippet opens a file named data.txt
in read mode and reads its content into the variable data
. However, this code has a potential issue related to resource management, which can lead to a memory leak or other problems, such as exceeding the file descriptor limit.
Issues:
- File Not Closed:
- The file is being opened but not explicitly closed. When a file is opened, a file descriptor is allocated. If it is not closed, the file descriptor remains active, which could eventually lead to reaching the limit of open files, especially in long-running applications or if opening many files.
- Lack of Exception Handling:
- If an exception occurs while reading the file, the
file.close()
may never get executed, causing the file to remain open.
Recommendations for Improvement:
To ensure that the file is closed properly and to handle any potential exceptions, you can use a try-finally
block or, more preferably, a with
statement in Python, which automatically handles closing the file, even if an error occurs.
Using a with
statement:
with open('data.txt', 'r') as file:
data = file.read()
Advantages of Using with
statement:
- Automatic Resource Management: The
with
statement ensures that thefile
is closed as soon as the block inside it is exited, even if an exception is raised. - Cleaner Syntax: It is more concise and readable, reducing the risk of errors related to forgetting to close the file.
Using a with
statement is the recommended modern practice in Python as it helps prevent resource leaks and makes the code more robust and easier to maintain.
Potential Memory Leak Analysis:
This code has a memory leak because the file is never properly closed. Here are the issues:
- Missing file closure
- The file handle remains open
- System resources aren't properly released
- May lead to resource exhaustion if done repeatedly
How to fix:
Option 1 - Using close()
:
file = open('data.txt', 'r')
data = file.read()
file.close()
Option 2 - Using context manager (recommended):
with open('data.txt', 'r') as file:
data = file.read()
Benefits of fixing:
- Ensures proper resource cleanup
- Handles exceptions gracefully (with context manager)
- Follows Python best practices
- Prevents file descriptor leaks
The context manager (with
statement) is the preferred solution as it automatically handles file closure even if an exception occurs.
The code snippet you provided has a potential resource leak, specifically a file descriptor leak, not a memory leak in the traditional sense. While Python's garbage collector usually handles memory effectively, explicitly closing open files is essential.
Here's why:
Open Files Consume Resources: When you open a file using
open()
, the operating system allocates a file descriptor. This descriptor is a limited resource. If you open many files without closing them, you could eventually run out of available file descriptors, leading to errors.Unpredictable Behavior: Leaving files open can lead to unpredictable behavior, especially when writing. Data might not be flushed to disk immediately, or the file might remain locked, preventing other processes from accessing it.
Best Practice: It's crucial to close files as soon as you're finished with them. This releases the file descriptor back to the operating system.
How to Fix the Leak:
The most straightforward way to fix this is using the with
statement (context manager):
with open('data.txt', 'r') as file:
data = file.read()
# File is automatically closed outside the 'with' block
print(data)
The with
statement ensures that the file is closed automatically, even if exceptions occur within the block.
Alternatively, you can use a try...finally
block, but the with
statement is generally preferred for its conciseness and clarity:
file = open('data.txt', 'r')
try:
data = file.read()
finally:
file.close()
print(data)
In summary, while this isn't strictly a memory leak in the sense of allocated memory being lost, it's a resource leak that can have similar consequences. Always close files when you're done with them. The with
statement is the idiomatic and safest way to achieve this in Python.
Memory Leak Analysis
The provided code snippet reads the contents of a file named 'data.txt' into the data
variable. However, there is a potential memory leak issue with this code.
The Problem
The file file
is not explicitly closed after it is opened. In Python, if a file is not explicitly closed, it will remain open until it goes out of scope and is garbage collected. This can lead to a memory leak if the file is large or if the file is opened and closed many times in a row.
Best Practice
To avoid the memory leak issue, it's recommended to use a with
statement when opening files in Python. The with
statement automatically closes the file when the block of code within it is exited, regardless of whether an exception is thrown or not.
Improved Code
with open('data.txt', 'r') as file:
data = file.read()
By using a with
statement, we ensure that the file is properly closed after it is no longer needed, preventing potential memory leaks.
Alternative Solution
Alternatively, you can also use the close()
method to explicitly close the file. However, this approach is less recommended, as it can lead to errors if an exception is thrown before the file is closed.
file = open('data.txt', 'r')
try:
data = file.read()
finally:
file.close()
In this alternative solution, a try-finally
block is used to ensure that the file is closed, even if an exception is thrown. However, the with
statement is generally preferred for its readability and exception-handling benefits.
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
The code snippet provided opens a file (data.txt
) for reading and reads its contents into the variable data
.
Here's the code with some extra context for analysis:
file = open('data.txt', 'r')
data = file.read()
Memory Leak Analysis
Resource Management: The main concern in this code is not necessarily a memory leak in the traditional sense (such as leftover objects in memory), but rather improper management of system resources. In Python, the
open()
function allocates system resources (like file descriptors) that should be explicitly released when they are no longer needed.Closing the File: Since there is no
file.close()
statement in the code, the file may not be properly closed after it is read. This could lead to a resource leak if the program runs for an extended time and repeatedly opens files without closing them. In practice, while Python’s garbage collector can reclaim some of the memory, it might not close the file descriptor until the program terminates, which can be harmful to systems with limited resources.Using with Statement: A more Pythonic way to manage files is to use the
with
statement. This ensures that files are properly closed after their suite finishes, even if an error occurs:
with open('data.txt', 'r') as file:
data = file.read()
This code automatically closes the file once the block under with
is exited.
Conclusion
In summary, the initial code does not have a memory leak in the traditional sense, but it does potentially lead to resource leaks due to not closing the file. Always aim to close resources that are no longer needed or use context managers (with
statement) to ensure proper resource management in Python.
This code snippet has a potential memory leak. While it doesn't explicitly allocate memory in an uncontrolled way, it suffers from a resource leak.
The problem lies in the fact that the file object file
is created but never closed. The file.read()
method reads the entire contents of the file into the data
variable. However, the operating system still holds a file handle, and resources associated with that open file (like buffers) are not released until the file is explicitly closed using file.close()
.
While the memory used by data
will be reclaimed by Python's garbage collector once it goes out of scope, the operating system resources associated with the open file will remain tied up until the program terminates. In a long-running process or if this code is called repeatedly within a loop, this can lead to a significant accumulation of unclosed file handles and thus a form of resource leak. This isn't a memory leak in the purest sense (heap memory), but rather a file descriptor leak, which can still cause problems (e.g., reaching the system's limit on open files).
To fix this, you should explicitly close the file:
file = open('data.txt', 'r')
data = file.read()
file.close()
Even better, use a with
statement, which automatically handles closing the file even if exceptions occur:
with open('data.txt', 'r') as file:
data = file.read()
The with
statement ensures that the file.close()
method is called regardless of whether the code within the block executes successfully or raises an exception. This is the preferred and most robust way to handle file I/O in Python and prevents resource leaks.
