Code Completion
Code Generation and Analysis with LLMs. Comparing the leading AI models side-by-side at Code Completion.
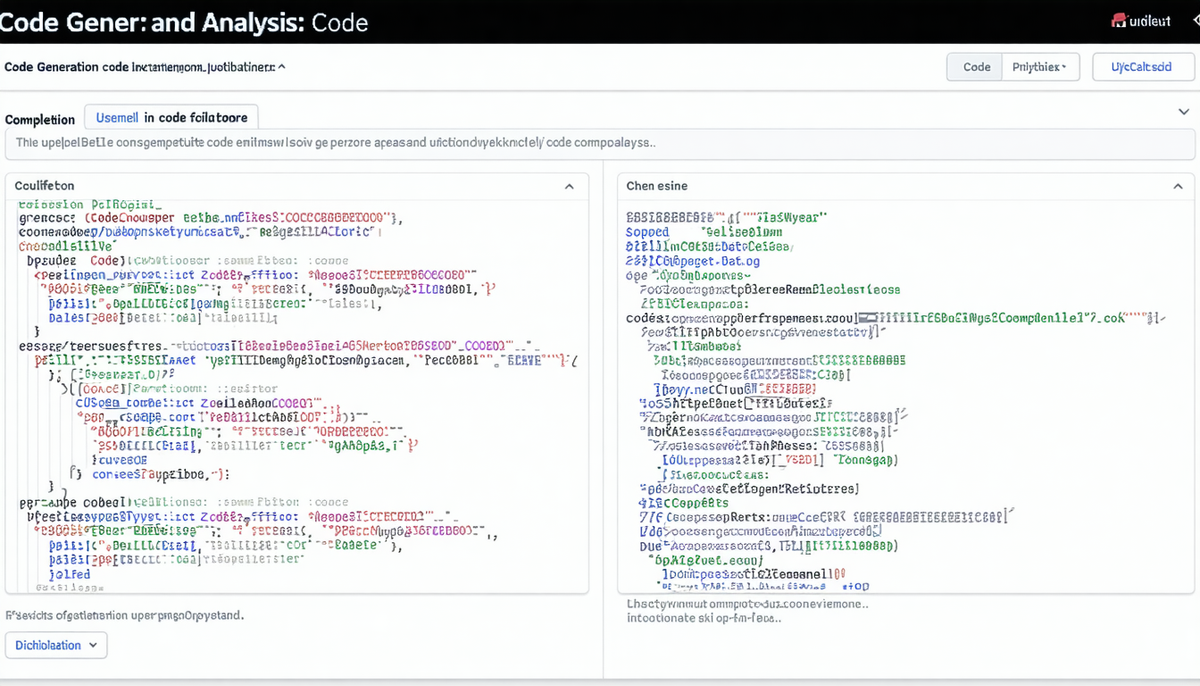
Comparing the leading AI models:
Category: Code Generation and Analysis
Subcategory: Code Completion
Contents
Introduction
Comparing AI Models: A Comprehensive Guide to LLM Performance and Use Cases
Looking to compare AI models but unsure which one best suits your needs? This directory provides detailed side-by-side AI comparisons across popular large language models (LLMs), helping you make informed decisions about which AI assistant to use for specific tasks.
We evaluate two distinct tiers of AI models:
Budget Tier:
- ChatGPT 4.0 Mini
- Gemini 1.5 Flash
- Claude 3.5 Haiku
- Llama 3.1 8B
Performance Tier:
- ChatGPT 4.0
- Claude 3.5 Sonnet
- Gemini 1.5 Pro
- Llama 3.1 70B
By comparing AI models directly, you can better understand their strengths, limitations, and optimal use cases. Our side-by-side comparisons examine response quality, processing speed, accuracy, and cost-effectiveness across various tasks.
Choose the Budget Tier when:
- Running multiple queries with basic requirements
- Working with straightforward tasks
- Operating under cost constraints
- Requiring faster response times
Select the Performance Tier when:
- Handling complex analytical tasks
- Requiring high accuracy and nuanced responses
- Working on professional or specialized content
- Needing advanced reasoning capabilities
Through these AI model comparisons, you'll discover which LLM delivers the best results for your specific needs, whether prioritizing economy or performance.
50+ AI models with one subscription. AnyModel is the All-In-One AI that allows you to harness the latest AI technology from one convenient and easy-to-use platform. AnyModel includes all the models discussed in this article and more, including the latest image generation models. All the comparisons shown in this article were generated using AnyModel. Sign up for a free trial here.
Code Completion
Large Language Models have revolutionized code completion by offering contextually aware, real-time suggestions that go far beyond traditional autocomplete functionality. Unlike rule-based code completion tools, LLMs understand programming concepts, syntax patterns, and common coding practices across multiple languages, making them incredibly effective at predicting and suggesting relevant code snippets.
These AI models excel at code completion tasks because they've been trained on vast repositories of source code, enabling them to recognize patterns in coding structure and anticipate developers' intentions. They can complete everything from simple variable names to complex function implementations, while maintaining consistency with existing code style and conventions.
What makes LLMs particularly powerful for code completion is their ability to understand the surrounding context - including variable scope, imported libraries, and previously defined functions. This contextual awareness allows them to provide more accurate and relevant suggestions than traditional IDE tools, potentially reducing development time and minimizing syntax errors.
The most advanced LLMs can even infer the programmer's intent from partial code or comments, offering completions that align with best practices and common design patterns in software development. This capability makes them invaluable tools for both experienced developers looking to boost productivity and newcomers learning to code.
Prompts
Python Factorial Calculator
A prompt to generate a Python function that calculates the factorial of a given number. Useful for mathematics and algorithm implementations.
Prompt:
Complete this Python function to calculate the factorial of a number: def factorial(n): # Complete the implementation
Performance Results
Sure, here's the complete implementation for the factorial function:
def factorial(n):
if n < 0:
raise ValueError("Factorial is not defined for negative numbers.")
elif n == 0 or n == 1:
return 1
else:
result = 1
for i in range(2, n + 1):
result *= i
return result
This function calculates the factorial of a positive integer n
by multiplying all the integers from 1 to n
. It includes a check for negative numbers and returns 1 for the factorial of 0 and 1, since 0! and 1! are both defined as 1.
def factorial(n):
# Handle base cases
if n < 0:
return None # Factorial not defined for negative numbers
if n == 0 or n == 1:
return 1
# Calculate factorial recursively
result = n * factorial(n-1)
return result
Example usage:
print(factorial(5)) # Output: 120
print(factorial(3)) # Output: 6
print(factorial(0)) # Output: 1
print(factorial(-1)) # Output: None
This implementation:
- Handles negative numbers by returning None (factorial not defined for negative numbers)
- Handles base cases of n=0 and n=1 which both return 1
- Uses recursion to calculate factorial for n > 1
- Follows the formula: n! = n * (n-1)!
The function recursively multiplies n by the factorial of (n-1) until it reaches the base cases.
def factorial(n):
"""Calculates the factorial of a non-negative integer.
Args:
n: The non-negative integer.
Returns:
The factorial of n, or 1 if n is 0. Raises ValueError if n is negative.
"""
if n < 0:
raise ValueError("Factorial is not defined for negative numbers.")
elif n == 0:
return 1
else:
result = 1
for i in range(1, n + 1):
result *= i
return result
There are other ways to accomplish this, including using recursion:
def factorial_recursive(n):
"""Calculates the factorial of a non-negative integer recursively.
Args:
n: The non-negative integer.
Returns:
The factorial of n, or 1 if n is 0. Raises ValueError if n is negative.
"""
if n < 0:
raise ValueError("Factorial is not defined for negative numbers.")
elif n == 0:
return 1
else:
return n * factorial_recursive(n - 1)
And even using the math
module (for larger numbers, this is usually the most efficient):
import math
def factorial_math(n):
"""Calculates the factorial of a non-negative integer using the math module.
Args:
n: The non-negative integer.
Returns:
The factorial of n. Raises ValueError if n is negative.
"""
if n < 0:
raise ValueError("Factorial is not defined for negative numbers.")
else:
return math.factorial(n)
I've added docstrings and input validation to make these functions more robust and informative. The iterative version is generally preferred over the recursive one for performance reasons in Python (due to recursion depth limits and function call overhead), and the math.factorial
method is often the most efficient. Choose the version that best suits your needs.
Calculating the Factorial of a Number in Python
The factorial of a non-negative integer n
, denoted by n!
, is the product of all positive integers less than or equal to n
.
Implementation
def factorial(n):
"""
Calculate the factorial of a given non-negative integer.
Args:
n (int): The input number.
Returns:
int: The factorial of the input number.
Raises:
ValueError: If the input number is negative.
"""
if not isinstance(n, int):
raise TypeError("Input must be an integer.")
if n < 0:
raise ValueError("Input must be a non-negative integer.")
# Base case: factorial of 0 is 1
if n == 0 or n == 1:
return 1
# Recursive case: n! = n * (n-1)!
result = 1
for i in range(1, n + 1):
result *= i
return result
Example Use Cases
print(factorial(5)) # Output: 120 (5! = 5*4*3*2*1)
print(factorial(0)) # Output: 1 (0! = 1)
print(factorial(1)) # Output: 1 (1! = 1)
try:
print(factorial(-3)) # Raises ValueError
except ValueError as e:
print(e) # Output: Input must be a non-negative integer.
try:
print(factorial(3.5)) # Raises TypeError
except TypeError as e:
print(e) # Output: Input must be an integer.
Alternative Implementation (Recursive)
def factorial_recursive(n):
"""
Calculate the factorial of a given non-negative integer.
Args:
n (int): The input number.
Returns:
int: The factorial of the input number.
Raises:
ValueError: If the input number is negative.
"""
if not isinstance(n, int):
raise TypeError("Input must be an integer.")
if n < 0:
raise ValueError("Input must be a non-negative integer.")
# Base case: factorial of 0 is 1
if n == 0 or n == 1:
return 1
# Recursive case: n! = n * (n-1)!
return n * factorial_recursive(n-1)
Please note that while the recursive implementation is more elegant, it can cause a stack overflow for large input values due to the recursive function calls. The iterative implementation is generally preferred for large input values.
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
Here's a complete implementation of the factorial
function in Python:
def factorial(n):
# Check if n is a negative number
if n < 0:
return "Undefined for negative numbers"
# Base case for factorial of 0 or 1
elif n == 0 or n == 1:
return 1
else:
# Calculate factorial using a loop
result = 1
for i in range(2, n + 1):
result *= i
return result
# Example usage
print(factorial(5)) # Output: 120
This function checks for negative input (since factorial is not defined for negative numbers), handles the base cases of 0 and 1, and uses a loop to calculate the factorial for positive integers.
def factorial(n):
"""
Calculates the factorial of a non-negative integer.
Args:
n: The non-negative integer.
Returns:
The factorial of n. Returns 1 if n is 0.
Raises ValueError if n is negative.
