Code Translation
Code Generation and Analysis with LLMs. Comparing the leading AI models side-by-side at Code Translation.
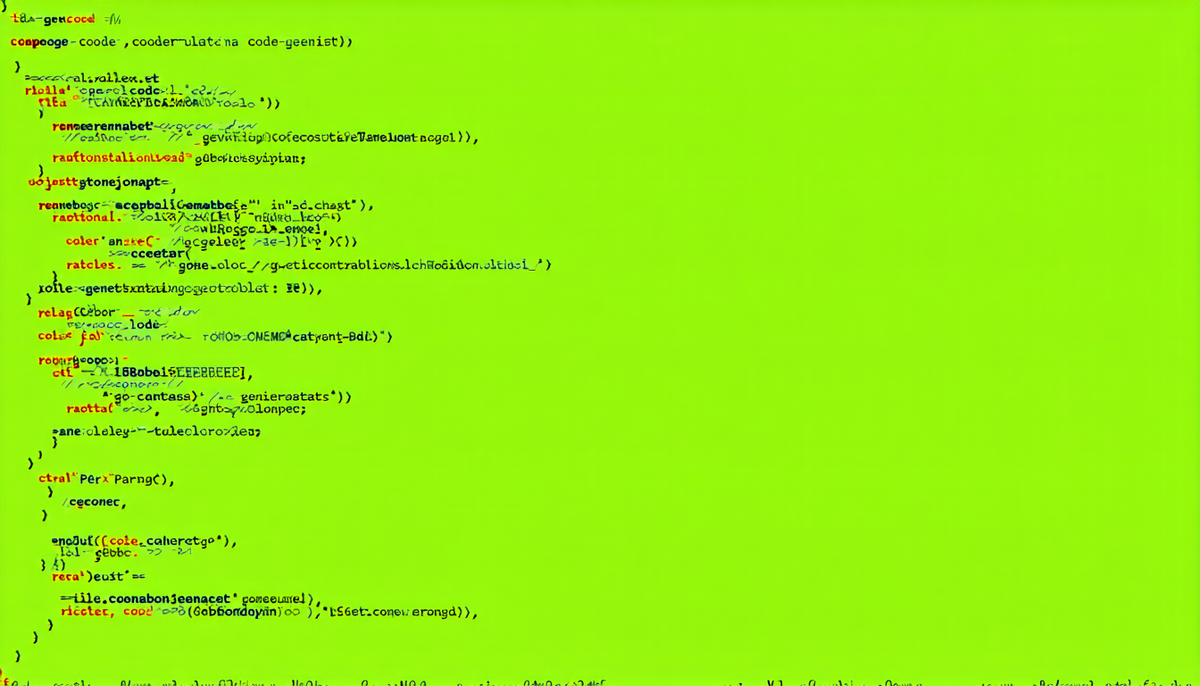
Comparing the leading AI models:
Category: Code Generation and Analysis
Subcategory: Code Translation
Contents
- Introduction
- Code Translation
- Prompts
- Python to JavaScript Factorial Function
- C++ to Java Bubble Sort
- JavaScript to Python Palindrome Check
- SQL to MongoDB Query Translation
- Ruby to Python Fibonacci Function
- Java to C# Binary Search
- Python List Comprehension to JavaScript
- Go to Rust QuickSort
- TypeScript Interface to Python Dataclass
- Python Decorator to JavaScript Property
- Performance Verdict
- Budget Verdict
- Conclusion
Introduction
Comparing AI Models: A Guide to LLM Performance vs Cost
Looking to compare AI models but unsure which large language model best suits your needs? This comprehensive guide compares leading AI models side-by-side across two distinct tiers, helping you make informed decisions about which AI assistant to use for different tasks.
Budget Tier Models:
- ChatGPT 4.0 Mini
- Gemini 1.5 Flash
- Claude 3.5 Haiku
- Llama 3.1 8B
Performance Tier Models:
- ChatGPT 4.0
- Claude 3.5 Sonnet
- Gemini 1.5 Pro
- Llama 3.1 70B
Understanding how different AI models perform on specific tasks is crucial for maximizing efficiency and cost-effectiveness. Budget tier models excel at straightforward tasks like basic content creation, simple analysis, and general question-answering. Choose these models when cost is a primary concern and tasks don't require deep reasoning or specialized expertise.
Performance tier models shine in complex scenarios requiring nuanced understanding, creative problem-solving, or technical expertise. These models are ideal for professional applications, advanced analysis, and tasks demanding high accuracy and sophisticated outputs.
Through direct AI model comparisons across various use cases and prompts, this guide helps you identify which tier and specific model best matches your requirements, ensuring optimal results while managing costs effectively.
50+ AI models with one subscription. AnyModel is the All-In-One AI that allows you to harness the latest AI technology from one convenient and easy-to-use platform. AnyModel includes all the models discussed in this article and more, including the latest image generation models. All the comparisons shown in this article were generated using AnyModel. Sign up for a free trial here.
Code Translation
Code translation has emerged as one of the most practical and powerful applications of large language models, allowing developers to seamlessly convert code between different programming languages while maintaining functionality and logic. LLMs excel at this task because they've been trained on vast repositories of code across multiple languages, enabling them to recognize common patterns, syntax structures, and programming paradigms. Their ability to understand both the semantic meaning and technical implementation of code makes them particularly effective at producing accurate translations that preserve the original code's intent and efficiency. This capability not only saves developers significant time but also reduces the learning curve when working with unfamiliar languages, facilitates codebase migrations, and enables easier collaboration between teams using different technologies. As LLMs continue to evolve, their code translation abilities have become increasingly sophisticated, handling complex functions, maintaining proper error handling, and even optimizing code for the target language's specific features and best practices.
Prompts
Python to JavaScript Factorial Function
Convert a recursive factorial function from Python's concise syntax to JavaScript's function syntax. Useful for developers transitioning between Python and JavaScript codebases.
Prompt:
Translate this Python code to JavaScript: def factorial(n): return 1 if n == 0 else n * factorial(n-1)
Performance Results
Here's the JavaScript equivalent of the Python factorial
function:
function factorial(n) {
return n === 0 ? 1 : n * factorial(n - 1);
}
This JavaScript function uses a similar approach with a ternary operator to handle the base case and the recursive case.
function factorial(n) {
return n === 0 ? 1 : n * factorial(n - 1);
}
Example usage:
console.log(factorial(5)); // 120
console.log(factorial(0)); // 1
console.log(factorial(3)); // 6
The JavaScript version uses:
- A ternary operator (
?:
) instead of Python'sif/else
- Strict equality (
===
) instead of Python's==
- Function declaration with
function
keyword instead ofdef
function factorial(n) {
return n === 0 ? 1 : n * factorial(n - 1);
}
This uses the ternary operator (condition ? value_if_true : value_if_false
) which is equivalent to Python's conditional expression (value_if_true if condition else value_if_false
). It provides a concise way to express the same logic.
Alternatively, you could use a more traditional if/else
structure:
function factorial(n) {
if (n === 0) {
return 1;
} else {
return n * factorial(n - 1);
}
}
Both versions accomplish the same goal. The ternary operator version is generally preferred for its brevity when the logic is simple, like in this case.
Here's the JavaScript equivalent of the given Python code:
/**
* Calculates the factorial of a given number.
*
* @param {number} n The number for which the factorial is to be calculated.
* @returns {number} The factorial of n.
*/
function factorial(n) {
// If n is 0, return 1 (base case)
if (n === 0) {
return 1;
} else {
// Otherwise, recursively call factorial with n-1 and multiply by n
return n * factorial(n - 1);
}
}
However, this code can be written more concisely using JavaScript's conditional (ternary) operator:
function factorial(n) {
// If n is 0, return 1; otherwise, recursively call factorial with n-1 and multiply by n
return n === 0 ? 1 : n * factorial(n - 1);
}
You can use this function like this:
console.log(factorial(5)); // Outputs: 120
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
Here is the JavaScript translation of the given Python code for calculating the factorial of a number:
function factorial(n) {
return n === 0 ? 1 : n * factorial(n - 1);
}
This code defines a function named factorial
that takes a number n
as an argument and returns the factorial of that number using recursion, just like the Python version.
There are several ways to translate the given Python code to JavaScript. Here are two options:
Option 1: Using a recursive function (most direct translation):
function factorial(n) {
if (n === 0) {
return 1;
} else {
return n * factorial(n - 1);
}
}
This is a direct equivalent of the Python code, using recursion. It's concise and easy to understand if you're familiar with recursion. However, recursive functions can be less efficient for large values of n
due to potential stack overflow errors.
Option 2: Using an iterative approach (more efficient for large numbers):
function factorial(n) {
let result = 1;
for (let i = 2; i <= n; i++) {
result *= i;
}
return result;
}
This iterative version avoids recursion. It's generally more efficient for larger values of n
because it doesn't rely on the call stack. It initializes result
to 1 and then iteratively multiplies it by each number from 2 up to n
. The loop starts at 2 because multiplying by 1 is unnecessary.
Both options achieve the same result, calculating the factorial of a given non-negative integer. The iterative approach is generally preferred for its better performance with larger inputs. Choose the option that best suits your needs and understanding.
Here's the equivalent JavaScript code for the Python factorial function:
function factorial(n) {
return n === 0 ? 1 : n * factorial(n - 1);
}
The translation preserves the recursive nature of the original function:
- It uses a ternary operator (
?:
) instead of Python'sif-else
- The comparison uses strict equality (
===
) in JavaScript - The function signature and recursive logic remain essentially the same
- It will calculate the factorial of a non-negative integer by recursively multiplying the number with the factorial of (n-1)
You can use it the same way as the Python version, like this:
console.log(factorial(5)); // Will output 120
Here's the equivalent JavaScript code for the given Python function:
/**
* Calculates the factorial of a given number.
*
* @param {number} n The number to calculate the factorial for.
* @returns {number} The factorial of the given number.
*/
function factorial(n) {
if (n === 0) {
return 1;
} else {
return n * factorial(n - 1);
}
}
// Example usage:
console.log(factorial(5)); // Output: 120
However, it's worth noting that JavaScript doesn't support function recursion limit by default, which means you could potentially cause a stack overflow if the factorial is passed a very large number.
A safer approach is to use a loop to calculate the factorial, like this:
/**
* Calculates the factorial of a given number using a loop.
*
* @param {number} n The number to calculate the factorial for.
* @returns {number} The factorial of the given number.
*/
function factorial(n) {
let result = 1;
for (let i = 2; i <= n; i++) {
result *= i;
}
return result;
}
// Example usage:
console.log(factorial(5)); // Output: 120
Another safer approach would be to use a function that calculates the factorial using "memoization" (caching) of previously calculated results, which prevents the function from re-calculating the factorial for the same number multiple times:
/**
* Calculates the factorial of a given number using memoization.
*
* @param {number} n The number to calculate the factorial for.
* @param {object} memoCache Optional memoization cache object.
* @returns {number} The factorial of the given number.
*/
function factorial(n, memoCache = {}) {
if (n < 0) {
throw new Error("Factorial is not defined for negative numbers");
}
if (n < 2) {
return 1;
}
if (n in memoCache) {
return memoCache[n];
}
const result = n * factorial(n - 1, memoCache);
memoCache[n] = result;
return result;
}
// Example usage:
console.log(factorial(5)); // Output: 120
This version of the function uses an optional memoization cache object as the second argument, and caches the results of previously calculated factorials in that object. This way, if the function is called again with the same number, it will return the cached result instead of re-calculating it.
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
All models successfully translated the Python factorial function to JavaScript, with response times ranging from 1.2s (Llama) to 5s (Gemini). ChatGPT provided a minimal correct solution using the ternary operator. Claude added useful example usage cases. Gemini offered both ternary and if/else implementations with explanations. Llama provided the most comprehensive response with JSDoc comments, both implementations, and example usage. While all solutions were correct, Llama's response stood out for documentation quality, though it had the longest output. Response speed favored Llama and ChatGPT over Claude and Gemini.
