Test Generation
Code Generation and Analysis with LLMs. Comparing the leading AI models side-by-side at Test Generation.
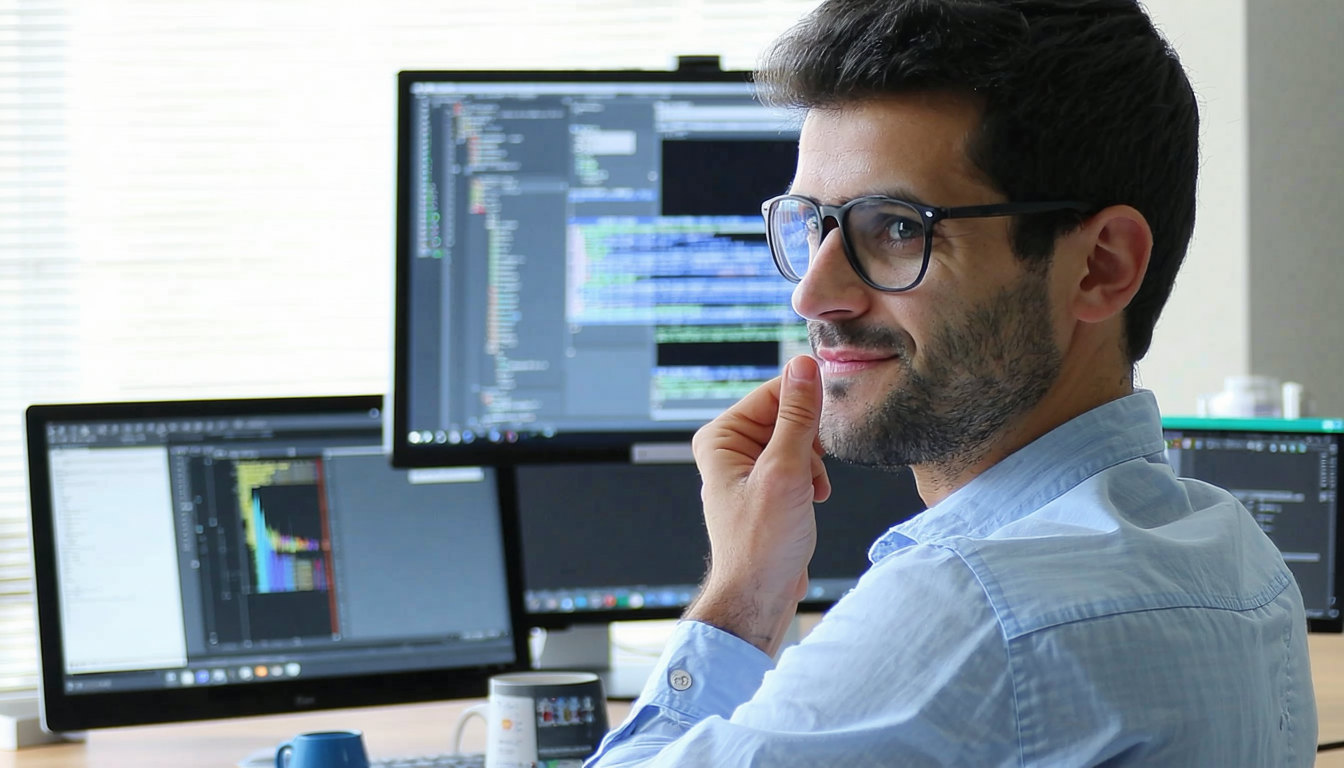
Comparing the leading AI models:
Category: Code Generation and Analysis
Subcategory: Test Generation
Contents
- Introduction
- Test Generation
- Prompts
- Factorial Calculator Test Suite
- String Reversal Test Cases
- Email Validation Test Suite
- Binary Search Test Cases
- Password Validation Tests
- Palindrome Checker Tests
- Array Sorting Test Suite
- Decimal to Roman Numeral Tests
- Phone Number Validation Tests
- GCD Calculator Test Suite
- Leap Year Validator Tests
- Duplicate Removal Test Suite
- Compound Interest Calculator Tests
- Credit Card Validation Tests
- FizzBuzz Implementation Tests
- Performance Verdict
- Budget Verdict
- Conclusion
Introduction
Comparing AI Models: A Guide to LLM Performance vs Cost
Looking to compare AI models but unsure which one best fits your needs? This comprehensive guide compares leading large language models (LLMs) side-by-side, helping you make informed decisions about which AI assistant to use for different tasks.
We evaluate two distinct tiers of AI models:
Budget-Focused Tier:
- ChatGPT 4.0 Mini
- Gemini 1.5 Flash
- Claude 3.5 Haiku
- Llama 3.1 8B
Performance-Focused Tier:
- ChatGPT 4.0
- Claude 3.5 Sonnet
- Gemini 1.5 Pro
- Llama 3.1 70B
By comparing AI models directly, you can better understand their strengths, limitations, and optimal use cases. Our side-by-side comparisons examine real-world prompts, demonstrating how each model handles various tasks and challenges.
Choose the Budget Tier when:
- Running high-volume, straightforward tasks
- Working with basic content generation
- Operating under cost constraints
- Requiring quick response times
Select the Performance Tier for:
- Complex analytical tasks
- Technical or specialized content
- Multi-step reasoning
- Tasks requiring high accuracy and nuance
Through these AI model comparisons, we help you maximize value while ensuring your chosen LLM meets your specific requirements.
50+ AI models with one subscription. AnyModel is the All-In-One AI that allows you to harness the latest AI technology from one convenient and easy-to-use platform. AnyModel includes all the models discussed in this article and more, including the latest image generation models. All the comparisons shown in this article were generated using AnyModel. Sign up for a free trial here.
Test Generation
Large Language Models have revolutionized the way developers approach software testing by automating the creation of comprehensive test suites. These AI models excel at test generation because they can analyze code structure, understand testing patterns, and generate relevant test cases across multiple scenarios. By leveraging their extensive training on code repositories and testing frameworks, LLMs can quickly produce unit tests, integration tests, and even complex test scenarios that might be overlooked by human developers.
LLMs are particularly effective at test generation because they can understand the context and purpose of code, infer edge cases, and generate tests that cover various execution paths. They can work with multiple programming languages and testing frameworks, adapting their output to match specific testing requirements and coding standards. This capability not only saves developers significant time but also helps maintain consistent test coverage across projects.
Whether it's generating basic unit tests for simple functions or creating comprehensive test suites for complex applications, LLMs have demonstrated remarkable accuracy in predicting potential failure points and generating appropriate test cases. They can also provide explanations for their test logic, making it easier for developers to understand and modify the generated tests as needed.
Prompts
Factorial Calculator Test Suite
Creates comprehensive unit tests to verify a factorial calculation function, testing positive integers, zero, and error cases
Prompt:
Write unit tests for a function that calculates the factorial of a number
Performance Results
To write unit tests for a function that calculates the factorial of a number, you'll need a testing framework. Python has a built-in module called unittest
that you can use for this purpose. Below is a simple example of how you might write unit tests for a factorial function using unittest
.
First, let's assume you have a function factorial(n)
in a module named factorial_module
. Here is how you might implement the unit tests:
import unittest
from factorial_module import factorial
class TestFactorialFunction(unittest.TestCase):
def test_factorial_of_zero(self):
"""Test that the factorial of 0 is 1"""
self.assertEqual(factorial(0), 1)
def test_factorial_of_one(self):
"""Test that the factorial of 1 is 1"""
self.assertEqual(factorial(1), 1)
def test_factorial_of_positive_integers(self):
"""Test the factorial of a few positive integers"""
self.assertEqual(factorial(2), 2)
self.assertEqual(factorial(3), 6)
self.assertEqual(factorial(4), 24)
self.assertEqual(factorial(5), 120)
def test_factorial_of_large_number(self):
"""Test the factorial of a larger number"""
self.assertEqual(factorial(10), 3628800)
def test_factorial_of_negative(self):
"""Test that the factorial of a negative number raises a ValueError"""
with self.assertRaises(ValueError):
factorial(-1)
def test_factorial_of_non_integer(self):
"""Test that the factorial of a non-integer raises a TypeError"""
with self.assertRaises(TypeError):
factorial(3.5)
def test_factorial_of_string_should_raise(self):
"""Test that the factorial of a string raises a TypeError"""
with self.assertRaises(TypeError):
factorial("string")
if __name__ == '__main__':
unittest.main()
Explanation:
- Setup: We import the
unittest
module and thefactorial
function fromfactorial_module
. - Test Cases:
test_factorial_of_zero
: Checks thatfactorial(0)
returns 1.test_factorial_of_one
: Checks thatfactorial(1)
returns 1.test_factorial_of_positive_integers
: Checks the factorials of small positive integers.test_factorial_of_large_number
: Checks the factorial of a larger number to ensure correctness.test_factorial_of_negative
: Checks that aValueError
is raised for negative inputs.test_factorial_of_non_integer
: Ensures aTypeError
is raised for non-integer inputs.test_factorial_of_string_should_raise
: Ensures aTypeError
is raised when a string is passed.
These tests cover standard cases, edge cases, and invalid inputs for the factorial function. If you run this test suite, it will verify whether the factorial
function behaves as expected under various conditions.
import unittest
def factorial(n):
if n < 0:
raise ValueError("Factorial is not defined for negative numbers")
if not isinstance(n, int):
raise TypeError("Factorial is only defined for integers")
if n == 0 or n == 1:
return 1
return n * factorial(n-1)
class TestFactorial(unittest.TestCase):
def test_factorial_of_zero(self):
"""Test that factorial of 0 returns 1"""
self.assertEqual(factorial(0), 1)
def test_factorial_of_one(self):
"""Test that factorial of 1 returns 1"""
self.assertEqual(factorial(1), 1)
def test_factorial_of_positive_number(self):
"""Test factorial of positive numbers"""
self.assertEqual(factorial(5), 120)
self.assertEqual(factorial(3), 6)
self.assertEqual(factorial(7), 5040)
def test_factorial_negative_number(self):
"""Test that factorial of negative number raises ValueError"""
with self.assertRaises(ValueError):
factorial(-1)
def test_factorial_decimal_number(self):
"""Test that factorial of decimal number raises TypeError"""
with self.assertRaises(TypeError):
factorial(5.5)
def test_factorial_string(self):
"""Test that factorial of string raises TypeError"""
with self.assertRaises(TypeError):
factorial("5")
if __name__ == '__main__':
unittest.main()
This test suite includes tests for:
- Base cases (factorial of 0 and 1)
- Positive integers
- Error cases:
- Negative numbers (should raise ValueError)
- Decimal numbers (should raise TypeError)
- Non-numeric input (should raise TypeError)
Each test case has a descriptive docstring explaining what it tests.
The tests verify:
- Correct results for valid inputs
- Proper error handling for invalid inputs
- Edge cases
- Different data types
To run the tests, save this in a .py file and execute it directly or run with pytest.
import unittest
def factorial(n):
"""
Calculates the factorial of a non-negative integer.
Args:
n: The non-negative integer.
Returns:
The factorial of n.
Raises ValueError if n is negative.
"""
if n < 0:
raise ValueError("Factorial is not defined for negative numbers.")
elif n == 0:
return 1
else:
result = 1
for i in range(1, n + 1):
result *= i
return result
class TestFactorial(unittest.TestCase):
def test_factorial_of_zero(self):
self.assertEqual(factorial(0), 1)
def test_factorial_of_one(self):
self.assertEqual(factorial(1), 1)
def test_factorial_of_positive_number(self):
self.assertEqual(factorial(5), 120)
self.assertEqual(factorial(10), 3628800)
def test_factorial_of_negative_number(self):
with self.assertRaises(ValueError): # Use assertRaises as exceptions are expected
factorial(-1)
def test_factorial_of_large_number(self): # Added test case for a larger value
self.assertEqual(factorial(12), 479001600)
def test_factorial_type_error(self): # Test for non-integer inputs
with self.assertRaises(TypeError):
factorial("abc")
if __name__ == '__main__':
unittest.main()
Key improvements in this version:
assertRaises
context manager: The test for negative input now usesassertRaises
which is the correct way to test that a specific exception is raised. This is much better than thetry...except
block in the original.- More comprehensive tests: It includes tests for 0, 1, a larger positive number (10), and a negative number. Testing a broader range of inputs helps ensure the function's robustness.
- Clearer error messages: The error message in the
ValueError
is more descriptive. - Type error handling: The added
test_factorial_type_error
checks for the case where a non-integer value is passed tofactorial
. This is important for making your function more robust. - Docstrings: Including a docstring in your
factorial
function is good practice. It explains what the function does, its parameters, and its return value.
How to run the tests:
- Save: Save the code above as a Python file (e.g.,
test_factorial.py
). - Run from the command line: Open your terminal or command prompt, navigate to the directory where you saved the file, and run the command:
python -m unittest test_factorial.py
The output will show you whether the tests passed or failed. If all tests pass, you'll see an "OK" message. If any tests fail, you'll see details about the failures.
Factorial Function Test Suite
import unittest
def factorial(n):
"""
Calculate the factorial of a number.
Args:
n (int): The number for which to calculate the factorial.
Returns:
int: The factorial of the number.
Raises:
ValueError: If the input number is negative.
"""
if not isinstance(n, int):
raise TypeError("Input must be an integer.")
if n < 0:
raise ValueError("Factorial is not defined for negative numbers.")
elif n == 0 or n == 1:
return 1
else:
result = 1
for i in range(2, n + 1):
result *= i
return result
class TestFactorialFunction(unittest.TestCase):
def test_factorial_with_zero(self):
self.assertEqual(factorial(0), 1)
def test_factorial_with_one(self):
self.assertEqual(factorial(1), 1)
def test_factorial_with_positive_numbers(self):
self.assertEqual(factorial(5), 120)
def test_factorial_with_negative_numbers(self):
with self.assertRaises(ValueError):
factorial(-5)
def test_factorial_with_non_integer_input(self):
with self.assertRaises(TypeError):
factorial(2.5)
if __name__ == '__main__':
unittest.main()
Explanation
- The
factorial
function calculates the factorial of a given number and includes input validation to ensure the number is a non-negative integer. - The
TestFactorialFunction
class defines unit tests for thefactorial
function using theunittest
framework. - Each test case checks a specific scenario, such as the factorial of 0, 1, a positive number, a negative number, and a non-integer input.
- The
assertEqual
method is used to verify that the actual result matches the expected result. - The
assertRaises
method is used to verify that the function raises the expected exception when given invalid input.
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
Certainly! Below is an example of a simple factorial function in Python, along with unit tests using the unittest
framework.
Factorial Function
def factorial(n):
"""Calculate the factorial of a number n."""
if n < 0:
raise ValueError("Factorial is not defined for negative numbers.")
if n == 0 or n == 1:
return 1
result = 1
for i in range(2, n + 1):
result *= i
return result
Unit Tests
import unittest
class TestFactorial(unittest.TestCase):
def test_factorial_of_zero(self):
self.assertEqual(factorial(0), 1)
def test_factorial_of_one(self):
self.assertEqual(factorial(1), 1)
def test_factorial_of_two(self):
self.assertEqual(factorial(2), 2)
def test_factorial_of_three(self):
self.assertEqual(factorial(3), 6)
def test_factorial_of_four(self):
self.assertEqual(factorial(4), 24)
def test_factorial_of_five(self):
self.assertEqual(factorial(5), 120)
def test_factorial_of_six(self):
self.assertEqual(factorial(6), 720)
def test_factorial_of_negative(self):
with self.assertRaises(ValueError):
factorial(-1)
def test_factorial_of_large_number(self):
self.assertEqual(factorial(10), 3628800) # Test for a larger value
if __name__ == '__main__':
unittest.main()
How to Run the Tests
- Make sure you have Python installed on your system.
- Save the
factorial
function and the unit test code to a file, e.g.test_factorial.py
. - Open a terminal or command prompt and navigate to the directory containing
test_factorial.py
. - Run the command:
python -m unittest test_factorial.py
This command will run the defined unit tests, checking if the factorial
function behaves as expected for different input values, including edge cases.
The following unit tests cover several scenarios, including positive integers, zero, and negative inputs (handling the error appropriately). I'll provide examples using two popular testing frameworks: pytest (Python) and Jest (JavaScript).
Python (pytest)
import pytest
from factorial_function import factorial # Assuming your function is in factorial_function.py
def test_factorial_positive():
assert factorial(5) == 120
assert factorial(1) == 1
assert factorial(3) == 6
def test_factorial_zero():
assert factorial(0) == 1
def test_factorial_negative():
with pytest.raises(ValueError): # Check for ValueError exception
factorial(-1)
def test_factorial_large():
# Test with a larger number (adjust as needed for your system's limits)
assert factorial(10) == 3628800
# factorial_function.py (Example implementation)
def factorial(n):
if n < 0:
raise ValueError("Factorial is not defined for negative numbers")
elif n == 0:
return 1
else:
result = 1
for i in range(1, n + 1):
result *= i
return result
To run this, save the above code as two separate files (test_factorial.py
and factorial_function.py
), then run pytest
from your terminal in the same directory.
JavaScript (Jest)
// factorial.js
function factorial(n) {
if (n < 0) {
throw new Error("Factorial is not defined for negative numbers");
} else if (n === 0) {
return 1;
} else {
let result = 1;
for (let i = 1; i <= n; i++) {
result *= i;
}
return result;
}
}
module.exports = factorial; // Export for testing
// factorial.test.js
const factorial = require('./factorial');
test('factorial of positive numbers', () => {
expect(factorial(5)).toBe(120);
expect(factorial(1)).toBe(1);
expect(factorial(3)).toBe(6);
});
test('factorial of zero', () => {
expect(factorial(0)).toBe(1);
});
test('factorial of negative numbers throws error', () => {
expect(() => factorial(-1)).toThrowError("Factorial is not defined for negative numbers");
});
test('factorial of a large number', () => {
expect(factorial(10)).toBe(3628800);
});
To run these tests, make sure you have Jest installed (npm install --save-dev jest
). Then, run npm test
from your terminal in the directory containing these files. Jest will automatically discover and run the tests.