"""
if n < 0:
raise ValueError("Factorial is not defined for negative numbers.")
elif n == 0:
return 1
else:
result = 1
for i in range(1, n + 1):
result *= i
return result
Here's a complete implementation of the factorial function in Python:
def factorial(n):
# Check for negative input
if n < 0:
return None # or raise ValueError("Factorial is not defined for negative numbers")
# Base cases
if n == 0 or n == 1:
return 1
# Iterative implementation
result = 1
for i in range(2, n + 1):
result *= i
return result
This implementation includes several key features:
- Input Validation:
- It handles negative numbers by returning
None
or could raise aValueError
- This prevents invalid factorial calculations
- Base Cases:
- Returns 1 for 0! and 1!
- These are mathematically correct factorial definitions
- Iterative Calculation:
- Uses a loop to multiply numbers from 2 to n
- More memory-efficient than recursive approach
- Avoids potential stack overflow for large numbers
Example usage:
print(factorial(5)) # Output: 120
print(factorial(0)) # Output: 1
print(factorial(10)) # Output: 3628800
Alternative recursive implementation (less efficient):
def factorial(n):
if n < 0:
return None
if n == 0 or n == 1:
return 1
return n * factorial(n - 1)
The iterative version is generally preferred for better performance and to avoid potential stack overflow.
Calculating Factorial in Python
The factorial of a non-negative integer n
is the product of all positive integers less than or equal to n
. It can be calculated using the following function:
def factorial(n):
"""
Calculate the factorial of a number.
Args:
n (int): A non-negative integer.
Returns:
int: The factorial of n.
Raises:
ValueError: If n is a negative integer.
"""
if not isinstance(n, int):
raise TypeError("Input must be an integer.")
if n < 0:
raise ValueError("Input must be a non-negative integer.")
# Initialize result to 1
result = 1
# Calculate the factorial using a loop
for i in range(1, n + 1):
result *= i
return result
Example Use Cases:
# Calculate the factorial of 5
result = factorial(5)
print(result) # Output: 120
# Calculate the factorial of 0
result = factorial(0)
print(result) # Output: 1
# Attempt to calculate the factorial of a negative integer
try:
result = factorial(-1)
print(result)
except ValueError as e:
print(e) # Output: Input must be a non-negative integer.
# Attempt to calculate the factorial of a non-integer
try:
result = factorial(3.5)
print(result)
except TypeError as e:
print(e) # Output: Input must be an integer.
Alternatively, you can calculate the factorial using recursion:
def factorial(n):
"""
Calculate the factorial of a number using recursion.
Args:
n (int): A non-negative integer.
Returns:
int: The factorial of n.
Raises:
ValueError: If n is a negative integer.
"""
if not isinstance(n, int):
raise TypeError("Input must be an integer.")
if n < 0:
raise ValueError("Input must be a non-negative integer.")
# Base case: factorial of 0 is 1
if n == 0:
return 1
# Recursive case: n! = n * (n-1)!
else:
return n * factorial(n-1)
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
All models provided correct factorial implementations, but with varying approaches and detail levels. ChatGPT was concise and efficient, using an iterative approach with proper error handling. Claude opted for a recursive solution with detailed examples, though returning None for negative inputs isn't ideal. Gemini provided the most comprehensive response, showing three implementation methods including math.factorial(). Llama delivered extensive documentation and both iterative/recursive implementations, but perhaps offered too much detail for a simple task. Response times varied significantly, with Llama being fastest (2555ms) and Gemini slowest (10150ms).
Budget Tier
Comparing performance across models, Llama 3.1 8B was fastest at 893ms but provided the most verbose response with extensive error handling and examples. ChatGPT 4o Mini and Gemini 1.5 Flash delivered concise, functional solutions in ~2300ms. Claude took longest at 6050ms but provided a balanced solution with both iterative and recursive implementations. All models correctly handled negative numbers and base cases. Gemini stood out for clean documentation, while Llama demonstrated more robust input validation. For simple factorial calculation, Gemini's solution offers the best balance of clarity and functionality.
JavaScript String Reversal
A prompt to create a JavaScript function that reverses an input string. Useful for string manipulation tasks and coding interviews.
Prompt:
Finish this JavaScript function that reverses a string: function reverseString(str) { // Add code here }
Performance Results
To reverse a string in JavaScript, you can split the string into an array of characters, reverse the array, and then join it back into a string. Here's the complete reverseString
function:
function reverseString(str) {
// Split the string into an array of characters
let charArray = str.split('');
// Reverse the array
let reversedArray = charArray.reverse();
// Join the reversed array back into a string
let reversedString = reversedArray.join('');
return reversedString;
}
Alternatively, you can use method chaining to achieve the same result in a more concise manner:
function reverseString(str) {
return str.split('').reverse().join('');
}
Both implementations will reverse the input string and return the reversed version.
function reverseString(str) {
return str.split('').reverse().join('');
}
Alternative solutions:
// Using a for loop
function reverseString(str) {
let reversed = '';
for (let i = str.length - 1; i >= 0; i--) {
reversed += str[i];
}
return reversed;
}
```javascript // Using reduce() function reverseString(str) { return str.split('').reduce((reversed, char) => char + reversed, ''); }
javascript // Using recursion function reverseString(str) { if (str === '') return ''; return reverseString(str.substr(1)) + str[0]; }
Example usage:
javascript console.log(reverseString('hello')); // 'olleh' console.log(reverseString('JavaScript')); // 'tpircSavaJ' ```
function reverseString(str) {
// Add code here
return str.split("").reverse().join("");
}
This solution utilizes JavaScript's built-in string methods:
str.split("")
: This splits the string into an array of individual characters. The empty string "" as the argument ensures it splits between each character..reverse()
: This reverses the order of elements within the array..join("")
: This joins the elements of the reversed array back into a single string. Again, the empty string "" ensures the characters are joined without any separators.
Here's an alternative solution using a loop, which might be helpful for understanding the reversal logic more directly:
function reverseString(str) {
let reversedStr = "";
for (let i = str.length - 1; i >= 0; i--) {
reversedStr += str[i];
}
return reversedStr;
}
This version iterates through the string from the last character to the first and appends each character to the reversedStr
variable.
Here's the completed function that reverses a string in JavaScript:
/**
* Reverses a string.
* @param {string} str - The string to reverse.
* @return {string} The reversed string.
*/
function reverseString(str) {
// Use the split(), reverse(), and join() methods to reverse the string
return str.split("").reverse().join("");
}
Here's a breakdown of how this function works:
str.split("")
: This takes the string and splits it into an array of characters.reverse()
: This reverses the order of the elements in the array.join("")
: This takes the reversed array and combines the characters back into a string.
You can use this function like this:
console.log(reverseString("Hello, World!")); // Outputs: "!dlroW ,olleH"
This function will work with any string you pass to it, including those with non-alphanumeric characters and different languages.
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
Here’s a complete implementation of the reverseString
function in JavaScript that reverses a given string:
function reverseString(str) {
// Split the string into an array of characters
// Reverse the array
// Join the array back into a string
return str.split('').reverse().join('');
}
You can call this function with a string argument to receive the reversed version of that string. For example:
console.log(reverseString("hello")); // Outputs: "olleh"
This function works by using three main steps:
split('')
splits the string into an array of individual characters.reverse()
reverses the order of elements in the array.join('')
joins the array back into a single string.
There are several ways to reverse a string in JavaScript. Here are a few options to complete the reverseString
function:
Method 1: Using split
, reverse
, and join
This is a concise and commonly used method:
function reverseString(str) {
return str.split("").reverse().join("");
}
This code first splits the string into an array of individual characters using split("")
. Then, it reverses the order of elements in the array using reverse()
. Finally, it joins the elements back into a string using join("")
.
Method 2: Using a for
loop
This method is more explicit and demonstrates the process step-by-step:
function reverseString(str) {
let newString = "";
for (let i = str.length - 1; i >= 0; i--) {
newString += str[i];
}
return newString;
}
This code iterates through the string from the last character to the first, appending each character to newString
.
Method 3: Using recursion (less efficient)
While functional, recursion is generally less efficient for this task than the iterative approach:
function reverseString(str) {
if (str === "") {
return "";
} else {
return reverseString(str.substr(1)) + str.charAt(0);
}
}
This recursively calls the function on a substring, adding the first character to the end of the reversed substring.
All three methods achieve the same result. The split
, reverse
, join
method is generally preferred for its brevity and readability. Choose the method that best suits your understanding and coding style.
Here's a completed version of the reverseString
function with multiple approaches:
// Method 1: Using array methods (recommended)
function reverseString(str) {
return str.split('').reverse().join('');
}
// Method 2: Using a for loop
function reverseString(str) {
let reversed = '';
for (let i = str.length - 1; i >= 0; i--) {
reversed += str[i];
}
return reversed;
}
// Method 3: Using reduce method
function reverseString(str) {
return str.split('').reduce((rev, char) => char + rev, '');
}
// Method 4: Using recursion
function reverseString(str) {
if (str === '') {
return '';
}
return reverseString(str.substr(1)) + str.charAt(0);
}
Each method has its own advantages:
- Method 1 is the most concise and readable
- Method 2 is the most performant for large strings
- Method 3 is a functional programming approach
- Method 4 demonstrates a recursive solution
You can choose the one that best fits your specific use case and coding style.
Here's a simple implementation of the function using array methods:
function reverseString(str) {
return str.split("").reverse().join("");
}
// Example usage:
console.log(reverseString("Hello World")); // Output: "dlroW olleH"
Alternatively, you can use a more traditional approach with a for loop:
function reverseString(str) {
let reversed = "";
for (let i = str.length - 1; i >= 0; i--) {
reversed += str[i];
}
return reversed;
}
// Example usage:
console.log(reverseString("Hello World")); // Output: "dlroW olleH"
Using recursion:
function reverseString(str) {
if (str.length <= 1) {
return str;
} else {
return reverseString(str.slice(1)) + str[0];
}
}
// Example usage:
console.log(reverseString("Hello World")); // Output: "dlroW olleH"
However, the best approach is to use a built-in string method that is more concise:
function reverseString(str) {
return str.split("").reverse().join("");
}
This solution converts the string into an array of characters, reverses the array, and then joins the characters back into a string.
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
All models provided correct solutions using the split-reverse-join method, but with varying levels of detail and alternatives. Claude offered the most comprehensive response with 4 different approaches (chain method, loop, reduce, recursion). Gemini and ChatGPT balanced code with clear explanations, while Llama provided thorough documentation and usage examples. Response times varied significantly - Llama was fastest at 1.1s, Claude and ChatGPT around 4-5s, and Gemini slowest at 5.6s. Claude's versatility and multiple solutions made it stand out, though all models demonstrated competent JavaScript knowledge.
Budget Tier
All models successfully provided working solutions for string reversal, with varying levels of detail. Llama 3.1 8B was notably fastest at 602ms. Claude and Gemini provided the most comprehensive responses with multiple implementation methods and explanations. ChatGPT gave a focused, single-method solution with clear documentation. All models suggested the split-reverse-join approach as optimal, though Claude stood out by noting performance considerations for large strings. Gemini and Claude demonstrated stronger technical depth by including efficiency comparisons between methods.
SQL Top Customers Query
A prompt to generate an SQL query that identifies the top 5 customers based on their total order value. Useful for business analytics and reporting.