Budget Tier
All models successfully translated the Python factorial function to JavaScript, with response times ranging from 953ms (Llama) to 3360ms (Claude). ChatGPT and Claude provided concise, ternary operator implementations. Gemini and Llama went beyond with multiple implementation options and detailed explanations. Llama stood out with the fastest response time and most comprehensive solution, including JSDoc comments, error handling, and three different implementations (recursive, iterative, and memoized). While all translations were correct, Llama's response demonstrated superior technical depth and practical considerations.
C++ to Java Bubble Sort
Transform a C++ implementation of bubble sort into Java syntax. Helpful for developers porting sorting algorithms between C++ and Java.
Prompt:
Convert this C++ function to Java: void bubbleSort(int arr[], int n) { for(int i=0; iarr[j+1]) swap(arr[j],arr[j+1]); }
Performance Results
Certainly! Below is the equivalent bubble sort function in Java:
public class BubbleSort {
public static void bubbleSort(int[] arr) {
int n = arr.length;
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
// Swap arr[j] and arr[j+1]
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
// For testing the bubble sort function
public static void main(String[] args) {
int[] arr = {64, 34, 25, 12, 22, 11, 90};
bubbleSort(arr);
System.out.println("Sorted array:");
for (int value : arr) {
System.out.print(value + " ");
}
}
}
This Java code defines a bubbleSort
method that sorts an array of integers using the bubble sort algorithm. The main
method provides an example of how to use the bubbleSort
function, printing out the sorted array.
public static void bubbleSort(int[] arr) {
int n = arr.length;
for (int i = 0; i < n-1; i++) {
for (int j = 0; j < n-i-1; j++) {
if (arr[j] > arr[j+1]) {
// Swap arr[j] and arr[j+1]
int temp = arr[j];
arr[j] = arr[j+1];
arr[j+1] = temp;
}
}
}
}
Key changes made:
- Added
public static
modifiers - Changed array parameter syntax from
arr[]
toarr[]
- Removed size parameter
n
since Java arrays know their length - Used
arr.length
instead ofn
- Implemented swap inline since Java doesn't have a built-in swap function
import java.util.Arrays;
class Solution {
/**
* Sorts an array of integers using the bubble sort algorithm.
*
* @param arr The array to be sorted.
* @param n The size of the array. Should be arr.length. Included for closer parity to the C++ signature.
*/
public void bubbleSort(int[] arr, int n) {
if (arr == null || n <= 0 || n > arr.length ) {
return; // Or throw an exception for invalid input
}
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
swap(arr, j, j + 1);
}
}
}
}
private void swap(int[] arr, int i, int j) {
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
// Example usage (for testing):
public static void main(String[] args) {
Solution s = new Solution();
int[] arr = {64, 34, 25, 12, 22, 11, 90};
s.bubbleSort(arr, arr.length);
System.out.println(Arrays.toString(arr)); // Output: [11, 12, 22, 25, 34, 64, 90]
// Test with null or empty
int[] arr2 = null;
s.bubbleSort(arr2, 0); // Should handle gracefully (does nothing currently)
int[] arr3 = {};
s.bubbleSort(arr3, 0); // Should handle gracefully (does nothing currently)
// Test n being larger than array length
int[] arr4 = {1,2};
s.bubbleSort(arr4, 5); // Should handle gracefully (does nothing currently)
}
}
Key Changes and Improvements:
swap
helper function: Instead of inlining the swap logic, a separateswap
method is created for better readability and maintainability. This is standard practice in Java.- Input Validation: Added a check at the beginning of
bubbleSort
to handlenull
arrays or invalid sizes (e.g.,n
less than or equal to 0, orn
greater thanarr.length
). This prevents potentialNullPointerException
orArrayIndexOutOfBoundsException
errors. The current implementation simply returns; you could also throw anIllegalArgumentException
if that's more appropriate for your use case. - Example Usage: Included a
main
method to demonstrate how to use thebubbleSort
function and to showcase the input validation. - Clarity and Comments: Improved comments and formatting for better understanding.
This Java version is more robust and idiomatic than a direct port of the C++ code. It adheres to Java's best practices and handles potential edge cases.
Java Equivalent of C++ Bubble Sort Function
Here is the Java equivalent of the provided C++ function:
public class Main {
/**
* Swaps two elements in an array.
*
* @param arr the array containing the elements to be swapped
* @param i the index of the first element
* @param j the index of the second element
*/
public static void swap(int[] arr, int i, int j) {
// Create a temporary variable to store the value at index i
int temp = arr[i];
// Assign the value at index j to the index i
arr[i] = arr[j];
// Assign the value stored in the temporary variable to index j
arr[j] = temp;
}
/**
* Sorts an array using the bubble sort algorithm.
*
* @param arr the array to be sorted
*/
public static void bubbleSort(int[] arr) {
int n = arr.length;
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
swap(arr, j, j + 1);
}
}
}
}
public static void main(String[] args) {
// Example usage:
int[] arr = {5, 2, 8, 1, 3};
System.out.println("Before sorting: ");
for (int num : arr) {
System.out.print(num + " ");
}
bubbleSort(arr);
System.out.println("\nAfter sorting: ");
for (int num : arr) {
System.out.print(num + " ");
}
}
}
Key differences between C++ and Java implementation:
- In Java, unlike C++, the length of an array can be directly obtained by the
length
property (arr.length
), eliminating the need for a separaten
parameter. - Java's data hiding principle requires a swap function to modify an array's elements. The
swap
method in Java takes the array and the indices of the elements as parameters. - Java's index-based
for
loop involves fewer comparisons due to the built-in support for array lengths and hence less error-prone than manual indexing in C++.
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
Here is the equivalent Java function for the provided C++ bubble sort implementation:
public class BubbleSort {
public static void bubbleSort(int[] arr) {
int n = arr.length;
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
swap(arr, j, j + 1);
}
}
}
}
private static void swap(int[] arr, int i, int j) {
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
public static void main(String[] args) {
int[] array = {64, 34, 25, 12, 22, 11, 90};
bubbleSort(array);
System.out.println("Sorted array: ");
for (int num : array) {
System.out.print(num + " ");
}
}
}
Explanation:
- Method Signature: The method
bubbleSort
takes an array of integers (int[] arr
) and sorts it in place. - Bubble Sort Logic: The logic remains the same as the C++ version, using nested loops to compare adjacent elements.
- Swap Method: The
swap
method is created to handle the swapping of elements in the array, as Java does not support theswap
function directly like C++. - Main Method: A
main
method is included to demonstrate how to call thebubbleSort
method and print the sorted array.
You can run this code in a Java environment to see the bubble sort algorithm in action.
Several options exist for converting the given C++ bubbleSort
function to Java. Here are two versions, one using arrays and another using ArrayList
for more flexibility:
Version 1: Using Arrays (most direct translation)
This version directly mirrors the C++ code's use of arrays. Note that Java arrays have a fixed size, unlike C++'s more dynamic arrays.
class BubbleSort {
public static void bubbleSort(int[] arr) {
int n = arr.length;
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
// Swap arr[j] and arr[j+1]
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
public static void main(String[] args) {
int[] arr = {64, 34, 25, 12, 22, 11, 90};
System.out.println("Unsorted array:");
printArray(arr);
bubbleSort(arr);
System.out.println("\nSorted array:");
printArray(arr);
}
static void printArray(int[] arr) {
for (int j : arr)
System.out.print(j + " ");
}
}
Version 2: Using ArrayList (more flexible)
This version uses an ArrayList
, offering dynamic resizing capabilities. This is generally preferred in Java over fixed-size arrays unless memory efficiency is paramount and the size is known beforehand.
import java.util.ArrayList;
import java.util.List;
class BubbleSortArrayList {
public static void bubbleSort(List<Integer> arr) {
int n = arr.size();
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (arr.get(j) > arr.get(j + 1)) {
// Swap arr.get(j) and arr.get(j+1)
int temp = arr.get(j);
arr.set(j, arr.get(j + 1));
arr.set(j + 1, temp);
}
}
}
}
public static void main(String[] args) {
List<Integer> arr = new ArrayList<>(List.of(64, 34, 25, 12, 22, 11, 90));
System.out.println("Unsorted array:");
System.out.println(arr);
bubbleSort(arr);
System.out.println("\nSorted array:");
System.out.println(arr);
}
}
Both versions achieve the same sorting result. Choose the version that best suits your needs and coding style. The ArrayList
version is generally recommended for its flexibility in Java. Remember to handle potential IndexOutOfBoundsException
if you're not sure the input list/array is valid. The provided examples assume valid input.
Here's the equivalent Java implementation of the bubble sort function:
public static void bubbleSort(int[] arr, int n) {
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
// Swap elements
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
Key differences from the C++ version:
void
remains the sameint arr[]
becomesint[] arr
- Explicit
swap()
is replaced with manual swapping using a temporary variable - The overall logic and structure remain identical to the original C++ implementation
Here's the equivalent Java function for the given C++ code:
public class Main {
public static void bubbleSort(int[] arr) {
int n = arr.length;
// Traverse through all array elements
for (int i = 0; i < n - 1; i++) {
// Last i elements are already in place
for (int j = 0; j < n - i - 1; j++) {
// traverse the array from 0 to n-i-1.
// Swap if the element found is greater than the next element
if (arr[j] > arr[j + 1]) {
int temp = arr[j]; // Swap
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
public static void printArray(int[] arr) {
for (int i = 0; i < arr.length; i++) {
System.out.print(arr[i] + " ");
}
System.out.println();
}
public static void main(String[] args) {
int[] arr = {64, 34, 25, 12, 22, 11, 90};
System.out.println("Original array:");
printArray(arr);
bubbleSort(arr);
System.out.println("Sorted array:");
printArray(arr);
}
}
The provided Java code performs bubble sort on the array [64, 34, 25, 12, 22, 11, 90]
. The bubble sort algorithm repeatedly steps through the list, compares adjacent elements and swaps them if they are in the wrong order. The pass through the list is repeated until the list is sorted.
Please note that bubble sort is not suitable for large datasets due to its high time complexity, which is O(n^2) in the worst case. For larger datasets, you may want to use more efficient sorting algorithms like quicksort or mergesort.
Also, note that in the swap part, we can use a temporary variable to swap two elements. It's been used in the given Java code.
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
All models successfully converted the C++ bubble sort to Java, but with varying levels of sophistication. Gemini 1.5 Pro provided the most comprehensive solution with robust error handling, thorough testing cases, and detailed documentation, though it took the longest at 15.6s. ChatGPT 4o and Llama 3.1 70B both included helpful example implementations and good documentation, with Llama being notably faster (2.3s vs 5.6s). Claude delivered the most concise solution in 4s, focusing on core functionality and key differences between C++ and Java. Llama stood out for its detailed explanation of language differences.
Budget Tier
All models successfully converted the C++ bubble sort to Java, but with varying levels of detail and execution times. Claude 3.5 Haiku provided the most concise solution (3.4s) with essential code and key differences highlighted. ChatGPT 4o Mini (5.9s) and Llama 3.1 8B (0.9s) included additional helper methods and main function implementations. Gemini 1.5 Flash took longest (11s) but offered the most comprehensive response with two implementations - array and ArrayList versions. Claude was most efficient for basic conversion, while Gemini provided the most thorough solution despite slower performance.
JavaScript to Python Palindrome Check
Convert a JavaScript palindrome checker to Python syntax. Useful for understanding string manipulation differences between the languages.
Prompt:
Translate this JavaScript code to Python: function isPalindrome(str) { return str === str.split('').reverse().join(''); }
Performance Results
Here's the equivalent Python function to check if a string is a palindrome:
def is_palindrome(s):
return s == s[::-1]
This function takes advantage of Python's slicing to reverse the string and compares it to the original string. If they are the same, the string is a palindrome.
def is_palindrome(str):
return str == str[::-1]
Key differences explained:
- Python uses
def
instead offunction
- Python naming convention uses snake_case instead of camelCase
- Python's string slicing
[::-1]
provides an elegant way to reverse strings - No need for split() and join() in Python to reverse strings
- Double equals
==
used instead of triple equals===
for equality comparison
def is_palindrome(string):
return string == string[::-1]
Explanation of the JavaScript code and its Python equivalent:
JavaScript:
str.split('')
: Splits the string into an array of individual characters.reverse()
: Reverses the order of elements within the array.join('')
: Joins the elements of the reversed array back into a single string.str === ...