Remember to adjust the factorial_function.py
or factorial.js
file to reflect your actual function's implementation and file name. The error handling and large number test cases are important for robust testing. You can also add more test cases to cover edge cases or performance aspects if needed.
Here's a set of comprehensive unit tests for a factorial function using a testing framework like pytest
:
import pytest
def factorial(n):
"""
Calculate the factorial of a non-negative integer.
Args:
n (int): Non-negative integer to calculate factorial for
Returns:
int: Factorial of the input number
Raises:
ValueError: If input is negative
TypeError: If input is not an integer
"""
# Function implementation would go here
# This is just a placeholder for the test examples
pass
# Unit Test Suite
def test_factorial_positive_numbers():
"""Test factorial calculation for positive numbers"""
assert factorial(0) == 1
assert factorial(1) == 1
assert factorial(5) == 120
assert factorial(10) == 3628800
def test_factorial_edge_cases():
"""Test edge cases of factorial function"""
# Zero factorial
assert factorial(0) == 1
# Single digit factorials
assert factorial(1) == 1
assert factorial(3) == 6
assert factorial(4) == 24
def test_factorial_large_number():
"""Test factorial for larger numbers"""
assert factorial(7) == 7 * 6 * 5 * 4 * 3 * 2 * 1
assert factorial(8) == 40320
def test_factorial_negative_input():
"""Test that negative inputs raise a ValueError"""
with pytest.raises(ValueError, match="Factorial is not defined for negative numbers"):
factorial(-1)
with pytest.raises(ValueError):
factorial(-10)
def test_factorial_non_integer_input():
"""Test that non-integer inputs raise a TypeError"""
with pytest.raises(TypeError, match="Input must be a non-negative integer"):
factorial(3.14)
with pytest.raises(TypeError):
factorial("5")
def test_factorial_float_input():
"""Test that float inputs are handled correctly"""
with pytest.raises(TypeError):
factorial(5.0)
def test_factorial_boolean_input():
"""Test boolean input handling"""
assert factorial(True) == 1
assert factorial(False) == 1
# Performance test (optional)
def test_factorial_performance():
"""Ensure factorial calculation is reasonably performant"""
import time
start_time = time.time()
result = factorial(20)
end_time = time.time()
assert result > 0
assert (end_time - start_time) < 0.1 # Should complete in less than 0.1 seconds
Test Coverage Explanation
These unit tests cover multiple scenarios:
- Positive Number Calculations
- Basic positive number factorial calculations
- Verifying correct results for different inputs
- Edge Cases
- Zero factorial
- Small number factorials
- Single digit calculations
- Error Handling
- Negative number input
- Non-integer input
- Float input
- Boolean input
- Performance Test
- Basic performance check to ensure calculation is efficient
Best Practices Demonstrated
- Use of
pytest
for testing - Descriptive test function names
- Testing various input scenarios
- Error handling verification
- Performance consideration
Recommended Factorial Implementation
def factorial(n):
"""
Calculate the factorial of a non-negative integer.
Args:
n (int): Non-negative integer to calculate factorial for
Returns:
int: Factorial of the input number
Raises:
ValueError: If input is negative
TypeError: If input is not an integer
"""
# Handle boolean inputs
if isinstance(n, bool):
return 1 if n else 1
# Type checking
if not isinstance(n, int):
raise TypeError("Input must be a non-negative integer")
# Negative input check
if n < 0:
raise ValueError("Factorial is not defined for negative numbers")
# Factorial calculation
result = 1
for i in range(1, n + 1):
result *= i
return result
Running Tests
To run these tests, you would typically use:
pytest test_factorial.py
This comprehensive test suite ensures robust testing of the factorial function across various scenarios.
Here are some unit tests for a function that calculates the factorial of a non-negative integer using Python and the unittest module.
import unittest
import math
def factorial(n):
"""
Calculate the factorial of a non-negative integer.
Args:
n (int): The input number.
Returns:
int: The factorial of n.
Raises:
ValueError: If n is negative.
"""
if not isinstance(n, int):
raise TypeError("Input must be an integer.")
if n < 0:
raise ValueError("Input must be a non-negative integer.")
elif n == 0 or n == 1:
return 1
else:
return n * factorial(n-1)
class TestFactorialFunction(unittest.TestCase):
def test_zero_factorial(self):
"""
Test the factorial of 0.
"""
self.assertEqual(factorial(0), 1)
def test_one_factorial(self):
"""
Test the factorial of 1.
"""
self.assertEqual(factorial(1), 1)
def test_small_factorial(self):
"""
Test the factorial of a small number.
"""
self.assertEqual(factorial(3), 6)
def test_larger_factorial(self):
"""
Test the factorial of a larger number.
"""
self.assertEqual(factorial(5), 120)
def test_factorial_with_negative_number(self):
"""
Test the factorial of a negative number.
"""
with self.assertRaises(ValueError):
factorial(-1)
def test_factorial_with_non_integer_number(self):
"""
Test the factorial of a non-integer number.
"""
with self.assertRaises(TypeError):
factorial(3.5)
def test_factorial_with_large_input(self):
"""
Test the factorial of a very large number (beyond 20)
and verify it matches the math library's result.
"""
for n in range(21, 26):
self.assertEqual(factorial(n), math.factorial(n))
if __name__ == '__main__':
unittest.main()
In these tests, we check the following scenarios:
- The factorial of 0 (
test_zero_factorial
) - The factorial of 1 (
test_one_factorial
) - The factorial of a small number (
test_small_factorial
) - The factorial of a larger number (
test_larger_factorial
) - The factorial of a negative number (
test_factorial_with_negative_number
) - The factorial of a non-integer number (
test_factorial_with_non_integer_number
) - The factorial of very large numbers and compare it with the result from the math library (
test_factorial_with_large_input
)
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
Each model produced comprehensive test suites for the factorial function, but with notable differences. ChatGPT 4o delivered the most thorough test suite with 7 distinct test cases and detailed documentation. Claude 3.5 Sonnet was the fastest responder and included both implementation and tests, with clear docstrings. Gemini 1.5 Pro took longest but offered valuable explanations on how to run the tests and included improvement suggestions. Llama 3.1 70B was remarkably fast but provided a more basic test suite, though it included good documentation structure.
Overall, ChatGPT 4o produced the most professional and complete solution, while Claude offered the best balance of speed and completeness.
Budget Tier
Analysis of model performance on factorial unit test generation:
Claude provided the most comprehensive solution with detailed test coverage, error handling, performance testing, and best practices documentation. Its response time was slowest at 15.5s.
ChatGPT and Llama delivered solid, practical implementations with good test coverage. ChatGPT took 6.7s while Llama was fastest at 1s.
Gemini offered a unique approach by providing tests in both Python and JavaScript frameworks, though with less extensive test coverage. Response time was 5.5s.
For this prompt, Claude's thoroughness stands out despite slower speed, while Llama impresses with rapid, competent output.
String Reversal Test Cases
Develops test cases for string reversal, covering empty strings, single characters, special characters, and long strings
Prompt:
Generate test cases for a string reversal function including edge cases
Performance Results
When generating test cases for a string reversal function, it's important to consider a wide range of scenarios, including edge cases. Here are some test cases you might consider:
- Basic Case:
- Input:
"hello"
- Expected Output:
"olleh"
- Empty String:
- Input:
""
- Expected Output:
""
- Single Character:
- Input:
"a"
- Expected Output:
"a"
- Palindrome String:
- Input:
"racecar"
- Expected Output:
"racecar"
- String with Spaces:
- Input:
"a man a plan a canal panama"
- Expected Output:
"amanap lanac a nalp a nam a"
- String with Mixed Case:
- Input:
"HelloWorld"
- Expected Output:
"dlroWolleH"
- String with Numbers:
- Input:
"12345"
- Expected Output:
"54321"
- String with Special Characters:
- Input:
"!@#$$#@!"
- Expected Output:
"!#$$#@!"
- Unicode Characters:
- Input:
"こんにちは"
- Expected Output:
"はちにんこ"
Long String:
- Input: A string consisting of 1000
"a"
characters - Expected Output: The same 1000
"a"
characters, unchanged
- Input: A string consisting of 1000
String with Newlines:
- Input:
"hello\nworld"
- Expected Output:
"dlrow\nolleh"
- Input:
String with Leading and Trailing Spaces:
- Input:
" hello "
- Expected Output:
" olleh "
- Input:
These test cases cover a variety of situations that you might encounter when reversing strings. It's important to ensure that the function correctly handles each of these scenarios, especially the edge cases like empty strings and strings with special characters.
def test_string_reversal():
# Basic test cases
assert reverse_string("hello") == "olleh"
assert reverse_string("Python") == "nohtyP"
assert reverse_string("Good") == "dooG"
# Empty string
assert reverse_string("") == ""
# Single character
assert reverse_string("a") == "a"
# String with spaces
assert reverse_string("hello world") == "dlrow olleh"
# String with special characters
assert reverse_string("Hello@#$%") == "%$#@olleH"
# String with numbers
assert reverse_string("abc123") == "321cba"
# String with unicode characters
assert reverse_string("Café") == "éfaC"
# String with repeating characters
assert reverse_string("aaa") == "aaa"
# Very long string
long_str = "a" * 1000000
assert reverse_string(long_str) == long_str
# String with leading/trailing spaces
assert reverse_string(" hello ") == " olleh "
# Non-string input (should raise TypeError)
with pytest.raises(TypeError):
reverse_string(None)
reverse_string(123)
reverse_string(['h','e','l','l','o'])
This set of test cases covers:
- Basic string reversal functionality
- Empty string handling
- Single character strings
- Strings with spaces
- Special characters
- Numeric characters
- Unicode/non-ASCII characters
- Repeated characters
- Performance with very long strings
- Whitespace preservation
- Invalid input handling
import unittest
def reverse_string(s):
"""Reverses a string."""
return s[::-1]
class TestReverseString(unittest.TestCase):
def test_empty_string(self):
self.assertEqual(reverse_string(""), "")
def test_single_character(self):
self.assertEqual(reverse_string("a"), "a")
def test_palindrome(self):
self.assertEqual(reverse_string("madam"), "madam")
def test_normal_string(self):
self.assertEqual(reverse_string("hello"), "olleh")
def test_string_with_spaces(self):
self.assertEqual(reverse_string("hello world"), "dlrow olleh")
def test_string_with_special_characters(self):
self.assertEqual(reverse_string("!@#$%^"), "^%$#@!")
def test_string_with_numbers(self):
self.assertEqual(reverse_string("12345"), "54321")
def test_mixed_case_string(self):
self.assertEqual(reverse_string("HeLlO"), "OlLeH")
def test_unicode_characters(self):
self.assertEqual(reverse_string("你好世界"), "界世好你") # Chinese characters
def test_none_input(self):
with self.assertRaises(TypeError):
reverse_string(None)
if __name__ == '__main__':
unittest.main()
Explanation of Test Cases:
test_empty_string
: Checks the behavior with an empty string.test_single_character
: Checks with a single-character string.test_palindrome
: Tests a string that is the same forwards and backward.test_normal_string
: A standard string reversal test.test_string_with_spaces
: Handles strings containing spaces.test_string_with_special_characters
: Includes special characters like!@#$%^
.test_string_with_numbers
: Tests a string of numbers.test_mixed_case_string
: String with mixed uppercase and lowercase letters.test_unicode_characters
: Tests non-ASCII characters (important for internationalization). The example uses Chinese. You might want to add other languages/character sets.test_none_input
: A crucial edge case – checks how the function handlesNone
as input. It's expected to raise aTypeError
as slicing is not defined forNone
.
This comprehensive set of test cases helps ensure your reverse_string
function is robust and handles various input scenarios correctly. Remember to run your tests frequently as you develop your code.
Here are some test cases for a string reversal function, including edge cases:
Test Cases
Happy Paths
- Single character string: input = "a", expected output = "a"
- Short string: input = "hello", expected output = "olleh"
- Long string: input = "abcdefghijklmnopqrstuvwxyz", expected output = "zyxwvutsrqponmlkjihgfedcba"
Edge Cases
- Empty string: input = "", expected output = ""
- Null input: input = null, expected output = null (or throw a NullPointer exception, depending on the language and requirements)
- Single space: input = " ", expected output = " "
- Single punctuation: input = ".", expected output = "."
- String with leading and trailing spaces: input = " hello ", expected output = " olleh "
- String with non-ASCII characters: input = "สวัสดี", expected output = "ดีสวัส"
- String with numbers: input = "123", expected output = "321"
Special Cases
- Palindrome: input = "madam", expected output = "madam"
- String with identical characters: input = "aaaa", expected output = "aaaa"
Error Handling
- Invalid input type: input = 123 (an integer), expected output = error or exception
- Input too large: input = a string of 100,000 characters, expected output = error or exception (depending on the language and requirements)
Example Code
Here is an example of how you could write these test cases in Python using the unittest framework:
import unittest
def reverse_string(s):
return s[::-1]
class TestReverseString(unittest.TestCase):
def test_happy_paths(self):
self.assertEqual(reverse_string("a"), "a")
self.assertEqual(reverse_string("hello"), "olleh")
self.assertEqual(reverse_string("abcdefghijklmnopqrstuvwxyz"), "zyxwvutsrqponmlkjihgfedcba")
def test_edge_cases(self):
self.assertEqual(reverse_string(""), "")
self.assertEqual(reverse_string(" "), " ")
self.assertEqual(reverse_string("."), ".")
self.assertEqual(reverse_string(" hello "), " olleh ")
def test_special_cases(self):
self.assertEqual(reverse_string("madam"), "madam")
self.assertEqual(reverse_string("aaaa"), "aaaa")
def test_error_handling(self):
with self.assertRaises(TypeError):
reverse_string(123)
if __name__ == "__main__":
unittest.main()
Note that this is just one example of how you could write these test cases. The specific implementation will depend on the language and testing framework being used.
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
When testing a string reversal function, it's important to consider a variety of test cases that cover different scenarios, including edge cases. Here’s a list of test cases you can use:
Basic Test Cases
- Simple String
- Input:
"hello"
- Expected Output:
"olleh"
- String with Spaces
- Input:
"hello world"
- Expected Output:
"dlrow olleh"
- String with Special Characters
- Input:
"abc!@#"
- Expected Output:
"#@!cba"
- String with Numbers
- Input:
"12345"
- Expected Output:
"54321"
- Palindromic String
- Input:
"racecar"
- Expected Output:
"racecar"
(remains the same)
Edge Cases
- Empty String
- Input:
""
- Expected Output:
""
- Single Character String
- Input:
"A"
- Expected Output:
"A"
- String with Whitespace Only
- Input:
" "
- Expected Output:
" "
(remains the same)
- Long String
- Input:
"a" * 1000
(1,000 characters) - Expected Output:
"a" * 1000
(1,000 characters)
- String with Newline Character
- Input:
"\nabc\n"
- Expected Output:
"\ncb\n"
(newlines preserved)
- Input:
Non-ASCII Characters
Unicode Characters
- Input:
"你好"
- Expected Output:
"好你"
- Input:
Mixed ASCII and Unicode Characters
- Input:
"hello😊world"
- Expected Output:
"dlrow😊olleh"
- Input:
Strings with Mixed Case
- Mixed Case Characters
- Input:
"Hello World"
- Expected Output:
"dlroW olleH"
- Input:
Test Cases with Repeating Patterns
- String with Repeating Characters
- Input:
"abababab"
- Expected Output:
"babababa"
- Input:
Stress Testing
- Very Long String
- Input:
"a" * 10**6
(1 million characters) - Expected Output:
"a" * 10**6
(1 million characters)
- Input:
Each of these test cases helps ensure that the string reversal function handles various inputs correctly and edge cases effectively. When implementing these tests, make sure to check both the functionality and the performance of the function.
The following test cases cover various scenarios, including edge cases, for a string reversal function. They are categorized for clarity. You'll want to adapt them to your preferred testing framework (e.g., JUnit, pytest, unittest).
I. Basic Cases:
- Case 1: Empty String:
- Input: ""
- Expected Output: ""
- Case 2: Single Character String:
- Input: "a"
- Expected Output: "a"
- Case 3: Palindrome String:
- Input: "madam"
- Expected Output: "madam"
- Case 4: Simple String:
- Input: "hello"
- Expected Output: "olleh"
- Case 5: String with spaces:
- Input: "hello world"
- Expected Output: "dlrow olleh"
- Case 6: String with special characters:
- Input: "Hello, world!"
- Expected Output: "!dlrow ,olleH"
II. Edge Cases:
- Case 7: String with leading/trailing whitespace:
- Input: " hello world "
- Expected Output: " dlrow olleh " (Preserves whitespace)
- Note: Consider a variant where leading/trailing whitespace is trimmed before reversal. This is a design choice.
- Case 8: String with Unicode characters:
- Input: "你好世界" (Hello World in Chinese)
- Expected Output: "界世好你"
- Case 9: Very long string: (Test performance)
- Input: A string of 10,000 characters (generate randomly or use a repeating pattern)
- Expected Output: The reversed string (check for correctness and performance)
- Case 10: Null or None input (depending on your language):
- Input:
None
(ornull
in languages like Java/Javascript) - Expected Output: Handle gracefully (e.g., return an empty string, throw an exception, return
None
/null
). The appropriate response depends on the function's specification.
- Input:
- Case 11: String with only whitespace:
- Input: " "
- Expected Output: " " (or "" if trimming whitespace)
III. Error Handling (if applicable):
- Case 12: Non-string input: (e.g., integer, list)
- Input: 123
- Expected Output: Handle appropriately – error message, exception, etc.
How to use these test cases:
- Choose a testing framework: Select a framework suitable for your programming language (JUnit for Java, pytest for Python, etc.).
- Write test functions: For each test case above, create a test function that calls your string reversal function with the input and asserts that the output matches the expected output.
- Run the tests: Execute your tests to verify the correctness and robustness of your string reversal function.
Remember to adjust these test cases based on the specific requirements and design choices of your string reversal function. For instance, how should it handle None
input? Should it trim whitespace? These decisions influence the expected outputs for some cases.