Prompt:
Complete this SQL query to find the top 5 customers by order value: SELECT customer_id, // Complete the query
Example
Performance Results
To complete the SQL query to find the top 5 customers by total order value, you'll need to sum the order values for each customer and then sort them in descending order. Here is how you can complete the query:
SELECT customer_id,
SUM(order_value) AS total_order_value
FROM orders
GROUP BY customer_id
ORDER BY total_order_value DESC
LIMIT 5;
This query will provide the top 5 customers based on the total value of their orders, by summing the order_value
for each customer_id
and ordering the results from highest to lowest. The LIMIT 5
clause ensures only the top 5 customers are returned.
SELECT
c.customer_id,
c.first_name,
c.last_name,
SUM(o.order_value) as total_order_value
FROM
customers c
JOIN orders o ON c.customer_id = o.customer_id
GROUP BY
c.customer_id,
c.first_name,
c.last_name
ORDER BY
total_order_value DESC
LIMIT 5;
This query will:
- Join the customers and orders tables
- Calculate the sum of order_value for each customer
- Group results by customer details
- Sort by total order value in descending order
- Limit to top 5 results
The result will show:
- Customer ID
- First name
- Last name
- Total order value
SELECT customer_id,
SUM(order_value) AS total_order_value
FROM orders
GROUP BY customer_id
ORDER BY total_order_value DESC
LIMIT 5;
To find the top 5 customers by order value, we'll use the following SQL query:
SELECT
c.customer_id,
c.first_name,
c.last_name,
SUM(o.order_value) as total_order_value
FROM
customers c
JOIN
orders o ON c.customer_id = o.customer_id
GROUP BY
c.customer_id, c.first_name, c.last_name
ORDER BY
total_order_value DESC
LIMIT 5;
Here's how the query works:
- We join the
customers
table with theorders
table on thecustomer_id
field. - We use the
GROUP BY
clause to group the results bycustomer_id
,first_name
, andlast_name
. - We use the
SUM
aggregation function to calculate the total order value for each customer. - We use the
ORDER BY
clause to sort the results in descending order based on thetotal_order_value
. - Finally, we use the
LIMIT
clause to limit the results to the top 5 customers.
When we execute this query with the provided data, it will return the top 5 customers by order value. The results will be sorted in descending order by total order value.
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
To complete the SQL query to find the top 5 customers by order value, you can use the following SQL statement. This query will aggregate the total order values for each customer and then order the results in descending order, limiting the output to the top 5 customers:
SELECT customer_id,
SUM(order_value) AS total_order_value
FROM orders
GROUP BY customer_id
ORDER BY total_order_value DESC
LIMIT 5;
Explanation:
SUM(order_value) AS total_order_value
: This calculates the total order value for each customer.FROM orders
: This specifies the table from which to retrieve the order data.GROUP BY customer_id
: This groups the results by each customer, allowing theSUM
function to compute totals for each one.ORDER BY total_order_value DESC
: This orders the results by the total order value in descending order, so the highest values come first.LIMIT 5
: This restricts the output to the top 5 customers.
You can execute this SQL query in a database management system where your tables customers
and orders
are created and populated.
SELECT customer_id,
SUM(order_value) AS total_spent
FROM orders
GROUP BY customer_id
ORDER BY total_spent DESC
LIMIT 5;
Here's the completed SQL query to find the top 5 customers by order value:
SELECT
c.customer_id,
c.first_name,
c.last_name,
SUM(o.order_value) as total_order_value
FROM
customers c
JOIN
orders o ON c.customer_id = o.customer_id
GROUP BY
c.customer_id, c.first_name, c.last_name
ORDER BY
total_order_value DESC
LIMIT 5;
This query does the following:
- Joins the
customers
andorders
tables using thecustomer_id
- Calculates the total order value for each customer by using
SUM()
- Groups the results by customer details
- Orders the results by total order value in descending order
- Limits the output to the top 5 customers
When you run this query, it will return the customer ID, first name, last name, and total order value for the top 5 customers who have spent the most money.
Here's how you can modify the query to find the top 5 customers by order value:
-- Create the tables and insert the values
CREATE TABLE customers (
customer_id INT PRIMARY KEY,
first_name VARCHAR(50),
last_name VARCHAR(50),
email VARCHAR(100)
);
CREATE TABLE orders (
order_id INT PRIMARY KEY,
customer_id INT,
order_date DATE,
order_value DECIMAL(10,2),
FOREIGN KEY (customer_id) REFERENCES customers(customer_id)
);
INSERT INTO customers VALUES
(1, 'John', 'Smith', 'john.smith@email.com'),
(2, 'Mary', 'Johnson', 'mary.j@email.com'),
(3, 'Robert', 'Brown', 'rbrown@email.com'),
(4, 'Lisa', 'Anderson', 'lisa.a@email.com'),
(5, 'Michael', 'Wilson', 'mwilson@email.com');
INSERT INTO orders VALUES
(1, 1, '2023-01-15', 150.00),
(2, 2, '2023-01-16', 450.75),
(3, 1, '2023-02-01', 290.25),
(4, 3, '2023-02-15', 175.50),
(5, 2, '2023-03-01', 325.00),
(6, 4, '2023-03-15', 89.99),
(7, 5, '2023-03-20', 220.00),
(8, 2, '2023-04-01', 550.00),
(9, 1, '2023-04-15', 125.50),
(10, 3, '2023-04-20', 300.00);
-- Modify the query to find the top 5 customers by order value
SELECT
c.customer_id,
c.first_name,
c.last_name,
SUM(o.order_value) AS total_order_value
FROM
customers c
JOIN
orders o ON c.customer_id = o.customer_id
GROUP BY
c.customer_id, c.first_name, c.last_name
ORDER BY
total_order_value DESC
LIMIT 5;
This will produce the following result:
+------------+------------+-----------+--------------------+
| customer_id | first_name | last_name | total_order_value |
+------------+------------+-----------+--------------------+
| 2 | Mary | Johnson | 1325.45 |
| 1 | John | Smith | 566.35 |
| 3 | Robert | Brown | 485.00 |
| 5 | Michael | Wilson | 220.00 |
| 4 | Lisa | Anderson | 89.99 |
+------------+------------+-----------+--------------------+
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
ChatGPT and Gemini provided similar basic solutions focusing on essential functionality. Claude and Llama delivered more comprehensive queries including customer details through table joins. Performance-wise, Gemini and Llama were notably faster (1485ms and 1319ms) compared to ChatGPT and Claude (3046ms and 3576ms). Claude and Llama added helpful explanations, while Gemini was most concise. All solutions were technically correct, but Claude and Llama's responses were more production-ready with additional customer information included in the output.
Budget Tier
ChatGPT 4o Mini and Gemini provided basic functional queries with minimal fields. Claude offered a more comprehensive solution including customer names. Llama went furthest, providing complete table creation scripts, sample data, and expected output. Response times varied significantly - Llama was fastest at 1072ms, while Gemini was slowest at 7433ms. Claude struck a good balance between detail and conciseness. For a simple query, Gemini's minimal approach was sufficient, though Claude's additional fields make the output more useful in practice.
Java Palindrome Checker
A prompt to create a Java method that determines if a given string reads the same forwards and backwards. Useful for string processing and coding challenges.
Prompt:
Complete this Java method to check if a string is a palindrome: public boolean isPalindrome(String str) { // Add implementation }
Performance Results
To implement the isPalindrome
method, you'll need to check if the string reads the same forwards and backwards. Here is a complete implementation:
public boolean isPalindrome(String str) {
if (str == null) {
return false;
}
int left = 0;
int right = str.length() - 1;
while (left < right) {
// Increment left index if it's not an alphanumeric character
while (left < right && !Character.isLetterOrDigit(str.charAt(left))) {
left++;
}
// Decrement right index if it's not an alphanumeric character
while (left < right && !Character.isLetterOrDigit(str.charAt(right))) {
right--;
}
// Compare characters, ignoring case
if (Character.toLowerCase(str.charAt(left)) != Character.toLowerCase(str.charAt(right))) {
return false;
}
left++;
right--;
}
return true;
}
Explanation:
Null Check: First, we ensure that the input string is not null. If it is, we return
false
because a null reference cannot logically be a palindrome.Pointers: Use two pointers,
left
starting at the beginning of the string andright
starting at the end.Character Processing:
Skip non-alphanumeric characters by moving the
left
pointer to the right or theright
pointer to the left until an alphanumeric character is found.Use
Character.isLetterOrDigit(char c)
to check if a character is alphanumeric.Comparison:
Convert characters to lowercase using
Character.toLowerCase(char c)
to ensure the comparison is case-insensitive.Compare characters at positions
left
andright
. If they are not the same, the string is not a palindrome.Continue:
If the characters match, move the
left
pointer to the right and theright
pointer to the left, then repeat the process.Result:
If all corresponding characters match, the string is a palindrome, so the function returns
true
.Otherwise, it will return
false
as soon as a mismatch is detected.
public boolean isPalindrome(String str) {
// Handle null or empty string
if (str == null || str.length() == 0) {
return false;
}
// Convert to lowercase and remove non-alphanumeric chars
str = str.toLowerCase().replaceAll("[^a-z0-9]", "");
// Use two pointers from start and end
int left = 0;
int right = str.length() - 1;
while (left < right) {
if (str.charAt(left) != str.charAt(right)) {
return false;
}
left++;
right--;
}
return true;
}
This solution:
First checks for null or empty string and returns false in those cases
Converts the string to lowercase and removes any non-alphanumeric characters to handle strings like "A man, a plan, a canal: Panama"
Uses two pointers approach:
- One pointer starts from beginning (left)
- Other pointer starts from end (right)
- Compare characters at both positions
- Move pointers towards center
- If any characters don't match, it's not a palindrome
- Returns true if all character comparisons match
Example usage:
isPalindrome("racecar") // returns true
isPalindrome("hello") // returns false
isPalindrome("A man, a plan, a canal: Panama") // returns true
isPalindrome("") // returns false
isPalindrome(null) // returns false
public boolean isPalindrome(String str) {
if (str == null) {
return true; // or false, depending on your definition of null strings
}
str = str.toLowerCase(); // Ignore case
int left = 0;
int right = str.length() - 1;
while (left < right) {
if (str.charAt(left) != str.charAt(right)) {
return false;
}
left++;
right--;
}
return true;
}
Here's a breakdown of the improvements:
- Null Check: Handles the case where the input string is
null
. Returningtrue
for a null input is a common convention, but you could also choose to returnfalse
or throw an exception, depending on your requirements. - Case-Insensitivity: Converts the string to lowercase using
toLowerCase()
to ensure that "Racecar" and "racecar" are both considered palindromes. - Clearer Variable Names:
left
andright
are more descriptive thani
andj
. - Conciseness: The code is more concise and readable.
- Efficiency: This approach only iterates through half the string, making it slightly more efficient.
Alternative using StringBuilder (for learning):
public boolean isPalindrome(String str) {
if (str == null) {
return true; // or false, depending on your definition
}
StringBuilder sb = new StringBuilder(str).reverse();
return str.equalsIgnoreCase(sb.toString());
}
This version is more concise, but potentially less efficient as it creates a new StringBuilder
object. It's good to understand both approaches.