Here's an analysis of the potential memory leak in the provided code:
Memory Leak Analysis
Potential Issue
file = open('data.txt', 'r')
data = file.read()
Problems:
- The file is opened but never explicitly closed
- If
data.txt
is large, the entire file contents are read into memory at once - The file handle remains open, which can lead to resource leaks
Recommended Solutions
Option 1: Use with
statement (Recommended)
with open('data.txt', 'r') as file:
data = file.read()
# File is automatically closed after this block
Option 2: Manually close the file
file = open('data.txt', 'r')
try:
data = file.read()
finally:
file.close()
Option 3: For large files, read in chunks
with open('data.txt', 'r') as file:
data = []
for chunk in iter(lambda: file.read(4096), ''):
data.append(chunk)
data = ''.join(data)
Best Practices
- Always close files after use
- Use
with
statement when possible - For large files, consider reading in chunks
- Be mindful of memory consumption when reading entire files
Potential Improvements
- Add error handling
- Consider file size before reading entire contents
- Use appropriate reading method based on file size and usage
Here's an analysis of the code snippet for potential memory leaks:
file = open('data.txt', 'r')
data = file.read()
This code reads the contents of a file called 'data.txt' into the memory. As for memory leaks, Python's garbage collector will handle the memory deallocation in both the 'file' and 'data' variables. However, there are some concerns:
File descriptor leak: The file object created by the
open
function holds onto the file descriptor until it is closed. If the file object is not closed, the file descriptor will be held open indefinitely, potentially leading to a resource leak. Although this is less likely to cause a significant issue in most standard use cases, it's still a good practice to close files when you're done with them.Fix: Use a
with
statement to guarantee that the file is properly closed after use:
with open('data.txt', 'r') as file:
data = file.read()
This ensures that the file is automatically closed when the block is exited, regardless of whether an exception occurs or not.
Memory usage: In Python, reading the entire file into memory at once can be memory-intensive for large files. This may not be a memory leak per se, but it's essential to be aware of it. If you're working with massive files, you might want to consider reading the file line by line or using a streaming approach to avoid loading the entire file into memory.
Fix: Use a streaming approach or read the file line by line to conserve memory:
with open('data.txt', 'r') as file:
for line in file:
# Process each line without loading the entire file into memory
data = line.strip()
# Do something with the line
Unclosed file: Although less likely, if the file object
file
is not properly closed due to certain exceptions, it could cause aFileNotFoundError
when another part of the code attempts to open the same file later.Fix: Ensure that the file is properly closed in all scenarios by using a
try-finally
block or a context manager (with
statement) as shown above.No exception handling: If
open
orread
fails, it will raise an exception. Consider adding exception handling to make your code more robust.Fix: Add exception handling to handle potential errors:
try:
with open('data.txt', 'r') as file:
data = file.read()
except FileNotFoundError:
print(f"File 'data.txt' not found.")
except IOError as e:
print(f"An I/O error occurred: {e}")
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
Comparing performance on code analysis across models shows interesting variations. ChatGPT 4o provided comprehensive analysis with clear structure and practical recommendations, though took nearly 8 seconds. Claude 3.5 Sonnet was fastest at 4.3 seconds and gave concise yet thorough feedback. Gemini 1.5 Pro took longest (9.4s) but offered detailed technical explanation distinguishing between memory and resource leaks. Llama 3.1 70B was impressively quick (1.6s) with solid technical analysis. All models correctly identified the core issue and recommended using a 'with' statement, but differed in depth and presentation of explanations.
Budget Tier
The analysis reveals varying depths of insight across the models. Gemini and Claude provided the most comprehensive responses, detailing both the resource leak issue and multiple solution approaches. ChatGPT gave a solid but more concise analysis focusing on basic resource management. Llama offered the fastest response time (1007ms) but was overly verbose. While all models correctly identified the file descriptor leak issue, Claude stood out by providing the most structured and actionable advice with clear code examples. Gemini took the longest to respond (10597ms) but offered detailed technical explanations. For this prompt, Claude demonstrated the best balance of speed, comprehensiveness, and practical solutions.
Concurrent Access Analysis
Detects potential race conditions in shared resource access. Important for multi-threaded application development.
Prompt:
Identify race conditions in this code: shared_counter = 0 def increment(): global shared_counter shared_counter += 1
Performance Results
The code snippet you provided is a classic example where race conditions might occur, particularly in a multithreaded or concurrent programming environment. A race condition happens when two or more threads access shared data and try to change it at the same time. Below are the specific points where race conditions can occur in this code:
Shared Resource Access: The variable
shared_counter
is a shared resource. If multiple threads call theincrement
function simultaneously, each will read the value ofshared_counter
, increment it, and then write it back. This can lead to incorrect results because the operation is not atomic.Increment is Non-Atomic: The operation
shared_counter += 1
involves multiple steps (reading the variable, incrementing its value, and writing it back). These steps are not performed atomically, meaning a thread switch could occur between any of these steps, leading to inconsistent modifications.No Synchronization Mechanism: There is no locking or synchronization mechanism to prevent multiple threads from entering the increment function at the same time. Without locks or other concurrency control mechanisms, threads interfere with each other, resulting in race conditions.
Global State Modification: Accessing and modifying a global variable from multiple threads without proper synchronization mechanisms increases the risk of race conditions.
To resolve or mitigate these race conditions, you can introduce synchronization techniques such as:
Using Locks: Employ a threading lock (like a mutex) to ensure that only one thread can execute the critical section modifying the shared variable at a time.
import threading shared_counter = 0 counter_lock = threading.Lock() def increment(): global shared_counter with counter_lock: shared_counter += 1
Atomic Operations: If the language/environment supports atomic operations for counter increments, use them to make sure the increment operation is performed atomically.
Concurrency Libraries: Use higher-level concurrency constructs provided by libraries, such as atomic variables, that handle synchronization internally.
Implementing one of these strategies can help eliminate the race condition by managing the access and modification of shared_counter
safely.
Race Condition Analysis
Identified Race Condition:
The code contains a critical race condition in the increment()
function when multiple threads access it simultaneously.
Problem Location:
shared_counter += 1
Why It's a Race Condition:
The +=
operation is not atomic and actually consists of three separate steps:
- Read the current value of
shared_counter
- Add 1 to that value
- Store the result back in
shared_counter
Example Scenario:
- Thread A reads
shared_counter
(value = 5) - Thread B reads
shared_counter
(value = 5) - Thread A adds 1 (5 + 1 = 6)
- Thread B adds 1 (5 + 1 = 6)
- Thread A stores 6
- Thread B stores 6
- Result: Counter only incremented once instead of twice
Solution: Use a synchronization mechanism like a mutex/lock:
from threading import Lock
shared_counter = 0
counter_lock = Lock()
def increment():
global shared_counter
with counter_lock:
shared_counter += 1
The race condition exists in the increment()
function because it's not atomic. The shared_counter += 1
operation is actually composed of three separate steps:
- Read: The current value of
shared_counter
is read. - Increment: The read value is incremented.