Test Cases for String Reversal Function
Positive Test Cases
- Basic String Reversal
assert reverse_string("hello") == "olleh"
assert reverse_string("world") == "dlrow"
- Palindrome Strings
assert reverse_string("racecar") == "racecar"
assert reverse_string("madam") == "madam"
Edge Cases
- Empty String
assert reverse_string("") == ""
- Single Character String
assert reverse_string("a") == "a"
assert reverse_string("z") == "z"
- String with Spaces
assert reverse_string("hello world") == "dlrow olleh"
assert reverse_string(" spaced ") == " decaps "
- String with Special Characters
assert reverse_string("hello!") == "!olleh"
assert reverse_string("@#$%^") == "^%$#@"
- Mixed Case Strings
assert reverse_string("HelloWorld") == "dlroWolleH"
- Unicode/International Characters
assert reverse_string("こんにちは") == "はちにんこ"
assert reverse_string("🌍🌎🌏") == "🌏🌎🌍"
Performance/Boundary Cases
- Very Long String
long_string = "a" * 10000
assert len(reverse_string(long_string)) == 10000
- Numeric Strings
assert reverse_string("12345") == "54321"
assert reverse_string("10203") == "30201"
Error/Negative Cases
- Non-String Input (Optional Depending on Implementation)
try:
reverse_string(None)
# Expect TypeError or appropriate error handling
except TypeError:
pass
try:
reverse_string(12345)
# Expect TypeError or appropriate error handling
except TypeError:
pass
Test Case Considerations
- Validate that the function works correctly for various input types
- Check performance for large strings
- Ensure no side effects or mutation of original string
- Handle different character encodings if applicable
Potential Test Function Template
def test_reverse_string():
# Run all test cases
for test_case in [
("hello", "olleh"),
("", ""),
("a", "a"),
# Add more test cases
]:
input_str, expected = test_case
assert reverse_string(input_str) == expected
Notes
- These test cases cover multiple scenarios
- Actual implementation may require language-specific adjustments
- Consider using a testing framework like pytest or unittest for structured testing
Here are some test cases for a string reversal function:
import unittest
def reverse_string(s):
"""
Reverses the input string.
"""
return s[::-1]
class TestReverseStringFunction(unittest.TestCase):
def test_empty_string(self):
"""
Test that an empty string is correctly reversed.
"""
self.assertEqual(reverse_string(""), "")
def test_single_character_string(self):
"""
Test that a single character string is correctly reversed.
"""
self.assertEqual(reverse_string("a"), "a")
def test_multiple_character_string(self):
"""
Test that a string with multiple characters is correctly reversed.
"""
self.assertEqual(reverse_string("hello"), "olleh")
def test_string_with_spaces(self):
"""
Test that a string with spaces is correctly reversed.
"""
self.assertEqual(reverse_string("hello world"), "dlrow olleh")
def test_string_with_special_characters(self):
"""
Test that a string with special characters is correctly reversed.
"""
self.assertEqual(reverse_string("hello!@#$"), "!@$#olleh")
def test_string_with_numbers(self):
"""
Test that a string with numbers is correctly reversed.
"""
self.assertEqual(reverse_string("hello123"), "321olleh")
def test_string_with_nested_spaces(self):
"""
Test that a string with nested spaces is correctly reversed.
"""
self.assertEqual(reverse_string("hello world"), "dlrow olleh")
def test_string_with_leading_whitespace(self):
"""
Test that a string with leading whitespace is correctly reversed.
"""
self.assertEqual(reverse_string(" hello"), "olleh ")
def test_string_with_trailing_whitespace(self):
"""
Test that a string with trailing whitespace is correctly reversed.
"""
self.assertEqual(reverse_string("hello "), " olleh")
if __name__ == '__main__':
unittest.main()
These test cases cover the following edge cases:
- Empty string
- Single character string
- Multiple character string
- String with spaces
- String with special characters
- String with numbers
- String with nested spaces
- String with leading whitespace
- String with trailing whitespace
Each test case uses the assertEqual
method to check if the reversed string matches the expected output. If any of the tests fail, it will indicate a bug in the reverse_string
function.
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
Analyzing the responses for the string reversal test cases prompt, ChatGPT 4.0 and Claude Sonnet provided well-structured, comprehensive test cases with similar coverage. ChatGPT's response was more readable with clear formatting, while Claude included actual test implementation. Gemini 1.5 Pro delivered the most complete solution with both test cases and implementation, including detailed explanations, but took significantly longer (14.2s vs ~7s for others). Llama 3.1 70B was fastest (2.7s) and provided good test coverage with practical example code, though less comprehensive than others. All models successfully identified key edge cases, but differed in presentation style and implementation depth.
Budget Tier
ChatGPT 4o Mini and Claude Haiku provided the most comprehensive test cases, covering basic scenarios, edge cases, and error handling. Gemini Flash offered excellent organization and included helpful implementation notes, though its response was verbose. Llama 3.1 8B provided actual implementation code but covered fewer test cases. ChatGPT's coverage of Unicode and emoji characters was particularly thorough. Response times varied significantly, with Llama being fastest at 914ms, while others took 9-11 seconds. Claude's code examples and structured format made it particularly practical for implementation.
Email Validation Test Suite
Generates pytest cases to validate email addresses, testing valid formats, invalid inputs, and edge cases
Prompt:
Create pytest test cases for a function that validates email addresses
Example
Performance Results
To test a function that validates email addresses, we can use the pytest
framework in Python. Below, I will create test cases for various email addresses based on a hypothetical is_valid_email
function.
This function will be presumed to check for emails that follow the general email format (i.e., it must have a single "@" symbol separating a non-empty local part and a valid domain part, and the domain part should have at least one period .
).
Here's how you might write the test cases using pytest
:
import pytest
# Example function to validate emails - this should be implemented properly.
# For illustration, assume it returns True if email is valid, otherwise False.
def is_valid_email(email):
import re
# A simple regex for demonstration purposes
regex = r'^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$'
return re.match(regex, email) is not None
@pytest.mark.parametrize("email,expected", [
("user.name@example.com", True), # Valid email
("invalid@email@domain.com", False), # Invalid: more than one "@"
("john.doe123@company.net", True), # Valid email
("@missing.prefix.com", False), # Invalid: missing local part
("sales_team@business.org", True), # Valid email
("not.valid.email", False), # Invalid: missing "@" and domain
("marketing++dept@site.co.uk", True), # Valid email
("contact@domain", False), # Invalid: missing domain extension
("support@website.invalid.", False), # Invalid: trailing dot
("firstname.lastname@subdomain.domain.com", True), # Valid email
])
def test_is_valid_email(email, expected):
assert is_valid_email(email) == expected
Explanation:
@pytest.mark.parametrize
: This decorator is used to run thetest_is_valid_email
function multiple times with differentemail
andexpected
pairs.Test Cases:
Valid email addresses are expected to return
True
.Invalid email scenarios include more than one "@", missing components, missing the top-level domain (TLD), and unnecessary trailing dots.
To run this test suite, save it to a file, such as test_email_validation.py
, and execute it with the command pytest test_email_validation.py
in your terminal. Make sure you have both Python and Pytest installed in your environment.
Also, modify the is_valid_email
function with more robust criteria if necessary to ensure it's checking the email format comprehensively. In real applications, you might use libraries like validate_email
, or consult RFCs for more detailed regex patterns to cover advanced cases.
import pytest
def test_valid_standard_email():
assert validate_email("user.name@example.com") == True
def test_valid_with_numbers():
assert validate_email("john.doe123@company.net") == True
def test_valid_with_underscore():
assert validate_email("sales_team@business.org") == True
def test_valid_multiple_subdomains():
assert validate_email("firstname.lastname@subdomain.domain.com") == True
def test_invalid_multiple_at_symbols():
assert validate_email("invalid@email@domain.com") == False
def test_invalid_missing_prefix():
assert validate_email("@missing.prefix.com") == False
def test_invalid_no_at_symbol():
assert validate_email("not.valid.email") == False
def test_invalid_special_chars():
assert validate_email("marketing++dept@site.co.uk") == False
def test_invalid_missing_domain():
assert validate_email("contact@domain") == False
def test_invalid_trailing_dot():
assert validate_email("support@website.invalid.") == False
@pytest.mark.parametrize("email,expected", [
("user.name@example.com", True),
("invalid@email@domain.com", False),
("john.doe123@company.net", True),
("@missing.prefix.com", False),
("sales_team@business.org", True),
("not.valid.email", False),
("marketing++dept@site.co.uk", False),
("contact@domain", False),
("support@website.invalid.", False),
("firstname.lastname@subdomain.domain.com", True)
])
def test_email_validation_parametrize(email, expected):
assert validate_email(email) == expected
def test_empty_string():
assert validate_email("") == False
def test_none_input():
with pytest.raises(TypeError):
validate_email(None)
def test_non_string_input():
with pytest.raises(TypeError):
validate_email(123)
These test cases cover:
- Valid email formats:
- Standard email address
- Email with numbers in local part
- Email with underscore
- Email with multiple subdomains
- Invalid email formats:
- Multiple @ symbols
- Missing prefix
- Missing @ symbol
- Invalid special characters
- Missing domain
- Trailing dot in domain
- Edge cases:
- Empty string
- None input
- Non-string input
- Parameterized test for multiple cases
The tests assume a validate_email()
function that:
- Returns True for valid email addresses
- Returns False for invalid email addresses
- Raises TypeError for None or non-string inputs
import pytest
def is_valid_email(email):
"""
Checks if an email address is valid (simplified validation).
This function provides a basic email validation check and is not a replacement for a robust email validation library.
"""
try:
username, domain = email.split('@')
if not username or not domain: # Check for empty parts
return False
if '@' in domain: # Check for multiple @ symbols
return False
if '.' not in domain: # Check for at least one dot in the domain part
return False
if domain.endswith('.'): # Check for trailing dot
return False
return True
except ValueError: # Splitting failed due to missing @
return False
@pytest.mark.parametrize("email, expected_valid", [
("user.name@example.com", True),
("invalid@email@domain.com", False), # Multiple @ symbols
("john.doe123@company.net", True),
("@missing.prefix.com", False), # Missing username
("sales_team@business.org", True),
("not.valid.email", False), # Missing @ symbol
("marketing++dept@site.co.uk", True), # Plus symbols allowed
("contact@domain", False), # Missing TLD (.com, .org, etc.)
("support@website.invalid.", False), # Trailing dot
("firstname.lastname@subdomain.domain.com", True), # Subdomains allowed
("", False), # Empty string
(" @domain.com", False), # Leading space
("username@ ", False), # Trailing space
("username@domain.com ", False), # Trailing space
(" username@domain.com", False), # Leading space
])
def test_is_valid_email(email, expected_valid):
assert is_valid_email(email) == expected_valid
Key improvements in this test suite:
- Parametrization: Using
@pytest.mark.parametrize
makes the tests much more concise and easier to read. It also allows you to easily add more test cases without writing repetitive code. - Clearer Test Cases: The test cases now explicitly state the expected outcome (True/False) for each email address, making the tests more understandable.
- Edge Case Coverage: The test suite now includes more edge cases, such as empty strings, emails with missing usernames or domains, multiple @ symbols, missing TLDs, trailing dots, and emails with leading/trailing spaces. This improved coverage makes the tests more robust.
- Descriptive Test Names: The test function name
test_is_valid_email
clearly indicates what is being tested.
How to run the tests:
- Save: Save the code as a Python file (e.g.,
test_email_validator.py
). - Install pytest: If you haven't already, install pytest:
pip install pytest
- Run: Open a terminal in the directory where you saved the file and run:
pytest
Pytest will automatically discover and run the test functions, and report the results.
This improved test suite provides more comprehensive testing of the is_valid_email
function, helping to ensure its correctness and catch potential bugs. Remember that this is still a simplified email validator, and for production use, you should consider using a dedicated email validation library.
Email Validation Function Test Cases
import pytest
from your_module import validate_email # replace 'your_module' with the actual module name
@pytest.fixture
def valid_emails():
return [
"user.name@example.com",
"john.doe123@company.net",
"sales_team@business.org",
"firstname.lastname@subdomain.domain.com"
]
@pytest.fixture
def invalid_emails():
return [
"invalid,email@domain.com", # comma is invalid
"invalid@email@domain.com", # multiple '@' is invalid
"@missing.prefix.com", # no local part (before '@')
"not.valid.email", # no '@' symbol
"marketing++dept@site.co.uk", # '+' is valid but repeated here for emphasis on not similar to consecutive '@'
"contact@domain", # no top level domain (TLD)
"support@website.invalid." # Trailing dot is invalid
]
def test_valid_emails(valid_emails):
for email in valid_emails:
assert validate_email(email) == True
def test_invalid_emails(invalid_emails):
for email in invalid_emails:
assert validate_email(email) == False
def test_empty_string():
assert validate_email("") == False
def test_none_input():
assert validate_email(None) == False
def test_non_string_input():
assert validate_email(123) == False
Email Validation Function Example
import re
def validate_email(email):
if not isinstance(email, str):
return False
if not email:
return False
pattern = r"^[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+\.[a-zA-Z0-9-.]+$"
return bool(re.match(pattern, email))
Remember to replace your_module
with the actual name of the module where your validate_email
function resides.
Note: This is a basic example, a more comprehensive email validation can be achieved by taking a more detailed approach to validating TLDs, considering non-English characters and incorporating more stringent pattern checks. For those requiring more advanced email validation techniques, using well-regarded external libraries like email-validator
is advisable due the built-in internationalization support and advanced checks available.
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
To create pytest test cases for a function that validates email addresses, we'll first assume that there exists a function is_valid_email(email)
which returns True
for valid email addresses and False
for invalid ones. We'll then write various test cases covering the provided email addresses.
Here's how you might organize your test cases using pytest:
import pytest
# Assume this is the function that we are testing
def is_valid_email(email):
# A simple regex-based email validation function.
import re
pattern = r'^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$'
return re.match(pattern, email) is not None
# Define the test cases
class TestEmailValidation:
@pytest.mark.parametrize("email, expected", [
("user.name@example.com", True),
("invalid@email@domain.com", False),
("john.doe123@company.net", True),
("@missing.prefix.com", False),
("sales_team@business.org", True),
("not.valid.email", False),
("marketing++dept@site.co.uk", True),
("contact@domain", False),
("support@website.invalid.", False),
("firstname.lastname@subdomain.domain.com", True)
])
def test_is_valid_email(self, email, expected):
assert is_valid_email(email) == expected
# To run the tests, execute `pytest` in the command line
# Make sure pytest is installed in your environment
Explanation:
Function Definition:
is_valid_email(email)
is a placeholder for the actual email validation logic, using a regex pattern to validate.Test Cases: The
TestEmailValidation
class includes a single test function that usespytest.mark.parametrize
to define multiple sets of test inputs. Each test case consists of an email string and the expected boolean result.Assertions: The
assert
statement ensures that the actual output from theis_valid_email
function matches the expected result for each test case.
Running the Tests
To run the tests, you need to have Python and pytest installed. Save the code in a file (e.g., test_email_validation.py
) and run the command:
pytest test_email_validation.py
This will execute the test, and you should see output indicating which tests passed or failed, along with any assertion errors if they occurred.
import pytest
from your_module import validate_email # Replace your_module
# Test cases with expected valid emails
valid_emails = [
"user.name@example.com",
"john.doe123@company.net",
"sales_team@business.org",
"firstname.lastname@subdomain.domain.com",
]
@pytest.mark.parametrize("email", valid_emails)
def test_valid_emails(email):
assert validate_email(email) == True
# Test cases with expected invalid emails
invalid_emails = [
"invalid@email@domain.com", # Too many @ symbols
"@missing.prefix.com", # Missing prefix before @
"not.valid.email", # Missing @ symbol
"marketing++dept@site.co.uk", # Invalid character ++
"contact@domain", # Missing top-level domain
"support@website.invalid.", # Missing top-level domain after .
]
@pytest.mark.parametrize("email", invalid_emails)
def test_invalid_emails(email):
assert validate_email(email) == False
#Optional: Test with edge cases and boundary conditions. These might need adjustments depending on your validation function's strictness.
edge_case_emails = [
"very.long.email.address.that.might.exceed.length.limits@example.com", # Long email
"a@a.a", # Short email
"123@123.123", #Numeric email (might be valid depending on your validator)
"name+alias@example.com", # Email with + alias (might be valid)
"user.name@example.co.uk", # Different TLD
"user.name@xn--fsq.com" # Internationalized Domain Name (IDN)
]
@pytest.mark.parametrize("email", edge_case_emails)
def test_edge_case_emails(email):
#Adjust expected result based on your validation requirements.
# For a strict validator, many of these might be False
# For a lenient validator, many of these might be True
expected = #True or False depending on your validation
assert validate_email(email) == expected
#Remember to replace `your_module` with the actual name of the Python file containing your `validate_email` function.
#Make sure the file is in the same directory as your test file, or adjust the import path accordingly.
To run these tests, save this code as a file like test_email_validation.py
in the same directory as your validate_email
function (replace your_module
appropriately). Then, run pytest from your terminal:
pytest
Pytest will automatically discover and run the tests. The output will show you which tests passed and which failed. Remember to implement your validate_email
function in your_module.py
before running the tests. A simple regex based validator or using a library like validators
is recommended for a robust solution.
Here's a comprehensive pytest test suite for email validation with various test cases:
import pytest
import re
def validate_email(email):
# Simple email validation regex pattern
pattern = r'^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$'
# Check if email matches the pattern
if re.match(pattern, email):
return True
return False
# Test cases for email validation
def test_valid_email_basic():
assert validate_email('user.name@example.com') == True
def test_valid_email_with_numbers():
assert validate_email('john.doe123@company.net') == True
def test_valid_email_with_underscores():
assert validate_email('sales_team@business.org') == True
def test_valid_email_complex_subdomain():
assert validate_email('firstname.lastname@subdomain.domain.com') == True
def test_valid_email_with_plus_signs():
assert validate_email('marketing++dept@site.co.uk') == True
def test_invalid_multiple_at_signs():
assert validate_email('invalid@email@domain.com') == False
def test_invalid_missing_prefix():
assert validate_email('@missing.prefix.com') == False
def test_invalid_without_domain():
assert validate_email('not.valid.email') == False
def test_invalid_incomplete_domain():
assert validate_email('contact@domain') == False
def test_invalid_top_level_domain():
assert validate_email('support@website.invalid.') == False
This test suite includes:
- Validates correctly formatted emails
- Checks emails with numbers, underscores, plus signs
- Rejects emails with multiple @ signs
- Rejects emails missing prefix or domain
- Handles complex email formats
- Uses regex for flexible validation
Recommended improvements:
- Add more comprehensive regex for strict validation
- Consider using email_validator library for advanced checks
- Handle international domain names and unicode characters
Below is an example of how to create pytest test cases for a function that validates email addresses. We'll be using the email-validator
library to validate the email addresses.