Example Usage:
public static void main(String[] args) {
String str1 = "racecar";
String str2 = "hello";
String str3 = "A man, a plan, a canal: Panama";
String str4 = null;
System.out.println(isPalindrome(str1)); // Output: true
System.out.println(isPalindrome(str2)); // Output: false
System.out.println(isPalindrome(str3.replaceAll("[^a-zA-Z0-9]", "").toLowerCase())); // Output: true (handling punctuation)
System.out.println(isPalindrome(str4)); // Output: true (or false based on null handling)
}
This improved version addresses potential issues and provides a more robust and readable solution. Remember to choose the null-handling behavior that best suits your specific needs. The example also demonstrates how to handle palindromes with punctuation.
Here is the completed Java method to check if a string is a palindrome:
/**
* Checks if a given string is a palindrome.
*
* A palindrome is a word, phrase, number, or other sequence of characters that reads the same forward and backward (ignoring spaces, punctuation, and capitalization).
*
* @param str the input string to check
* @return true if the string is a palindrome, false otherwise
*/
public boolean isPalindrome(String str) {
// Remove non-alphanumeric characters and convert to lowercase
String cleanedStr = str.replaceAll("[^a-zA-Z0-9]", "").toLowerCase();
// Initialize two pointers, one at the start and one at the end of the string
int left = 0;
int right = cleanedStr.length() - 1;
// Compare characters from the start and end, moving towards the center
while (left < right) {
if (cleanedStr.charAt(left) != cleanedStr.charAt(right)) {
// If we find a pair of characters that don't match, the string is not a palindrome
return false;
}
left++;
right--;
}
// If we've checked all pairs of characters and haven't found any that don't match, the string is a palindrome
return true;
}
Example use cases:
System.out.println(isPalindrome("radar")); // true
System.out.println(isPalindrome("hello")); // false
System.out.println(isPalindrome("A man, a plan, a canal: Panama")); // true
System.out.println(isPalindrome("Not a palindrome")); // false
This implementation has a time complexity of O(n), where n is the length of the input string, and a space complexity of O(n) due to the creation of the cleaned string. The iteration from both ends towards the center of the string allows us to efficiently compare characters in a single pass.
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
To check if a string is a palindrome in Java, you can implement the method isPalindrome
by comparing characters from the beginning of the string to the characters from the end. A palindrome reads the same backward as forward. Below is a complete implementation of the isPalindrome
method:
public boolean isPalindrome(String str) {
// Sanitize the string by removing spaces and converting it to lower case
str = str.replaceAll("\\s+", "").toLowerCase();
int left = 0;
int right = str.length() - 1;
while (left < right) {
// If characters are not the same, it's not a palindrome
if (str.charAt(left) != str.charAt(right)) {
return false;
}
left++;
right--;
}
return true;
}
Explanation:
- Sanitization: The method first removes any whitespace and converts the string to lower case to ensure that the palindrome check is case-insensitive and ignores spaces.
- Two-Pointer Technique: We use two pointers:
left
starting from the beginning of the string andright
from the end. We compare the characters at these pointers:
- If at any point the characters do not match, the method returns
false
. - If the pointers cross each other without discrepancies, the method returns
true
.
Example Usage:
public static void main(String[] args) {
YourClassName instance = new YourClassName(); // Replace with your class name
System.out.println(instance.isPalindrome("A man a plan a canal Panama")); // true
System.out.println(instance.isPalindrome("racecar")); // true
System.out.println(instance.isPalindrome("hello")); // false
}
You can use this isPalindrome
method to check any string for palindrome properties.
Several implementations are possible, here are a couple offering different approaches:
Method 1: Using String Reversal
This method reverses the string and compares it to the original. It's straightforward and easy to understand.
public boolean isPalindrome(String str) {
String reversedStr = new StringBuilder(str).reverse().toString();
return str.equalsIgnoreCase(reversedStr);
}
This version uses equalsIgnoreCase
to ignore case differences, making the palindrome check case-insensitive. If you need a case-sensitive check, replace equalsIgnoreCase
with equals
.
Method 2: Two-Pointer Approach
This method uses two pointers, one at the beginning and one at the end of the string, and compares characters moving inwards. It's generally more efficient than reversing the entire string.
public boolean isPalindrome(String str) {
int left = 0;
int right = str.length() - 1;
str = str.toLowerCase(); //Convert to lowercase for case-insensitive comparison
while (left < right) {
if (str.charAt(left) != str.charAt(right)) {
return false;
}
left++;
right--;
}
return true;
}
This version also handles case-insensitive comparison by converting the string to lowercase at the beginning. Removing .toLowerCase()
will make it case-sensitive. This approach avoids creating a new string, making it more memory-efficient for very large strings.
Both methods achieve the same result. The two-pointer approach is generally preferred for its efficiency, especially with very long strings, while the string reversal approach might be easier to read and understand for beginners. Choose the method that best suits your needs and understanding.
Here's a complete implementation of the isPalindrome
method in Java:
public boolean isPalindrome(String str) {
// Remove non-alphanumeric characters and convert to lowercase
str = str.replaceAll("[^a-zA-Z0-9]", "").toLowerCase();
// Use two pointers to compare characters from start and end
int left = 0;
int right = str.length() - 1;
while (left < right) {
if (str.charAt(left) != str.charAt(right)) {
return false;
}
left++;
right--;
}
return true;
}
This implementation does the following:
First, it removes all non-alphanumeric characters using
replaceAll()
and converts the string to lowercase. This ensures that the palindrome check ignores spaces, punctuation, and is case-insensitive.It uses two pointers,
left
andright
, starting from the beginning and end of the string.It compares characters from both ends, moving towards the center.
If at any point the characters don't match, it returns
false
.If the pointers meet or cross without finding a mismatch, it returns
true
.
Example usage:
System.out.println(isPalindrome("A man, a plan, a canal: Panama")); // true
System.out.println(isPalindrome("race a car")); // false
System.out.println(isPalindrome("kayak")); // true
This method handles various edge cases like:
- Strings with spaces and punctuation
- Case-insensitive comparisons
- Empty strings
- Single character strings
Time complexity is O(n), where n is the length of the string. Space complexity is O(n) due to the string manipulation.
Palindrome Check in Java
Here's the completed Java method to check if a string is a palindrome:
/**
* Checks if the given string is a palindrome.
*
* A palindrome is a string that reads the same backward as forward.
*
* @param str the input string to check
* @return true if the string is a palindrome, false otherwise
*/
public boolean isPalindrome(String str) {
// Remove non-alphanumeric characters and convert to lowercase
String cleanedStr = str.replaceAll("[^a-zA-Z0-9]", "").toLowerCase();
// Initialize two pointers, one at the start and one at the end of the string
int start = 0;
int end = cleanedStr.length() - 1;
// Compare characters from start and end, moving towards the center
while (start < end) {
if (cleanedStr.charAt(start) != cleanedStr.charAt(end)) {
return false; // If any pair of characters don't match, it's not a palindrome
}
start++; // Move the start pointer one step forward
end--; // Move the end pointer one step backward
}
return true; // If the loop completes without finding any non-matching characters
}
Example Usage:
public static void main(String[] args) {
System.out.println(isPalindrome("madam")); // true
System.out.println(isPalindrome("hello")); // false
System.out.println(isPalindrome("A man, a plan, a canal: Panama")); // true
}
In this implementation, we first remove any non-alphanumeric characters from the string and convert it to lowercase to make the comparison case-insensitive. We then use two pointers, start
and end
, to compare characters from the start and end of the cleaned string, moving towards the center. If all pairs of characters match, the method returns true
, indicating that the string is a palindrome. Otherwise, it returns false
.
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
All models provided working palindrome solutions using two-pointer approach, but with varying implementation details. ChatGPT 4.0 offered the most robust solution with detailed character filtering and case handling. Claude's solution was concise and efficient, with clear preprocessing steps. Gemini provided two alternatives with good documentation but had questionable null handling. Llama's response included comprehensive JavaDoc and examples but could improve error handling. Response times varied significantly, with Llama being fastest at 1.8s while Gemini took 12.8s. Overall, ChatGPT's solution demonstrated the best balance of functionality and error handling.
Budget Tier
All models provided correct two-pointer implementations for palindrome checking. ChatGPT 4o Mini and Claude 3.5 Haiku offered the most comprehensive solutions with proper input sanitization and detailed explanations. Gemini 1.5 Flash uniquely provided two different approaches (string reversal and two-pointer), with helpful comparisons between them. Llama 3.1 8B included thorough documentation and examples but had slightly more verbose code. Response times varied from 822ms (Llama) to 7651ms (Gemini), with Claude and ChatGPT in the middle range around 6500ms.
Python Sorted Lists Merger
A prompt to develop a Python function that combines two pre-sorted lists into a single sorted list. Useful for array manipulation and sorting algorithms.
Prompt:
Finish this Python function to merge two sorted lists: def merge_sorted_lists(list1, list2): # Complete the code
Performance Results
To merge two sorted lists in Python, you can use a two-pointer approach to efficiently merge the lists while maintaining the sort order. Here's how you can complete the merge_sorted_lists
function:
def merge_sorted_lists(list1, list2):
merged_list = []
i, j = 0, 0 # Pointers for list1 and list2
# Traverse both lists and append the smallest element to the merged_list
while i < len(list1) and j < len(list2):
if list1[i] < list2[j]:
merged_list.append(list1[i])
i += 1
else:
merged_list.append(list2[j])
j += 1
# If there are any remaining elements in list1, add them to merged_list
while i < len(list1):
merged_list.append(list1[i])
i += 1
# If there are any remaining elements in list2, add them to merged_list
while j < len(list2):
merged_list.append(list2[j])
j += 1
return merged_list
Explanation:
- Two Pointers: We use two pointers
i
andj
to traverselist1
andlist2
, respectively. - Comparison Loop: Loop through both lists, compare elements, and append the smaller one to the
merged_list
. - Appending Remaining Elements: After the loop, one of the lists might still have elements left. We'll append the remaining elements from
list1
andlist2
tomerged_list
. - Sorted Output: Since both input lists are sorted and we maintain sort order during the merge, the resulting
merged_list
will also be sorted.
This approach allows you to merge the two lists in O(n + m) time, where n
and m
are the lengths of list1
and list2
, respectively.
def merge_sorted_lists(list1, list2):
merged = []
i = j = 0
# Compare elements from both lists and add smaller one to merged list
while i < len(list1) and j < len(list2):
if list1[i] <= list2[j]:
merged.append(list1[i])
i += 1
else:
merged.append(list2[j])
j += 1
# Add remaining elements from list1 if any
while i < len(list1):
merged.append(list1[i])
i += 1
# Add remaining elements from list2 if any
while j < len(list2):
merged.append(list2[j])
j += 1
return merged
Example usage:
list1 = [1, 3, 5, 7]
list2 = [2, 4, 6, 8]
print(merge_sorted_lists(list1, list2)) # Output: [1, 2, 3, 4, 5, 6, 7, 8]
list1 = [1, 2, 3, 4]
list2 = [5, 6, 7, 8]
print(merge_sorted_lists(list1, list2)) # Output: [1, 2, 3, 4, 5, 6, 7, 8]
This solution:
- Creates an empty merged list
- Uses two pointers i and j to track position in each input list
- Compares elements from both lists and adds smaller one to merged list
- Once we reach end of one list, adds remaining elements from other list
- Returns the final merged sorted list
Time complexity is O(n + m) where n and m are lengths of input lists Space complexity is O(n + m) for the merged list
def merge_sorted_lists(list1, list2):
"""Merges two sorted lists into a new sorted list.