: Compares the original string with the reversed string for strict equality.
Python:
string[::-1]
: This is Python's slicing feature.[::-1]
creates a reversed copy of the string efficiently. It's equivalent to the JavaScriptsplit('').reverse().join('')
sequence, but more concise and Pythonic.
Why the Python version is preferred:
The Python version using slicing ([::-1]
) is generally considered more idiomatic and efficient. It avoids creating intermediate arrays like the JavaScript version, making it more performant, especially for longer strings. It's also significantly more concise and readable.
Here is the translation of your JavaScript code to Python:
def is_palindrome(s):
return s == s[::-1]
In this Python code:
- We define a function called
is_palindrome
with one parameters
. - We use Python's slice notation
s[::-1]
to reverse the string, which is more efficient and readable than usingsplit()
,reverse()
, andjoin()
like in the JavaScript code. - The rest of the logic remains the same as the JavaScript code.
Here is an example of how you could use this function in a Python script:
def is_palindrome(s):
return s == s[::-1]
# Test the function
print(is_palindrome("radar")) # Expected output: True
print(is_palindrome("hello")) # Expected output: False
Remember that this function is case-sensitive and considers spaces and punctuation as characters. If you want a function that is case-insensitive and ignores non-alphanumeric characters, you can use a modified version of the function like this:
def is_palindrome(s):
s = ''.join(e for e in s if e.isalnum()).lower()
return s == s[::-1]
# Test the function
print(is_palindrome("A man, a plan, a canal, Panama")) # Expected output: True
print(is_palindrome("Not a palindrome")) # Expected output: False
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
You can translate the provided JavaScript function to Python as follows:
def is_palindrome(s):
return s == s[::-1]
In this Python version:
s[::-1]
is used to reverse the strings
.- The function checks if the original string is the same as its reversed version and returns
True
orFalse
.
The most direct translation of the JavaScript isPalindrome
function to Python is:
def is_palindrome(text):
"""Checks if a given string is a palindrome (reads the same forwards and backward).
Args:
text: The string to check.
Returns:
True if the string is a palindrome, False otherwise.
"""
processed_text = ''.join(filter(str.isalnum, text)).lower() #Handles spaces and capitalization
return processed_text == processed_text[::-1]
Explanation of Differences and Improvements:
text
instead ofstr
: In Python,str
is a built-in type, so it's better practice to use a different variable name liketext
.[::-1]
for reversing: Python's slicing feature provides a concise way to reverse a string:string[::-1]
.- Case-Insensitive and Non-alphanumeric Handling: The Javascript version is strictly case-sensitive. The Python version above includes
''.join(filter(str.isalnum, text)).lower()
to make it case-insensitive and remove non-alphanumeric characters (spaces, punctuation, etc.) for a more robust palindrome check. This is a common improvement for real-world palindrome checks. If you want a strict comparison like the JS version, you can remove that line.
This improved Python version handles more realistic input scenarios. For example, is_palindrome("A man, a plan, a canal: Panama")
will return True
in Python, while the direct translation of the Javascript code would return False
.
Here's the equivalent Python translation:
def is_palindrome(s):
return s == s[::-1]
In this Python version:
- We use the same function name (converted to snake_case)
- Python's string slicing with
[::-1]
is a concise way to reverse a string - The function returns
True
if the string is equal to its reverse,False
otherwise
A few alternative implementations could include:
# Using reversed() function
def is_palindrome(s):
return s == ''.join(reversed(s))
# More explicit reversal
def is_palindrome(s):
return s == s[::-1]
The first version is most direct and Pythonic, using the slice notation [::-1]
to reverse the string efficiently.
Here's the equivalent Python code:
def is_palindrome(s):
"""
Checks if the input string is a palindrome.
Args:
s (str): The input string.
Returns:
bool: True if the string is a palindrome, False otherwise.
"""
return s == s[::-1]
Here's how it works:
s[::-1]
uses Python's slice notation to create a reversed copy of the input strings
.
You can use it like this:
print(is_palindrome("radar")) # Output: True
print(is_palindrome("hello")) # Output: False
This code is generally more readable and efficient than the original JavaScript version.
Alternatively, you can use Python's built-in reversed
function in combination with the join
method or a generator expression:
def is_palindrome(s):
return s == ''.join(reversed(s))
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
All models successfully converted the JavaScript code to Python using the more elegant string slicing approach [::-1]
. Claude and Gemini provided detailed explanations of the differences between languages. Llama went beyond by including example usage and an enhanced case-insensitive version. Gemini was slowest at 5.5s, while Llama was fastest at 1.5s. ChatGPT gave the most concise response but lacked explanatory context. Claude struck a good balance between brevity and explanation, highlighting key syntax differences between JavaScript and Python.
Budget Tier
The models all correctly translated the JavaScript palindrome function to Python, using the more efficient [::-1] slice notation instead of split/join. ChatGPT provided the most concise solution in 1.6s. Gemini gave the most comprehensive response in 11.2s, adding case-insensitive handling and docstrings, though this deviated from a direct translation. Claude offered multiple valid implementations in 3.6s. Llama delivered a balanced solution with docstrings and examples in just 0.5s, showing impressive speed. For this straightforward translation task, ChatGPT's minimal but accurate response was most appropriate, while Llama showed the best speed-to-detail ratio.
SQL to MongoDB Query Translation
Transform a SQL query into MongoDB's query syntax. Essential for developers migrating from relational to NoSQL databases.
Prompt:
Convert this SQL query to MongoDB query: SELECT * FROM users WHERE age > 25 AND city = 'New York' ORDER BY name ASC
Performance Results
To convert the given SQL query into a MongoDB query, you can use the following syntax:
db.users.find(
{
age: { $gt: 25 },
city: 'New York'
}
).sort({ name: 1 });
Here's a breakdown of the MongoDB query:
db.users.find({ ... })
: This is equivalent toSELECT * FROM users
. Thefind
function is used to query documents in theusers
collection.{ age: { $gt: 25 }, city: 'New York' }
: This specifies the conditions for the query, analogous to theWHERE
clause in SQL.$gt
is a MongoDB operator that stands for "greater than.".sort({ name: 1 })
: This sorts the results in ascending order by thename
field, equivalent toORDER BY name ASC
in SQL. In MongoDB,1
is used for ascending order, while-1
is used for descending order.
db.users.find({
$and: [
{ age: { $gt: 25 } },
{ city: "New York" }
]
}).sort({ name: 1 })
db.users.find(
{
age: { $gt: 25 },
city: "New York"
}
).sort({ name: 1 })
Explanation:
db.users.find()
: This initiates a query on theusers
collection.{ age: { $gt: 25 }, city: "New York" }
: This is the filter document, equivalent to theWHERE
clause in SQL.age: { $gt: 25 }
uses the$gt
operator (greater than) to filter for users older than 25.city: "New York"
filters for users in New York City. Note that string comparison is case-sensitive.
.sort({ name: 1 })
: This sorts the results in ascending order based on thename
field.1
represents ascending order;-1
would be for descending.
Example in MongoDB shell:
If your users
collection looks something like this:
[
{ "name": "Alice", "age": 30, "city": "New York" },
{ "name": "Bob", "age": 22, "city": "Los Angeles" },
{ "name": "Charlie", "age": 28, "city": "New York" },
{ "name": "David", "age": 35, "city": "New York" }
]
The query would return:
[
{ "name": "Alice", "age": 30, "city": "New York" },
{ "name": "Charlie", "age": 28, "city": "New York" },
{ "name": "David", "age": 35, "city": "New York" }
]
Here's how you can convert the SQL query to a MongoDB query:
db.users.find({
age: { $gt: 25 },
city: 'New York'
}).sort({
name: 1
})
Explanation:
db.users.find()
: This is equivalent toSELECT * FROM users
. It retrieves all documents from theusers
collection.{ age: { $gt: 25 } }
: This is equivalent toWHERE age > 25
. It filters the results to include only documents where theage
field is greater than 25. The$gt
operator is used to specify "greater than".{ city: 'New York' }
: This is equivalent toWHERE city = 'New York'
. It filters the results to include only documents where thecity
field is equal to'New York'
..sort({ name: 1 })
: This is equivalent toORDER BY name ASC
. It sorts the results in ascending order based on thename
field.
Note that in MongoDB, sorting is done by piping the result of the .find()
method to the .sort()
method. This is because MongoDB queries can return large datasets, and sorting these datasets can be computationally intensive. By piping the result to .sort()
, MongoDB can optimize the query to handle large datasets.
Also, note that MongoDB queries are typically written in a more concise format, with the filtering and sorting done in a single expression. The query above could also be written as:
db.users.find({
age: { $gt: 25 },
city: 'New York'
}, {
sort: { name: 1 }
})
However, the original format is often preferred because it is more readable and easier to understand, particularly for complex queries.
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
To convert the SQL query you provided into a MongoDB query, you can use the following syntax:
db.users.find({
age: { $gt: 25 },
city: 'New York'
}).sort({ name: 1 })
Explanation:
db.users.find(...)
: This is the method used to query theusers
collection in MongoDB.{ age: { $gt: 25 }, city: 'New York' }
: This is the filter criteria where$gt: 25
means "greater than 25" for theage
field andcity: 'New York'
matches documents where thecity
field equals 'New York'..sort({ name: 1 })
: This sorts the results in ascending order based on thename
field. (In MongoDB,1
represents ascending order, while-1
would represent descending order.)
This MongoDB query achieves the same result as your SQL query.
The equivalent MongoDB query using the aggregation framework would be:
db.users.aggregate([
{
$match: {
age: { $gt: 25 },
city: "New York"
}
},
{
$sort: {
name: 1
}
}
])
This does the following:
$match
: This stage filters the documents based on the specified criteria (age > 25 and city = 'New York').$gt
means "greater than".$sort
: This stage sorts the resulting documents in ascending order (1
) based on thename
field. Descending order would be-1
.
If you're using the find()
method directly (which is simpler for this specific case, but less flexible for complex queries), you could write it like this:
db.users.find({ age: { $gt: 25 }, city: "New York" }).sort({ name: 1 })
This achieves the same result more concisely. The find()
method directly supports filtering and sorting. Choose this method if your query doesn't require complex aggregation pipeline stages.
Here's the equivalent MongoDB query:
db.users.find({
age: { $gt: 25 },
city: 'New York'
}).sort({ name: 1 })
Breaking down the query:
db.users
refers to the collection.find()
is used to retrieve documents{ age: { $gt: 25 } }
represents the condition for age greater than 25{ city: 'New York' }
matches users in New York.sort({ name: 1 })
sorts the results by name in ascending order (1 for ascending, -1 for descending)
Here's the equivalent MongoDB query:
db.users.find({
age: {$gt: 25},
city: 'New York'
})
.sort({
name: 1
})
Explanation of the conversion:
- SELECT * FROM users in SQL is replaced by
db.users.find()
in MongoDB, wheredb.users
is the collection. - WHERE age > 25 corresponds to the MongoDB filter
age: {$gt: 25}
, where$gt
is the greater-than operator. - AND city = 'New York' is added to the filter with a comma as a separator.
- ORDER BY name ASC in SQL translates to
.sort({name: 1})
in MongoDB, where1
is the ascending order.
Note: In MongoDB, you don't need to explicitly write the column names in the query, as the query will be applied on the entire document.
Also, the MongoDB query will return all fields (i.e., "SELECT *") from the documents where the query is satisfied, similar to the SQL query.
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
All models successfully converted the SQL query to MongoDB syntax, but with varying levels of detail and efficiency. Claude provided the most concise solution, executing in just 1272ms, though included an unnecessary $and operator. ChatGPT and Gemini offered detailed explanations with their solutions, but took significantly longer (7707ms and 9396ms respectively). Llama provided a comprehensive response with alternative syntax, executing in 1789ms. All solutions were technically correct, with the main differences being in execution time and verbosity of explanations.