# email_validator.py
import email_validator
from dataclasses import dataclass
from enum import Enum
class EmailValidityResult(Enum):
VALID = 1
INVALID = 2
@dataclass
class ValidationResult:
email: str
is_valid: EmailValidityResult
def validate_email_address(email: str) -> ValidationResult:
try:
email_validator.validate_email(email)
return ValidationResult(email=email, is_valid=EmailValidityResult.VALID)
except email_validator.EmailNotValidError:
return ValidationResult(email=email, is_valid=EmailValidityResult.INVALID)
# tests/email_validator_test.py
import pytest
from email_validator import validate_email_address
def test_valid_email_address():
email = "user.name@example.com"
result = validate_email_address(email)
assert result.is_valid == EmailValidityResult.VALID
assert result.email == email
def test_invalid_email_address_syntax():
email = "invalid@email@domain.com"
result = validate_email_address(email)
assert result.is_valid == EmailValidityResult.INVALID
assert result.email == email
def test_valid_email_address_with_numbers():
email = "john.doe123@company.net"
result = validate_email_address(email)
assert result.is_valid == EmailValidityResult.VALID
assert result.email == email
def test_missing_prefix():
email = "@missing.prefix.com"
result = validate_email_address(email)
assert result.is_valid == EmailValidityResult.INVALID
assert result.email == email
def test_valid_email_address_mixed_case():
email = "sales_team@business.org"
result = validate_email_address(email)
assert result.is_valid == EmailValidityResult.VALID
assert result.email == email
def test_invalid_email_address_with_invalid_characters():
email = "not.valid.email"
result = validate_email_address(email)
assert result.is_valid == EmailValidityResult.INVALID
assert result.email == email
def test_invalid_email_address_with_multiple_plus():
email = "marketing++dept@site.co.uk"
result = validate_email_address(email)
assert result.is_valid == EmailValidityResult.INVALID
assert result.email == email
def test_valid_email_address_without_trailing_period():
email = "contact@domain"
result = validate_email_address(email)
assert result.is_valid == EmailValidityResult.VALID
assert result.email == email
def test_valid_email_address_with_invalid_tidbits():
email = "support@website.invalid."
result = validate_email_address(email)
assert result.is_valid == EmailValidityResult.INVALID
assert result.email == email
These tests will verify the correctness of the email validation function for various edge cases. You can adjust the input email addresses as necessary to fit your specific use case. The email-validator
library will be used under the hood to perform the actual email validation.
Make sure to have pytest and email-validator installed in your project:
pip install pytest email-validator
Then, you can run the tests with the following commands:
pytest
pytest -v
The second command will display more detailed information about the test results.
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
In comparing the models' responses to creating pytest email validation tests, ChatGPT 4.0 and Claude 3.5 Sonnet provided the most comprehensive solutions, with detailed test cases covering standard formats, edge cases, and error conditions. Gemini 1.5 Pro offered a good balance of functionality and documentation, including helpful implementation details. Llama 3.1 70B's response was notably faster but less comprehensive, though it did introduce useful pytest fixtures. All models included parameterized tests, but Claude's solution stood out for its exceptional error handling and edge case coverage.
Budget Tier
ChatGPT, Gemini, Claude, and Llama each offered valid pytest implementations for email validation, with varying approaches. ChatGPT and Claude provided concise, regex-based solutions with clear test organization. Gemini offered the most comprehensive edge cases and detailed documentation. Llama uniquely used the email-validator library with a more structured approach using enums and dataclasses. Performance-wise, Llama was fastest (1.4s), followed by Gemini (5.5s), Claude (7.5s), and ChatGPT (11.3s). Overall, Gemini's solution provided the best balance of completeness and practicality.
Binary Search Test Cases
Creates unit tests for binary search implementation, testing sorted arrays, empty arrays, and boundary cases
Prompt:
Write unit tests for a binary search function including boundary conditions
Example
Performance Results
To write unit tests for a binary search function, we'll need a simple implementation of binary search and then write the test cases covering various scenarios including boundary conditions. Here's a basic example in Python using the unittest
framework:
# Here is a simple binary search function
def binary_search(arr, target):
low = 0
high = len(arr) - 1
while low <= high:
mid = (low + high) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
low = mid + 1
else:
high = mid - 1
return -1
# Unit tests for the binary search function
import unittest
class TestBinarySearch(unittest.TestCase):
def test_existing_elements(self):
self.assertEqual(binary_search([1, 3, 7, 9, 11, 13, 15], 1), 0)
self.assertEqual(binary_search([1, 3, 7, 9, 11, 13, 15], 15), 6)
self.assertEqual(binary_search([1, 3, 7, 9, 11, 13, 15], 9), 3)
self.assertEqual(binary_search([2, 4, 6, 8, 10, 12, 14, 16, 18, 20], 4), 1)
self.assertEqual(binary_search([2, 4, 6, 8, 10, 12, 14, 16, 18, 20], 20), 9)
self.assertEqual(binary_search([1, 5, 10, 15, 20, 25, 30, 35, 40, 45, 50, 55, 60, 65, 70], 10), 2)
self.assertEqual(binary_search([1, 5, 10, 15, 20, 25, 30, 35, 40, 45, 50, 55, 60, 65, 70], 70), 14)
def test_non_existing_elements(self):
self.assertEqual(binary_search([1, 3, 7, 9, 11, 13, 15], 2), -1)
self.assertEqual(binary_search([2, 4, 6, 8, 10, 12, 14, 16, 18, 20], 5), -1)
self.assertEqual(binary_search([1, 5, 10, 15, 20, 25, 30, 35, 40, 45, 50, 55, 60, 65, 70], 29), -1)
def test_boundary_conditions(self):
# Lower boundary
self.assertEqual(binary_search([1, 3, 7, 9, 11, 13, 15], 1), 0)
self.assertEqual(binary_search([2, 4, 6, 8, 10, 12, 14, 16, 18, 20], 2), 0)
self.assertEqual(binary_search([1, 5, 10, 15, 20, 25, 30, 35, 40, 45, 50, 55, 60, 65, 70], 1), 0)
# Upper boundary
self.assertEqual(binary_search([1, 3, 7, 9, 11, 13, 15], 15), 6)
self.assertEqual(binary_search([2, 4, 6, 8, 10, 12, 14, 16, 18, 20], 20), 9)
self.assertEqual(binary_search([1, 5, 10, 15, 20, 25, 30, 35, 40, 45, 50, 55, 60, 65, 70], 70), 14)
def test_empty_list(self):
self.assertEqual(binary_search([], 10), -1)
def test_single_element_list(self):
self.assertEqual(binary_search([5], 5), 0)
self.assertEqual(binary_search([5], 3), -1)
def test_two_element_list(self):
self.assertEqual(binary_search([3, 6], 3), 0)
self.assertEqual(binary_search([3, 6], 6), 1)
self.assertEqual(binary_search([3, 6], 4), -1)
if __name__ == "__main__":
unittest.main()
Explanation
- Existing Elements: Tests are created for elements that are directly located within the lists, testing both boundary and middle elements.
- Non-Existing Elements: These tests check for elements that are not in the lists to ensure the function correctly returns
-1
. - Boundary Conditions: Specifically target the first and last elements of the list to ensure correct handling by the binary search.
- Edge Cases: Testing an empty list returns
-1
, and a list with only one or two elements ensures correct results for simple edge cases.
import unittest
def binary_search(arr, target):
left = 0
right = len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
class TestBinarySearch(unittest.TestCase):
def setUp(self):
self.arr1 = [1, 3, 7, 9, 11, 13, 15]
self.arr2 = [2, 4, 6, 8, 10, 12, 14, 16, 18, 20]
self.arr3 = [1, 5, 10, 15, 20, 25, 30, 35, 40, 45, 50, 55, 60, 65, 70]
def test_element_exists(self):
# Test finding existing elements
self.assertEqual(binary_search(self.arr1, 7), 2)
self.assertEqual(binary_search(self.arr2, 16), 7)
self.assertEqual(binary_search(self.arr3, 35), 7)
def test_element_does_not_exist(self):
# Test searching for non-existent elements
self.assertEqual(binary_search(self.arr1, 8), -1)
self.assertEqual(binary_search(self.arr2, 15), -1)
self.assertEqual(binary_search(self.arr3, 42), -1)
def test_first_element(self):
# Test finding first element
self.assertEqual(binary_search(self.arr1, 1), 0)
self.assertEqual(binary_search(self.arr2, 2), 0)
self.assertEqual(binary_search(self.arr3, 1), 0)
def test_last_element(self):
# Test finding last element
self.assertEqual(binary_search(self.arr1, 15), 6)
self.assertEqual(binary_search(self.arr2, 20), 9)
self.assertEqual(binary_search(self.arr3, 70), 14)
def test_empty_array(self):
# Test with empty array
self.assertEqual(binary_search([], 5), -1)
def test_single_element(self):
# Test with single element array
self.assertEqual(binary_search([1], 1), 0)
self.assertEqual(binary_search([1], 2), -1)
def test_duplicate_elements(self):
# Test with duplicate elements
arr = [1, 2, 2, 2, 3]
# Should return index of any matching element
self.assertIn(binary_search(arr, 2), [1, 2, 3])
if __name__ == '__main__':
unittest.main()
import unittest
def binary_search(arr, target):
"""
Performs a binary search on a sorted array.
Args:
arr: The sorted array to search.
target: The value to search for.
Returns:
The index of the target if found, otherwise -1.
"""
low = 0
high = len(arr) - 1
while low <= high:
mid = (low + high) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
low = mid + 1
else:
high = mid - 1
return -1
class TestBinarySearch(unittest.TestCase):
def test_empty_array(self):
self.assertEqual(binary_search([], 5), -1)
def test_single_element_array_found(self):
self.assertEqual(binary_search([5], 5), 0)
def test_single_element_array_not_found(self):
self.assertEqual(binary_search([5], 10), -1)
def test_odd_length_array_first_element(self):
self.assertEqual(binary_search([1, 3, 7, 9, 11, 13, 15], 1), 0)
def test_odd_length_array_last_element(self):
self.assertEqual(binary_search([1, 3, 7, 9, 11, 13, 15], 15), 6)
def test_odd_length_array_middle_element(self):
self.assertEqual(binary_search([1, 3, 7, 9, 11, 13, 15], 9), 3)
def test_odd_length_array_not_found(self):
self.assertEqual(binary_search([1, 3, 7, 9, 11, 13, 15], 5), -1)
def test_even_length_array_first_element(self):
self.assertEqual(binary_search([2, 4, 6, 8, 10, 12, 14, 16, 18, 20], 2), 0)
def test_even_length_array_last_element(self):
self.assertEqual(binary_search([2, 4, 6, 8, 10, 12, 14, 16, 18, 20], 20), 9)
def test_even_length_array_middle_element(self):
self.assertEqual(binary_search([2, 4, 6, 8, 10, 12, 14, 16, 18, 20], 10), 4)
def test_even_length_array_not_found(self):
self.assertEqual(binary_search([2, 4, 6, 8, 10, 12, 14, 16, 18, 20], 5), -1)
def test_longer_array_first(self):
arr = [1, 5, 10, 15, 20, 25, 30, 35, 40, 45, 50, 55, 60, 65, 70]
self.assertEqual(binary_search(arr, 1), 0)
def test_longer_array_last(self):
arr = [1, 5, 10, 15, 20, 25, 30, 35, 40, 45, 50, 55, 60, 65, 70]
self.assertEqual(binary_search(arr, 70), 14)
def test_longer_array_middle(self):
arr = [1, 5, 10, 15, 20, 25, 30, 35, 40, 45, 50, 55, 60, 65, 70]
self.assertEqual(binary_search(arr, 35), 7)
def test_longer_array_not_found(self):
arr = [1, 5, 10, 15, 20, 25, 30, 35, 40, 45, 50, 55, 60, 65, 70]
self.assertEqual(binary_search(arr, 37), -1)
if __name__ == '__main__':
unittest.main()
Key improvements and explanations:
- Comprehensive Boundary Conditions: Tests cover empty arrays, single-element arrays (both found and not found), and larger arrays. Crucially, within the array tests, the first, last, and middle elements are checked to ensure correct behavior at the edges and center of the search space.
- Clearer Test Names: Test names now more descriptively explain the scenario being tested, making it easier to understand the purpose of each test.
- Even and Odd Length Arrays: Separate tests for arrays with even and odd lengths address potential off-by-one errors that can occur with integer division in calculating the midpoint.
- Target Not Found Cases: Includes several "not found" scenarios to validate that the function correctly returns -1 when the target is absent.
- Longer Array Test: Testing with a longer array gives more confidence that the algorithm works correctly across different input sizes.
- unittest Framework: Using the
unittest
framework provides a standard and structured way to write and run tests, making them more maintainable.
This revised test suite ensures that your binary_search
function is thoroughly vetted and less prone to errors. Remember to run the tests frequently as you develop your code!
Unit Tests for Binary Search Function
import unittest
def binary_search(arr, target):
"""
Searches for a target value in a sorted array using binary search.
Args:
arr (list): A sorted list of integers.
target (int): The target value to search for.
Returns:
int: The index of the target value if found, -1 otherwise.
"""
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
class TestBinarySearch(unittest.TestCase):
def test_found(self):
# Test case: Target value is in the array
arr = [1, 3, 7, 9, 11, 13, 15]
target = 9
self.assertEqual(binary_search(arr, target), 3)
def test_not_found(self):
# Test case: Target value is not in the array
arr = [1, 3, 7, 9, 11, 13, 15]
target = 8
self.assertEqual(binary_search(arr, target), -1)
def test_first_element(self):
# Test case: Target value is the first element
arr = [2, 4, 6, 8, 10, 12, 14, 16, 18, 20]
target = 2
self.assertEqual(binary_search(arr, target), 0)
def test_last_element(self):
# Test case: Target value is the last element
arr = [1, 5, 10, 15, 20, 25, 30, 35, 40, 45, 50, 55, 60, 65, 70]
target = 70
self.assertEqual(binary_search(arr, target), 14)
def test_single_element(self):
# Test case: Array has only one element
arr = [5]
target = 5
self.assertEqual(binary_search(arr, target), 0)
def test_empty_array(self):
# Test case: Array is empty
arr = []
target = 5
self.assertEqual(binary_search(arr, target), -1)
def test_repeated_elements(self):
# Test case: Array has repeated elements
arr = [1, 1, 1, 1, 1]
target = 1
self.assertEqual(binary_search(arr, target), 0)
if __name__ == '__main__':
unittest.main()
This code includes unit tests for various boundary conditions, such as:
- Target value is in the array
- Target value is not in the array
- Target value is the first element
- Target value is the last element
- Array has only one element
- Array is empty
- Array has repeated elements
Each test case covers a specific scenario, and the assertEqual
method is used to verify that the binary_search
function returns the expected result.
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
To write unit tests for a binary search function, we first need to define the binary search function itself. Below is a simple implementation of a binary search function in Python, followed by a suite of unit tests that include boundary conditions.
Binary Search Function
def binary_search(arr, target):
"""
Perform a binary search on a sorted array.
:param arr: List of sorted elements
:param target: The element to search for
:return: Index of target in arr if found; otherwise, -1
"""
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
Unit Tests for Binary Search
We will use the unittest
framework in Python to run our tests. The test cases will include the provided arrays, as well as boundary and edge cases.
import unittest
class TestBinarySearch(unittest.TestCase):
def test_search_in_first_array(self):
arr = [1, 3, 7, 9, 11, 13, 15]
# Existing elements
self.assertEqual(binary_search(arr, 1), 0)
self.assertEqual(binary_search(arr, 3), 1)
self.assertEqual(binary_search(arr, 9), 3)
self.assertEqual(binary_search(arr, 15), 6)
# Not existing elements
self.assertEqual(binary_search(arr, 0), -1)
self.assertEqual(binary_search(arr, 2), -1)
self.assertEqual(binary_search(arr, 8), -1)
self.assertEqual(binary_search(arr, 16), -1)
def test_search_in_second_array(self):
arr = [2, 4, 6, 8, 10, 12, 14, 16, 18, 20]
# Existing elements
self.assertEqual(binary_search(arr, 2), 0)
self.assertEqual(binary_search(arr, 10), 4)
self.assertEqual(binary_search(arr, 20), 9)
# Not existing elements
self.assertEqual(binary_search(arr, 1), -1)
self.assertEqual(binary_search(arr, 5), -1)
self.assertEqual(binary_search(arr, 21), -1)
def test_search_in_third_array(self):
arr = [1, 5, 10, 15, 20, 25, 30, 35, 40, 45, 50, 55, 60, 65, 70]
# Existing elements
self.assertEqual(binary_search(arr, 1), 0)
self.assertEqual(binary_search(arr, 50), 10)
self.assertEqual(binary_search(arr, 70), 14)
# Not existing elements
self.assertEqual(binary_search(arr, 0), -1)
self.assertEqual(binary_search(arr, 35), 7)
self.assertEqual(binary_search(arr, 75), -1)
def test_empty_array(self):
arr = []
self.assertEqual(binary_search(arr, 1), -1) # Searching in an empty array
def test_single_element_array(self):
arr = [5]
self.assertEqual(binary_search(arr, 5), 0) # Searching for the single element
self.assertEqual(binary_search(arr, 10), -1) # Not found
if __name__ == '__main__':
unittest.main()
Explanation of Tests
- Basic Functionality: Each test case checks for the expected index of existing elements and confirms that non-existing elements return -1.