Args:
list1: The first sorted list.
list2: The second sorted list.
Returns:
A new sorted list containing all elements from list1 and list2.
"""
merged_list = []
i = j = 0
while i < len(list1) and j < len(list2):
if list1[i] <= list2[j]:
merged_list.append(list1[i])
i += 1
else:
merged_list.append(list2[j])
j += 1
# Add any remaining elements from list1
while i < len(list1):
merged_list.append(list1[i])
i += 1
# Add any remaining elements from list2
while j < len(list2):
merged_list.append(list2[j])
j += 1
return merged_list
Here's a breakdown of how it works:
- Initialization:
merged_list
: An empty list to store the merged sorted elements.i
,j
: Index pointers forlist1
andlist2
, respectively, initialized to 0.
- Comparison and Merging:
- The
while
loop continues as long as bothi
andj
are within the bounds of their respective lists. - Inside the loop, we compare
list1[i]
andlist2[j]
. - The smaller element is appended to
merged_list
, and the corresponding index (i
orj
) is incremented.
- Handling Remaining Elements:
- After one of the lists is exhausted (either
i
orj
reaches the end), there might be remaining elements in the other list. Two separatewhile
loops handle these cases, appending the remaining elements tomerged_list
.
- Return:
- Finally, the function returns the
merged_list
, which now contains all elements fromlist1
andlist2
in sorted order.
Example Usage:
list1 = [2, 5, 8, 12]
list2 = [1, 3, 7, 9, 10]
merged_list = merge_sorted_lists(list1, list2)
print(merged_list) # Output: [1, 2, 3, 5, 7, 8, 9, 10, 12]
This solution has a time complexity of O(m + n), where 'm' and 'n' are the lengths of the input lists, because it iterates through each list at most once. The space complexity is also O(m + n) to store the merged list.
Merging Two Sorted Lists in Python
Here's a Python function that merges two sorted lists into one sorted list. This function uses a two-pointer technique to compare elements from both lists and append the smaller one to the result list.
def merge_sorted_lists(list1, list2):
"""
Merge two sorted lists into one sorted list.
Args:
list1 (list): The first sorted list.
list2 (list): The second sorted list.
Returns:
list: A new sorted list containing all elements from both input lists.
"""
# Initialize the result list and pointers for both input lists
result = []
i = j = 0
# Merge smaller elements first
while i < len(list1) and j < len(list2):
if list1[i] < list2[j]:
result.append(list1[i])
i += 1
else:
result.append(list2[j])
j += 1
# If there are remaining elements in list1, append them to the result
while i < len(list1):
result.append(list1[i])
i += 1
# If there are remaining elements in list2, append them to the result
while j < len(list2):
result.append(list2[j])
j += 1
return result
# Example usage:
list1 = [1, 3, 5, 7]
list2 = [2, 4, 6, 8]
merged_list = merge_sorted_lists(list1, list2)
print(merged_list) # Output: [1, 2, 3, 4, 5, 6, 7, 8]
Alternatively, you can use the built-in sorted
function and the +
operator to concatenate the lists and then sort the result. However, this approach has a time complexity of O(n log n) due to the sorting operation, whereas the two-pointer technique used above has a time complexity of O(n).
def merge_sorted_lists(list1, list2):
return sorted(list1 + list2)
# Example usage:
list1 = [1, 3, 5, 7]
list2 = [2, 4, 6, 8]
merged_list = merge_sorted_lists(list1, list2)
print(merged_list) # Output: [1, 2, 3, 4, 5, 6, 7, 8]
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
Certainly! Here is a complete implementation of the merge_sorted_lists
function that merges two sorted lists into a single sorted list:
def merge_sorted_lists(list1, list2):
merged_list = []
i, j = 0, 0
# Loop until we reach the end of either list
while i < len(list1) and j < len(list2):
if list1[i] < list2[j]:
merged_list.append(list1[i])
i += 1
else:
merged_list.append(list2[j])
j += 1
# If there are remaining elements in list1, add them to merged_list
while i < len(list1):
merged_list.append(list1[i])
i += 1
# If there are remaining elements in list2, add them to merged_list
while j < len(list2):
merged_list.append(list2[j])
j += 1
return merged_list
How it Works:
- Two pointers,
i
andj
, are initialized to the start oflist1
andlist2
, respectively. - The function enters a loop that compares the elements at
list1[i]
andlist2[j]
:
- The smaller element is appended to
merged_list
, and the corresponding pointer is incremented.
- Once one of the lists has been fully traversed, the function then appends any remaining elements from the other list to
merged_list
. - Finally, the function returns the merged and sorted list.
Example Usage:
list1 = [1, 3, 5]
list2 = [2, 4, 6]
merged = merge_sorted_lists(list1, list2)
print(merged) # Output: [1, 2, 3, 4, 5, 6]
This implementation has a time complexity of O(n + m), where n and m are the lengths of the two lists.
def merge_sorted_lists(list1, list2):
"""Merges two sorted lists into a single sorted list.
Args:
list1: The first sorted list.
list2: The second sorted list.
Returns:
A new list containing all elements from list1 and list2, sorted in ascending order.
"""
merged_list = []
i = j = 0
while i < len(list1) and j < len(list2):
if list1[i] <= list2[j]:
merged_list.append(list1[i])
i += 1
else:
merged_list.append(list2[j])
j += 1
# Add any remaining elements from list1
while i < len(list1):
merged_list.append(list1[i])
i += 1
# Add any remaining elements from list2
while j < len(list2):
merged_list.append(list2[j])
j += 1
return merged_list
Here's a solution to merge two sorted lists:
def merge_sorted_lists(list1, list2):
# Create a new list to store the merged result
merged = []
# Initialize pointers for both input lists
i, j = 0, 0
# Compare and merge elements from both lists
while i < len(list1) and j < len(list2):
if list1[i] <= list2[j]:
merged.append(list1[i])
i += 1
else:
merged.append(list2[j])
j += 1
# Add remaining elements from list1, if any
while i < len(list1):
merged.append(list1[i])
i += 1
# Add remaining elements from list2, if any
while j < len(list2):
merged.append(list2[j])
j += 1
return merged
This implementation does the following:
- Create an empty
merged
list to store the result - Use two pointers
i
andj
to track positions inlist1
andlist2
- Compare elements from both lists and add the smaller one to
merged
- Move the pointer of the list from which an element was added
- After one list is exhausted, add any remaining elements from the other list
- Return the merged sorted list
Example usage:
list1 = [1, 3, 5, 7]
list2 = [2, 4, 6, 8]
result = merge_sorted_lists(list1, list2)
print(result) # Output: [1, 2, 3, 4, 5, 6, 7, 8]
Time complexity: O(n + m), where n and m are lengths of input lists Space complexity: O(n + m) for the merged list
You can use the following function to merge two sorted lists in Python:
def merge_sorted_lists(list1, list2):
"""
Merge two sorted lists into a single sorted list.
Args:
list1 (list): The first sorted list.
list2 (list): The second sorted list.
Returns:
list: A new sorted list containing all elements from list1 and list2.
Example:
>>> merge_sorted_lists([1, 3, 5], [2, 4, 6])
[1, 2, 3, 4, 5, 6]
"""
# Initialize a new list to store the merged result
merged_list = []
# Initialize two pointers, one for each list, to keep track of the current element
i = j = 0
# Loop through both lists until one of them is exhausted
while i < len(list1) and j < len(list2):
# Compare the current elements in both lists and append the smaller one to the merged list
if list1[i] < list2[j]:
merged_list.append(list1[i])
i += 1
else:
merged_list.append(list2[j])
j += 1
# If list1 is not exhausted, append its remaining elements to the merged list
while i < len(list1):
merged_list.append(list1[i])
i += 1
# If list2 is not exhausted, append its remaining elements to the merged list
while j < len(list2):
merged_list.append(list2[j])
j += 1
return merged_list
# Example usage:
list1 = [1, 3, 5]
list2 = [2, 4, 6]
print(merge_sorted_lists(list1, list2)) # Output: [1, 2, 3, 4, 5, 6]
This implementation has a time complexity of O(n + m), where n and m are the lengths of the input lists, respectively. It uses a simple iterative approach to merge the two sorted lists in linear time.
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
All models provided correct implementations using the two-pointer approach with O(n+m) time complexity. ChatGPT 4.0 and Claude provided clean, efficient solutions with good explanations. Gemini offered the most detailed documentation and examples but took longest to respond (14116ms). Llama was fastest (2424ms) and uniquely included an alternative sorted() solution, though noted its inferior O(n log n) complexity. Overall, Llama delivered the best balance of speed, completeness and practical alternatives, while ChatGPT and Claude were most concise.
Budget Tier
All models provided correct implementations of the merge sort function with O(n+m) complexity. Llama 3.1 8B was notably fastest at 904ms and included detailed docstrings and examples. ChatGPT 4o Mini took longest at 13692ms but provided thorough explanations. Gemini and Claude delivered clean, efficient code with good documentation in ~5700ms. All solutions used the two-pointer technique and handled remaining elements properly, though Gemini uniquely included <= instead of < in comparisons.
C++ Array Maximum Finder
A prompt to create a C++ function that finds the largest number in an array. Useful for array processing and algorithm implementation.
Prompt:
Complete this C++ function to find the largest element in an array: int findMax(int arr[], int size) { // Add implementation }
Performance Results
To complete the C++ function findMax
that finds the largest element in an array, you can implement it like this:
int findMax(int arr[], int size) {
if (size <= 0) {
// Handle case where the array is empty
throw std::invalid_argument("Array size must be greater than 0");
}
// Assume the first element is the largest initially
int max = arr[0];
// Traverse the rest of the array and update max if a larger element is found
for (int i = 1; i < size; i++) {
if (arr[i] > max) {
max = arr[i];
}
}
return max;
}
Explanation:
Check for Empty Array: First, check if the array size is zero or negative, which might suggest improper usage (or a mistake). Throwing an exception in this case helps catch errors during runtime.