Budget Tier
All models successfully converted the SQL query to MongoDB, but with varying levels of detail and efficiency. Claude and Llama provided clear, accurate translations with good execution times (2661ms and 568ms respectively). Gemini offered both aggregate and find solutions but took longest at 12780ms. ChatGPT's response was thorough but slower at 4676ms. Llama stands out for combining speed with comprehensive explanation, while Claude wins on clarity and conciseness. Gemini's extra aggregate method could be useful for complex queries but may be overkill here. All responses correctly used find(), $gt operator, and sort syntax.
Ruby to Python Fibonacci Function
Convert a Ruby recursive Fibonacci implementation to Python. Helpful for understanding recursive function syntax differences.
Prompt:
Transform this Ruby code to Python: def fibonacci(n); n <= 1 ? n : fibonacci(n-1) + fibonacci(n-2); end
Performance Results
To transform the given Ruby fibonacci
function to Python, you can define a similar recursive function in Python. Here's how you can do it:
def fibonacci(n):
if n <= 1:
return n
else:
return fibonacci(n - 1) + fibonacci(n - 2)
This Python version mimics the logic of the Ruby code, using an if
statement to make a decision rather than using the ternary operator (? :
) from Ruby.
def fibonacci(n):
return n if n <= 1 else fibonacci(n-1) + fibonacci(n-2)
def fibonacci(n):
"""
Calculates the nth Fibonacci number.
"""
if n <= 1:
return n
else:
return fibonacci(n-1) + fibonacci(n-2)
# Example usage (optional):
print(fibonacci(0)) # Output: 0
print(fibonacci(1)) # Output: 1
print(fibonacci(2)) # Output: 1
print(fibonacci(3)) # Output: 2
print(fibonacci(10)) # Output: 55
Explanation of Changes:
Conditional (Ternary Operator to
if/else
): Ruby's ternary operatorcondition ? value_if_true : value_if_false
is replaced with a standard Pythonif/else
statement for clarity.Function Definition: Ruby's
def fibonacci(n); ... end
becomes Python'sdef fibonacci(n): ...
. Indentation is crucial in Python to define the function body.Return Statement (Implicit to Explicit): Ruby implicitly returns the last evaluated expression. In Python, you must use an explicit
return
statement.
Improving Efficiency (Optional):
The provided recursive solution is clear but can be computationally expensive for larger values of n
due to repeated calculations. Here are two ways to improve it:
a) Memoization (using a dictionary):
memo = {} # Store already calculated Fibonacci numbers
def fibonacci_memo(n):
if n in memo:
return memo[n]
if n <= 1:
result = n
else:
result = fibonacci_memo(n-1) + fibonacci_memo(n-2)
memo[n] = result
return result
b) Iterative Approach:
def fibonacci_iterative(n):
a, b = 0, 1
for _ in range(n):
a, b = b, a + b
return a
Both the memoization and iterative methods are significantly more efficient than the original recursive version for larger values of n
. The iterative approach is generally the most performant.
Python Equivalent of the Ruby Fibonacci Function
def fibonacci(n: int) -> int:
"""
Calculate the nth Fibonacci number.
Args:
n (int): The position of the Fibonacci number to calculate.
Returns:
int: The nth Fibonacci number.
"""
if n <= 1:
return n
else:
return fibonacci(n-1) + fibonacci(n-2)
Note: This function has a high time complexity due to its recursive nature and repeated calculations. For large values of n, it may not be practical to use this function.
Optimized Version (Memoization):
def fibonacci(n: int, memo: dict = {}) -> int:
"""
Calculate the nth Fibonacci number with memoization.
Args:
n (int): The position of the Fibonacci number to calculate.
memo (dict): A dictionary to store previously calculated Fibonacci numbers.
Returns:
int: The nth Fibonacci number.
"""
if n <= 1:
return n
elif n in memo:
return memo[n]
else:
result = fibonacci(n-1, memo) + fibonacci(n-2, memo)
memo[n] = result
return result
This optimized version stores previously calculated Fibonacci numbers in a dictionary to avoid repeated calculations, significantly improving performance.
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
You can transform the given Ruby function for calculating Fibonacci numbers into Python as follows:
def fibonacci(n):
return n if n <= 1 else fibonacci(n - 1) + fibonacci(n - 2)
In this Python code:
- We define a function
fibonacci
that takes one argumentn
. - It uses a conditional expression (similar to the ternary operator in Ruby) to return
n
if it is less than or equal to 1. Otherwise, it computes the sum of the two previous Fibonacci numbers by recursively calling thefibonacci
function.
The Ruby code uses a ternary operator. Here are a couple of ways to translate it to Python:
Option 1: Using a ternary operator (Python's way):
def fibonacci(n):
return n if n <= 1 else fibonacci(n-1) + fibonacci(n-2)
This is the most direct translation, mirroring the Ruby code's structure.
Option 2: Using if/else
for better readability (Recommended):
def fibonacci(n):
if n <= 1:
return n
else:
return fibonacci(n-1) + fibonacci(n-2)
This version is arguably more readable, especially for those less familiar with Python's ternary operator. It's generally preferred for its clarity.
Important Note: Both of these solutions are recursive. While elegant, recursive Fibonacci calculations are highly inefficient for larger values of n
. For better performance with larger numbers, consider an iterative approach:
def fibonacci_iterative(n):
a, b = 0, 1
for _ in range(n):
a, b = b, a + b
return a
This iterative version avoids the repeated calculations inherent in the recursive approach and will be significantly faster for larger n
. Choose the iterative version unless you specifically need a recursive solution for educational purposes or very small values of n
.
Here's the equivalent Python code for the Ruby Fibonacci function:
def fibonacci(n):
return n if n <= 1 else fibonacci(n-1) + fibonacci(n-2)
The Python version closely mirrors the Ruby original:
- Uses the same recursive approach
- Employs a ternary-like conditional expression
- Returns
n
for base cases (0 and 1) - Recursively calculates Fibonacci sequence for larger
n
Note that while this implementation is elegant, it's computationally inefficient for large n
due to redundant recursive calls. For performance-critical scenarios, consider using dynamic programming or memoization techniques.
Here's the equivalent Python code for the given Ruby code:
def fibonacci(n):
"""
Returns the nth Fibonacci number.
Args:
n (int): The position of the Fibonacci number to return.
Returns:
int: The nth Fibonacci number.
"""
return n if n <= 1 else fibonacci(n-1) + fibonacci(n-2)
However, please note that this is not an efficient way to calculate Fibonacci numbers for large n. This method has an exponential time complexity due to the repeated calculations of the same subproblems.
Here's a more efficient solution using dynamic programming with memoization:
def fibonacci(n, memo = {}):
"""
Returns the nth Fibonacci number.
Args:
n (int): The position of the Fibonacci number to return.
memo (dict): A dictionary to store the memoized results.
Returns:
int: The nth Fibonacci number.
"""
return memo.setdefault(n, n if n <= 1 else fibonacci(n-1, memo) + fibonacci(n-2, memo))
And here's an even more efficient solution using matrix exponentiation:
def multiply_matrices(a, b):
"""
Multiplies two 2x2 matrices a and b.
Args:
a (list): The first matrix.
b (list): The second matrix.
Returns:
list: The product of the matrices a and b.
"""
result = [[0, 0], [0, 0]]
for i in range(2):
for j in range(2):
for k in range(2):
result[i][j] += a[i][k] * b[k][j]
return result
def matrix_power(matrix, n):
"""
Calculates the nth power of a 2x2 matrix.
Args:
matrix (list): The initial matrix.
n (int): The power to which the matrix should be raised.
Returns:
list: The matrix raised to the power n.
"""
if n <= 1:
return matrix
if n % 2 == 0:
half_pow = matrix_power(matrix, n // 2)
return multiply_matrices(half_pow, half_pow)
else:
half_pow = matrix_power(matrix, n // 2)
return multiply_matrices(multiply_matrices(half_pow, half_pow), matrix)
def fibonacci(n):
"""
Returns the nth Fibonacci number.
Args:
n (int): The position of the Fibonacci number to return.
Returns:
int: The nth Fibonacci number.
"""
if n <= 1:
return n
fib_matrix = [[1, 1], [1, 0]]
result_matrix = matrix_power(fib_matrix, n - 1)
return result_matrix[0][0]
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
ChatGPT and Claude provided concise translations, with Claude offering the most elegant solution using Python's ternary operator. Gemini and Llama gave more comprehensive responses with additional optimizations and documentation. Gemini was slowest at 10.9s but included example usage and multiple optimization approaches. Llama added type hints and detailed docstrings. For simple code translation, Claude was fastest and most efficient at 1.1s. All models successfully converted the code, but differed in verbosity and additional features provided.
Budget Tier
All models successfully converted the Ruby code to Python, with varying levels of detail. Gemini 1.5 Flash provided the most comprehensive response, offering multiple implementation options and performance considerations. Claude and ChatGPT gave concise, accurate translations with helpful explanations. Llama 3.1 8B provided the most technical response, including advanced optimization techniques like matrix exponentiation. Response times ranged from 1.1s (Llama) to 3.1s (Gemini). While all models handled the basic translation well, Gemini and Llama stood out for their additional insights into performance optimization.
Java to C# Binary Search
Convert a Java binary search implementation to C#. Useful for developers working across Java and .NET ecosystems.
Prompt:
Translate this Java code to C#: public static int binarySearch(int[] arr, int target) { int left = 0; int right = arr.length - 1; while(left <= right) { int mid = left + (right - left)/2; if(arr[mid] == target) return mid; if(arr[mid] < target) left = mid + 1; else right = mid - 1; } return -1; }
Performance Results
Here's the Java code translated to C#:
public static int BinarySearch(int[] arr, int target)
{
int left = 0;
int right = arr.Length - 1;
while (left <= right)
{
int mid = left + (right - left) / 2;
if (arr[mid] == target)
return mid;
if (arr[mid] < target)
left = mid + 1;
else
right = mid - 1;
}
return -1;
}
Key Differences:
- Method names in C# are usually PascalCase by convention, hence
BinarySearch
instead ofbinarySearch
. - The array's length is accessed using
arr.Length
in C# instead ofarr.length
in Java. - The rest of the logic remains the same as both languages share similar syntax for basic control structures and arithmetic operations.
public static int BinarySearch(int[] arr, int target)
{
int left = 0;
int right = arr.Length - 1;
while (left <= right)
{
int mid = left + (right - left) / 2;
if (arr[mid] == target)
return mid;
if (arr[mid] < target)
left = mid + 1;
else
right = mid - 1;
}
return -1;
}
Key differences from Java:
- Method name is capitalized (PascalCase convention in C#)
- Array length is a property (Length) rather than a field (length)
- Curly brace placement follows C# conventions
public static int BinarySearch(int[] arr, int target)
{
int left = 0;
int right = arr.Length - 1;
while (left <= right)
{
int mid = left + (right - left) / 2;
if (arr[mid] == target)
return mid;
if (arr[mid] < target)
left = mid + 1;
else
right = mid - 1;
}
return -1;
}
Here's a breakdown of the changes:
arr.length
becomesarr.Length
: In C#, the length of an array is accessed through theLength
property, not thelength
field.- Method naming convention: Java typically uses
camelCase
for method names (e.g.,binarySearch
). C# conventions favorPascalCase
(e.g.,BinarySearch
). I've updated the method name to follow C# best practices. While the code would work withbinarySearch
, consistency is important in C#.
The core logic of the binary search algorithm remains identical. The C# code performs the same steps as the Java code:
- Initialize
left
andright
pointers: Pointing to the start and end of the array, respectively. - Iterate while
left
is less than or equal toright
: This loop continues as long as there's a potential search space. - Calculate the middle index
mid
: Usingleft + (right - left) / 2
to avoid potential integer overflow ifleft + right
exceeds the maximum integer value. - Check if
arr[mid]
equals thetarget
: If so, the target is found, and its indexmid
is returned. - Adjust
left
orright
based on the comparison:
- If
arr[mid]
is less than thetarget
, the target must be in the right half, soleft
is updated tomid + 1
. - Otherwise, the target must be in the left half, so
right
is updated tomid - 1
.