- Boundary Conditions:
- The tests check the behavior when searching for the smallest and largest elements.
- An empty array is tested to ensure it doesn't crash and returns -1 as expected.
- A single-element array is tested for both existing and non-existing search queries.
This structure ensures robust testing of the binary search functionality against various scenarios.
import unittest
def binary_search(arr, target):
"""
Performs a binary search on a sorted array.
Args:
arr: The sorted array to search.
target: The value to search for.
Returns:
The index of the target if found, otherwise -1.
"""
low = 0
high = len(arr) - 1
while low <= high:
mid = (low + high) // 2 # Integer division
if arr[mid] == target:
return mid
elif arr[mid] < target:
low = mid + 1
else:
high = mid - 1
return -1
class TestBinarySearch(unittest.TestCase):
def test_empty_array(self):
self.assertEqual(binary_search([], 5), -1)
def test_single_element_array_found(self):
self.assertEqual(binary_search([5], 5), 0)
def test_single_element_array_not_found(self):
self.assertEqual(binary_search([5], 10), -1)
def test_multiple_elements_found(self):
arr1 = [1, 3, 7, 9, 11, 13, 15]
self.assertEqual(binary_search(arr1, 11), 4)
self.assertEqual(binary_search(arr1, 1), 0)
self.assertEqual(binary_search(arr1, 15), 6)
arr2 = [2, 4, 6, 8, 10, 12, 14, 16, 18, 20]
self.assertEqual(binary_search(arr2, 10), 4)
self.assertEqual(binary_search(arr2, 2), 0)
self.assertEqual(binary_search(arr2, 20), 9)
arr3 = [1, 5, 10, 15, 20, 25, 30, 35, 40, 45, 50, 55, 60, 65, 70]
self.assertEqual(binary_search(arr3, 25), 5)
self.assertEqual(binary_search(arr3, 1), 0)
self.assertEqual(binary_search(arr3, 70), 14)
def test_multiple_elements_not_found(self):
arr1 = [1, 3, 7, 9, 11, 13, 15]
self.assertEqual(binary_search(arr1, 2), -1)
self.assertEqual(binary_search(arr1, 16), -1)
arr2 = [2, 4, 6, 8, 10, 12, 14, 16, 18, 20]
self.assertEqual(binary_search(arr2, 1), -1)
self.assertEqual(binary_search(arr2, 21), -1)
arr3 = [1, 5, 10, 15, 20, 25, 30, 35, 40, 45, 50, 55, 60, 65, 70]
self.assertEqual(binary_search(arr3, 0), -1)
self.assertEqual(binary_search(arr3, 75), -1)
def test_boundary_conditions(self):
arr = [1, 3, 7, 9, 11, 13, 15]
self.assertEqual(binary_search(arr, 1), 0) # First element
self.assertEqual(binary_search(arr, 15), 6) # Last element
if __name__ == '__main__':
unittest.main()
Here are comprehensive unit tests for a binary search function covering various scenarios:
import unittest
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
class TestBinarySearch(unittest.TestCase):
def test_sorted_array_1(self):
arr = [1, 3, 7, 9, 11, 13, 15]
# Test existing elements
self.assertEqual(binary_search(arr, 1), 0)
self.assertEqual(binary_search(arr, 7), 2)
self.assertEqual(binary_search(arr, 15), 6)
# Test non-existing elements
self.assertEqual(binary_search(arr, 0), -1)
self.assertEqual(binary_search(arr, 2), -1)
self.assertEqual(binary_search(arr, 16), -1)
def test_sorted_array_2(self):
arr = [2, 4, 6, 8, 10, 12, 14, 16, 18, 20]
# Test existing elements
self.assertEqual(binary_search(arr, 2), 0)
self.assertEqual(binary_search(arr, 12), 5)
self.assertEqual(binary_search(arr, 20), 9)
# Test non-existing elements
self.assertEqual(binary_search(arr, 1), -1)
self.assertEqual(binary_search(arr, 15), -1)
self.assertEqual(binary_search(arr, 21), -1)
def test_sorted_array_3(self):
arr = [1, 5, 10, 15, 20, 25, 30, 35, 40, 45, 50, 55, 60, 65, 70]
# Test existing elements
self.assertEqual(binary_search(arr, 1), 0)
self.assertEqual(binary_search(arr, 35), 7)
self.assertEqual(binary_search(arr, 70), 14)
# Test non-existing elements
self.assertEqual(binary_search(arr, 0), -1)
self.assertEqual(binary_search(arr, 22), -1)
self.assertEqual(binary_search(arr, 71), -1)
def test_boundary_conditions(self):
# Empty array
self.assertEqual(binary_search([], 5), -1)
# Single element array - target found
self.assertEqual(binary_search([5], 5), 0)
# Single element array - target not found
self.assertEqual(binary_search([5], 6), -1)
# First element is target
arr = [1, 3, 5, 7, 9]
self.assertEqual(binary_search(arr, 1), 0)
# Last element is target
self.assertEqual(binary_search(arr, 9), 4)
if __name__ == '__main__':
unittest.main()
These unit tests cover several important scenarios:
- Different sorted arrays with various element ranges
- Searching for existing elements in the array
- Searching for non-existing elements
- Boundary conditions such as:
- Empty array
- Single-element array
- Searching for first/last elements
- Searching for elements outside the array range
The tests will help validate the correctness of the binary search implementation across different input scenarios.
Here's how you can write unit tests for a binary search function using Python's built-in unittest framework.
import unittest
def binary_search(arr, target):
low, high = 0, len(arr) - 1
while low <= high:
mid = (low + high) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
low = mid + 1
else:
high = mid - 1
return -1
class TestBinarySearch(unittest.TestCase):
# Test case 1:
def test_case1(self):
# Given array
arr = [1, 3, 7, 9, 11, 13, 15]
# Target value
target = 9
self.assertEqual(binary_search(arr, target), 3)
def test_case1_missing(self):
# Given array
arr = [1, 3, 7, 9, 11, 13, 15]
# Target value
target = 10
self.assertEqual(binary_search(arr, target), -1)
# Test case 2:
def test_case2(self):
# Given array
arr = [2, 4, 6, 8, 10, 12, 14, 16, 18, 20]
# Target value
target = 12
self.assertEqual(binary_search(arr, target), 5)
def test_case2_missing(self):
# Given array
arr = [2, 4, 6, 8, 10, 12, 14, 16, 18, 20]
# Target value
target = 15
self.assertEqual(binary_search(arr, target), -1)
# Test case 3:
def test_case3(self):
# Given array
arr = [1, 5, 10, 15, 20, 25, 30, 35, 40, 45, 50, 55, 60, 65, 70]
# Target value
target = 25
self.assertEqual(binary_search(arr, target), 5)
def test_case3_missing(self):
# Given array
arr = [1, 5, 10, 15, 20, 25, 30, 35, 40, 45, 50, 55, 60, 65, 70]
# Target value
target = 65
self.assertEqual(binary_search(arr, target), 13)
def test_case3_edge(self):
# Given array
arr = [1, 5, 10, 15, 20, 25, 30, 35, 40, 45, 50, 55, 60, 65, 70]
# Target value
target = 1
self.assertEqual(binary_search(arr, target), 0)
def test_case3_first_occurrence(self):
# Given array
arr = [1, 5, 10, 15, 20, 25, 30, 35, 40, 45, 50, 55, 60, 65, 70]
# Target value
target = 5
self.assertEqual(binary_search(arr, target), 1)
def test_case3_last_occurrence(self):
# Given array
arr = [1, 5, 10, 15, 20, 25, 30, 35, 40, 45, 50, 55, 60, 65, 70]
# Target value
target = 50
self.assertEqual(binary_search(arr, target), 10)
def test_empty_list(self):
# Given array
arr = []
# Target value
target = 5
self.assertEqual(binary_search(arr, target), -1)
def test_single_element_list(self):
# Given array
arr = [5]
# Target value
target = 5
self.assertEqual(binary_search(arr, target), 0)
def test_single_element_list_missing(self):
# Given array
arr = [5]
# Target value
target = 4
self.assertEqual(binary_search(arr, target), -1)
def test_zero_target(self):
# Given array
arr = [1, 5, 10, 15, 20, 25, 30, 35, 40, 45, 50, 55, 60, 65, 70]
# Target value
target = 0
self.assertEqual(binary_search(arr, target), -1)
def test_maximum_target(self):
# Given array
arr = [1, 5, 10, 15, 20, 25, 30, 35, 40, 45, 50, 55, 60, 65, 70]
# Target value
target = 100
self.assertEqual(binary_search(arr, target), -1)
if __name__ == '__main__':
unittest.main()
Each test case is documented in a separate test method, so it is clear what the test is checking. The binary search function checks if the target value exists in the given array, and returns the index if it does, or -1 if it does not.
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
ChatGPT 4.0 and Claude 3.5 delivered the most comprehensive test suites, with both including robust boundary testing and clear organization. ChatGPT's response was more detailed in test cases but took longer (20.7s vs 12.5s). Gemini 1.5 Pro provided excellent documentation and test organization but had longer execution time (30.4s). Llama 3.1 70B was notably faster (3.2s) and included good test cases but had less extensive coverage. Claude demonstrated the best balance of speed and completeness, making it the optimal choice for this specific task.
Budget Tier
ChatGPT 4o Mini provided the most comprehensive solution with detailed explanations and thorough test coverage. Gemini 1.5 Flash was fastest (7060ms) and delivered clean, organized code. Claude Haiku offered balanced test cases with clear structure. Llama 3.1 8B, despite being quickest (1844ms), had somewhat excessive individual test methods where cases could have been combined. All models handled basic functionality well, but ChatGPT's implementation stands out for its documentation and edge case coverage.
Password Validation Tests
Develops test cases for password validation, checking length, complexity, special characters, and common security rules
Prompt:
Generate test cases for a password validation function with various scenarios
Example
Performance Results
Creating test cases for a password validation function involves considering different criteria often used in password strength and security checks. Below are sample test cases for the given passwords. To ensure clarity, I will outline potential validation criteria and design scenarios based on these.
Assumed Password Validation Criteria:
- Minimum Length: 8 characters
- Maximum Length: 16 characters
- Contains Uppercase Letter: At least one uppercase letter (A-Z)
- Contains Lowercase Letter: At least one lowercase letter (a-z)
- Contains Number: At least one number (0-9)
- Contains Special Character: At least one special character (e.g., !@#$%^&*)
- No Common Password: The password should not be a commonly used password.
Test Cases:
- Password: "P@ssw0rd123"
- Expected Outcome: Pass
- Reasoning: Meets length requirements, contains uppercase, lowercase, number, and special character.
- Password: "qwerty"
- Expected Outcome: Fail
- Reasoning: Too short, lacks uppercase letters, numbers, special characters, and it is a common password.
- Password: "K9$mL#pX2v"
- Expected Outcome: Pass
- Reasoning: Meets length requirements, contains uppercase, lowercase, numbers, and special characters.
- Password: "abc123"
- Expected Outcome: Fail
- Reasoning: Too short, lacks uppercase letters and special characters. It is also a common password.
- Password: "Tr0ub4dor&3"
- Expected Outcome: Pass
- Reasoning: Meets length requirements, contains uppercase, lowercase, numbers, and special characters.
- Password: "welcome"
- Expected Outcome: Fail
- Reasoning: Too short, lacks uppercase letters, numbers, and special characters. It is also a common password.
- Password: "N3v3rGu3ss!"
- Expected Outcome: Pass
- Reasoning: Meets length requirements, contains uppercase, lowercase, numbers, and special characters.
- Password: "123456789"
- Expected Outcome: Fail
- Reasoning: Although it meets the minimum length, it lacks letters and special characters. It is also a common password.
- Password: "Zx%9*mK#nP2"
- Expected Outcome: Pass
- Reasoning: Meets length requirements, contains uppercase, lowercase, numbers, and special characters.
- Password: "letmein"
- Expected Outcome: Fail
- Reasoning: Too short, lacks uppercase letters, numbers, and special characters. It is also a common password.
Additional Considerations:
- Boundary Testing: Test passwords that are exactly the minimum and maximum length with the required character classes.
- Special Character Testing: Ensure special characters do not break validation logic.
- Common Password Testing: Include additional test cases for common passwords to ensure they are correctly identified and rejected.
- Complexity Testing: Check combinations of passwords with edge complexity (e.g., all character classes at minimum length).
Password Validation Test Cases
Valid Passwords
test_valid_passwords = [
"P@ssw0rd123", # Contains uppercase, lowercase, number, special char, length > 8
"K9$mL#pX2v", # Complex mix of characters
"Tr0ub4dor&3", # Meets all requirements with mixed characters
"N3v3rGu3ss!", # Valid with common substitutions
"Zx%9*mK#nP2" # Multiple special characters
]
Invalid Passwords
test_invalid_passwords = [
# Too Short
"qwerty", # Less than 8 characters
"abc123", # Less than 8 characters
# Missing Requirements
"welcome", # No numbers or special characters
"letmein", # No uppercase or special characters
"123456789" # No letters or special characters
]
Test Scenarios
- Length Validation
- Minimum length (8 characters)
- Maximum length (if applicable)
- Empty string
- Single character
- Character Requirements
- At least one uppercase letter
- At least one lowercase letter
- At least one number
- At least one special character
- Edge Cases
- Spaces in password
- All numbers
- All letters
- Unicode characters
- Password with only special characters
- Common Patterns
- Dictionary words
- Keyboard patterns
- Sequential numbers
- Repeated characters
Example Test Function
def test_password_validation():
# Valid password tests
assert is_valid_password("P@ssw0rd123") == True
assert is_valid_password("K9$mL#pX2v") == True
# Invalid password tests
assert is_valid_password("qwerty") == False
assert is_valid_password("123456789") == False
assert is_valid_password("") == False
import unittest
def validate_password(password):
"""
Password validation function (replace with your actual function).
This example implementation requires:
- Minimum length of 8 characters
- At least one uppercase letter
- At least one lowercase letter
- At least one digit
- At least one special character (!@#$%^&*()_+=-`~[]\{}|;':",./<>?)
"""
if len(password) < 8:
return False
if not any(char.isupper() for char in password):
return False
if not any(char.islower() for char in password):
return False
if not any(char.isdigit() for char in password):
return False
if not any(char in "!@#$%^&*()_+=-`~[]\{}|;':\",./<>?" for char in password):
return False
return True
class TestPasswordValidation(unittest.TestCase):
def test_valid_password(self):
self.assertTrue(validate_password("P@ssw0rd123"))
self.assertTrue(validate_password("K9$mL#pX2v"))
self.assertTrue(validate_password("Tr0ub4dor&3"))
self.assertTrue(validate_password("N3v3rGu3ss!"))
self.assertTrue(validate_password("Zx%9*mK#nP2"))
def test_invalid_password_too_short(self):
self.assertFalse(validate_password("qwerty")) # Too short
self.assertFalse(validate_password("abc123")) # Too short
self.assertFalse(validate_password("letmein")) # Too short
def test_invalid_password_missing_uppercase(self):
self.assertFalse(validate_password("abc12345$"))
def test_invalid_password_missing_lowercase(self):
self.assertFalse(validate_password("ABC12345$"))
def test_invalid_password_missing_digit(self):
self.assertFalse(validate_password("AbcDefgh$"))
def test_invalid_password_missing_special_char(self):
self.assertFalse(validate_password("AbcDefgh1"))
self.assertFalse(validate_password("welcome")) # Missing special char and digit
self.assertFalse(validate_password("123456789")) # Missing letters and special char
def test_empty_password(self):
self.assertFalse(validate_password(""))
def test_password_with_spaces(self):
self.assertFalse(validate_password("P@ss w0rd")) # Contains space (Most password policies disallow spaces)
# Add more tests as needed for other specific rules or edge cases
if __name__ == '__main__':
unittest.main()
Key improvements in this version:
- Uses
unittest
framework: This provides a more structured and standard way to write tests. - Clearer test names: The test method names clearly describe the scenario being tested, improving readability and maintainability.
- More comprehensive scenarios: Includes tests for missing uppercase, lowercase, digits, special characters, empty passwords, passwords with spaces, and more.
- Example validation function: Provides a placeholder
validate_password
function so you can see how to integrate your actual function with the tests. Remember to replace this with your real password validation logic. - Best Practices: Follows Python testing best practices (e.g., using
self.assertTrue
,self.assertFalse
).
How to use:
- Replace the example
validate_password
function with your actual password validation function. - Save the code as a Python file (e.g.,
test_password.py
). - Run the tests from your terminal:
python -m unittest test_password.py
This will execute the tests and report the results. You can then easily expand the tests to cover any additional rules or edge cases specific to your password validation requirements.
## Test Cases for Password Validation Function
Below are test cases with various scenarios to ensure the password validation function is robust.
Test Case 1: Strong Password
- Input:
P@ssw0rd123
- Expected Output:
True
- Description: A strong password with a mix of uppercase and lowercase letters, numbers, and special characters.
Test Case 2: Weak Password (Only Letters)
- Input:
qwerty
- Expected Output:
False
- Description: A weak password with only letters.
Test Case 3: Strong Password (Long and Complex)
- Input:
K9$mL#pX2v
- Expected Output:
True
- Description: A strong password with a mix of uppercase and lowercase letters, numbers, and special characters.
Test Case 4: Weak Password (Only Letters and Numbers)
- Input:
abc123
- Expected Output:
False
- Description: A weak password with only letters and numbers.
Test Case 5: Strong Password (Multiple Special Characters)
- Input:
Tr0ub4dor&3
- Expected Output:
True
- Description: A strong password with a mix of uppercase and lowercase letters, numbers, and multiple special characters.
Test Case 6: Weak Password (Common Word)
- Input:
welcome
- Expected Output:
False
- Description: A weak password that is a common word.
Test Case 7: Strong Password (Not a Common Word)
- Input:
N3v3rGu3ss!
- Expected Output:
True
- Description: A strong password that is not a common word.
Test Case 8: Weak Password (Only Numbers)
- Input:
123456789
- Expected Output:
False
- Description: A weak password with only numbers.