- Write: The incremented value is written back to
shared_counter
.
If multiple threads call increment()
concurrently, the following scenario can occur:
- Thread 1: Reads
shared_counter
(e.g., 0). - Thread 2: Reads
shared_counter
(also 0). - Thread 1: Increments its local copy (0 + 1 = 1).
- Thread 2: Increments its local copy (0 + 1 = 1).
- Thread 1: Writes its local copy (1) back to
shared_counter
. - Thread 2: Writes its local copy (1) back to
shared_counter
.
The final value of shared_counter
is 1, even though it should be 2 after two calls to increment()
. This is a classic example of a lost update due to a race condition. The increments from each thread interfere with each other because they are not executed atomically.
The given code has a potential race condition due to its use of the global
keyword and shared state.
In Python, the +=
operator is not atomic, meaning it consists of multiple operations:
- Read the current value of
shared_counter
. - Increment the value by 1.
- Assign the new value back to
shared_counter
.
When multiple threads or processes execute the increment
function concurrently, it's possible for them to interfere with each other, leading to incorrect results.
Here's a scenario that demonstrates the issue:
- Thread A reads the current value of
shared_counter
, which is 0. - Before Thread A can increment the value, Thread B reads the same value of 0.
- Thread A increments the value to 1 and assigns it back to
shared_counter
. - Thread B increments the value (which is still 0 from its perspective) to 1 and assigns it back to
shared_counter
.
As a result, the final value of shared_counter
is 1, but we would expect it to be 2 since both threads incremented the value.
To fix this issue, you can use a thread-safe or process-safe approach to update the shared state. Here are a few options:
Option 1: Use a lock
import threading
shared_counter = 0
lock = threading.Lock()
def increment():
global shared_counter
with lock:
shared_counter += 1
Option 2: Use an atomic increment operation
In Python 3.2 and later, you can use the threading
module's Lock
and the decimal
module to achieve an atomic increment operation:
import threading
import decimal
shared_counter = decimal.Decimal(0)
lock = threading.Lock()
def increment():
global shared_counter
with lock:
shared_counter += decimal.Decimal(1)
However, this approach is still not as efficient as using a lock.
Option 3: Use a thread-safe counter class
You can create a custom class that encapsulates the shared state and provides thread-safe increment methods:
import threading
class Counter:
def __init__(self):
self.value = 0
self.lock = threading.Lock()
def increment(self):
with self.lock:
self.value += 1
def get_value(self):
return self.value
shared_counter = Counter()
def increment():
shared_counter.increment()
In this example, the Counter
class uses a lock to synchronize access to the shared state, ensuring that the increment operation is atomic.
Option 4: Use the queue
module
If you're working in a multi-threaded environment, you can use the queue
module's Queue
class to manage the shared state. The Queue
class provides thread-safe enqueue and dequeue operations:
import queue
import threading
shared_counter_queue = queue.Queue()
def increment():
shared_counter_queue.put(1)
def get_counter():
counter = 0
while not shared_counter_queue.empty():
counter += shared_counter_queue.get()
return counter
In this example, the increment
function enqueues a value of 1, and the get_counter
function dequeues all values and returns the sum.
Choose the approach that best fits your use case and requirements.
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
The provided code is susceptible to a race condition because it modifies a shared variable, shared_counter
, without any form of synchronization. When multiple threads or processes attempt to increment shared_counter
simultaneously, they may interfere with each other's operations, leading to inconsistent or incorrect results.
Here's a breakdown of how the race condition can occur:
- Read-Modify-Write Operation: The operation
shared_counter += 1
consists of three steps:
- Read the current value of
shared_counter
. - Increment the value.
- Write the new value back to
shared_counter
.
- Concurrent Execution: If two threads execute the
increment()
function at the same time, both threads may read the same initial value ofshared_counter
before either has written back the incremented value. For instance, ifshared_counter
is initially 0:
- Thread A reads
shared_counter
(value 0). - Thread B reads
shared_counter
(value still 0). - Thread A increments the value and writes 1 back.
- Thread B increments the value (still 0) and writes 1 back.
As a result, the value of shared_counter
would incorrectly end up at 1 instead of 2, thereby losing one increment.
Potential Solutions
To avoid race conditions, you can use various synchronization mechanisms:
- Mutex (Mutual Exclusion): Use a lock to ensure that only one thread can increment the counter at a time.
import threading
shared_counter = 0
lock = threading.Lock()
def increment():
global shared_counter
with lock:
shared_counter += 1
Atomic Operations: If using a higher-level language or library that supports atomic operations or atomic variables, you could make the increment operation atomic.
Thread-safe Data Structures: In some scenarios, using thread-safe collections provided by libraries (like
queue.Queue
in Python) can also help avoid race conditions when managing shared data.
Using these mechanisms will prevent the race condition and allow for safe concurrent access to shared_counter
.
The code has a race condition because multiple threads (or processes) accessing and modifying shared_counter
concurrently can lead to incorrect results.
Let's break down why:
The instruction shared_counter += 1
is not atomic. It's actually a sequence of three operations:
- Read: The current value of
shared_counter
is read from memory. - Increment: The value is incremented by 1.
- Write: The new value is written back to memory.
If two threads execute increment()
simultaneously, they might both read the same value of shared_counter
(let's say it's 0). Then, both threads increment their local copy to 1. Finally, both threads write the value 1 back to shared_counter
, overwriting each other's changes. The final result is 1, instead of the expected 2. This is a classic race condition leading to data corruption. The outcome depends on the unpredictable timing of thread scheduling.
To fix this, you need to use synchronization primitives like locks (mutexes), atomic operations, or other thread-safe mechanisms to ensure that only one thread can access and modify shared_counter
at a time. For example, in Python:
import threading
shared_counter = 0
lock = threading.Lock()
def increment():
global shared_counter
with lock: # Acquire the lock before accessing shared_counter
shared_counter += 1
The with lock:
statement ensures that only one thread can hold the lock at a time. Other threads attempting to enter the with
block will block until the lock is released. This prevents the race condition. Alternatively, you could use threading.atomic.AtomicInteger
for a more efficient atomic increment.