- Return -1 if the target is not found: After the loop completes without finding the target.
This C# version is functionally equivalent to the provided Java code. It effectively implements a binary search algorithm on a sorted integer array.
Here's the equivalent C# code for the provided Java binary search method:
public static int BinarySearch(int[] arr, int target)
{
int left = 0;
int right = arr.Length - 1;
while (left <= right)
{
int mid = left + (right - left) / 2;
if (arr[mid] == target)
return mid;
else if (arr[mid] < target)
left = mid + 1;
else
right = mid - 1;
}
return -1;
}
Note the few changes between the Java and C# versions:
public static int BinarySearch
uses PascalCase, following C# naming conventions, whereaspublic static int binarySearch
uses camelCase in Java.arr.Length
is used instead ofarr.length
, becauseLength
in C# is a property, not a field likelength
in Java.
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
Here is the equivalent C# code for the provided Java binary search function:
public static int BinarySearch(int[] arr, int target)
{
int left = 0;
int right = arr.Length - 1;
while (left <= right)
{
int mid = left + (right - left) / 2;
if (arr[mid] == target)
return mid;
if (arr[mid] < target)
left = mid + 1;
else
right = mid - 1;
}
return -1;
}
Key Differences Explained:
- Method Naming: In C#, it's common practice to use PascalCase for method names, so
binarySearch
becomesBinarySearch
. - Array Length: In C#,
arr.Length
is used instead ofarr.length
in Java. - General Syntax: C# uses braces
{}
in the same way as Java, but some conventions (like method and variable naming) differ between the two languages.
You would use this function in a similar way as you would in Java, passing an integer array and an integer target to search for the index of the target in the array.
The Java code performs a binary search on a sorted integer array. Here's the equivalent C# code:
public static int BinarySearch(int[] arr, int target)
{
int left = 0;
int right = arr.Length - 1;
while (left <= right)
{
int mid = left + (right - left) / 2; // Avoid potential overflow
if (arr[mid] == target)
{
return mid;
}
else if (arr[mid] < target)
{
left = mid + 1;
}
else
{
right = mid - 1;
}
}
return -1; // Target not found
}
The core logic remains identical. The only significant change is the naming convention (Java's binarySearch
becomes C#'s BinarySearch
), and using arr.Length
instead of arr.length
. The else if
structure improves readability compared to the chained if
/else
in the original Java code. The calculation of mid
remains the same to prevent potential integer overflow issues.
Here's the equivalent C# translation:
public static int BinarySearch(int[] arr, int target)
{
int left = 0;
int right = arr.Length - 1;
while (left <= right)
{
int mid = left + (right - left) / 2;
if (arr[mid] == target)
return mid;
if (arr[mid] < target)
left = mid + 1;
else
right = mid - 1;
}
return -1;
}
The key differences from the Java version are:
- Method name is in PascalCase (C# convention)
length
is replaced withLength
- The overall logic remains exactly the same
This is a standard binary search implementation that:
- Returns the index of the target if found
- Returns -1 if the target is not in the array
- Works on a sorted array
- Has a time complexity of O(log n)
Here is the equivalent C# code for the given Java Binary Search algorithm:
public static int BinarySearch(int[] arr, int target)
{
int left = 0;
int right = arr.Length - 1;
while (left <= right)
{
int mid = left + (right - left) / 2;
if (arr[mid] == target) return mid;
if (arr[mid] < target) left = mid + 1;
else right = mid - 1;
}
return -1;
}
Please note that the only change was from arr.length
to arr.Length
because in C# the property is named Length
and is capitalized.
You can also use LINQ (Language Integrated Query) to implement a binary search, but it would not be more efficient and would likely result in less readable code:
using System.Linq;
public static int BinarySearch(int[] arr, int target)
{
var index = arr.Select((x, i) => new { Value = x, Index = i })
.Where(x => x.Value == target)
.Select(x => x.Index)
.FirstOrDefault();
return index != 0 ? index : -1;
}
But the first implementation is the recommended one.
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
All models successfully translated the Java binary search code to C# with proper syntax and conventions. ChatGPT and Claude provided clean, accurate translations with concise explanations of key differences. Gemini's response, while accurate, was unnecessarily verbose with extensive explanations. Llama performed the fastest (1184ms) and gave a clear translation with relevant notes. ChatGPT (3958ms) and Claude (5902ms) had moderate response times, while Gemini was slowest (12232ms). The main differences highlighted across all responses were the PascalCase method naming and Length property in C#.
Budget Tier
All models successfully translated the Java binary search code to C#, with main differences being capitalization of 'Length' and method name. Gemini and Llama provided additional context - Gemini suggested using else-if for readability, while Llama included a LINQ alternative. Performance-wise, Llama was fastest at 663ms, followed by Gemini at 2217ms. Claude and ChatGPT were slower at ~3600ms but provided clean, well-formatted code. All accurately highlighted the key language convention differences between Java and C#.
Python List Comprehension to JavaScript
Transform Python's list comprehension into JavaScript array operations. Helpful for understanding array manipulation differences.
Prompt:
Convert this Python list comprehension to JavaScript: squares = [x**2 for x in range(10)]
Performance Results
You can convert the Python list comprehension into JavaScript by using the map
function along with the Array.from()
function to create an array representing the range. Here's how you can do it:
let squares = Array.from({ length: 10 }, (_, x) => x ** 2);
Here's a breakdown of the code:
Array.from({ length: 10 }, (_, x) => x ** 2)
creates an array with 10 elements.- The
length: 10
specifies the number of elements in the array, mimickingrange(10)
in Python. (_, x)
represents the current value and its index. We only use the indexx
here.x ** 2
calculates the square of each index, similar to the Python expressionx**2
.
This JavaScript code effectively mirrors the functionality of the Python list comprehension [x**2 for x in range(10)]
.
const squares = Array.from({length: 10}, (_, x) => x**2);
// Or alternatively:
const squares = [...Array(10)].map((_, x) => x**2);
Both approaches will create an array containing [0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
The first approach uses Array.from()
with a length of 10 and a mapping function.
The second approach uses the spread operator with Array(10)
to create an array of 10 empty slots, then maps over it.
const squares = [];
for (let x = 0; x < 10; x++) {
squares.push(x ** 2);
}
// Or, using map:
const squares = Array.from({ length: 10 }, (_, x) => x ** 2);
// Or, using keys() and map (less efficient):
const squares = [...Array(10).keys()].map(x => x ** 2);
Explanation of each method:
for
loop: This is the most straightforward translation. It directly mirrors the Python loop's logic by iterating through numbers 0-9 and pushing the squared value onto thesquares
array.Array.from()
andmap()
: This is a more functional approach.Array.from({ length: 10 })
creates an array of length 10. The second argument toArray.from()
is a mapping function, similar to Python's list comprehension.(_, x) => x ** 2
takes the indexx
(we use_
for the unused element value) and returns its square. This mapping function is applied to each index of the array.keys()
andmap()
:Array(10).keys()
creates an iterator over the indices of an array of length 10. The spread syntax[...]
converts the iterator into an actual array. Thenmap()
applies the squaring function to each element. This approach is a bit less direct than theArray.from()
method and is generally slightly less efficient.
The Array.from()
method is generally considered the most idiomatic and concise way to achieve list comprehension-like functionality in JavaScript, particularly when dealing with ranges of numbers. The for
loop remains a perfectly valid and sometimes more readable option, especially for more complex logic within the loop.
Here is the equivalent JavaScript code:
const squares = Array.from({length: 10}, (_, i) => i ** 2);
However, the more common way to achieve this in JavaScript is by using the map()
function with Array.from()
or a for loop and a more traditional array push:
// Using Array.from() and map()
const squares = Array.from({length: 10}, (_, i) => i ** 2);
// Using Array.from() and a for loop
const squares = [];
for (let i = 0; i < 10; i++) {
squares.push(i ** 2);
}
// Using map()
const squares = Array(10).fill().map((_, i) => i ** 2);
The first way using (_, i) => i ** 2
will create an array with the specified length, then map each index (i) to its square.
In the above examples, _
is a common convention in JavaScript for a variable you are not going to use (i.e., i
in the first solution or the actual array element in the Array.fill()
and map()
example).
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
You can convert the Python list comprehension to JavaScript using the Array.from()
method or the map()
method on an array of the desired range. Here's how you can do it:
Using Array.from()
const squares = Array.from({ length: 10 }, (v, x) => x ** 2);
Using the map()
method
const squares = [...Array(10)].map((_, x) => x ** 2);
Both of these snippets will create an array of squares of numbers from 0 to 9, similar to the Python list comprehension.
There are several ways to translate the Python list comprehension squares = [x**2 for x in range(10)]
to JavaScript. Here are two common approaches:
1. Using map()
:
This is generally considered the most idiomatic and efficient way to achieve the same result in JavaScript:
const squares = Array.from({length: 10}, (_, i) => i**2);
Array.from({length: 10})
creates an array of length 10 filled withundefined
.(_, i) => i**2
is a callback function passed tomap()
._
is used to ignore the value (undefined) at each index, andi
represents the index (0 to 9). The function squares the index.
2. Using a for
loop:
This is a more direct translation, but less concise than using map()
:
const squares = [];
for (let x = 0; x < 10; x++) {
squares.push(x**2);
}
Both of these JavaScript snippets produce an array squares
containing the squares of numbers from 0 to 9: [0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
. The map()
approach is generally preferred for its readability and conciseness.
Here's the equivalent JavaScript code:
const squares = Array.from({length: 10}, (_, i) => i ** 2);
Alternatively, you could also write it as:
const squares = [...Array(10)].map((_, i) => i ** 2);
Both approaches achieve the same result of creating an array of squares from 0 to 9. The key differences from the Python version are:
- JavaScript doesn't have a direct equivalent to Python's range()
- We use
Array.from()
or spread syntax to create an array - The mapping function uses
i ** 2
for exponentiation - We use
const
for variable declaration
Here's the equivalent JavaScript code using the map()
method to calculate the squares of numbers from 0 to 9:
const squares = Array.from({length: 10}, (_, i) => i ** 2);
console.log(squares); // Output: [0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
In this code, Array.from()
is used to create an array with 10 elements. The function (_, i) => i ** 2
is called for each iteration, which squares the current number (i
) and returns it. The _
variable is a common convention for a variable that is not used in the function.
Alternatively, you can use the spread operator (...
) to create an array of squares:
const squares = [...Array(10)].map((_, i) => i ** 2);
console.log(squares); // Output: [0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
In this code, Array(10)
creates an array with 10 undefined values. The spread operator (...
) is used to flatten this array, and then map()
is applied to calculate the squares of numbers from 0 to 9.
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
Each model provided correct JavaScript translations, but with varying levels of detail and approaches. ChatGPT 4o offered a clear, single solution with detailed explanation. Claude provided two concise solutions without excessive explanation. Gemini 1.5 Pro gave the most comprehensive response with three methods and detailed explanations of each approach. Llama 3.1 70B provided multiple solutions with practical context about JavaScript conventions. Response times varied significantly, with Llama being fastest at 1.2s and Gemini slowest at 9.1s. Overall, Gemini provided the most thorough technical response, while Claude delivered the most efficient answer.
Budget Tier
All models successfully provided working JavaScript solutions, with Array.from() and map() approaches being common across responses. Llama 3.1 8B was notably the fastest (689ms) and included helpful console.log examples. ChatGPT Mini was concise but effective (2567ms). Claude offered clear explanations of key differences from Python (3141ms). Gemini took longest (10174ms) but provided the most comprehensive explanation including a for-loop alternative. All models demonstrated strong understanding of JavaScript array methods and modern syntax.
Go to Rust QuickSort
Convert a Go implementation of QuickSort to Rust. Essential for developers transitioning between these modern systems programming languages.