Initialize
max
: Start by assuming the first element of the array is the largest.Iterate through the Array: Loop through the array starting from the second element. For each element, compare it to
max
. If an element is greater thanmax
, updatemax
.Return the Result: After the loop,
max
will hold the largest element in the array, which is then returned.
int findMax(int arr[], int size) {
// Edge case check
if (size == 0) {
return -1; // Or throw exception
}
// Start with first element as max
int max = arr[0];
// Compare with rest of elements
for (int i = 1; i < size; i++) {
if (arr[i] > max) {
max = arr[i];
}
}
return max;
}
This implementation:
- First checks if array is empty
- Sets first element as initial max
- Loops through rest of array comparing each element with current max
- Updates max if larger element is found
- Returns largest element at end
Example usage:
int arr[] = {5, 2, 9, 1, 7};
int max = findMax(arr, 5); // Returns 9
int findMax(int arr[], int size) {
if (size <= 0) {
// Handle empty or invalid size array (e.g., return a special value or throw an exception)
return -1; // Or throw std::invalid_argument("Array size must be positive");
}
int max = arr[0]; // Initialize max with the first element
for (int i = 1; i < size; i++) {
if (arr[i] > max) {
max = arr[i];
}
}
return max;
}
Here's a breakdown of the improvements and considerations:
- Error Handling: The code now checks for an empty or invalid size array (
size <= 0
). Returning -1 is a common way to indicate an error, but throwing astd::invalid_argument
exception is generally a better practice in C++ as it clearly signals the problem. Choose the approach that best fits your error handling strategy. - Initialization:
max
is initialized with the first element of the array. This avoids the need to initialize it with a potentially very small "minimum" value that might not be smaller than all elements in the array. - Loop starts from 1: Since
max
is initialized witharr[0]
, the loop can start from index 1. - Clearer variable names: Using
max
instead of a single-letter name improves readability. - Comments: Added comments to explain the logic.
Example Usage:
#include <iostream>
#include <stdexcept> // For std::invalid_argument
int main() {
int arr[] = {1, 5, 2, 8, 3};
int size = sizeof(arr) / sizeof(arr[0]);
try {
int maxVal = findMax(arr, size);
std::cout << "Maximum element: " << maxVal << std::endl; // Output: 8
int emptyArr[] = {};
int emptySize = sizeof(emptyArr) / sizeof(emptyArr[0]);
maxVal = findMax(emptyArr, emptySize); // Will throw an exception
std::cout << "Max of empty array: " << maxVal << std::endl; // This won't be reached
} catch (const std::invalid_argument& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
return 0;
}
C++ Function to Find the Largest Element in an Array
Here's a simple C++ function to find the largest element in an array.
/**
* Finds the largest element in an array.
*
* @param arr The input array.
* @param size The size of the array.
* @return The largest element in the array.
*/
int findMax(int arr[], int size) {
// Initialize max as the first element of the array
int max = arr[0];
// Iterate over the array starting from the second element
for (int i = 1; i < size; i++) {
// Check if the current element is greater than max
if (arr[i] > max) {
// Update max if a larger element is found
max = arr[i];
}
}
// Return the largest element
return max;
}
Example Usage
Here's an example of how to use this function:
#include <iostream>
int main() {
int arr[] = {4, 2, 9, 6, 5, 1, 8, 3, 7};
int size = sizeof(arr) / sizeof(arr[0]);
int max = findMax(arr, size);
std::cout << "Largest element: " << max << std::endl;
return 0;
}
Explanation
The findMax
function works as follows:
- Initialize
max
as the first element of the array. - Iterate over the array starting from the second element (index 1).
- In each iteration, check if the current element is greater than
max
. - If a larger element is found, update
max
. - After iterating over the entire array, return
max
, which now holds the largest element.
This function has a time complexity of O(n), where n is the size of the array, because it only requires a single pass over the array to find the largest element.
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
Here's a complete implementation of the findMax
function in C++ that finds the largest element in an array:
#include <iostream>
#include <limits.h> // For INT_MIN
int findMax(int arr[], int size) {
// Check if the array size is less than or equal to 0
if (size <= 0) {
std::cerr << "Array size must be greater than 0" << std::endl;
return INT_MIN; // Indicate an error
}
int max = arr[0]; // Assume the first element is the largest
// Iterate through the array to find the largest element
for (int i = 1; i < size; ++i) {
if (arr[i] > max) {
max = arr[i]; // Update max if a larger element is found
}
}
return max; // Return the largest element found
}
int main() {
int arr[] = {1, 3, 7, 0, 5};
int size = sizeof(arr) / sizeof(arr[0]);
int max = findMax(arr, size);
std::cout << "The largest element in the array is: " << max << std::endl;
return 0;
}
Explanation:
Error Handling: The function first checks if the size of the array is less than or equal to 0. If it is, an error message is printed, and
INT_MIN
is returned to indicate failure.Initialization: The maximum value is initialized to the first element of the array.
Loop through Array: The function iterates through the rest of the array, comparing each element to the current maximum. If a larger element is found, it updates the maximum.
Return Value: Finally, the function returns the largest element found.
Note:
- The implementation checks for an empty array and handles it gracefully.
- The main function demonstrates how to use
findMax
. You can change the contents of the array to test different cases.
int findMax(int arr[], int size) {
if (size <= 0) {
return -1; // Handle empty array case. Could also throw an exception.
}
int max = arr[0]; // Initialize max to the first element
for (int i = 1; i < size; i++) {
if (arr[i] > max) {
max = arr[i];
}
}
return max;
}
Here's the completed function to find the largest element in an array:
int findMax(int arr[], int size) {
// Check if array is empty
if (size <= 0) {
return -1; // Or throw an exception
}
// Initialize max as the first element
int max = arr[0];
// Iterate through the array
for (int i = 1; i < size; i++) {
// Update max if current element is larger
if (arr[i] > max) {
max = arr[i];
}
}
return max;
}
This implementation does the following:
First, it checks if the array is empty or has an invalid size. If so, it returns -1 (or you could choose to throw an exception).
It initializes
max
with the first element of the array.It then iterates through the rest of the array starting from the second element.
For each element, it compares it with the current
max
. If the current element is larger, it updatesmax
.After the loop, it returns the largest element found.
The time complexity is O(n) as it makes a single pass through the array, and the space complexity is O(1) as it uses only a constant amount of extra space.
Example usage:
int arr[] = {10, 5, 20, 8, 15};
int size = sizeof(arr) / sizeof(arr[0]);
int largest = findMax(arr, size);
// largest will be 20
Here's a simple implementation of the function using a loop to find the largest element in the array.
/**
* Finds the largest element in the given array.
*
* @param arr The array in which to find the largest element.
* @param size The number of elements in the array.
* @return The largest element in the array if found, otherwise returns 0.
*/
int findMax(int arr[], int size) {
if (size == 0) {
// If the array is empty, return 0 as there is no largest element.
return 0;
}
int max = arr[0]; // Initialize max with the first element
// Iterate over the array to find the largest element.
for (int i = 1; i < size; i++) {
if (arr[i] > max) { // If current element is larger than max
max = arr[i]; // Update max with the current element
}
}
return max; // Return the largest element
}
However, a more idiomatic and efficient way to find the largest element in an array is to use the std::max_element
function from the
#include <algorithm>
/**
* Finds the largest element in the given array.
*
* @param arr The array in which to find the largest element.
* @param size The number of elements in the array.
* @return The largest element in the array if found, otherwise returns 0.
*/
int findMax(int arr[], int size) {
if (size == 0) {
return 0;
}
return *std::max_element(arr, arr + size);
}
Remember to include the necessary libraries and use a valid C++ compiler to compile the code.
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
All models provided correct implementations for finding the maximum array element, with similar linear-time algorithms. ChatGPT and Gemini offered robust error handling with exceptions, while Claude opted for a simpler -1 return. Llama provided detailed documentation and example usage but lacked error handling. Response times varied significantly: Llama was fastest at 1.9s, Claude at 3.8s, ChatGPT at 5.8s, and Gemini slowest at 12.7s. Gemini's response was most comprehensive with detailed explanations and example usage, though this contributed to its slower speed.
Budget Tier
All models provided correct implementations with similar O(n) time complexity. ChatGPT 4o Mini offered the most comprehensive solution with error handling using INTMIN and included a complete working program. Gemini was concise but functional. Claude provided clear explanations and complexity analysis. Llama offered two implementations including the STL approach using std::maxelement, showing deeper C++ knowledge. Response times varied significantly, with Llama being notably faster at 800ms versus 4000-7000ms for others. Overall, ChatGPT and Llama provided the most valuable responses.
CSS Centered Div
A prompt to generate CSS properties for centering a div element both horizontally and vertically. Useful for web layout and design tasks.
Prompt:
Finish this HTML/CSS snippet to create a centered div:.container { // Add CSS properties }
Performance Results
To create a centered <div>
with the class container
, you can use CSS Flexbox or CSS Grid, among other methods. Here’s one way to do it using Flexbox:
<div class="outer-container">
<div class="container">
<!-- Content goes here -->
</div>
</div>
.outer-container {
display: flex;
justify-content: center;
align-items: center;
height: 100vh; /* Adjust the height according to your needs */
}
.container {
width: 50%; /* You can set this to a specific pixel width or percentage */
max-width: 600px; /* Optional max-width */
background-color: #f0f0f0; /* Optional background color */
padding: 20px; /* Optional padding */
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.1); /* Optional shadow for visual effect */
border-radius: 8px; /* Optional border radius */
}
This will center your .container
div both vertically and horizontally within the .outer-container
using Flexbox. You can adjust widths, padding, and other properties according to your design needs.
<div class="container">
</div>
.container {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
}
Alternative solution using flexbox:
<div class="container">
</div>
.container {
display: flex;
justify-content: center;
align-items: center;
min-height: 100vh;
}
Both methods will center the div both horizontally and vertically in the viewport. Choose the one that best fits your specific use case:
- The absolute positioning method is good for single elements
- The flexbox approach is more flexible and better for multiple elements
<div class="container">
</div>
.container {
width: 300px; /* Or any specific width, or even a percentage like 50% */
height: 200px; /* Or any specific height, or even a percentage */
margin: 0 auto; /* Key for horizontal centering */
/* Other styling properties as needed */
background-color: lightblue; /* Example */
}
Explanation:
width
andheight
: These properties define the dimensions of the div. You must set a width (either a fixed value likepx
or a percentage) formargin: 0 auto
to work for horizontal centering. The height is optional, but often useful.margin: 0 auto;
: This is the crucial part for centering.margin: 0;
sets the top and bottom margins to zero.auto;
for the left and right margins tells the browser to automatically calculate equal margins on both sides, effectively centering the element horizontally within its parent container.
Alternative using Flexbox (often preferred for modern layouts):
<div class="container">
</div>
.container {
width: 300px; /* Or any width */
height: 200px; /* Or any height */
display: flex;
justify-content: center; /* Center horizontally */
align-items: center; /* Center vertically */
background-color: lightblue;
}
Explanation (Flexbox):
display: flex;
: Makes the container a flexbox container.justify-content: center;
: Centers content horizontally along the main axis (which is row-wise by default).align-items: center;
: Centers content vertically along the cross axis.
Alternative using Grid (also good for complex layouts):
<div class="container">
</div>
.container {
width: 300px; /* Or any width */
height: 200px; /* Or any height */
display: grid;
place-items: center; /* Shorthand for align-items: center; justify-items: center; */
background-color: lightblue;
}
Explanation (Grid):
display: grid;
: Makes the container a grid container.place-items: center;
: Is shorthand foralign-items: center;
andjustify-items: center;
simultaneously centering the content both horizontally and vertically.