I'll analyze the code for potential race conditions and explain the issues:
Race Condition Analysis
shared_counter = 0
def increment():
global shared_counter
shared_counter += 1
Race Condition Explanation
The code exhibits a classic race condition in a multi-threaded environment. Here's why:
- Non-Atomic Operation:
- The
shared_counter += 1
operation is not atomic - It actually involves three steps: a. Read the current value b. Increment the value c. Write the new value back
- Potential Interleaving:
- If multiple threads call
increment()
simultaneously, they might:- Read the same initial value
- Increment independently
- Overwrite each other's increments
Example Scenario
# Thread 1 reads shared_counter as 0
# Thread 2 reads shared_counter as 0
# Thread 1 increments to 1
# Thread 2 increments to 1 (instead of 2)
Mitigation Strategies
- Use Threading Locks:
import threading
lock = threading.Lock()
def increment():
global shared_counter
with lock:
shared_counter += 1
- Use Atomic Operations:
from threading import Lock
shared_counter = 0
counter_lock = Lock()
- Use Thread-Safe Counters:
from threading import Lock
from itertools import count
class ThreadSafeCounter:
def __init__(self):
self._value = 0
self._lock = Lock()
def increment(self):
with self._lock:
self._value += 1
Best Practices
- Always use synchronization mechanisms in multi-threaded environments
- Minimize the critical section (locked code)
- Consider using atomic operations or thread-safe data structures
The original code is not thread-safe and will likely produce incorrect results when multiple threads access increment()
concurrently.
Race Conditions in the Given Code
The given code can exhibit a race condition in a multi-threaded environment. A race condition occurs when two or more threads try to access and modify a shared resource simultaneously, leading to unpredictable behavior.
Here's why it's problematic:
shared_counter
is a shared global variable accessed by multiple threads (in this case, assumed to be threads executing theincrement()
function).- The
increment()
function is not thread-safe. It acquires no locks or other synchronization mechanisms to prevent concurrent modifications.
Example Failure Scenario
Suppose two threads, T1
and T2
, execute the increment()
function concurrently:
T1
reads the current value ofshared_counter
, which is0
.- Before
T1
can updateshared_counter
to1
,T2
reads the current value ofshared_counter
, which is still0
. T2
updatesshared_counter
to1
.T1
updatesshared_counter
to2
, now overwriting the increment intended byT2
.
As a result, the shared counter value might not reflect the actual number of increments. This scenario demonstrates how a race condition can lead to incorrect behavior.
Solution
To prevent race conditions, ensure that shared resources are accessed in a thread-safe manner. In this case, you can use a threading.Lock
object to synchronize access to shared_counter
. Here's an updated version of the code:
import threading
shared_counter = 0
lock = threading.Lock()
def increment():
global shared_counter
with lock:
shared_counter += 1
By acquiring the lock before modifying shared_counter
and releasing it after the modification, you ensure that only one thread can update the shared counter value at a time, preventing race conditions.
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
The models provided detailed analyses of the race condition in the shared counter code. ChatGPT 4.0 gave the most comprehensive response, covering both the issue and multiple solutions. Claude 3.5 provided a clear, structured analysis with a practical example scenario. Gemini 1.5 focused on explaining the mechanics of the race condition step-by-step. Llama 3.1 offered the most solution options but may have been overly verbose. All models correctly identified the core issue with the non-atomic increment operation, with ChatGPT showing the best balance of technical accuracy and practical solution guidance.
Budget Tier
Each model accurately identified the race condition in the shared counter code, but with varying levels of detail and clarity. ChatGPT 4o Mini provided thorough analysis with clear explanations and multiple solutions. Gemini 1.5 Flash offered a concise yet complete breakdown of the issue, including practical code examples. Claude 3.5 Haiku gave the most structured and comprehensive response, with detailed mitigation strategies. Llama 3.1 8B was notably faster (738ms vs 6000-8000ms for others) but provided less detailed analysis. Overall, Claude 3.5 Haiku's response was most valuable for practical implementation, while Llama 3.1 8B would be best for quick assessments.
Recursive Function Error Detection
Identifies missing base cases and potential stack overflow issues in recursive functions. Essential for recursive algorithm implementation.
Prompt:
What's the error in this recursive function? def factorial(n): return n * factorial(n-1)
Performance Results
The provided recursive function for calculating the factorial of a number has a couple of issues. Here's what needs to be addressed:
Base Case: The function lacks a base case, which is necessary to stop the recursion. Without a base case, the function will continue to call itself indefinitely, leading to a stack overflow error. For a factorial calculation, the typical base case is when ( n ) is 0 or 1, as ( 0! = 1! = 1 ).
Handling of Negative Values: Factorial is not defined for negative numbers. The function should account for this by either returning an error or handling such input appropriately.
Here's the corrected version of the function:
def factorial(n):
if n < 0:
raise ValueError("Factorial is not defined for negative numbers.")
elif n == 0 or n == 1:
return 1
else:
return n * factorial(n-1)
This corrected function includes:
- A base case that stops the recursion when ( n ) is 0 or 1.
- An error raise for negative inputs to indicate that the factorial is not defined for such cases.
Here's the key issue with this recursive factorial function:
Missing Base Case
def factorial(n):
return n * factorial(n-1) # Will recurse infinitely
The function will cause a stack overflow because:
- It doesn't have a base case to stop the recursion
- It will keep calling itself with decreasing values of
n
indefinitely - Eventually, it will exceed Python's maximum recursion depth
Corrected Version:
def factorial(n):
if n == 0 or n == 1: # Base case
return 1
return n * factorial(n-1)
The corrected version includes a base case that stops recursion when n
reaches 0 or 1, preventing infinite recursion.
The provided recursive function for factorial is missing a base case. Without a base case, the recursion will never stop, leading to a RecursionError: maximum recursion depth exceeded
.
Here's why:
- The function calls itself:
factorial(n)
callsfactorial(n-1)
. - Infinite loop: This continues:
factorial(n-1)
callsfactorial(n-2)
, thenfactorial(n-3)
, and so on. - No stopping condition: There's nothing to tell the recursion when to stop. It will keep trying to compute factorials of smaller and smaller numbers, eventually reaching negative numbers and continuing indefinitely.
How to fix it:
You need to add a base case. For the factorial function, the base case is when n
is 0 (0! = 1). Here's the corrected code:
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n-1)
Now, when n
reaches 0, the function returns 1, stopping the recursion and allowing the chain of multiplications to unwind and produce the correct result.
The error in this recursive function is that it does not have a base case to stop the recursion.
In recursion, a base case is a condition that, when met, stops the recursive calls. In this function, no matter what value of n
is passed, the function will keep calling itself with decreasing values of n
until it encounters a negative number. This will lead to a RecursionError
due to exceeding the maximum recursion depth.
To fix this, we need to add a base case to handle the condition when n
is 0 or 1 (since the factorial of 0 and 1 is 1). Here's the corrected function:
def factorial(n):
if n == 0 or n == 1: # base case
return 1
elif n < 0: # error handling
raise ValueError("Factorial is not defined for negative numbers")
else:
return n * factorial(n-1)
With this base case, the function will stop calling itself once n
is 0 or 1, and it will correctly calculate the factorial of a given number. Additionally, it will raise an error for negative numbers since factorial is not defined for them.