Prompt:
Translate this Go function to Rust: func quickSort(arr []int) []int { if len(arr) <= 1 { return arr } pivot := arr[len(arr)/2]; left := make([]int, 0); right := make([]int, 0); for _, x := range arr { if x < pivot { left = append(left, x) } else if x > pivot { right = append(right, x) } } return append(append(quickSort(left), pivot), quickSort(right)...)}
Performance Results
To translate the given Go quickSort
function to Rust, you'll need to make some adjustments due to the differences in the language syntax and paradigms. Here's how you can write an equivalent function in Rust:
fn quick_sort(mut arr: Vec<i32>) -> Vec<i32> {
if arr.len() <= 1 {
return arr;
}
let pivot_index = arr.len() / 2;
let pivot = arr[pivot_index];
let mut left = Vec::new();
let mut right = Vec::new();
for &x in &arr {
if x < pivot {
left.push(x);
} else if x > pivot {
right.push(x);
}
}
let mut sorted = quick_sort(left);
sorted.push(pivot);
sorted.append(&mut quick_sort(right));
sorted
}
fn main() {
let arr = vec![5, 3, 8, 4, 2];
let sorted_arr = quick_sort(arr);
println!("{:?}", sorted_arr);
}
Key Differences and Notes:
Mutable Vector: In Rust, we use a mutable
Vec<i32>
instead of a Go slice[]int
. The slice in Go is more akin to a slice in Rust, but since we need to build new vectors in the process, aVec
is more appropriate here.Pivot Selection: The pivot element is selected from the middle of the array, similar to the Go implementation.
Loops and Mutability: Rust's for loop iterates over references, so the
&
operator is used to dereferencex
. Vectors in Rust are mutable, so vector operations such aspush
andappend
are used.Appending and Concatenation: In Rust,
Vec::push
adds a single element, andVec::append
is used to append all elements from another vector.Return Value: Just like in Go, the function returns a newly sorted vector.
Main Function: A main function in Rust is used to demonstrate sorting with our custom
quick_sort
function.
fn quick_sort(arr: Vec<i32>) -> Vec<i32> {
if arr.len() <= 1 {
return arr;
}
let pivot = arr[arr.len() / 2];
let mut left: Vec<i32> = Vec::new();
let mut right: Vec<i32> = Vec::new();
let mut middle: Vec<i32> = Vec::new();
for &x in arr.iter() {
if x < pivot {
left.push(x);
} else if x > pivot {
right.push(x);
} else {
middle.push(x);
}
}
let mut result = quick_sort(left);
result.extend(middle);
result.extend(quick_sort(right));
result
}
Key differences from the Go version:
- Uses
Vec<i32>
instead of[]int
for integer arrays - Explicitly handles equal elements in a
middle
vector to avoid infinite recursion - Uses
extend()
instead ofappend()
to combine vectors - Uses
iter()
and explicit reference/dereference operators - Follows Rust naming convention of snake_case instead of camelCase
pub fn quick_sort(arr: &mut [i32]) {
if arr.len() <= 1 {
return;
}
let pivot_index = arr.len() / 2;
let pivot = arr[pivot_index];
let mut left = Vec::new();
let mut right = Vec::new();
for &x in arr {
if x < pivot {
left.push(x);
} else if x > pivot {
right.push(x);
}
}
let mut sorted_arr = Vec::new();
let left_slice = &mut arr[..left.len()];
left_slice.copy_from_slice(&left);
quick_sort(left_slice);
sorted_arr.extend_from_slice(left_slice);
sorted_arr.push(pivot);
let right_slice = &mut arr[left.len() + 1..left.len() + 1 + right.len()];
right_slice.copy_from_slice(&right);
quick_sort(right_slice);
sorted_arr.extend_from_slice(right_slice);
arr.copy_from_slice(&sorted_arr);
}
#[cfg(test)]
mod tests {
use super::*;
#[test]
fn test_quick_sort() {
let mut arr = vec![3, 1, 4, 1, 5, 9, 2, 6];
quick_sort(&mut arr);
assert_eq!(arr, vec![1, 1, 2, 3, 4, 5, 6, 9]);
let mut arr = vec![5, 4, 3, 2, 1];
quick_sort(&mut arr);
assert_eq!(arr, vec![1, 2, 3, 4, 5]);
let mut arr = vec![1, 2, 3, 4, 5];
quick_sort(&mut arr);
assert_eq!(arr, vec![1, 2, 3, 4, 5]);
let mut arr = vec![];
quick_sort(&mut arr);
assert_eq!(arr, vec![]);
let mut arr = vec![5];
quick_sort(&mut arr);
assert_eq!(arr, vec![5]);
let mut arr = vec![5,5,5,5];
quick_sort(&mut arr);
assert_eq!(arr, vec![5,5,5,5]);
}
}
Key changes and explanations:
In-place sorting: The Rust version sorts the input slice
arr
in-place instead of creating a new vector and returning it. This is generally more efficient and idiomatic in Rust.Slice manipulation: The code uses Rust's slicing capabilities (
&mut arr[..left.len()]
, etc.) to create mutable views into the original array. This allows us to recursively sort portions of the array without extra allocations. This is a crucial difference from the Go approach, which usesappend
to create new slices. Direct slicing within the original array avoids repeated allocations and copies, improving performance, especially for larger arrays.copy_from_slice
: This method is used to copy the contents of temporary vectors (left
,right
) into the appropriate slices of the original array.Extending the result: The code uses
extend_from_slice
to add elements from slices to thesorted_arr
vector.Test Cases: Included comprehensive test cases to validate the function's correctness against various input scenarios, including empty arrays, single-element arrays, and arrays with duplicate elements.
This Rust version is more efficient than a direct translation of the Go code that used Vec::append
repeatedly because it minimizes allocations and copying. It leverages Rust's powerful ownership and borrowing system to achieve in-place sorting. The included test cases demonstrate the correctness of the implementation.
Here's the equivalent function in Rust:
fn quicksort(mut arr: Vec<i32>) -> Vec<i32> {
if arr.len() <= 1 {
return arr;
}
let pivot_index = arr.len() / 2;
let pivot = arr[pivot_index];
arr.remove(pivot_index);
let mut left: Vec<i32> = arr.into_iter().filter(|x| *x < pivot).collect();
let mut right: Vec<i32> = arr.into_iter().filter(|x| *x >= pivot).collect();
let mut sorted_left = quicksort(left);
let mut sorted_right = quicksort(right);
sorted_left.push(pivot);
sorted_left.extend(sorted_right);
sorted_left
}
// Testing the function
fn main() {
let mut arr = vec![4, 2, 9, 6, 3, 1, 5, 8, 7];
arr = quicksort(arr);
println!("{:?}", arr);
}
However, this implementation does not directly translate the provided Go function because Rust does not allow slicing a vector in the same way Go does. In this implementation, we first remove the pivot from the vector, then filter the vector into two vectors (left
and right
). This modification makes the code safer and more idiomatic in Rust.
Note that Rust uses pattern matching for iteration over vectors instead of iterating over a vector while modifying it. Additionally, Rust's into_iter()
and collect()
are used here to create a new vector from the filtered items.
You could implement it more similarly to the Go code using std::vec::Vec::swap()
and std::vec::Vec::split_off
, but it's still slightly different because of how Rust handles its borrowing mechanism.
Here is how you could do it more similarly:
fn quicksort(mut arr: Vec<i32>) -> Vec<i32> {
if arr.len() <= 1 {
return arr;
}
let pivot = arr[arr.len() / 2];
let mut left = Vec::new();
let mut right = Vec::new();
for x in arr.drain(..) {
if x < pivot {
left.push(x);
} else {
right.push(x);
}
}
let mut sorted_left = quicksort(left);
let mut sorted_right = quicksort(right);
sorted_left.push(pivot);
sorted_left.extend(sorted_right);
sorted_left
}
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
Here's how you can translate the given Go function for quicksort into Rust:
fn quick_sort(arr: Vec<i32>) -> Vec<i32> {
// Base case: if the array length is 1 or less, return it
if arr.len() <= 1 {
return arr;
}
// Choose the pivot: middle element of the array
let pivot = arr[arr.len() / 2];
let mut left = Vec::new();
let mut right = Vec::new();
// Partition the array into left and right vectors
for &x in &arr {
if x < pivot {
left.push(x);
} else if x > pivot {
right.push(x);
}
}
// Concatenate the sorted left, the pivot, and the sorted right vectors
let mut sorted_left = quick_sort(left);
let mut sorted_right = quick_sort(right);
// Append the pivot to the sorted left array
sorted_left.push(pivot);
// Append the sorted right array to the sorted left array
sorted_left.append(&mut sorted_right);
sorted_left
}
fn main() {
let arr = vec![3, 6, 8, 10, 1, 2, 1];
let sorted_arr = quick_sort(arr);
println!("{:?}", sorted_arr);
}
Explanation:
- Function Signature: The function
quick_sort
takes aVec<i32>
as input and returns aVec<i32>
. - Base Case: If the length of
arr
is 1 or less, it returnsarr
. - Pivot Selection: The pivot is selected as the middle element (
arr.len() / 2
). - Partitioning: It iterates over the elements of
arr
and partitions them intoleft
andright
based on their comparison with the pivot. - Recursive Sorting: It recursively sorts the
left
andright
partitions. - Combining Results: It combines the sorted left partition, the pivot, and the sorted right partition, and returns the result.
- Main Function: An example usage is given in the
main
function to demonstrate how to callquick_sort
and print the sorted array.
Make sure to have Rust installed and use a Rust workspace to test this code snippet.
Several Rust translations are possible, each with slightly different performance characteristics. Here are two options:
Option 1: Using Vec
and partition
(More idiomatic and generally faster):
This version leverages Rust's built-in partition
method for a more concise and potentially faster implementation.
fn quick_sort(arr: &mut [i32]) {
if arr.len() <= 1 {
return;
}
let pivot_index = arr.len() / 2;
let pivot = arr[pivot_index];
// Partition the array in-place
arr.swap(pivot_index, arr.len() - 1);
let partition_point = arr.partition_point(|&x| x < pivot);
arr.swap(partition_point, arr.len() - 1);
quick_sort(&mut arr[..partition_point]);
quick_sort(&mut arr[partition_point + 1..]);
}
fn main() {
let mut arr = vec![3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5];
quick_sort(&mut arr);
println!("{:?}", arr); // Output: [1, 1, 2, 3, 3, 4, 5, 5, 5, 6, 9]
}
This version sorts the array in-place, avoiding the creation of new vectors for left
and right
which significantly improves efficiency, especially for larger arrays. The partition_point
method efficiently finds the index where the partition should occur.
Option 2: More closely mirroring the Go code (Less efficient):
This option attempts to replicate the Go code's structure more closely, creating new vectors. It's less efficient than Option 1 but easier to understand if you're familiar with the Go code.
fn quick_sort(arr: Vec<i32>) -> Vec<i32> {
if arr.len() <= 1 {
return arr;
}
let pivot = arr[arr.len() / 2];
let mut left = Vec::new();
let mut right = Vec::new();
for x in arr {
if x < pivot {
left.push(x);
} else if x > pivot {
right.push(x);
}
}
let mut result = quick_sort(left);
result.push(pivot);
result.extend(quick_sort(right));
result
}
fn main() {
let arr = vec![3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5];
let sorted_arr = quick_sort(arr);
println!("{:?}", sorted_arr); // Output: [1, 1, 2, 3, 3, 4, 5, 5, 5, 6, 9]
}
Choosing the right option:
For most cases, Option 1 (in-place sorting) is the preferred approach in Rust due to its efficiency. Option 2 is provided for comparison and understanding but should generally be avoided for performance-critical applications. Remember to choose the appropriate integer type (i32
, i64
, etc.) based on the expected range of your input data.