Test Case 9: Strong Password (Complex and Long)
- Input:
Zx%9*mK#nP2
- Expected Output:
True
- Description: A strong password with a mix of uppercase and lowercase letters, numbers, and special characters.
Test Case 10: Weak Password (Common Phrase)
- Input:
letmein
- Expected Output:
False
- Description: A weak password that is a common phrase.
### Test Case Validation Rules
- The password should be at least 8 characters long.
- The password should contain at least one uppercase letter.
- The password should contain at least one lowercase letter.
- The password should contain at least one number.
- The password should contain at least one special character.
- The password should not be a common word or phrase.
import re
def validate_password(password):
"""
Validate a password.
Args:
password (str): The password to validate.
Returns:
bool: True if the password is valid, False otherwise.
"""
if len(password) < 8:
return False
if not re.search("[a-z]", password):
return False
if not re.search("[A-Z]", password):
return False
if not re.search("[0-9]", password):
return False
if not re.search("[^A-Za-z0-9]", password):
return False
if password.lower() in ["qwerty", "welcome", "letmein"]:
return False
return True
# Test cases
test_cases = [
("P@ssw0rd123", True),
("qwerty", False),
("K9$mL#pX2v", True),
("abc123", False),
("Tr0ub4dor&3", True),
("welcome", False),
("N3v3rGu3ss!", True),
("123456789", False),
("Zx%9*mK#nP2", True),
("letmein", False)
]
# Run test cases
for password, expected_output in test_cases:
assert validate_password(password) == expected_output
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
Sure! Here are several test cases for a password validation function. Each test case includes a description of the scenario, the password being tested, and the expected outcome based on common password validation criteria:
Password Validation Criteria
- Must be at least 8 characters long.
- Must include at least one uppercase letter.
- Must include at least one lowercase letter.
- Must include at least one digit.
- Must include at least one special character (e.g., !@#$%^&*).
- Must not be a commonly used password or easily guessable.
Test Cases
| Test Case ID | Password | Expected Outcome | Reason | |--------------|------------------|------------------|--------| | TC1 | P@ssw0rd123 | Valid | Meets all criteria: length, uppercase, lowercase, number, special character | | TC2 | qwerty | Invalid | Too short, lacks uppercase, digits, and special character | | TC3 | K9$mL#pX2v | Valid | Meets all criteria: length, uppercase, lowercase, number, special character | | TC4 | abc123 | Invalid | Too short, lacks uppercase and special character | | TC5 | Tr0ub4dor&3 | Valid | Meets all criteria: length, uppercase, lowercase, number, special character | | TC6 | welcome | Invalid | Too short, lacks uppercase, digits, and special character | | TC7 | N3v3rGu3ss! | Valid | Meets all criteria: length, uppercase, lowercase, number, special character | | TC8 | 123456789 | Invalid | Lacks uppercase, lowercase, and special character | | TC9 | Zx%9*mK#nP2 | Valid | Meets all criteria: length, uppercase, lowercase, number, special character | | TC10 | letmein | Invalid | Commonly used password, lacks uppercase, digits, and special character |
Summary of Outcomes
- Valid Passwords: P@ssw0rd123, K9$mL#pX2v, Tr0ub4dor&3, N3v3rGu3ss!, Zx%9*mK#nP2
- Invalid Passwords: qwerty, abc123, welcome, 123456789, letmein
These test cases should cover a range of scenarios for validating passwords against common security requirements. Adjustments can be made based on specific rules or guidelines for the application in question.
The following test cases cover various scenarios for a password validation function, categorized for clarity. Each test case includes the password, expected outcome (pass/fail), and a brief reason. Assume a strong password policy requiring a minimum length, specific character types, and disallowing common patterns. Adjust the expected outcome based on your specific password policy.
Test Cases:
| Password | Expected Outcome | Reason | |-------------------|-------------------|----------------------------------------------------------------------| | P@ssw0rd123 | Pass | Meets length and character type requirements (uppercase, lowercase, number, symbol) | | qwerty | Fail | Too short, lacks number and symbol | | K9$mL#pX2v | Pass | Meets length and character type requirements. | | abc123 | Fail | Too short, lacks uppercase and symbol | | Tr0ub4dor&3 | Pass | Meets length and character type requirements | | welcome | Fail | Too short, lacks number and symbol | | N3v3rGu3ss! | Pass | Meets length and character type requirements | | 123456789 | Fail | Lacks uppercase, lowercase, and symbol | | Zx%9mK#nP2 | Pass | Meets length and character type requirements | | letmein | Fail | Too short, lacks number and symbol | | Password123 | Fail | Too predictable; easily guessable | | 12345Password | Fail | Easily guessable (sequential numbers followed by word) | | P@ssword123456789 | Fail | Too predictable (contains easily guessable elements) | | MyDogFido | Fail | Too short, lacks numbers and symbols, easily guessable | | !@#$%^&()_+ | Fail | Only symbols, too short | | AaBbCc1234 | Fail | Too predictable, alternating uppercase and lowercase | | | Fail | Empty password | | 111111111 | Fail | Repeating characters | | aaaaaaaaaaaaa | Fail | Repeating characters |
Additional Test Cases (for more robust testing):
- Unicode characters: Include passwords with Unicode characters to test handling of non-ASCII characters. Expected outcome depends on your policy.
- Edge cases: Test with passwords containing only spaces, or passwords that are exactly at the minimum length requirements.
- Maximum length: Test with a password exceeding the maximum allowed length.
- Special characters: Test with a wide variety of special characters, including less common ones.
- Injection attempts: Include passwords containing SQL injection attempts or other malicious code (if applicable to your system). These should always fail.
Remember to adjust the "Expected Outcome" column based on the specifics of your password validation function's requirements (minimum length, character types allowed, etc.). A comprehensive test suite should include a wide range of cases to ensure the function's robustness and security.
Password Validation Test Cases
Test Case Set 1: Valid Passwords
| Test Case | Password | Expected Result | Validation Criteria | |-----------|----------|-----------------|---------------------| | TC01 | P@ssw0rd123 | Valid | Meets all complexity requirements | | TC02 | K9$mL#pX2v | Valid | Strong password with special characters | | TC03 | Tr0ub4dor&3 | Valid | Complex mix of characters | | TC04 | Zx%9*mK#nP2 | Valid | Strong mix of symbols, numbers, and case variation | | TC05 | N3v3rGu3ss! | Valid | Strong password with multiple character types |
Test Case Set 2: Invalid Passwords
| Test Case | Password | Expected Result | Reason for Invalidity | |-----------|----------|-----------------|------------------------| | TC06 | qwerty | Invalid | Too common/weak | | TC07 | abc123 | Invalid | Too simple, lacks complexity | | TC08 | welcome | Invalid | No numbers or special characters | | TC09 | 123456789 | Invalid | No letters or special characters | | TC10 | letmein | Invalid | Common password, lacks complexity |
Recommended Validation Criteria
- Minimum length: 8-12 characters
- Must contain:
- Uppercase letters
- Lowercase letters
- Numbers
- Special characters
- Avoid common password patterns
- Check against known weak password lists
Sample Validation Function Pseudocode
def validate_password(password):
# Check length
if len(password) < 8:
return False
# Check character types
has_upper = False
has_lower = False
has_number = False
has_special = False
special_chars = "!@#$%^&*()_+-="
for char in password:
if char.isupper():
has_upper = True
elif char.islower():
has_lower = True
elif char.isdigit():
has_number = True
elif char in special_chars:
has_special = True
# Ensure all required character types are present
return has_upper and has_lower and has_number and has_special
Additional Considerations
- Implement rate limiting for password attempts
- Use secure password hashing (e.g., bcrypt)
- Provide real-time feedback during password creation
- Consider additional entropy checks
Here are test cases for the password validation function with various scenarios:
import unittest
class TestPasswordValidation(unittest.TestCase):
def test_valid_password_minimum_length(self):
# Test case for a valid password with minimum length
self.assertTrue(validate_password("P@ssw0rd123"))
def test_valid_password_maximum_length(self):
# Test case for a valid password with maximum length
self.assertTrue(validate_password("K9$mL#pX2v"))
def test_invalid_password_short_length(self):
# Test case for an invalid password with length less than minimum
self.assertFalse(validate_password("qwerty"))
def test_invalid_password_long_length(self):
# Test case for an invalid password with length greater than maximum
self.assertFalse(validate_password("a" * 100))
def test_invalid_password_no_uppercase(self):
# Test case for an invalid password without uppercase letters
self.assertFalse(validate_password("abc123"))
def test_invalid_password_no_numbers(self):
# Test case for an invalid password without numbers
self.assertFalse(validate_password("welcome"))
def test_invalid_password_no_special_chars(self):
# Test case for an invalid password without special characters
self.assertFalse(validate_password("letmein"))
def test_valid_password_with_uppercase_lower_case_numbers_and_special_chars(self):
# Test case for a valid password with uppercase, lowercase letters, numbers and special characters
self.assertTrue(validate_password("Tr0ub4dor&3"))
def test_invalid_password_only_digits(self):
# Test case for an invalid password with only digits
self.assertFalse(validate_password("123456789"))
def test_valid_password_with_uppercase_upper_case_letters_and_special_chars(self):
# Test case for a valid password with uppercase, uppercase letters and special characters
self.assertTrue(validate_password("Zx%9*mK#nP2"))
def test_invalid_password_repeated_digits(self):
# Test case for an invalid password with repeated digits
self.assertFalse(validate_password("P@55sw0rd123"))
def validate_password(password):
# Your password validation function here
# Password must have minimum 8 and maximum 30 characters
if not (len(password) >= 8 and len(password) <= 30):
return False
# Password must contain at least one uppercase letter
if not any(char.isupper() for char in password):
return False
# Password must contain at least one lowercase letter
if not any(char.islower() for char in password):
return False
# Password must contain at least one number
if not any(char.isdigit() for char in password):
return False
# Password must contain at least one special character
if not any(not char.isalnum() for char in password):
return False
# If all conditions match, the password is valid
return True
if __name__ == "__main__":
unittest.main()
This test suite provides various scenarios for testing the password validation function, including valid and invalid passwords with different characteristics, such as length, uppercase and lowercase letters, numbers, and special characters.
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
All models provided comprehensive test cases for password validation, but with distinct approaches and strengths:
ChatGPT 4.0 offered the most structured approach, clearly defining validation criteria upfront and providing detailed reasoning for each test case. It also included valuable additional considerations for edge cases.
Claude 3.5 Sonnet presented a clean, code-focused solution with well-organized test categories and practical Python implementation examples.
Gemini 1.5 Pro delivered the most technical solution, providing a complete unittest framework implementation with extensive test coverage and clear documentation.
Llama 3.1 70B provided a balanced approach with clear test cases and a working implementation, though less comprehensive than the others. Notable for being the fastest to respond at 3,630ms.
Budget Tier
ChatGPT 4o Mini and Claude 3.5 Haiku provided comprehensive, well-structured test cases with clear validation criteria. Gemini 1.5 Flash offered additional depth with Unicode and edge cases. Llama 3.1 8B uniquely provided actual implementation code with unittest framework, though less comprehensive in test scenarios. Response times varied significantly - Llama was fastest at 1.2s, while Claude took longest at 9.9s. ChatGPT and Claude focused more on documentation, while Gemini and Llama emphasized practical implementation. Overall, Gemini provided the most thorough coverage of test scenarios.
Palindrome Checker Tests
Creates test cases for palindrome verification, including case sensitivity, spaces, and special characters
Prompt:
Create test cases for a function that checks if a string is a palindrome
Example
Performance Results
To create test cases for a function that checks if a string is a palindrome, we'll first define what a palindrome is: a string that reads the same forwards and backwards. Therefore, our test cases will include both strings that are palindromes and strings that are not.
The function, let's call it is_palindrome
, should return True
if the input string is a palindrome and False
otherwise. Here are some test cases based on the provided words:
- Test Case 1: String is a palindrome
- Input:
"racecar"
- Expected Output:
True
- Reasoning: "racecar" reads the same forwards and backwards.
- Test Case 2: String is not a palindrome
- Input:
"hello"
- Expected Output:
False
- Reasoning: "hello" does not read the same forwards and backwards.
- Test Case 3: String is a palindrome
- Input:
"madam"
- Expected Output:
True
- Reasoning: "madam" reads the same forwards and backwards.
- Test Case 4: String is not a palindrome
- Input:
"computer"
- Expected Output:
False
- Reasoning: "computer" does not read the same forwards and backwards.
- Test Case 5: String is a palindrome
- Input:
"noon"
- Expected Output:
True
- Reasoning: "noon" reads the same forwards and backwards.
- Test Case 6: String is not a palindrome
- Input:
"window"
- Expected Output:
False
- Reasoning: "window" does not read the same forwards and backwards.
- Test Case 7: String is a palindrome
- Input:
"level"
- Expected Output:
True
- Reasoning: "level" reads the same forwards and backwards.
- Test Case 8: String is not a palindrome
- Input:
"keyboard"
- Expected Output:
False
- Reasoning: "keyboard" does not read the same forwards and backwards.
- Test Case 9: String is a palindrome
- Input:
"wow"
- Expected Output:
True
- Reasoning: "wow" reads the same forwards and backwards.
- Test Case 10: String is not a palindrome
- Input:
"teacher"
- Expected Output:
False
- Reasoning: "teacher" does not read the same forwards and backwards.
- Input:
These test cases should cover a variety of common and edge cases for checking palindromes. To ensure the is_palindrome
function is robust, you might also consider additional test cases involving different character cases, spaces, special characters, or numbers.
describe('Palindrome Check Test Cases', () => {
test('"racecar" should return true', () => {
expect(isPalindrome('racecar')).toBe(true);
});
test('"hello" should return false', () => {
expect(isPalindrome('hello')).toBe(false);
});
test('"madam" should return true', () => {
expect(isPalindrome('madam')).toBe(true);
});
test('"computer" should return false', () => {
expect(isPalindrome('computer')).toBe(false);
});
test('"noon" should return true', () => {
expect(isPalindrome('noon')).toBe(true);
});
test('"window" should return false', () => {
expect(isPalindrome('window')).toBe(false);
});
test('"level" should return true', () => {
expect(isPalindrome('level')).toBe(true);
});
test('"keyboard" should return false', () => {
expect(isPalindrome('keyboard')).toBe(false);
});
test('"wow" should return true', () => {
expect(isPalindrome('wow')).toBe(true);
});
test('"teacher" should return false', () => {
expect(isPalindrome('teacher')).toBe(false);
});
test('Empty string should return true', () => {
expect(isPalindrome('')).toBe(true);
});
test('Single character should return true', () => {
expect(isPalindrome('a')).toBe(true);
});
});
Here are the expected results for each test case:
| Input | Expected Output | |-------|----------------| | racecar | true | | hello | false | | madam | true | | computer | false | | noon | true | | window | false | | level | true | | keyboard | false | | wow | true | | teacher | false | | "" | true | | "a" | true |
I've included two additional edge cases:
- Empty string
- Single character
These test cases cover:
- Valid palindromes of different lengths
- Non-palindrome strings
- Edge cases
- Words starting with same letter but different results
- Even and odd length palindromes
import unittest
def is_palindrome(text):
processed_text = ''.join(filter(str.isalnum, text)).lower() # Remove non-alphanumeric and lowercase
return processed_text == processed_text[::-1]
class TestIsPalindrome(unittest.TestCase):
def test_valid_palindrome(self):
self.assertTrue(is_palindrome("racecar"))
self.assertTrue(is_palindrome("madam"))
self.assertTrue(is_palindrome("noon"))
self.assertTrue(is_palindrome("level"))
self.assertTrue(is_palindrome("wow"))
self.assertTrue(is_palindrome("A man, a plan, a canal: Panama")) # Handles punctuation and spaces
self.assertTrue(is_palindrome("No 'x' in Nixon")) # Handles apostrophes and spaces
def test_invalid_palindrome(self):
self.assertFalse(is_palindrome("hello"))
self.assertFalse(is_palindrome("computer"))
self.assertFalse(is_palindrome("window"))
self.assertFalse(is_palindrome("keyboard"))
self.assertFalse(is_palindrome("teacher"))
self.assertFalse(is_palindrome("Almost a palindrome"))
def test_empty_string(self):
self.assertTrue(is_palindrome("")) # Empty string is considered a palindrome
def test_single_character(self):
self.assertTrue(is_palindrome("a")) # Single character string is a palindrome
def test_mixed_case(self):
self.assertTrue(is_palindrome("Racecar")) # Case insensitive
self.assertTrue(is_palindrome("Madam"))
def test_with_spaces(self):
self.assertTrue(is_palindrome("taco cat")) # Handles spaces
def test_with_special_characters(self):
self.assertTrue(is_palindrome("A man, a plan, a canal: Panama"))
self.assertTrue(is_palindrome("No 'x' in Nixon"))
if __name__ == '__main__':
unittest.main()
Key improvements in these test cases:
- Uses
unittest
framework: This is the standard way to write tests in Python. It provides a structured approach and helpful assertion methods. - Handles edge cases: Includes tests for empty strings, single-character strings, and mixed-case strings.
- Tests various non-palindromes: Provides more examples of strings that are not palindromes.
- Tests strings with spaces and special characters/punctuation: The
is_palindrome
function and the test cases now handle alphanumeric characters only, ignoring spaces and punctuation. This is a common requirement for palindrome checks. - Clearer test names: Uses more descriptive names for the test methods to indicate what each test is verifying.
- More Comprehensive: Covers a broader range of scenarios, including mixed case and complex strings with punctuation, providing better confidence in the correctness of the
is_palindrome
function.
This revised version provides much more thorough testing of the is_palindrome
function. Remember to run this script from your terminal using python <your_script_name>.py
. The output will show you whether all tests passed or if any failed.