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
The recursive function you've written for calculating the factorial of a number has a critical error: it lacks a base case. Without a base case, the function will keep calling itself indefinitely for values of n
greater than 0, leading to a recursion error (maximum recursion depth exceeded) when n
reaches a negative value.
Here's how you can fix it by adding a base case:
def factorial(n):
if n == 0 or n == 1: # Base case
return 1
return n * factorial(n - 1) # Recursive case
In this corrected version:
- The base case
if n == 0 or n == 1
handles the fact that the factorial of 0 and the factorial of 1 is 1. - For any other positive integer
n
, the function calls itself withn - 1
, multiplyingn
by the factorial ofn - 1
. This will eventually reach the base case and stop the recursion.
The error is that the function lacks a base case. A recursive function needs a condition to stop the recursion; otherwise, it will keep calling itself until it runs out of stack space (resulting in a RecursionError
).
The factorial(n)
function continuously calls itself with a smaller n
but never stops. It needs a base case, typically when n
reaches 0 (where the factorial is 1).
Here's the corrected version:
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n-1)
This version checks if n
is 0. If it is, it returns 1 (the factorial of 0). Otherwise, it proceeds with the recursive step. This base case prevents the infinite recursion.
The error in this recursive function is that it lacks a base case, which would terminate the recursion. Without a base case, the function will continue calling itself indefinitely, leading to a RecursionError.
Here's a corrected version of the factorial function:
def factorial(n):
# Base case: factorial of 0 or 1 is 1
if n == 0 or n == 1:
return 1
# Recursive case
return n * factorial(n-1)
The key issues with the original function were:
- No Base Case: The function would recursively call itself without any condition to stop.
- Infinite Recursion: This would cause a
RecursionError
when the call stack becomes too deep.
The corrected version:
- Adds a base case for 0 and 1 (both have a factorial of 1)
- Ensures the recursion will terminate
- Properly calculates the factorial by multiplying the current number with the factorial of the number below it
Note: This function will still raise an error for negative numbers, so you might want to add additional error checking if needed.
The error in this recursive function is that it does not handle the base case.
In a recursive function, every recursive call must eventually reach a base case where it returns without making further recursive calls. This base case is necessary to prevent infinite recursion and stack overflows.
For the factorial function, the base case should be when n
is 0 or 1, because the factorial of 0 and 1 is 1.
Here's the corrected code:
def factorial(n):
if n == 0 or n == 1: # base case
return 1
else:
return n * factorial(n-1)
However, this recursive function has a high risk of causing a RecursionError if n
is a large number because it does not check if n
is a non-negative integer. A more robust version of the function could handle this:
def factorial(n):
if not isinstance(n, int) or n < 0:
raise ValueError("Input n must be a non-negative integer.")
if n == 0 or n == 1: # base case
return 1
else:
return n * factorial(n-1)
For large values of n
, it's recommended to use an iterative solution to avoid potential stack overflow issues:
def factorial(n):
if not isinstance(n, int) or n < 0:
raise ValueError("Input n must be a non-negative integer.")
result = 1
for i in range(2, n + 1):
result *= i
return result
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
All models correctly identified the missing base case as the primary error. ChatGPT 4o and Llama 70B provided the most comprehensive solutions, including negative number handling. Claude Sonnet delivered the most concise explanation, while Gemini Pro offered detailed reasoning but focused solely on the base case. Response times varied significantly - Llama was fastest at 1257ms, while Gemini took longest at 6097ms. ChatGPT and Claude fell in between. For code debugging, Llama showed the best balance of speed, completeness, and clarity.
Budget Tier
All models correctly identified the missing base case as the key error in the recursive factorial function. Llama 3.1 8B was the fastest (724ms) and provided the most comprehensive solution, including input validation and an iterative alternative. ChatGPT 4o Mini (3279ms) and Gemini 1.5 Flash (1733ms) gave concise, accurate explanations with corrected code. Claude 3.5 Haiku was slowest (4571ms) but offered clear explanations of both the error and solution. While all models performed well, Llama 3.1 8B stood out for its speed and thorough coverage.
Authentication Security Audit
Evaluates authentication code for security vulnerabilities and hardcoded credentials. Critical for secure application development.
Prompt:
Find security vulnerabilities in this login code: def check_password(user_input): password = 'secret123' if user_input == password: return True
Performance Results
This Python code implements a simple password check function. While the code is quite minimal, there are several security issues and best practice violations that should be addressed:
Hardcoded Passwords: The password is hardcoded in the source code. This is a significant security vulnerability as it can be easily extracted if someone gains access to the source code. Hardcoded credentials should be avoided in production. Instead, use environment variables or a secure vault service to manage sensitive information.
No Account Management: The function uses only one password for authentication without any concept of user accounts. A proper authentication system should associate passwords with unique user identifiers.
Lack of Salting and Hashing: Passwords should never be stored or compared in plain text. Instead, when a password is registered, it should be hashed using a cryptographic hashing algorithm like bcrypt, Argon2, or PBKDF2 along with a unique salt. When checking passwords, compare the hashed user input with the stored hashed password.
Missing Rate Limiting: The function does not implement any form of brute force protection. An adversary could attempt to guess the password without any throttling mechanism to slow down their efforts. Implement rate limiting and locking mechanisms after several failed attempts.
No Error Feedback: Although not directly visible in this function alone, providing clear error messages (e.g., differentiating between a wrong username and a wrong password) can be used by attackers to gather information about the system. It’s better to give general error messages.
Input Validation: Ensure user inputs are validated and sanitized to prevent injection attacks or unexpected behavior, especially when expanding this code to handle more complex scenarios.
Insufficient Logging: Implement secure logging mechanisms to track successful and failed login attempts. Ensure logs do not contain sensitive information like passwords.
Consider using established libraries or frameworks that provide secure authentication mechanisms instead of implementing your own from scratch. These libraries often include well-tested methods for handling passwords securely, such as hashing strategies and session handling.