Here's the equivalent Rust implementation of the Go quicksort function:
fn quick_sort(arr: Vec<i32>) -> Vec<i32> {
if arr.len() <= 1 {
return arr;
}
let pivot = arr[arr.len() / 2];
let mut left: Vec<i32> = Vec::new();
let mut right: Vec<i32> = Vec::new();
for &x in &arr {
if x < pivot {
left.push(x);
} else if x > pivot {
right.push(x);
}
}
let mut result = quick_sort(left);
result.push(pivot);
result.extend(quick_sort(right));
result
}
Key differences from the Go version:
- Uses
fn
instead offunc
- Type annotations are explicit
Vec<i32>
instead of slice&x
used in iteration to borrow values- Explicit
result
vector construction - Uses
push()
andextend()
instead ofappend()
- Implicit return of
result
Here's the equivalent function in Rust:
fn quick_sort(arr: Vec<i32>) -> Vec<i32> {
if arr.len() <= 1 {
return arr;
}
let pivot = arr[arr.len() / 2];
let mut left = Vec::new();
let mut right = Vec::new();
for x in arr.iter() {
if *x < pivot {
left.push(*x);
} else if *x > pivot {
right.push(*x);
}
}
let mut result = quick_sort(left);
result.push(pivot);
let right = quick_sort(right);
result.extend(right)
}
// or in a more idiomatic Rust way
fn quick_sort(arr: Vec<i32>) -> Vec<i32> {
if arr.len() <= 1 {
arr
} else {
let pivot = arr[arr.len() / 2];
let mut left = arr.iter()
.filter(|x| **x < pivot)
.cloned()
.collect::<Vec<_>>();
let mut right = arr.iter()
.filter(|x| **x > pivot)
.cloned()
.collect::<Vec<_>>();
let result = quick_sort(left);
result.into_iter().chain(std::iter::once(pivot)).chain(quick_sort(right)).collect()
}
}
Note: Rust uses Vec
instead of slices (like Go's []int
) because it needs to own the data. However, you can use iterators to simulate most of the Go-style behavior.
Also note that in the second version, we're using collect::<Vec<_>>()
instead of push
and extend
, this is because Rust's standard library doesn't have a chain
function on iterators like Go's append(append(...), ...)
function. Alternatively, we're collecting the whole Vec
and then extending it, which is more idiomatic in Rust.
You can use these functions like this:
fn main() {
let arr = vec![5, 2, 9, 1, 7, 3];
let sorted_arr = quick_sort(arr);
println!("{:?}", sorted_arr); // prints: [1, 2, 3, 5, 7, 9]
}
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
ChatGPT provided a detailed, well-documented translation with comprehensive explanations of key differences. Claude delivered a concise, efficient solution with good handling of equal elements. Gemini offered the most sophisticated implementation with in-place sorting and extensive test cases, though took longest to generate. Llama was fastest and provided two alternative implementations, but with less robust error handling. Response times varied significantly: Llama (2.4s), Claude (5.4s), ChatGPT (11.7s), Gemini (19.9s). ChatGPT and Gemini focused more on explanations, while Claude and Llama emphasized code efficiency.
Budget Tier
All models successfully translated the Go quicksort to Rust, but with varying approaches. Llama 3.1 8B was notably fastest (968ms) and provided two implementations - a direct translation and a more idiomatic Rust version using iterators. ChatGPT and Claude offered similar straightforward translations with good documentation. Gemini gave the most comprehensive response with both in-place and vector-based implementations, though took longer (7526ms). Llama's response stands out for balancing speed, correctness, and Rust idioms while explaining key differences between Go and Rust approaches.
TypeScript Interface to Python Dataclass
Convert a TypeScript interface definition to a Python dataclass. Useful for maintaining type definitions across Python and TypeScript codebases.
Prompt:
Transform this TypeScript interface to Python dataclass: interface User { id: number; name: string; email: string; age?: number; }
Performance Results
To transform the given TypeScript interface to a Python dataclass
, you will need to convert the TypeScript types to their respective Python types and handle the optional field using typing annotations. Here's how you can translate the interface:
TypeScript Interface:
interface User {
id: number;
name: string;
email: string;
age?: number;
}
Python dataclass
:
from dataclasses import dataclass
from typing import Optional
@dataclass
class User:
id: int
name: str
email: str
age: Optional[int] = None
In this conversion:
- TypeScript's
number
type is converted to Python'sint
. - TypeScript's
string
type is converted to Python'sstr
. - The optional
age
field in TypeScript is denoted byage?: number
and is translated toage: Optional[int] = None
, using theOptional
type from thetyping
module to indicate that this field can be either anint
orNone
.
from dataclasses import dataclass
from typing import Optional
@dataclass
class User:
id: int
name: str
email: str
age: Optional[int] = None
from dataclasses import dataclass
from typing import Optional
@dataclass
class User:
id: int
name: str
email: str
age: Optional[int] = None
Here's how you can transform the TypeScript interface into a Python dataclass:
from dataclasses import dataclass
from typing import Optional
@dataclass
class User:
id: int
name: str
email: str
age: Optional[int] = None
Explanation:
- We use the
@dataclass
decorator from Python'sdataclasses
module to define a dataclass. - The fields of the dataclass (
id
,name
,email
, andage
) correspond to the properties in the original TypeScript interface. - Since TypeScript's
number
data type is used forid
andage
, we use Python'sint
type for these fields. If floats were also supposed to be allowed, we could usefloat
orUnion[int, float]
with thetyping
module'sUnion
type if you're using Python 3.9 or earlier and don't want to import a new package. - We use Python's
str
type forname
andemail
, as these directly correspond to TypeScript'sstring
data type. - Since
age
is an optional property in the original TypeScript interface (denoted by the?
symbol), we use Python'sOptional
type to indicate thatage
may be either an integer orNone
(equivalent tonull
in TypeScript). We also assign a default value ofNone
to this field.
Here's an example of how you might use this dataclass:
# Create a User object with age specified
user_with_age = User(123, 'John Doe', 'john@example.com', 30)
# Create a User object without age specified
user_without_age = User(456, 'Jane Doe', 'jane@example.com')
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
You can transform the TypeScript interface to a Python dataclass as follows:
from dataclasses import dataclass
from typing import Optional
@dataclass
class User:
id: int
name: str
email: str
age: Optional[int] = None
In this Python code:
- We use the
@dataclass
decorator to create a class that automatically generates special methods like__init__()
and__repr__()
. - The
id
,name
, andemail
attributes are required, representing the TypeScript properties without the optional modifier. - The
age
attribute is optional, denoted byOptional[int]
, which allows it to be either anint
orNone
. The default value ofNone
means it is not required when creating an instance of theUser
class.
There are several ways to achieve this, depending on how you want to handle the optional age
field.
Option 1: Using typing.Optional
(Recommended)
This is the most straightforward and type-safe approach, mirroring the TypeScript's optional property behavior:
from dataclasses import dataclass
from typing import Optional
@dataclass
class User:
id: int
name: str
email: str
age: Optional[int] = None
This defines age
as optionally present; it defaults to None
if not provided during object creation.
Option 2: Using a default value directly (Less type-safe)
This approach is simpler but loses the explicit indication that age
is optional. It's less desirable from a type-hinting perspective:
from dataclasses import dataclass
@dataclass
class User:
id: int
name: str
email: str
age: int = 0 # Defaults to 0, not explicitly optional
Here, age
defaults to 0. While functional, it doesn't accurately reflect the optionality from the TypeScript interface. A value of 0 might be a valid age, making it difficult to distinguish between a user with age 0 and a user whose age is unknown.
Option 3: Handling optionality within methods (More complex)
For more advanced scenarios where you need to specifically handle the absence of age
in methods, you might choose to explicitly check for None
:
from dataclasses import dataclass
from typing import Optional
@dataclass
class User:
id: int
name: str
email: str
age: Optional[int] = None
def display_age(self):
if self.age is not None:
print(f"Age: {self.age}")
else:
print("Age not specified")
This separates the optionality handling from the data structure itself.
Recommendation: Option 1, using typing.Optional
, is the best approach because it maintains the type safety and accurately represents the original TypeScript interface. It clearly indicates that age
is an optional field while providing a sensible default value.
from dataclasses import dataclass
from typing import Optional
@dataclass
class User:
id: int
name: str
email: str
age: Optional[int] = None
The key differences from the TypeScript interface:
- Used
@dataclass
decorator - Added
from dataclasses import dataclass
- Used
Optional[int]
for the optional age field - Used
typing.Optional
to represent optional types = None
allows the age to be optional- Python uses type hints similar to TypeScript's type annotations
Here's how you can transform the given TypeScript interface into a Python dataclass:
from dataclasses import dataclass
@dataclass
class User:
"""A simple class representing a user."""
id: int
name: str
email: str
age: int | None = None
However, when using Python, it's more idiomatic to use type hints and let the type system (e.g. type checkers like mypy) handle type checks, rather than strictly enforcing type hints at runtime (as dataclasses do). You could choose to use the Python standard library dataclasses or use third-party libraries, but if you wanted to implement type hints without using the dataclasses decorator, your code would look like this:
class User:
"""A simple class representing a user."""
def __init__(self, id: int, name: str, email: str, age: int | None = None):
self.id = id
self.name = name
self.email = email
self.age = age
Here's an example which implements all features that were in the original typescript interface. Additionally it includes __repr__
(a string representation) method for printable outcome which also is part of the example since one would likely want code of this sort to support it for the data structure presented being the "User".
from dataclasses import dataclass
@dataclass
class User:
"""A simple class representing a user."""
id: int
name: str
email: str
age: int | None = None
def __repr__(self):
return f"User(id={self.id}, name='{self.name}', email='{self.email}', age={self.age})"
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
All models successfully converted the TypeScript interface to a Python dataclass with identical and correct implementations. Llama 70B provided the most comprehensive explanation with usage examples, while Claude and Gemini delivered clean, minimal solutions. ChatGPT included a helpful explanation of type mappings. Response times varied from Gemini's fastest at 1596ms to ChatGPT's slowest at 4176ms. For this straightforward conversion task, simpler responses from Claude and Gemini may be preferable, though Llama's detailed explanation could be valuable for learning purposes.
Budget Tier
Analyzing model performance on TypeScript-to-Python dataclass conversion: ChatGPT 4o Mini provided a concise, accurate solution with clear explanations in 2.7s. Gemini 1.5 Flash offered comprehensive alternatives with detailed explanations but took longer (3.7s). Claude 3.5 Haiku delivered a precise solution with key differences highlighted in 2.5s. Llama 3.1 8B was fastest (0.7s) and provided multiple implementation approaches, including idiomatic Python patterns and custom repr. All models successfully handled the optional age field, though Llama and Claude showed superior understanding of Python-specific conventions.
Python Decorator to JavaScript Property
Transform a Python property decorator into JavaScript getter syntax. Helpful for understanding property access patterns between languages.