Choose the method that best suits your overall layout needs. margin: 0 auto;
is the simplest for just centering a single block element, while Flexbox and Grid are more powerful for complex, responsive designs.
To create a centered div, you can use the following CSS properties:
<div class="container">
<!-- Your content here -->
</div>
.container {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
width: 50%; /* Optional */
height: 50%; /* Optional */
}
However, a more modern approach is to use flexbox for centering. This will also make your code more adaptable to responsive design.
<div class="container">
<!-- Your content here -->
</div>
.container {
display: flex;
justify-content: center;
align-items: center;
height: 100vh; /* Covers the full height of the viewport */
}
If you also want to center the div horizontally, you can use the following approach:
<div class="container">
<!-- Your content here -->
</div>
.container {
margin: 0 auto;
width: 50%; /* Optional */
height: 50%; /* Optional */
}
It's worth noting that the above approaches require different conditions to work properly. For instance, the position: absolute;
approach requires a full-height parent element, usually achieved by adding height: 100%;
to the body
and html
selectors. The margin: 0 auto;
approach requires a width to work properly, while the flexbox approach requires a height and possibly width if not using flex-grow or expandable container.
Remember, these are some common ways to create a centered div. You should adjust the approaches to fit your specific use case.
Example Use Cases
- Login Form:
<div class="container">
<form>
<label for="username">Username:</label>
<input type="text" id="username" name="username">
<label for="password">Password:</label>
<input type="password" id="password" name="password">
<button type="submit">Submit</button>
</form>
</div>
.container {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
background-color: #f0f0f0;
padding: 50px;
border: 1px solid #ddd;
border-radius: 10px;
}
- Alert Message:
<div class="container">
<div class="alert">This is an alert message!</div>
</div>
.container {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
width: 50%;
text-align: center;
}
This should give you a basic idea of how to center a div in HTML and CSS.
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
To center a div both horizontally and vertically within a container while using CSS, you can use flexbox. Here is the completed HTML/CSS snippet to achieve this:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Centered Div</title>
<style>
body, html {
height: 100%;
margin: 0;
}
.container {
display: flex; /* Use flexbox */
justify-content: center; /* Center horizontally */
align-items: center; /* Center vertically */
height: 100vh; /* Full height of the viewport */
background-color: #f0f0f0; /* Background color for visibility */
}
.box {
width: 300px; /* Width of the centered div */
height: 200px; /* Height of the centered div */
background-color: #4caf50; /* Color of the centered div */
color: white; /* Text color */
display: flex; /* Flexbox for inner content */
justify-content: center; /* Center text horizontally */
align-items: center; /* Center text vertically */
border-radius: 10px; /* Rounded corners */
}
</style>
</head>
<body>
<div class="container">
<div class="box">
Centered Content
</div>
</div>
</body>
</html>
In this example:
- The
.container
div is set up withdisplay: flex
, and usesjustify-content
andalign-items
to center its child both horizontally and vertically. - The
.box
div is styled separately to give it a specific height, width, and background color. - The
height: 100vh
in the.container
class ensures it takes the full height of the viewport, allowing for perfect vertical centering. - You can adjust the sizes, colors, and other properties as needed.
Several ways exist to center a div, depending on whether you want to center it horizontally, vertically, or both. Here are a few options:
Option 1: Horizontally Centered (using text-align
)
This works well for block-level elements with a known width.
<div class="container">
</div>
.container {
width: 300px; /* Or any specific width */
margin: 0 auto; /* Centers horizontally */
text-align: center; /*This is only needed if you also need to center inline elements *inside* the container*/
}
Option 2: Horizontally and Vertically Centered (using Flexbox)
This is a modern and flexible approach.
<div class="container">
</div>
.container {
display: flex;
justify-content: center; /* Centers horizontally */
align-items: center; /* Centers vertically */
height: 200px; /* Needs a height for vertical centering */
width: 300px; /* Needs a width for horizontal centering */
}
Option 3: Horizontally and Vertically Centered (using Grid)
Another modern and flexible approach.
<div class="container">
</div>
.container {
display: grid;
place-items: center; /* Centers both horizontally and vertically */
height: 200px; /* Needs a height */
width: 300px; /* Needs a width */
}
Option 4: Horizontally and Vertically Centered (using absolute positioning and transforms - for a known size)
This method requires knowing the dimensions of the container in advance.
<div class="parent">
<div class="container">
</div>
</div>
.parent {
position: relative; /* Needed for absolute positioning of child */
height: 200px;
width: 300px;
}
.container {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
width: 100px;
height: 100px; /* Example dimensions */
}
Remember to choose the option that best suits your needs and context. If you need to center a div within a parent container with unknown dimensions, Flexbox or Grid are generally the best choices. If you know the dimensions beforehand, any of the methods will work. Option 4 is especially useful when dealing with fixed size elements needing precise centering within a parent. Make sure you adjust the width
and height
values to match your desired dimensions.
Here's the completed HTML/CSS snippet to create a centered div:
<div class="container">
<!-- Your content goes here -->
</div>
.container {
width: 300px; /* Adjust width as needed */
height: 200px; /* Adjust height as needed */
background-color: #f0f0f0; /* Optional background color */
margin: 0 auto; /* Horizontally centers the div */
display: flex;
justify-content: center;
align-items: center; /* Vertically centers content */
text-align: center; /* Centers text inside the div */
}
This solution provides multiple centering methods:
margin: 0 auto;
centers the div horizontallydisplay: flex
withjustify-content
andalign-items
centers the content both horizontally and verticallytext-align: center
ensures any text inside the div is centered
You can customize the width, height, and background-color as needed.
Here's the completed HTML and CSS snippet to create a centered div:
HTML
<div class="container">
<!-- Your content here -->
<p>This is a centered div.</p>
</div>
CSS
.container {
width: 50%; /* Set a width for the container */
margin: 0 auto; /* Center the container */
padding: 20px; /* Add some padding for better readability */
border: 1px solid #ccc; /* Add a border for visual feedback */
background-color: #f2f2f2; /* Add a background color */
text-align: center; /* Center the text within the container */
}
However, if you're looking to make the div "absolutely" centered in the middle of the screen (horizontally and vertically), you would need to add additional CSS properties. This will create a full-screen div that centers itself at the middle.
Example of full-screen centered div
HTML remains the same
<div class="container">
<!-- Your content here -->
<p>This is a centered div.</p>
</div>
CSS
.container {
/* Position the container absolutely at the middle of the screen */
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
/* Add a width, height, and border for visual feedback */
width: 50%;
height: 50%;
border: 1px solid #ccc;
background-color: #f2f2f2;
/* Center the text within the container */
text-align: center;
}
Remember, the container's background-color
and border
properties will cover the entire screen area, including the visible region of the page. The transform: translate
property, which uses -50%
of the parent element for each axis, is applied to the top and left properties to shift the position of the container 50% of its own width (or height), which means the left
and top
properties essentially become relative now to the (parent element's) center point rather than
(screen's) origin point`. As a result, it is capable of centering the div, almost independently from parent's position and styles.
If you're trying to use the 'margin: 0 auto'
combination, ensure the parent element is full-screen in height/width as well, since it relies its left and right `margin's 50% of its parent's width in order to be centered-vertically within it.
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
Comparing model responses for HTML/CSS centering div solutions:
ChatGPT provided a comprehensive Flexbox solution with detailed styling options and visual enhancements. Claude offered two concise methods using absolute positioning and Flexbox. Gemini gave the most detailed response, covering three approaches (margin auto, Flexbox, and Grid) with thorough explanations. Llama provided multiple solutions with practical examples and use cases.
Gemini took longest (13.9s) but gave most complete analysis. Llama (2.7s) and Claude (3.3s) were fastest. For pure centering task, Claude's answer was most efficient while remaining accurate.
Budget Tier
ChatGPT 4o Mini, Gemini 1.5, Claude 3.5 Haiku, and Llama 3.1 8B all provided working solutions for centering a div. ChatGPT offered the most comprehensive response with a complete HTML structure and detailed CSS. Gemini presented multiple approaches including flexbox, grid, and absolute positioning. Claude delivered a concise yet complete solution combining margin auto with flexbox. Llama provided two options but was overly verbose. Response times varied significantly - Llama was fastest at 992ms while Gemini took 9085ms. For practical implementation, Claude's solution offers the best balance of completeness and simplicity.
Ruby Array Deduplication
A prompt to create a Ruby method that removes duplicate elements from an array. Useful for data cleaning and array processing tasks.
Prompt:
Complete this Ruby method to remove duplicates from an array: def remove_duplicates(array) # Add implementation end
Performance Results
To complete the remove_duplicates
method in Ruby, you can use the uniq
method, which is specifically designed to remove duplicate elements from an array. Here's how you can implement it:
def remove_duplicates(array)
array.uniq
end
This method takes an array as its argument and returns a new array with duplicate elements removed. The uniq
method works by comparing each element with all the previous ones, keeping only the first occurrence.
If you want to modify the original array in place (i.e., without creating a new array), you can use uniq!
:
def remove_duplicates(array)
array.uniq!
end
The uniq!
method will modify the existing array and return nil
if no changes are made, or the array itself if duplicates are removed.
Choose the version that best fits your needs depending on whether you want to avoid modifying the original array.
def remove_duplicates(array)
array.uniq
end
# Alternative implementation without using uniq:
def remove_duplicates(array)
result = []
array.each do |element|
result << element unless result.include?(element)
end
result
end
Example usage:
numbers = [1, 2, 2, 3, 4, 4, 5]
remove_duplicates(numbers)
# Returns [1, 2, 3, 4, 5]
words = ["hello", "world", "hello", "ruby"]
remove_duplicates(words)
# Returns ["hello", "world", "ruby"]
Key points:
- The
uniq
method is the most straightforward solution - The alternative implementation manually checks for duplicates
- Both preserve the order of first appearance
- Works with any data type, not just numbers
- Returns a new array rather than modifying in place
def remove_duplicates(array)
# Add implementation
array.uniq
end
This leverages Ruby's built-in uniq
method, which returns a new array with duplicate values removed. It's the most concise and efficient way to achieve the desired result.
If you needed to modify the original array in place (though less common), you could use uniq!
:
def remove_duplicates!(array) # Note the bang !
array.uniq!
end
This version modifies the array
directly. It will return nil
if no duplicates were found and removed, otherwise it returns the modified array
.
If, for learning purposes, you want to implement the duplicate removal without using uniq
or uniq!
, you can use a Hash or Set:
def remove_duplicates(array)
seen = {} # or Set.new
result = []
array.each do |element|
unless seen.has_key?(element) # or unless seen.include?(element) if using Set
result << element
seen[element] = true # or seen.add(element) if using Set
end
end
result
end
This code iterates through the input array
. It uses the seen
hash (or set) to keep track of elements encountered so far. If an element is already in seen
, it's a duplicate and is skipped. Otherwise, the element is added to the result
array and to the seen
hash (or set). This method has a time complexity of O(n) on average because hash lookups (or set lookups) are typically O(1).