Security Vulnerabilities
- Hardcoded Password
- The password is directly stored in the code as plaintext
- Anyone with access to the source code can see the password
- Makes it impossible to change passwords without modifying code
- Unsafe Comparison
- Uses direct string comparison (
==
) which is vulnerable to timing attacks - Attackers can measure response time differences to guess password characters
- No Password Hashing
- Password stored as plaintext instead of using cryptographic hashing
- If system is compromised, actual password is exposed
- Should use secure hashing algorithms like bcrypt/Argon2
- No Salt
- No random salt used with password storage
- Makes passwords vulnerable to rainbow table attacks
- Same passwords will have identical hashes
- Missing Input Validation
- No checks for password length, complexity, or malicious input
- Could allow buffer overflow or injection attacks
- Should sanitize and validate all user input
- No Rate Limiting
- No protection against brute force attacks
- Attackers can make unlimited password attempts
- Should implement request throttling/account lockouts
Secure Implementation Example:
import bcrypt
from typing import Optional
from time import sleep
from random import random
def check_password(user_input: str) -> bool:
# Store hashed password
hashed = b'$2b$12$LQv3c1yqBWVHxkd0LHAkCOYz6TtxMQJqhN8/LedYQNB8UHUt2Cc7.'
# Add random delay to prevent timing attacks
sleep(random() * 0.1)
# Use constant-time comparison
try:
return bcrypt.checkpw(user_input.encode('utf-8'), hashed)
except Exception:
return False
This code has several security vulnerabilities:
Hardcoded Password: The most glaring issue. Storing passwords directly in code is extremely risky. If an attacker gains access to the code, they have the password. Passwords should be stored securely, typically using hashing and salting.
No Rate Limiting: The code doesn't prevent brute-force attacks. An attacker can try thousands of passwords per second. Rate limiting (e.g., delaying or blocking login attempts after a certain number of failures) is essential.
No Account Lockout: Similar to rate limiting, there's no mechanism to lock an account after a set number of incorrect attempts. This leaves accounts vulnerable to brute-force attacks.
Plaintext Password Comparison: While less of a concern than the hardcoded password itself, comparing the user input directly to the stored password means the password is exposed in memory during the comparison. This could be exploited by a sophisticated attacker. Hashing the user's input and comparing the hashes is safer.
No Input Validation: There are no checks on the
user_input
. Maliciously crafted input (extremely long strings, special characters, etc.) could cause unexpected behavior or even crash the application.No Logging: Without logging failed login attempts, it's difficult to detect brute-force attacks or other suspicious activity.
No Password Complexity Requirements: Although not directly related to the code snippet, a secure login system should enforce password complexity rules (minimum length, required character types, etc.) to make passwords harder to guess.
Here's an example of how to improve the code (still simplified, but addresses the key vulnerabilities):
import hashlib
import time
# Simulate a database of hashed passwords. In a real application,
# use a proper database and a more robust hashing method (e.g., bcrypt, scrypt)
hashed_passwords = {
'user1': 'pbkdf2:sha256:260000$5v7vR4820sK9oEB$fca467f043e75d1648240a842b784213e5c922844a255431a7f27597d49815f6', # Example hash
}
failed_attempts = {} # Store failed attempts per user
def check_password(username, user_input):
global failed_attempts
if username not in hashed_passwords:
return False
# Rate limiting
now = time.time()
if username in failed_attempts and now - failed_attempts[username][-1] < 5:
print("Too many attempts. Please wait.")
return False
stored_hash = hashed_passwords[username]
salt = stored_hash.split('$')[1] # extract the salt from the hash
key = hashlib.pbkdf2_hmac(
'sha256', # The hash digest algorithm for HMAC
user_input.encode('utf-8'), # Convert the password to bytes
salt.encode('utf-8'), # Provide the salt
260000 # It is recommended to use at least 100,000 iterations of SHA-256
)
derived_key = 'pbkdf2:sha256:260000$' + salt + '$' + key.hex()
if derived_key == stored_hash:
if username in failed_attempts:
del failed_attempts[username] # Reset failed attempts on successful login
return True
else:
if username not in failed_attempts:
failed_attempts[username] = []
failed_attempts[username].append(now)
if len(failed_attempts[username]) >= 3: # Example lockout after 3 attempts
print("Account locked due to too many failed attempts.")
# Implement account lockout mechanism here (e.g., disable the account)
return False
Key Improvements:
- PBKDF2 Hashing: Uses
hashlib.pbkdf2_hmac
to hash the password instead of storing it in plaintext. This is significantly more secure, although bcrypt or scrypt are generally preferred. - Rate Limiting: Introduces a basic delay between login attempts.
- Account Lockout (Simulated): Demonstrates the logic for locking an account after multiple failed attempts.
- Dictionary for Hashed Passwords: Simulates storing the hashed passwords in a dictionary instead of hardcoding them. In a real application, use a proper database.
- Failed Attempts Tracking: Uses a dictionary to track failed attempts per user.
This improved code is still a simplified example. In a real-world application, you would need to use more robust security measures, including:
- Using a strong password hashing function (bcrypt, scrypt, Argon2).
- Implementing proper input validation.
- Securely storing and managing salts.
- Integrating with a robust database solution.
- Implementing proper logging and monitoring.
- Using a well-tested security framework or library.
- Employing multi-factor authentication (MFA).
Remember that security is a continuous process, so stay up-to-date on best practices and vulnerabilities.
Security Vulnerabilities
The provided check_password
function is vulnerable to several security issues:
1. Hardcoded Password
The password 'secret123' is hardcoded directly in the function. This is insecure because:
- If an attacker gains access to the code, they can easily obtain the password.
- If the password needs to be changed, the code needs to be modified and redeployed.
2. Plain Text Storage
The password is stored in plain text. This means that if an attacker gains access to the password, they can use it directly.
3. Lack of Input Validation
The function does not validate the user's input. This makes it vulnerable to:
- Denial of Service (DoS) attacks: An attacker could flood the function with large inputs, causing the system to run out of resources.
- Special Character Injection: An attacker could inject special characters to cause unexpected behavior.
4. Missing Logging and Monitoring
The function does not log or monitor failed login attempts. This makes it difficult to detect and respond to potential security breaches.
5. No Rate Limiting
The function does not limit the number of login attempts. This makes it vulnerable to brute-force attacks, where an attacker tries all possible passwords until they find the correct one.
Securing the Code
Here's an example of how the code could be improved:
import bcrypt
import logging
# Set up logging
logging.basicConfig(level=logging.INFO)
# Store passwords securely (hashed and salted)
stored_password = bcrypt.hashpw('secret123'.encode('utf-8'), bcrypt.gensalt())
def check_password(user_input):
# Input validation
if not isinstance(user_input, str) or len(user_input) > 128:
logging.warning('Invalid input: {}'.format(user_input))
return False
# Compare hashed input with stored password
if bcrypt.checkpw(user_input.encode('utf-8'), stored_password):
logging.info('Login successful')
return True
else:
logging.warning('Login failed: {}'.format(user_input))
return False
# Rate limiting (example using a simple leaky bucket algorithm)
class RateLimiter:
def __init__(self):
self.tokens = 5 # Maximum 5 login attempts per minute
self.last_refill = 0
def allow(self):
import time
current_time = time.time()
if current_time - self.last_refill >= 60: # 1 minute has passed
self.tokens = 5 # Refill tokens
self.last_refill = current_time
if self.tokens > 0:
self.tokens -= 1
return True
else:
return False
rate_limiter = RateLimiter()
def check_password_rate_limited(user_input):
if rate_limiter.allow():
return check_password(user_input)
else:
logging.warning('Rate limit exceeded')
return False
In this improved version:
- Passwords are stored securely using bcrypt (hashed and salted).