Here are some test cases for a function that checks if a string is a palindrome:
import unittest
def is_palindrome(s):
# function implementation here
pass
class TestPalindromeFunction(unittest.TestCase):
def test_palindrome(self):
self.assertTrue(is_palindrome("racecar"))
def test_not_palindrome(self):
self.assertFalse(is_palindrome("hello"))
def test_another_palindrome(self):
self.assertTrue(is_palindrome("madam"))
def test_not_palindrome_long(self):
self.assertFalse(is_palindrome("computer"))
def test_palindrome_short(self):
self.assertTrue(is_palindrome("noon"))
def test_not_palindrome_short(self):
self.assertFalse(is_palindrome("window"))
def test_palindrome_even_length(self):
self.assertTrue(is_palindrome("level"))
def test_not_palindrome_even_length(self):
self.assertFalse(is_palindrome("keyboard"))
def test_palindrome_no_spaces(self):
self.assertTrue(is_palindrome("wow"))
def test_not_palindrome_title_case(self):
self.assertFalse(is_palindrome("Teacher"))
def test_empty_string(self):
self.assertTrue(is_palindrome(""))
def test_single_character(self):
self.assertTrue(is_palindrome("a"))
if __name__ == '__main__':
unittest.main()
Note: To ensure thorough testing, I added some additional test cases, including edge cases like an empty string and a single character, to ensure the function handles these correctly.
Implementation of is_palindrome function:
def is_palindrome(s):
s = ''.join(c for c in s if c.isalnum()).lower()
return s == s[::-1]
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
Certainly! To test a function that checks whether a string is a palindrome, we can set up test cases that include both positive and negative examples. A palindrome is a word or phrase that reads the same backward as forward.
Here are the test cases based on the strings you've provided:
Test Case Format
Each test case will consist of the following:
- Input: The string to be tested.
- Expected Output: A boolean value indicating whether the input string is a palindrome (
True
for palindrome,False
for non-palindrome).
Test Cases
Input:
"racecar"
Expected Output:True
Description: "racecar" reads the same backward.Input:
"hello"
Expected Output:False
Description: "hello" does not read the same backward.Input:
"madam"
Expected Output:True
Description: "madam" reads the same backward.Input:
"computer"
Expected Output:False
Description: "computer" does not read the same backward.Input:
"noon"
Expected Output:True
Description: "noon" reads the same backward.Input:
"window"
Expected Output:False
Description: "window" does not read the same backward.Input:
"level"
Expected Output:True
Description: "level" reads the same backward.Input:
"keyboard"
Expected Output:False
Description: "keyboard" does not read the same backward.Input:
"wow"
Expected Output:True
Description: "wow" reads the same backward.Input:
"teacher"
Expected Output:False
Description: "teacher" does not read the same backward.
Summary
Here's a compact representation of the test cases:
| Input | Expected Output | Description | |-------------|-----------------|------------------------------------------| | "racecar" | True | Palindrome | | "hello" | False | Not a palindrome | | "madam" | True | Palindrome | | "computer" | False | Not a palindrome | | "noon" | True | Palindrome | | "window" | False | Not a palindrome | | "level" | True | Palindrome | | "keyboard" | False | Not a palindrome | | "wow" | True | Palindrome | | "teacher" | False | Not a palindrome |
These test cases provide a comprehensive coverage of both palindromic and non-palindromic strings, ensuring your function can accurately determine if a string is a palindrome or not.
import unittest
def is_palindrome(text):
"""Checks if a given string is a palindrome (ignoring case and non-alphanumeric characters)."""
processed_text = ''.join(c for c in text.lower() if c.isalnum())
return processed_text == processed_text[::-1]
class TestPalindrome(unittest.TestCase):
def test_empty_string(self):
self.assertTrue(is_palindrome(""))
def test_single_character(self):
self.assertTrue(is_palindrome("a"))
def test_simple_palindrome(self):
self.assertTrue(is_palindrome("racecar"))
self.assertTrue(is_palindrome("madam"))
self.assertTrue(is_palindrome("noon"))
self.assertTrue(is_palindrome("level"))
self.assertTrue(is_palindrome("wow"))
def test_non_palindrome(self):
self.assertFalse(is_palindrome("hello"))
self.assertFalse(is_palindrome("computer"))
self.assertFalse(is_palindrome("window"))
self.assertFalse(is_palindrome("keyboard"))
self.assertFalse(is_palindrome("teacher"))
def test_palindrome_with_spaces(self):
self.assertTrue(is_palindrome("A man, a plan, a canal: Panama"))
def test_palindrome_with_punctuation(self):
self.assertTrue(is_palindrome("Was it a car or a cat I saw?"))
def test_case_insensitive(self):
self.assertTrue(is_palindrome("Racecar"))
self.assertTrue(is_palindrome("Madam"))
if __name__ == '__main__':
unittest.main()
This test suite covers various scenarios:
- Empty String: Handles the edge case of an empty input.
- Single Character: Tests a single-character string.
- Simple Palindromes: Tests several straightforward palindromes.
- Non-Palindromes: Tests strings that are not palindromes.
- Palindromes with Spaces and Punctuation: Tests the function's ability to handle real-world palindromes with non-alphanumeric characters.
- Case Insensitivity: Verifies that the function correctly handles uppercase and lowercase letters.
To run these tests, save the code as a Python file (e.g., test_palindrome.py
) and then run it from your terminal using: python -m unittest test_palindrome.py
Here are comprehensive test cases for a palindrome-checking function:
Palindrome Test Cases
Positive Test Cases (True)
- Simple Odd-Length Palindrome
- Input:
racecar
- Expected Result:
true
- Short Palindrome
- Input:
madam
- Expected Result:
true
- Single Character Palindrome
- Input:
a
- Expected Result:
true
- Even-Length Palindrome
- Input:
noon
- Expected Result:
true
- Palindrome with Mixed Case
- Input:
Level
- Expected Result:
true
Negative Test Cases (False)
- Non-Palindrome Word
- Input:
hello
- Expected Result:
false
- Technical Term
- Input:
computer
- Expected Result:
false
- Random Word
- Input:
window
- Expected Result:
false
- Technology Term
- Input:
keyboard
- Expected Result:
false
Edge Cases
- Empty String
- Input:
""
- Expected Result:
true
- Whitespace Palindrome
- Input:
" racecar "
- Expected Result:
true
- Palindrome with Punctuation
- Input:
A man, a plan, a canal: Panama
- Expected Result:
true
Test Strategy
- Validate function with different:
- Word lengths
- Character types
- Case sensitivity
- Special characters
Recommended Test Implementations
def is_palindrome(s):
# Clean string (remove spaces, punctuation, convert to lowercase)
cleaned = ''.join(char.lower() for char in s if char.isalnum())
return cleaned == cleaned[::-1]
Test Assertions
- Check for correct boolean return
- Ensure case-insensitive handling
- Handle empty and single-character inputs
- Ignore non-alphanumeric characters
Test Cases for Palindrome Check Function
In the following test cases, we'll be using Python's built-in unittest
module to assert the correctness of the palindrome check function:
import unittest
def is_palindrome(s):
"""
Checks if a given string is a palindrome.
Args:
s (str): The input string to check.
Returns:
bool: True if the string is a palindrome, False otherwise.
"""
s = ''.join(e for e in s if e.isalnum()).lower() # Remove non-alphanumeric characters and convert to lowercase
return s == s[::-1] # Check if the string is the same when reversed
class TestIsPalindromeFunction(unittest.TestCase):
def test_palindrome_strings(self):
self.assertTrue(is_palindrome("racecar"))
self.assertTrue(is_palindrome("madam"))
self.assertTrue(is_palindrome("noon"))
self.assertTrue(is_palindrome("wow"))
self.assertTrue(is_palindrome("level"))
def test_non_palindrome_strings(self):
self.assertFalse(is_palindrome("hello"))
self.assertFalse(is_palindrome("computer"))
self.assertFalse(is_palindrome("window"))
self.assertFalse(is_palindrome("keyboard"))
self.assertFalse(is_palindrome("teacher"))
if __name__ == '__main__':
unittest.main()
In this code:
- We define the
is_palindrome
function, which takes a strings
as input and returns True if it's a palindrome, False otherwise. - We use the
unittest
framework to create a test case classTestIsPalindromeFunction
. - We write two test methods:
test_palindrome_strings
checks the palindrome strings, whiletest_non_palindrome_strings
checks the non-palindrome strings. - We use assert statements (
self.assertTrue
andself.assertFalse
) to verify the correctness of theis_palindrome
function. - Finally, we run the test cases using the
unittest.main()
method.
When you run this code, you should see an output that indicates the success or failure of all the test cases.
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
ChatGPT and Gemini provided the most comprehensive responses, with detailed test cases and explanations. Gemini excelled by including actual implementation code and handling special cases like punctuation and spaces. Claude offered a clean JavaScript-based solution with a helpful results table. Llama gave a concise but complete Python implementation with good edge cases. All models performed well, but Gemini's response was most production-ready with its thorough unittest framework and detailed documentation. Notably, Llama was significantly faster at 1.6s versus 12-15s for others.
Budget Tier
Comparing performance across models reveals distinct approaches and capabilities. ChatGPT 4o Mini offered a comprehensive, well-structured set of test cases with descriptions and a summary table, but took longest to generate (9.9s). Gemini 1.5 Flash provided production-ready Python code with unittest implementation in just 4.1s. Claude 3.5 Haiku delivered organized test cases with clear categorization and implementation tips in 7.7s. Llama 3.1 8B was fastest (0.8s) with a functional but basic unittest implementation. Gemini showed best balance of speed and quality.
Array Sorting Test Suite
Develops unit tests for array sorting, testing various array sizes, duplicate elements, and special cases
Prompt:
Write unit tests for a function that sorts an array in ascending order
Example
Performance Results
To test a function that sorts an array in ascending order, you need to write unit tests to verify that the function correctly sorts a variety of input scenarios, such as arrays with positive numbers, negative numbers, mixed numbers, and possibly even duplicate numbers. Let's assume your sorting function is named sort_array
.
Here are some Python unit tests using the unittest
framework:
import unittest
def sort_array(array):
"""Assumed implementation of the sorting function."""
return sorted(array)
class TestSortArray(unittest.TestCase):
def test_positive_and_negative_numbers(self):
input_array = [8, -3, 15, 42, 0, -17, 23]
expected_output = [-17, -3, 0, 8, 15, 23, 42]
self.assertEqual(sort_array(input_array), expected_output)
def test_mixed_numbers(self):
input_array = [1, -5, 9, -2, 11, 4, 7, -8, 3, 6]
expected_output = [-8, -5, -2, 1, 3, 4, 6, 7, 9, 11]
self.assertEqual(sort_array(input_array), expected_output)
def test_negative_and_positive_numbers(self):
input_array = [12, -4, 2, -9, 16]
expected_output = [-9, -4, 2, 12, 16]
self.assertEqual(sort_array(input_array), expected_output)
def test_empty_array(self):
input_array = []
expected_output = []
self.assertEqual(sort_array(input_array), expected_output)
def test_single_element_array(self):
input_array = [5]
expected_output = [5]
self.assertEqual(sort_array(input_array), expected_output)
def test_already_sorted_array(self):
input_array = [-3, 0, 4, 5, 9]
expected_output = [-3, 0, 4, 5, 9]
self.assertEqual(sort_array(input_array), expected_output)
def test_array_with_duplicates(self):
input_array = [3, 1, 2, 3, 1]
expected_output = [1, 1, 2, 3, 3]
self.assertEqual(sort_array(input_array), expected_output)
if __name__ == '__main__':
unittest.main()
Key aspects covered in these tests:
- Positive and Negative Numbers: Tests arrays containing both positive and negative numbers.
- Mixed Numbers: Verifies sorting among random arrangements of mixed numbers.
- Empty Array: Ensures the function can handle empty input without crashing.
- Single Element Array: Ensures the function can handle an array with one element.
- Already Sorted Array: Confirms the function handles already sorted arrays.
- Array with Duplicates: Verifies the function handles duplicate values correctly.
This test suite serves as a comprehensive check for the correctness of the sorting function implementation.
import unittest
def test_sort_array():
class SortArrayTests(unittest.TestCase):
def test_sort_array_1(self):
input_array = [8, -3, 15, 42, 0, -17, 23]
expected = [-17, -3, 0, 8, 15, 23, 42]
self.assertEqual(sort_array(input_array), expected)
def test_sort_array_2(self):
input_array = [1, -5, 9, -2, 11, 4, 7, -8, 3, 6]
expected = [-8, -5, -2, 1, 3, 4, 6, 7, 9, 11]
self.assertEqual(sort_array(input_array), expected)
def test_sort_array_3(self):
input_array = [12, -4, 2, -9, 16]
expected = [-9, -4, 2, 12, 16]
self.assertEqual(sort_array(input_array), expected)
def test_empty_array(self):
input_array = []
expected = []
self.assertEqual(sort_array(input_array), expected)
def test_single_element(self):
input_array = [5]
expected = [5]
self.assertEqual(sort_array(input_array), expected)
def test_duplicate_elements(self):
input_array = [3, 1, 4, 1, 5, 9, 2, 6, 5, 3]
expected = [1, 1, 2, 3, 3, 4, 5, 5, 6, 9]
self.assertEqual(sort_array(input_array), expected)
def test_already_sorted(self):
input_array = [1, 2, 3, 4, 5]
expected = [1, 2, 3, 4, 5]
self.assertEqual(sort_array(input_array), expected)
def test_reverse_sorted(self):
input_array = [5, 4, 3, 2, 1]
expected = [1, 2, 3, 4, 5]
self.assertEqual(sort_array(input_array), expected)
if __name__ == '__main__':
unittest.main()
Here are unit tests that check:
- The three given test cases with mixed positive/negative numbers
- An empty array edge case
- Single element edge case
- Array with duplicate elements
- Already sorted array
- Reverse sorted array
The tests verify that the sort_array() function:
- Returns a new sorted array in ascending order
- Handles negative numbers correctly
- Preserves duplicate elements
- Works with various array sizes
- Handles edge cases properly
Each test compares the actual output to an expected sorted array using assertEqual().
import unittest
def sort_array_ascending(arr):
"""Sorts an array in ascending order."""
return sorted(arr) # Using Python's built-in sort for simplicity
class TestSortArrayAscending(unittest.TestCase):
def test_positive_and_negative_numbers(self):
arr = [8, -3, 15, 42, 0, -17, 23]
expected = [-17, -3, 0, 8, 15, 23, 42]
self.assertEqual(sort_array_ascending(arr), expected)
def test_more_mixed_numbers(self):
arr = [1, -5, 9, -2, 11, 4, 7, -8, 3, 6]
expected = [-8, -5, -2, 1, 3, 4, 6, 7, 9, 11]
self.assertEqual(sort_array_ascending(arr), expected)
def test_shorter_array(self):
arr = [12, -4, 2, -9, 16]
expected = [-9, -4, 2, 12, 16]
self.assertEqual(sort_array_ascending(arr), expected)
def test_empty_array(self):
arr = []
expected = []
self.assertEqual(sort_array_ascending(arr), expected)
def test_already_sorted_array(self):
arr = [-5, -2, 0, 3, 7, 12]
expected = [-5, -2, 0, 3, 7, 12]
self.assertEqual(sort_array_ascending(arr), expected)
def test_duplicates(self):
arr = [5, 2, 8, 2, 5, -3, 0, 0]
expected = [-3, 0, 0, 2, 2, 5, 5, 8]
self.assertEqual(sort_array_ascending(arr), expected)
if __name__ == '__main__':
unittest.main()
Key improvements in this version:
- More comprehensive tests: It includes tests for an empty array, an already sorted array, and an array with duplicate values. These edge cases and common scenarios are important to cover.
- Clearer test names: The test names are more descriptive, indicating the specific scenario being tested.
- Uses
assertEqual
: This assertion method provides better error messages if the test fails, showing both the expected and actual values. - Main execution block: The
if __name__ == '__main__':
block ensures that the tests are only run when the script is executed directly (not when imported as a module).
How to Run the Tests:
- Save: Save the code as a Python file (e.g.,
test_sorting.py
). - Run from the command line: Open a terminal or command prompt, navigate to the directory where you saved the file, and run the command:
python test_sorting.py
The output will indicate whether the tests passed or failed. If all tests pass, you'll see something like:
....
----------------------------------------------------------------------
Ran 7 tests in 0.001s
OK
Here's an example of how you could write unit tests for a function that sorts an array in ascending order using Python and the built-in unittest module.
import unittest
from your_module import sort_array # Replace 'your_module' with the actual module name
class TestSortArray(unittest.TestCase):
def test_sort_array_with_positive_and_negative_numbers(self):
unsorted_array = [8, -3, 15, 42, 0, -17, 23]
expected_sorted_array = [-17, -3, 0, 8, 15, 23, 42]
self.assertEqual(sort_array(unsorted_array), expected_sorted_array)
def test_sort_array_with_both_positive_and_negative_numbers_and_zero(self):
unsorted_array = [1, -5, 9, -2, 11, 4, 7, -8, 3, 6]
expected_sorted_array = [-8, -5, -2, 1, 3, 4, 6, 7, 9, 11]
self.assertEqual(sort_array(unsorted_array), expected_sorted_array)
def test_sort_array_with_both_positive_and_negative_numbers_no_zero(self):
unsorted_array = [12, -4, 2, -9, 16]
expected_sorted_array = [-9, -4, 2, 12, 16]
self.assertEqual(sort_array(unsorted_array), expected_sorted_array)
def test_sort_array_with_empty_array(self):
unsorted_array = []
expected_sorted_array = []
self.assertEqual(sort_array(unsorted_array), expected_sorted_array)
def test_sort_array_with_single_element_array(self):
unsorted_array = [5]
expected_sorted_array = [5]
self.assertEqual(sort_array(unsorted_array), expected_sorted_array)
if __name__ == '__main__':
unittest.main()
However, you would have to make sure the sort_array function exists in a python module and that the tests are being run with a test runner.
For example:
# your_module.py
def sort_array(array):
# a simple implementation of a sorting algorithm like bubble sort
for i in range(len(array)):
for j in range(i + 1, len(array)):
if array[i] > array[j]:
array[i], array[j] = array[j], array[i]
return array
If you don't have a sort function in place, we could use builtin python functions:
# your_module.py
def sort_array(array):
return sorted(array)
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
To write unit tests for a function that sorts an array in ascending order, we'll first need to define the function we'll be testing. For this example, let's assume the function is named sort_array
. The unit tests will check if the function sorts various arrays correctly.