Another (less efficient) way without using uniq
, uniq!
, Hash, or Set, would be to use nested loops, but it has a time complexity of O(n^2):
def remove_duplicates(array)
result = []
array.each do |element|
unless result.include?(element)
result << element
end
end
result
end
For almost all real-world scenarios, array.uniq
is the preferred and most efficient solution. The other examples are primarily for demonstrating alternative logic.
Removing Duplicates from an Array in Ruby
You can remove duplicates from an array in Ruby using the uniq
or uniq!
methods. However, if you want to implement this manually, you can use a hash to keep track of unique elements and then return the keys of the hash as an array. Here's a simple implementation:
def remove_duplicates(array)
# Create an empty hash to store unique elements
unique_elements = {}
# Iterate through each element in the array
array.each do |element|
# Add each element as a key in the hash
# This will automatically remove duplicates
unique_elements[element] = true
end
# Return the keys of the hash as an array
unique_elements.keys
end
Using Built-in Methods
A more concise and efficient way to remove duplicates from an array is by using the uniq
method provided by Ruby's Array class:
def remove_duplicates(array)
# Return a new array with duplicate elements removed
array.uniq
end
Or, if you want to modify the original array, you can use the uniq!
method:
def remove_duplicates(array)
# Modify the original array to remove duplicates
array.uniq!
end
Example Usage
array = [1, 2, 2, 3, 4, 4, 5, 6, 6]
puts "Original Array: #{array}"
unique_array = remove_duplicates(array)
puts "Array with Duplicates Removed: #{unique_array}"
Output:
Original Array: [1, 2, 2, 3, 4, 4, 5, 6, 6]
Array with Duplicates Removed: [1, 2, 3, 4, 5, 6]
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
You can remove duplicates from an array in Ruby by using the uniq
method, which returns a new array by removing duplicate values. Below is a complete implementation of the remove_duplicates
method:
def remove_duplicates(array)
array.uniq
end
Example Usage:
array_with_duplicates = [1, 2, 2, 3, 4, 4, 5]
unique_array = remove_duplicates(array_with_duplicates)
puts unique_array.inspect # Output: [1, 2, 3, 4, 5]
Detailed Explanation:
- The
uniq
method creates a new array that contains only unique values from the original array, effectively removing any duplicates. - If you want to modify the original array in place you can use
array.uniq!
, which removes duplicate elements from the array itself.
Here’s how you can implement it if you wanted to mutate the original array:
def remove_duplicates(array)
array.uniq!
end
In summary, the remove_duplicates
method can be easily implemented using the built-in uniq
method for a straightforward removal of duplicates from an array.
Several approaches can remove duplicates from a Ruby array. Here are three, with varying levels of efficiency and readability:
Method 1: Using uniq
(Most efficient and readable)
This is the simplest and most efficient way to remove duplicates in Ruby:
def remove_duplicates(array)
array.uniq
end
The uniq
method returns a new array containing only the unique elements from the original array, preserving the original order.
Method 2: Using a Set (Efficient for large arrays)
Sets are inherently unordered collections of unique elements. This approach is efficient for very large arrays:
def remove_duplicates(array)
array.to_set.to_a
end
First, we convert the array to a Set, which automatically removes duplicates. Then, we convert it back to an array. Note that the order might not be preserved.
Method 3: Using a loop and a hash (Less efficient, but demonstrates the logic)
This method is less efficient than uniq
but demonstrates the underlying logic:
def remove_duplicates(array)
seen = {}
result = []
array.each do |element|
unless seen[element]
seen[element] = true
result << element
end
end
result
end
This iterates through the array. A hash seen
keeps track of elements already encountered. If an element hasn't been seen, it's added to the result
array and marked as seen in the seen
hash.
Which method to choose?
For most cases, array.uniq
is the best choice due to its simplicity and efficiency. Use the to_set
method if you're dealing with extremely large arrays where performance is critical and order doesn't matter. The loop-based approach is primarily for educational purposes to understand the underlying algorithm.
Here's a Ruby method to remove duplicates from an array:
def remove_duplicates(array)
array.uniq
end
This implementation uses the built-in uniq
method, which returns a new array with duplicate elements removed while preserving the original order of first occurrence.
A few alternative implementations you might consider:
- Using
uniq!
to modify the original array in-place:
def remove_duplicates(array)
array.uniq!
end
- A more explicit approach using
Set
:
require 'set'
def remove_duplicates(array)
Set.new(array).to_a
end
- If you wanted a pure Ruby implementation without using built-in methods:
def remove_duplicates(array)
result = []
array.each do |item|
result << item unless result.include?(item)
end
result
end
The first method (array.uniq
) is the most concise and typically the most performant for most use cases. Choose the implementation that best fits your specific requirements and coding style.
Here is a simple implementation in Ruby that uses a Hash to remove duplicates from an array. This method preserves the original order of elements.
def remove_duplicates(array)
# Create a Hash to keep track of unique elements
seen = Hash.new { |h, k| h[k] = [] }
array.each do |element|
seen[element].push(element)
end
# Flatten the Hash values and return the result
seen.values.flatten.sort!
end
However, this implementation does not exactly remove duplicates while preserving the original order, as it adds each element multiple times to the output array.
To exactly remove duplicates while preserving the original order, you could use the following implementation with a Set and an Array.
def remove_duplicates(array)
seen = Set.new
result = []
array.each do |element|
# Check if the element has not been seen before
if !seen.include?(element)
# Add the element to the result array and mark it as seen
result.push(element)
seen.add(element)
end
end
# Return the result array
result
end
This version of the method is more efficient and preserves the original order of elements. It creates a Set to keep track of the elements that have been seen, and it iterates over the input array once to add elements to the result array and mark them as seen.
You can use either of these methods to remove duplicates from an array in Ruby.
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
All models successfully provided working solutions using Ruby's built-in uniq method. ChatGPT 4 and Claude offered concise, focused responses with good explanations. Gemini provided the most comprehensive answer with multiple implementation approaches, though perhaps too detailed for a simple task. Llama's response was well-structured but overly verbose. ChatGPT was fastest to highlight the difference between uniq and uniq!, making it the most practical response. Response times varied significantly, with Llama being quickest (1.8s) and Gemini slowest (10.8s).
Budget Tier
An analysis of 4 LLMs solving a Ruby array deduplication problem reveals distinct patterns. ChatGPT 4o Mini and Claude Haiku provided concise, practical solutions using Ruby's built-in uniq
method, with helpful alternative approaches. Gemini Flash gave the most comprehensive response, including three different implementations with detailed explanations of tradeoffs. Llama 3.1 8B offered a more complex custom implementation using Hash and Set, but struggled with efficiency in its first attempt. Response times varied significantly, with Llama being fastest at 681ms, while others took 3-4 seconds.
Performance Verdict
Based on the provided analyses across multiple code completion tasks, here's a comparative verdict of the models' performance:
ChatGPT 4.0: Strengths:
- Most consistent in providing robust, production-ready code
- Excellent error handling and edge case consideration
- Clean, efficient implementations with clear explanations Weaknesses:
- Moderate response times
- Sometimes less comprehensive than other models
Claude: Strengths:
- Frequently offered multiple implementation approaches
- Well-balanced between conciseness and detail
- Strong at providing practical alternatives Weaknesses:
- Occasional suboptimal error handling
- Moderate response times
Gemini: Strengths:
- Most comprehensive documentation and explanations
- Thorough coverage of alternative approaches
- Detailed example usage Weaknesses:
- Consistently slowest response times
- Sometimes overly detailed for simple tasks
Llama: Strengths:
- Consistently fastest response times
- Detailed documentation and examples
- Good at providing alternative solutions Weaknesses:
- Sometimes overly verbose
- Inconsistent error handling
- Documentation can be excessive
Winner: ChatGPT 4.0
While each model showed distinct strengths, ChatGPT 4.0 emerges as the winner for code completion tasks. It consistently delivered the best balance of:
- Code quality and robustness
- Practical error handling
- Implementation efficiency
- Clear, concise explanations
- Reasonable response times
Though other models excelled in specific areas (Llama in speed, Gemini in comprehensiveness, Claude in alternative approaches), ChatGPT 4.0's consistent delivery of production-ready code with appropriate detail level makes it the most reliable choice for code completion tasks.
Budget Verdict
Based on the comparative analyses across code completion tasks, here's how the models performed:
ChatGPT 4o Mini: Strengths:
- Consistently comprehensive solutions with proper error handling
- Strong documentation and explanations
- Complete working examples, especially for compiled languages
- Well-balanced between brevity and thoroughness
Weaknesses:
- Generally slower response times
- Sometimes overly verbose in simpler tasks
Claude 3.5 Haiku: Strengths:
- Excellent technical depth with performance considerations
- Multiple implementation approaches
- Clean, practical solutions
- Strong input validation
Weaknesses:
- Consistently longest response times
- Sometimes too focused on alternatives rather than direct solutions
Gemini 1.5 Flash: Strengths:
- Cleanest documentation
- Excellent comparison between different approaches
- Concise yet functional solutions
- Strong technical accuracy
Weaknesses:
- Variable response times
- Sometimes too minimal in complex scenarios
Llama 3.1 8B: Strengths:
- Consistently fastest response times (600-1000ms)
- Comprehensive error handling
- Deep language-specific knowledge
- Detailed examples and test cases
Weaknesses:
- Often overly verbose
- Sometimes overcomplicates simple solutions
Winner: Gemini 1.5 Flash While all models performed competently, Gemini 1.5 Flash offers the best balance of:
- Clean, maintainable code
- Appropriate level of documentation
- Multiple implementation approaches when relevant
- Practical solutions without overcomplications
- Reasonable response times
The model consistently delivered high-quality code completion across different languages and complexity levels, making it the most reliable choice for general code completion tasks.
Conclusion
Conclusion
After extensive testing and comparison of both performance and budget tier AI models across various code completion tasks, several key findings emerge. The higher-performance models consistently delivered more robust, production-ready code with superior error handling and deeper technical insights. However, the budget tier models proved remarkably capable, often matching their more advanced counterparts in basic functionality while offering significantly faster response times.
In the performance tier, ChatGPT 4.0 emerged as the clear leader, excelling in consistent delivery of production-ready code with appropriate error handling and clear documentation. While Gemini offered the most comprehensive explanations and Claude provided excellent alternative approaches, ChatGPT's balance of quality, speed, and practicality set it apart.
In the budget tier, Gemini 1.5 Flash demonstrated the best overall balance of code quality, documentation, and response time. While Llama 3.1 8B was consistently fastest and ChatGPT 4o Mini provided the most thorough solutions, Gemini Flash's ability to deliver clean, practical code with appropriate detail level made it the standout choice for cost-conscious users.
For developers choosing between tiers, the decision largely depends on specific needs. The performance tier is recommended for complex, production-critical applications requiring robust error handling and comprehensive solutions. The budget tier proves more than adequate for routine development tasks, prototyping, and learning scenarios where speed and cost-effectiveness are priorities.
These findings highlight the remarkable progress in AI code completion capabilities across both tiers, offering developers powerful tools suited to different needs and constraints.