- Input validation is performed to prevent invalid input.
- Logging and monitoring are added to detect security breaches.
- A simple rate limiter is implemented to prevent brute-force attacks.
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
There are several security vulnerabilities and best practices that should be considered in the provided code. Here are a few key issues:
Hardcoded Password: The password is hardcoded in the function, which is insecure. If someone gains access to the source code, they can easily find the password. Sensitive information like passwords should be stored securely in a configuration file or an environment variable, rather than being embedded directly in the code.
Lack of Input Sanitization: The code does not perform any input validation or sanitization. While this specific example is a simple password check, in a broader context, it is always a good practice to validate and sanitize user inputs to avoid potential injection attacks.
Ineffective Password Storage: In real-world applications, passwords should never be stored in plaintext. Instead, passwords should be hashed using a strong hashing algorithm (e.g., bcrypt, Argon2) with appropriate salting. This way, even if the database is compromised, the attackers can’t easily retrieve the actual passwords.
No Account Lockout Mechanism: The function does not handle failed login attempts. An account lockout mechanism would prevent brute force attacks by locking the account after a certain number of failed attempts.
No Security Logging: There’s no logging of authentication attempts. Logging failed attempts can help detect unauthorized access attempts.
Lack of Rate Limiting: There is no implementation for rate limiting which can prevent automated attempts to guess the password.
No Multi-Factor Authentication (MFA): This login mechanism does not consider adding an extra layer of security with multi-factor authentication, which would enhance security significantly.
Return Value Sensitivity: The function returns
True
for successful login without providing much information on failure. While this is good for security, it’s also important to consider user experience. A generic failure message can help guide the user without revealing specific details about what went wrong.
Here’s an improved version of the code that addresses some of these vulnerabilities:
import os
import bcrypt
# Retrieve hashed password from an environment variable or secure storage
hashed_password = os.environ.get('HASHED_PASSWORD')
def check_password(user_input):
if hashed_password is None:
raise Exception("Password not set up correctly.")
# Check if the input matches the hashed password
return bcrypt.checkpw(user_input.encode('utf-8'), hashed_password.encode('utf-8'))
# Example of how to securely store a password
def store_password(plain_password):
hashed = bcrypt.hashpw(plain_password.encode('utf-8'), bcrypt.gensalt())
# Store `hashed` in a secure location, e.g., a database or an environment variable
In this improved version, we use bcrypt to securely hash and check passwords, and we do not hardcode the password directly in the code. Instead, we recommend storing sensitive information securely.
This login code has several significant security vulnerabilities:
Plaintext Password Storage: The most glaring issue is storing the password ("secret123") directly in the code. This is extremely insecure. Anyone with access to the code (or even just a decompiled version of the code if it's compiled) can see the password. This is a fundamental flaw. Passwords should never be stored in plain text. They should be securely hashed using a strong, one-way hashing algorithm (like bcrypt, Argon2, or scrypt) and salted.
Lack of Input Validation: The code doesn't perform any input validation on
user_input
. A malicious user could potentially use techniques like SQL injection (if this was part of a larger system interacting with a database) or other injection attacks to bypass the simple equality check. Even without a database, they could try various techniques to disrupt the application.Lack of Rate Limiting: The code doesn't limit the number of login attempts. A brute-force attack could easily be launched to try many passwords until "secret123" is found. Implementing rate limiting (e.g., temporarily blocking an IP address after multiple failed attempts) is crucial.
No protection against timing attacks: If the comparison (
user_input == password
) is implemented in a way that takes slightly different time based on whether the input matches, attackers could use timing attacks to deduce information about the password.Lack of session management: There's no mention of session management. After successful authentication, the code doesn't provide any mechanism to securely manage the user's session, leaving the user vulnerable to session hijacking.
Hardcoded Password: The password itself is weak. "secret123" is an easily guessable password. Password complexity rules should be enforced and strong, unique passwords should be used, ideally managed securely by the system.
In short, this code snippet is a textbook example of how not to implement password authentication. A proper implementation would require a robust hashing algorithm, input validation, rate limiting, secure session management, and a strong password policy, potentially using a dedicated authentication library.
Here's an analysis of the security vulnerabilities in this login code:
Security Vulnerabilities
- Hardcoded Password
- The password is directly written in the code (
'secret123'
) - This is a critical security flaw as anyone with access to the source code can see the password
- Passwords should be securely hashed and stored, not in plain text
- Weak Password
'secret123'
is an extremely weak and predictable password- It uses a common word with simple numeric appending
- Easily guessable by brute-force or dictionary attacks
- Direct Comparison
- Using
==
for password comparison is insecure - Vulnerable to timing attacks where attackers can infer information about the password
- Secure comparison should use constant-time comparison methods
- No Input Validation
- No checks on input length, character types, or other security measures
- No protection against repeated login attempts
- No account lockout mechanism
Recommended Improvements
import hashlib
import secrets
def secure_password_check(user_input):
# Use secure password hashing
stored_hash = hash_password('secret123')
# Use constant-time comparison
return secrets.compare_digest(
hash_password(user_input),
stored_hash
)
def hash_password(password):
# Use strong hashing with salt
salt = secrets.token_hex(16)
return hashlib.sha256((salt + password).encode()).hexdigest()
Best Practices
- Use strong password hashing (bcrypt, Argon2)
- Implement account lockout mechanisms
- Add multi-factor authentication
- Never store passwords in plain text
- Use secure, constant-time comparison methods
Here's a list of security vulnerabilities found in the given login code:
Hardcoded Password: The password is hardcoded directly into the function. This is a significant security risk as an attacker who gains access to the function's code can easily obtain the password. To mitigate this, passwords should be stored securely separately from the rest of the application code.
No Input Validation: The function doesn't validate the input type of
user_input
. If the function is called with a non-string input, such as an integer or a list, it will be compared to the password as if it were a string, leading to unexpected behavior. At the very least, type validation should be performed.No Input Sanitization: Although input validation is performed to some extent by checking that the input is a string, no sanitization is performed to prevent SQL injection or cross-site scripting (XSS) attacks.
Potential for Timing Attack: The current implementation of the function performs a simple comparison between the input and the password. This comparison can potentially leak information about the password, as the function will take a different amount of time to execute depending on whether the input is correct or not. This opens up the possibility of timing attacks, where an attacker is able to infer information about the password by measuring the time it takes for the function to execute.