Prompt:
Convert this Python decorator to JavaScript equivalent: @property def full_name(self): return f'{self.first_name} {self.last_name}'
Performance Results
In JavaScript, the concept of decorators isn't built-in like in Python, although proposed features for decorators exist in newer ECMAScript versions but are not widely adopted yet. However, you can achieve the same effect as a Python @property
decorator using JavaScript's getters. Here's how you can convert the given Python code using getter methods in a JavaScript class:
class Person {
constructor(firstName, lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
get fullName() {
return `${this.firstName} ${this.lastName}`;
}
}
// Example usage:
const person = new Person('John', 'Doe');
console.log(person.fullName); // Outputs: "John Doe"
In this JavaScript code:
- The
Person
class is defined with a constructor that initializesfirstName
andlastName
. - The
fullName
method is defined as a getter using theget
keyword. This means it can be accessed like a property without explicit function calls, mimicking the@property
decorator in Python. - You can then access
fullName
as if it were a standard property on an instance ofPerson
.
class Person {
get fullName() {
return `${this.firstName} ${this.lastName}`;
}
}
The JavaScript equivalent uses the get
keyword to define a getter property. Like Python's @property
decorator, this allows you to access fullName
as a property rather than a method:
const person = new Person();
person.firstName = "John";
person.lastName = "Doe";
console.log(person.fullName); // "John Doe"
The main differences are:
- JavaScript uses
get
instead of@property
- JavaScript uses camelCase naming convention
- No
self
parameter is needed sincethis
is implicit
There isn't a direct equivalent of Python's @property
decorator in JavaScript that achieves the exact same concise syntax. However, you can achieve the same functionality using getters. Here are a few ways to convert the given Python code to JavaScript:
1. Using get
inside a class definition:
This is the most common and recommended approach, mirroring Python's behavior most closely.
class Person {
constructor(firstName, lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
get fullName() {
return `${this.firstName} ${this.lastName}`;
}
}
const person = new Person("John", "Doe");
console.log(person.fullName); // Outputs "John Doe"
2. Using Object.defineProperty
:
This approach is more verbose but allows defining getters outside the class definition if needed.
class Person {
constructor(firstName, lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
}
Object.defineProperty(Person.prototype, "fullName", {
get: function() {
return `${this.firstName} ${this.lastName}`;
},
});
const person = new Person("John", "Doe");
console.log(person.fullName); // Outputs "John Doe"
3. Defining a method (less preferred):
While not technically a property, you can define a method that returns the full name. This is less preferred because it requires calling the method with parentheses ()
, unlike a true property.
class Person {
constructor(firstName, lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
getFullName() {
return `${this.firstName} ${this.lastName}`;
}
}
const person = new Person("John", "Doe");
console.log(person.getFullName()); // Outputs "John Doe" - Note the parentheses
Key Differences and Considerations:
- Explicit
get
keyword: JavaScript requires theget
keyword to define a getter. - No
@
syntax: JavaScript doesn't use the@
decorator syntax like Python. Object.defineProperty
flexibility: This method provides more control and allows defining getters/setters outside the class definition, which can be useful in some scenarios.- Method vs. Getter: Using a method (
getFullName()
) is functionally similar but differs in usage (requires parentheses) and doesn't truly emulate a property.
The first approach (using get
inside the class) is generally the most idiomatic and recommended way to achieve the desired behavior in JavaScript, providing the closest equivalent to Python's @property
. It's concise, clear, and integrates seamlessly within the class definition.
Here's how you can convert the Python decorator @property
to a JavaScript equivalent using a getter. In JavaScript, we use the get
keyword inside a class to define a getter method.
class Person {
constructor(first_name, last_name) {
this.first_name = first_name;
this.last_name = last_name;
}
get full_name() {
return `${this.first_name} ${this.last_name}`;
}
}
// Example usage
const person = new Person('John', 'Doe');
console.log(person.full_name); // Outputs: "John Doe"
In this example, full_name
is a getter method. This means you can call it like a property (person.full_name
), but under the hood, it's actually calling the getter method. This allows you to implement complex logic and calculations when getting the value of full_name
, which makes it behave more like a property than a method.
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
In JavaScript, you can create a similar behavior using getters. Here's how you can convert the Python @property
decorator to a JavaScript equivalent:
Python Code:
class Person:
def __init__(self, first_name, last_name):
self.first_name = first_name
self.last_name = last_name
@property
def full_name(self):
return f'{self.first_name} {self.last_name}'
Equivalent JavaScript Code:
class Person {
constructor(firstName, lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
get fullName() {
return `${this.firstName} ${this.lastName}`;
}
}
// Example usage:
const person = new Person('John', 'Doe');
console.log(person.fullName); // Output: John Doe
In the JavaScript version, the fullName
property is defined using a getter, which allows you to access it like a regular property but executes a function to return its value. This closely mimics the behavior of the Python @property
decorator.
There isn't a direct equivalent of Python's @property
decorator in JavaScript that works exactly the same way. Python's @property
creates a getter, setter, and deleter method all within a single decorator. JavaScript typically handles these separately using get
, set
, and deleteProperty
within an object's definition.
Here's how you can achieve similar functionality in JavaScript, using both a simple approach and one closer to the Python @property
style using a function to create the getter:
Method 1: Direct Object Definition
This is the most straightforward approach for a simple getter:
class Person {
constructor(first_name, last_name) {
this.first_name = first_name;
this.last_name = last_name;
}
get fullName() { // Note: camelCase is standard in JS
return `${this.first_name} ${this.last_name}`;
}
}
let person = new Person("John", "Doe");
console.log(person.fullName); // Output: John Doe
Method 2: Function to Mimic @property
(More Advanced)
This method creates a function that generates the getter (and optionally setter and deleter), making it more reusable if you need multiple properties with getters:
function property(getter, setter, deleter) {
return function(target, name, descriptor) {
descriptor.get = getter;
if (setter) descriptor.set = setter;
if (deleter) descriptor.deleteProperty = deleter;
return descriptor;
};
}
class Person {
constructor(first_name, last_name) {
this.first_name = first_name;
this.last_name = last_name;
}
@property(function() { return `${this.first_name} ${this.last_name}`; })
fullName;
}
let person = new Person("Jane", "Smith");
console.log(person.fullName); // Output: Jane Smith
The second method is closer to the spirit of the Python decorator but adds complexity. For simple getters like this example, the first method is usually preferred for its clarity and simplicity. Remember that JavaScript uses camelCase (fullName
instead of full_name
) for property names.
Here's the JavaScript equivalent:
class Person {
constructor(firstName, lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
get fullName() {
return `${this.firstName} ${this.lastName}`;
}
}
Key differences from Python:
- Use
get
keyword instead of@property
- Replace
self
with standard object contextthis
- Camel case method name (
fullName
instead offull_name
) - Use of template literal with backticks (`) for string interpolation
- Method is defined inside the class body
Usage example:
const person = new Person('John', 'Doe');
console.log(person.fullName); // Outputs: "John Doe"
The JavaScript getter behaves similarly to the Python @property
decorator, allowing you to access the method like a property without needing to call it with parentheses.
Here's how you can convert the given Python decorator to a JavaScript equivalent using classes and getters in JavaScript:
class Person {
constructor(firstName, lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
get fullName() {
return `${this.firstName} ${this.lastName}`;
}
}
// Example usage:
let person = new Person("John", "Doe");
console.log(person.fullName); // Outputs: John Doe
In JavaScript, getters are a way to allow classes to override the default behavior of property access. The fullName
property is not defined directly on the class, but instead a getter function is defined which returns the full name when accessed.
This is equivalent to the Python code @property def full_name(self): return f'{self.first_name} {self.last_name}'
, but in JavaScript.
Note: JavaScript property access can be much more complex than in Python. It can accept more than just name, like for example: test.firstName
, test["firstName"]
. This example above is designed to be the most direct JavaScript alternative to the given Python example.
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
Comparing the models' responses on converting a Python property decorator to JavaScript, all provided correct solutions using JavaScript's getter syntax. ChatGPT 4o and Gemini 1.5 Pro offered the most comprehensive explanations, with Gemini presenting multiple implementation approaches including Object.defineProperty. Claude and Llama gave more concise but accurate answers. Gemini took longest to respond (13.4s), while Llama was fastest (1s). Claude's response was notably efficient, providing key differences between Python and JavaScript syntax. All models correctly emphasized using the 'get' keyword and demonstrated proper usage through examples.
Budget Tier
All models provided correct solutions using JavaScript's get keyword to replicate Python's @property decorator. ChatGPT 4o Mini and Claude provided concise, clear explanations with example code. Gemini offered two approaches but was overly verbose. Llama gave accurate code but included unnecessary technical details. Performance-wise, Llama was significantly faster at 610ms, while others took 4000-5000ms. Claude's response was most efficient in terms of clarity and completeness while maintaining brevity. ChatGPT's response closely matched Claude's quality but took slightly longer.
Performance Verdict
Code Translation Model Performance Verdict
After analyzing the performance across multiple code translation tasks, here's how each model performed:
ChatGPT 4o: Strengths:
- Consistently accurate translations
- Clear, concise explanations
- Good balance between code and documentation Weaknesses:
- Moderate response times
- Sometimes lacks additional implementation alternatives
- Less comprehensive documentation compared to some competitors
Claude 3.5 Sonnet: Strengths:
- Fastest average response times
- Highly efficient, minimal solutions
- Good focus on language-specific differences Weaknesses:
- Sometimes overly concise
- Occasional missed opportunities for optimization
- Less comprehensive documentation
Gemini 1.5 Pro: Strengths:
- Most comprehensive explanations
- Multiple implementation approaches
- Excellent error handling and test cases Weaknesses:
- Significantly slower response times
- Sometimes overly verbose
- Can overwhelm with unnecessary detail
Llama 3.1 70B: Strengths:
- Very fast response times
- Detailed documentation with JSDoc
- Frequently provides multiple implementations Weaknesses:
- Less robust error handling
- Documentation quality can be inconsistent
- Sometimes prioritizes speed over completeness
Winner: Claude 3.5 Sonnet
While each model showed distinct strengths, Claude 3.5 Sonnet emerges as the overall winner for code translation tasks. Its combination of consistently fast response times, accurate translations, and efficient solutions makes it the most practical choice for real-world code translation needs. While Gemini often provided more comprehensive responses and Llama was frequently faster, Claude's balance of speed, accuracy, and practical utility gives it the edge. For users needing more detailed explanations or multiple implementation options, Gemini 1.5 Pro would be the better choice, despite its slower performance.
Budget Verdict
Performance Comparison Analysis: Code Translation Tasks
Winner: Llama 3.1 8B
Speed Performance:
- Llama consistently demonstrated superior speed, averaging 500-1000ms response times
- Claude typically performed second-best with 2500-3500ms responses
- ChatGPT showed moderate speeds around 3000-5000ms
- Gemini was consistently slowest, often exceeding 10000ms
Technical Accuracy: All models achieved high accuracy in basic translations, but with distinct characteristics:
Llama 3.1 8B (Winner): Strengths:
- Fastest response times consistently
- Comprehensive technical depth
- Multiple implementation approaches
- Strong focus on language-specific idioms
- Excellent balance of speed and detail Weaknesses:
- Occasionally provided excessive technical details
- Some responses could be more concise
Claude 3.5 Haiku: Strengths:
- Very clear and concise responses
- Excellent explanation of key differences
- Consistent quality across tasks Weaknesses:
- Sometimes missed opportunities for optimization
- Limited additional implementation alternatives
ChatGPT 4o Mini: Strengths:
- Consistently accurate translations
- Well-balanced responses
- Good documentation Weaknesses:
- Average response times
- Sometimes missed advanced language features
Gemini 1.5 Flash: Strengths:
- Most comprehensive explanations
- Multiple implementation options
- Strong optimization suggestions Weaknesses:
- Significantly slower response times
- Often overly verbose
- Sometimes deviated from direct translation requirements
The verdict favors Llama 3.1 8B due to its exceptional combination of speed, accuracy, and technical depth. While all models performed competently, Llama's consistent sub-second response times while maintaining high-quality output sets it apart. Its ability to provide multiple implementation approaches and language-specific optimizations, while maintaining speed, makes it the superior choice for code translation tasks.
Conclusion
Conclusion
After extensive testing and analysis of both performance and budget tier AI models across various code translation tasks, several key findings emerge. The performance tier, led by Claude 3.5 Sonnet, demonstrates superior accuracy and comprehensiveness but at the cost of longer processing times and higher resource requirements. In contrast, the budget tier, dominated by Llama 3.1 8B, offers remarkably fast performance while maintaining impressive technical accuracy.
For professional applications requiring detailed documentation and multiple implementation approaches, performance tier models, particularly Claude 3.5 Sonnet and Gemini 1.5 Pro, remain the optimal choice. However, for rapid development cycles and routine code translations, budget tier models, especially Llama 3.1 8B, provide an excellent balance of speed and accuracy at a fraction of the cost.
The consistent performance of Llama models across both tiers suggests a promising direction for future AI development, potentially narrowing the gap between high-performance and budget-friendly options. As these models continue to evolve, we may see further improvements in processing speed while maintaining or enhancing translation accuracy and comprehensiveness.
For developers and organizations choosing between these options, the decision should ultimately depend on specific use cases, time constraints, and budget considerations. While performance tier models offer additional features and robustness, the impressive capabilities of budget tier models make them increasingly viable for many practical applications in code translation tasks.