No Password Strength Validation: There is no check to ensure that the user-provided password meets minimum password strength requirements. This can reduce the overall security of the system by allowing weak passwords to be used.
No Account Lockout Policy: There is no check to prevent brute-force attacks, where an attacker tries multiple passwords in succession. To prevent this, a feature to lock out a user after a certain number of attempts within a set timeframe could be implemented.
Here's an example of how to update the function to address these issues:
import time
import hashlib
import logging
class Login:
def __init__(self):
self.password_hash = hashlib.sha256('secret123'.encode()).hexdigest()
def check_password(self, user_input: str) -> bool:
"""
Checks whether the provided user input is equal to the password.
Args:
user_input: The user's input to check.
Returns:
True if the user input is correct, False otherwise.
"""
if not isinstance(user_input, str) or len(user_input) < 8:
logging.warning("Invalid input provided")
return False
start_time = time.time()
user_input_hash = hashlib.sha256(user_input.encode()).hexdigest()
if user_input_hash == self.password_hash:
return True
end_time = time.time()
logging.warning(f"Password check failed in {end_time - start_time:0.6f} seconds ({user_input})")
return False
In this example, the function:
- Hashes the actual password when the class is initialized.
- Checks whether the input begins with at least 8 characters.
- Uses SHA-256 to hash the provided input, and then checks whether the input is the same as the known password hash.
- Logs a warning if the user input is invalid or if the password check fails.
- Includes checks to reduce the risk of timing attacks.
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
The analysis reveals varying capabilities across models in identifying security vulnerabilities in the login code:
ChatGPT 4o provided comprehensive analysis focusing on practical concerns like hardcoded passwords, account management, and rate limiting. The response was well-structured but relatively brief.
Claude Sonnet offered detailed technical insights, including timing attacks and salt usage, plus provided secure implementation code using bcrypt.
Gemini Pro gave the most exhaustive analysis with extensive code examples and implementation details, though took significantly longer to respond.
Llama 70B was quickest to respond and organized findings clearly with both vulnerabilities and solutions, including practical code examples with logging and rate limiting.
Overall, Gemini provided the most thorough analysis, while Llama offered the best balance of speed and practical detail.
Budget Tier
The models varied significantly in their analysis of the login code vulnerabilities. Claude and Llama provided the most comprehensive responses, identifying nuanced issues like timing attacks and suggesting specific code improvements. ChatGPT gave a solid but more general analysis, while Gemini's response was well-structured but less technical. Response times ranged from Llama's quick 1.2s to ChatGPT's slower 9.3s. Llama stood out for balancing speed with detailed, implementable solutions, though all models correctly identified the critical hardcoded password vulnerability. Claude's response was particularly well-organized with clear sections and practical code examples.
Performance Verdict
Based on the series of code analysis comparisons across ChatGPT 4.0, Claude 3.5 Sonnet, Gemini 1.5 Pro, and Llama 3.1 70B, here's how the models performed in Bug Detection tasks:
ChatGPT 4.0:
- Consistently provided comprehensive yet well-balanced analyses
- Excelled at practical solution recommendations
- Strong at explaining complex concepts clearly
- Moderate response times
- Best overall at balancing technical depth with accessibility
Claude 3.5 Sonnet:
- Consistently concise and well-structured responses
- Strong focus on key issues without unnecessary detail
- Excellent at providing practical code solutions
- Good response times
- Best for efficient, practical analysis
Gemini 1.5 Pro:
- Most detailed and thorough technical explanations
- Offered extensive optimization suggestions
- Consistently slowest response times
- Sometimes overly verbose
- Best for in-depth technical analysis
Llama 3.1 70B:
- Consistently fastest response times
- Well-structured, academic-style analysis
- Strong code documentation
- Sometimes included superfluous information
- Best for rapid analysis needs
Winner: ChatGPT 4.0
ChatGPT 4.0 wins for maintaining the best balance of comprehensiveness, clarity, and practical utility across all test cases. While other models excelled in specific areas (Llama in speed, Gemini in depth, Claude in conciseness), ChatGPT consistently delivered well-rounded analyses that would be most valuable for real-world debugging scenarios.
Runner-up: Claude 3.5 Sonnet, for its efficient and practical approach to bug detection and solution implementation.
Budget Verdict
Based on the comparative analyses across multiple bug detection scenarios, here's how the models performed:
ChatGPT 4o Mini:
- Most comprehensive technical analysis
- Detailed code examples and solutions
- Strong at identifying multiple issues
- Consistently slowest response times
- Sometimes overly verbose
Claude 3.5 Haiku:
- Best structured and organized responses
- Clear, practical implementation advice
- Balanced technical depth with accessibility
- Strong security vulnerability analysis
- Generally slower response times
- Sometimes too concise on complex issues
Gemini 1.5 Flash:
- Clear, accessible explanations
- Well-structured responses
- Good at providing context
- Sometimes missed technical details
- Fewer code examples
- Inconsistent depth of analysis
Llama 3.1 8B:
- Consistently fastest response times
- Strong technical solutions
- Detailed code examples
- Good at providing alternatives
- Occasionally missed critical issues
- Sometimes overly verbose
- Less structured responses
Overall Winner: Claude 3.5 Haiku
While each model showed strengths in different areas, Claude 3.5 Haiku consistently delivered the most balanced and practical bug detection analysis. Its responses combined technical accuracy, clear structure, and actionable solutions. Though not the fastest, its superior organization and comprehensive coverage of security considerations make it the most reliable choice for code review and bug detection tasks. Llama 3.1 8B deserves an honorable mention for its impressive speed and technical depth, making it suitable for rapid code reviews where time is critical.
Conclusion
Conclusion
After extensive testing of both performance and budget-tier AI models in bug detection scenarios, clear patterns emerged in their capabilities and optimal use cases. In the performance tier, ChatGPT 4.0 demonstrated superior all-around capabilities, consistently delivering well-balanced analyses that combined technical accuracy with practical utility. Among budget options, Claude 3.5 Haiku proved most reliable for structured, comprehensive bug detection despite slower response times.
Response speed varied significantly between tiers, with budget models generally being 2-3 times faster but sacrificing some depth and accuracy. Notably, Llama models (both 70B and 8B versions) consistently provided the fastest responses in their respective tiers, making them ideal for rapid code reviews.
For professional development environments requiring thorough code analysis, the performance tier models justify their higher costs through superior detection of subtle bugs and security vulnerabilities. However, budget tier models, particularly Claude 3.5 Haiku and Llama 3.1 8B, proved more than capable for routine code reviews and basic bug detection tasks.
These findings suggest organizations should consider a hybrid approach - using budget tier models for initial rapid analysis and performance tier models for critical code reviews or complex debugging scenarios. This strategy optimizes both cost efficiency and code quality while leveraging the unique strengths of each model tier.