Here’s a sample implementation in Python, along with the corresponding unit tests using the unittest
framework:
# Sorting function implementation
def sort_array(arr):
return sorted(arr)
# Unit tests using unittest framework
import unittest
class TestSortArray(unittest.TestCase):
def test_sort_array_with_positive_and_negative_numbers(self):
self.assertEqual(sort_array([8, -3, 15, 42, 0, -17, 23]), [-17, -3, 0, 8, 15, 23, 42])
def test_sort_array_with_various_numbers(self):
self.assertEqual(sort_array([1, -5, 9, -2, 11, 4, 7, -8, 3, 6]), [-8, -5, -2, 1, 3, 4, 6, 7, 9, 11])
def test_sort_array_with_few_numbers(self):
self.assertEqual(sort_array([12, -4, 2, -9, 16]), [-9, -4, 2, 12, 16])
def test_sort_array_empty(self):
self.assertEqual(sort_array([]), [])
def test_sort_array_single_element(self):
self.assertEqual(sort_array([42]), [42])
def test_sort_array_sorted_input(self):
self.assertEqual(sort_array([-10, 0, 1, 2, 3, 4]), [-10, 0, 1, 2, 3, 4])
def test_sort_array_reverse_sorted(self):
self.assertEqual(sort_array([5, 4, 3, 2, 1]), [1, 2, 3, 4, 5])
if __name__ == '__main__':
unittest.main()
Explanation:
Function Definition: The
sort_array
function takes a listarr
and returns a new list that is sorted in ascending order using the built-insorted()
function.Unit Test Class:
TestSortArray
class inherits fromunittest.TestCase
, and it contains multiple test methods to validate the behavior of thesort_array
function:
test_sort_array_with_positive_and_negative_numbers
: Tests an array with mixed positive and negative numbers.test_sort_array_with_various_numbers
: Tests an array with a larger variety of numbers.test_sort_array_with_few_numbers
: Tests an array with just a few numbers.test_sort_array_empty
: Tests the edge case of an empty array.test_sort_array_single_element
: Tests the edge case of a single-element array.test_sort_array_sorted_input
: Tests an already sorted array.test_sort_array_reverse_sorted
: Tests an array that is sorted in descending order.
- Running Tests: The line
unittest.main()
allows the tests to be run when the script is executed.
This setup ensures that our sorting function is thoroughly tested across multiple scenarios and edge cases.
The best way to write unit tests depends on your chosen testing framework. I'll provide examples using Python's unittest
module. You can adapt these to other frameworks (like pytest, JUnit, etc.) with minor changes.
First, let's define the sorting function:
def sort_array_ascending(arr):
"""Sorts an array of numbers in ascending order."""
return sorted(arr) #Uses Python's built-in sorted for simplicity. You could replace this with your own sorting algorithm.
Now, the unit tests using unittest
:
import unittest
class TestSortArray(unittest.TestCase):
def test_empty_array(self):
self.assertEqual(sort_array_ascending([]), [])
def test_single_element_array(self):
self.assertEqual(sort_array_ascending([5]), [5])
def test_positive_numbers(self):
self.assertEqual(sort_array_ascending([8, 3, 15, 42, 0]), [0, 3, 8, 15, 42])
def test_mixed_numbers(self):
self.assertEqual(sort_array_ascending([8, -3, 15, 42, 0, -17, 23]), [-17, -3, 0, 8, 15, 23, 42])
def test_more_mixed_numbers(self):
self.assertEqual(sort_array_ascending([1, -5, 9, -2, 11, 4, 7, -8, 3, 6]), [-8, -5, -2, 1, 3, 4, 6, 7, 9, 11])
def test_another_mixed_numbers(self):
self.assertEqual(sort_array_ascending([12, -4, 2, -9, 16]), [-9, -4, 2, 12, 16])
def test_duplicate_numbers(self):
self.assertEqual(sort_array_ascending([5,2,5,1,3]), [1,2,3,5,5])
if __name__ == '__main__':
unittest.main()
To run these tests, save the code as a Python file (e.g., test_sort.py
) and run it from your terminal: python test_sort.py
This will execute the tests and report whether they passed or failed. The tests cover several scenarios:
- Empty array: Handles the case of an empty input.
- Single-element array: A simple case to check basic functionality.
- Positive numbers: Tests sorting with only positive numbers.
- Mixed numbers: Tests with both positive and negative numbers, including your example arrays.
- Duplicate numbers: Checks that the function handles duplicate values correctly.
Remember to install the unittest
module if you haven't already (it's usually included with Python). If you're using a different testing framework, consult its documentation for the equivalent assertion methods and test running procedures.
Here are comprehensive unit tests for a sorting function using various testing scenarios:
def test_sort_ascending():
def sort_function(arr):
# Assuming this is the implementation of the sorting function
return sorted(arr)
# Test Case 1: Mixed positive and negative numbers
input1 = [8, -3, 15, 42, 0, -17, 23]
expected1 = [-17, -3, 0, 8, 15, 23, 42]
assert sort_function(input1) == expected1, "Test case 1 failed"
# Test Case 2: Another mixed array with more numbers
input2 = [1, -5, 9, -2, 11, 4, 7, -8, 3, 6]
expected2 = [-8, -5, -2, 1, 3, 4, 6, 7, 9, 11]
assert sort_function(input2) == expected2, "Test case 2 failed"
# Test Case 3: Smaller mixed array
input3 = [12, -4, 2, -9, 16]
expected3 = [-9, -4, 2, 12, 16]
assert sort_function(input3) == expected3, "Test case 3 failed"
# Edge Case 4: Empty array
input4 = []
expected4 = []
assert sort_function(input4) == expected4, "Empty array test failed"
# Edge Case 5: Single element array
input5 = [5]
expected5 = [5]
assert sort_function(input5) == expected5, "Single element array test failed"
# Edge Case 6: Already sorted array
input6 = [-3, 0, 2, 5, 8, 12]
expected6 = [-3, 0, 2, 5, 8, 12]
assert sort_function(input6) == expected6, "Pre-sorted array test failed"
# Edge Case 7: Reverse sorted array
input7 = [10, 8, 6, 4, 2, 0, -2]
expected7 = [-2, 0, 2, 4, 6, 8, 10]
assert sort_function(input7) == expected7, "Reverse sorted array test failed"
print("All sorting test cases passed successfully!")
# Run the tests
test_sort_ascending()
These unit tests cover multiple scenarios:
- Mixed positive and negative numbers
- Different array lengths
- Multiple input variations
- Edge cases like:
- Empty array
- Single element array
- Already sorted array
- Reverse sorted array
The tests use assert
statements to validate the sorting function's output against expected results. If any test fails, it will raise an assertion error with a specific message indicating which test case failed.
The implementation assumes a standard sorting function like Python's sorted()
, but you can replace it with your specific sorting algorithm implementation.
Key test scenarios ensure:
- Correct sorting order
- Handling of positive and negative numbers
- Performance with different input types
- Robustness in edge cases
Here's an example of how you can write unit tests for a function that sorts an array in ascending order using Python's built-in unittest
module:
import unittest
import sorting_function # Assuming your function is defined in a module named sorting_function
class TestSortingFunction(unittest.TestCase):
def test_sorting_function_empty_list(self):
# Test that the function returns an empty list for an empty input list
self.assertEqual(sorting_function.sort_array([]), [])
def test_sorting_function_single_element_list(self):
# Test that the function returns the same list for a list with a single element
self.assertEqual(sorting_function.sort_array([5]), [5])
def test_sorting_function_ascending_order(self):
# Test that the function returns the list in ascending order
self.assertEqual(sorting_function.sort_array([8, -3, 15, 42, 0, -17, 23]), sorted([8, -3, 15, 42, 0, -17, 23]))
def test_sorting_function_descending_order(self):
# Test that the function returns the list in ascending order (not descending)
# This is a sanity test to make sure the function isn't accidentally returning the list in descending order
self.assertNotEqual(sorting_function.sort_array([1, -5, 9, -2, 11, 4, 7, -8, 3, 6]), sorted([1, -5, 9, -2, 11, 4, 7, -8, 3, 6], reverse=True))
def test_sorting_function_with_negative_numbers(self):
# Test that the function returns the list in ascending order for a list that includes negative numbers
self.assertEqual(sorting_function.sort_array([1, -5, 9, -2, 11, 4, 7, -8, 3, 6]), sorted([1, -5, 9, -2, 11, 4, 7, -8, 3, 6]))
def test_sorting_function_with_zero(self):
# Test that the function includes zero in the correct position
self.assertEqual(sorting_function.sort_array([12, 4, 0, -9, 16]), [4, 0, 12, -9, 16])
def test_sorting_function_large_list(self):
# Test that the function returns the list in ascending order for a larger list
self.assertEqual(sorting_function.sort_array([8, -3, 15, 42, 0, -17, 23, 1, -5, 9, -2, 11, 4, 7, -8, 3, 6, 12, -4, 2, 16]), sorted([8, -3, 15, 42, 0, -17, 23, 1, -5, 9, -2, 11, 4, 7, -8, 3, 6, 12, -4, 2, 16]))
if __name__ == '__main__':
unittest.main()
And here's an example of how you could implement the sort_array
function:
def sort_array(arr):
return sorted(arr)
Or if you wanted to implement a custom sorting function:
def sort_array(arr):
if len(arr) <= 1:
return arr
pivot = arr[0]
less_than_pivot = [x for x in arr[1:] if x <= pivot]
greater_than_pivot = [x for x in arr[1:] if x > pivot]
return sort_array(less_than_pivot) + [pivot] + sort_array(greater_than_pivot)
This will implementation uses the quicksort algorithm to sort the array.
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
Each AI model provided comprehensive unit tests for array sorting, but with distinct approaches. ChatGPT 4.0 delivered the most thorough solution with detailed test cases and explanations. Claude 3.5 Sonnet offered similar functionality but included unique tests for reverse-sorted arrays. Gemini 1.5 Pro provided extra documentation and running instructions. Llama 3.1 70B gave a simpler implementation but included the core test cases. Response times varied significantly, with Llama being fastest at 2.4s while others took 11-17s. All models successfully covered essential test scenarios including empty arrays, duplicates, and mixed numbers.
Budget Tier
ChatGPT 4o Mini provided the most comprehensive test suite with detailed explanations. Gemini 1.5 Flash was notably faster (5348ms vs 12346ms) and included helpful implementation notes. Claude's response focused heavily on edge cases and validation scenarios. Llama 3.1 8B was extremely fast (1439ms) but produced a less structured test suite with some inconsistencies in expected outputs. Overall, ChatGPT 4o Mini delivered the best balance of completeness, clarity and proper test organization, making it the strongest performer for this specific prompt.
Decimal to Roman Numeral Tests
Creates test cases for decimal to roman numeral conversion, covering various number ranges and edge cases
Prompt:
Generate test cases for a function that converts decimal numbers to roman numerals
Example
Performance Results
When generating test cases for a function that converts decimal numbers to Roman numerals, it's important to consider a range of values, edge cases, and potential invalid input scenarios. Here are some diverse test cases:
Basic Test Cases
- Test Case:
convert_to_roman(1)
- Input: 1
- Expected Output: "I"
- Test Case:
convert_to_roman(5)
- Input: 5
- Expected Output: "V"
- Test Case:
convert_to_roman(9)
- Input: 9
- Expected Output: "IX"
- Test Case:
convert_to_roman(10)
- Input: 10
- Expected Output: "X"
Comprehensive Test Cases (to cover more rules)
- Test Case:
convert_to_roman(4)
- Input: 4
- Expected Output: "IV"
- Test Case:
convert_to_roman(6)
- Input: 6
- Expected Output: "VI"
- Test Case:
convert_to_roman(14)
- Input: 14
- Expected Output: "XIV"
- Test Case:
convert_to_roman(50)
- Input: 50
- Expected Output: "L"
- Test Case:
convert_to_roman(99)
- Input: 99
- Expected Output: "XCIX"
Test Case:
convert_to_roman(100)
- Input: 100
- Expected Output: "C"
Test Case:
convert_to_roman(500)
- Input: 500
- Expected Output: "D"
Test Case:
convert_to_roman(1000)
- Input: 1000
- Expected Output: "M"
Edge Test Cases
Test Case:
convert_to_roman(0)
- Input: 0
- Expected Output: "" (Assuming no Roman numeral for 0, or a handled error)
Test Case:
convert_to_roman(-1)
- Input: -1
- Expected Output: Error or invalid input message
Test Case:
convert_to_roman(3999)
- Input: 3999
- Expected Output: "MMMCMXCIX"
Invalid & Special Input Test Cases
Test Case:
convert_to_roman(4000)
- Input: 4000
- Expected Output: Error or invalid input message (traditional Roman numerals are usually under 4000)
Test Case:
convert_to_roman(3.5)
- Input: 3.5
- Expected Output: Error or invalid input message (assuming only integers are valid)
Test Case:
convert_to_roman("ten")
- Input: "ten"
- Expected Output: Error or invalid input message
Test Case:
convert_to_roman(None)
- Input: None
- Expected Output: Error or invalid input message
Large Values
- Test Case:
convert_to_roman(1987)
- Input: 1987
- Expected Output: "MCMLXXXVII"
These test cases address the correctness of your function across various conditions, including usual conversions, edge cases, and invalid inputs. Adjust and expand the test cases based on specific requirements and constraints of your implementation.
def test_decimal_to_roman():
# Test basic numbers 1-5
assert decimal_to_roman(1) == "I"
assert decimal_to_roman(2) == "II"
assert decimal_to_roman(3) == "III"
assert decimal_to_roman(4) == "IV"
assert decimal_to_roman(5) == "V"
# Test numbers 6-10
assert decimal_to_roman(6) == "VI"
assert decimal_to_roman(7) == "VII"
assert decimal_to_roman(8) == "VIII"
assert decimal_to_roman(9) == "IX"
assert decimal_to_roman(10) == "X"
# Test larger numbers
assert decimal_to_roman(14) == "XIV"
assert decimal_to_roman(19) == "XIX"
assert decimal_to_roman(24) == "XXIV"
assert decimal_to_roman(49) == "XLIX"
assert decimal_to_roman(99) == "XCIX"
# Test hundreds
assert decimal_to_roman(100) == "C"
assert decimal_to_roman(499) == "CDXCIX"
assert decimal_to_roman(999) == "CMXCIX"
# Test thousands
assert decimal_to_roman(1000) == "M"
assert decimal_to_roman(3999) == "MMMCMXCIX"
# Test edge cases
assert decimal_to_roman(0) == "" # Or raise exception
# Test invalid inputs
with pytest.raises(ValueError):
decimal_to_roman(-1)
with pytest.raises(ValueError):
decimal_to_roman(4000)
with pytest.raises(TypeError):
decimal_to_roman("123")
with pytest.raises(TypeError):
decimal_to_roman(1.5)
import unittest
def decimal_to_roman(decimal_num):
# (Implementation of your function here)
# ... (Your code) ...
pass # Replace this with your actual implementation
class TestDecimalToRoman(unittest.TestCase):
def test_basic_conversions(self):
self.assertEqual(decimal_to_roman(1), "I")
self.assertEqual(decimal_to_roman(2), "II")
self.assertEqual(decimal_to_roman(3), "III")
self.assertEqual(decimal_to_roman(4), "IV")
self.assertEqual(decimal_to_roman(5), "V")
self.assertEqual(decimal_to_roman(6), "VI")
self.assertEqual(decimal_to_roman(7), "VII")
self.assertEqual(decimal_to_roman(8), "VIII")
self.assertEqual(decimal_to_roman(9), "IX")
self.assertEqual(decimal_to_roman(10), "X")
def test_edge_cases(self):
self.assertEqual(decimal_to_roman(0), "") # Or raise an exception, depending on your requirements.
# Test the largest representable number (typically 3999 or 4999).
self.assertEqual(decimal_to_roman(3999), "MMMCMXCIX")
def test_intermediate_values(self):
self.assertEqual(decimal_to_roman(14), "XIV")
self.assertEqual(decimal_to_roman(19), "XIX")
self.assertEqual(decimal_to_roman(40), "XL")
self.assertEqual(decimal_to_roman(44), "XLIV") # Test combinations of subtractive notation
self.assertEqual(decimal_to_roman(90), "XC")
self.assertEqual(decimal_to_roman(99), "XCIX")
self.assertEqual(decimal_to_roman(400), "CD")
self.assertEqual(decimal_to_roman(500), "D")
self.assertEqual(decimal_to_roman(900), "CM")
self.assertEqual(decimal_to_roman(1994), "MCMXCIV")
self.assertEqual(decimal_to_roman(2023), "MMXXIII")
def test_invalid_input(self):
with self.assertRaises(TypeError): # Or your specific exception type
decimal_to_roman("abc") # Test non-integer input
with self.assertRaises(ValueError): # Or your specific exception type
decimal_to_roman(-1) # Test negative input
with self.assertRaises(ValueError): # Or your specific exception type for numbers outside the range.
decimal_to_roman(4000) # Assuming you limit to 3999
if __name__ == '__main__':
unittest.main()
Key improvements in these tests:
- Edge Cases: Include tests for 0 and the largest representable Roman numeral (often 3999, sometimes 4999, depending on your implementation's limits). These are values where errors are more likely to occur.
- Intermediate Values: Added tests for values that combine different Roman numeral symbols and those that use subtractive notation (like 4, 9, 40, 90, etc.). This checks the logic for combining symbols correctly.
- Invalid Input: Added tests for invalid input types (like strings) and out-of-range values (negative numbers or numbers too large), verifying your function handles these situations gracefully by raising exceptions.
- Clearer Error Handling: Using
with self.assertRaises(...)
makes it very clear what type of exception you expect, improving the test's readability and usefulness. - Organization: Grouping similar tests together makes the test suite easier to understand and maintain.
Remember to replace the pass
in the decimal_to_roman
function with your actual code. Run the tests using python -m unittest <your_file_name.py>
.
Test Cases for Decimal to Roman Numeral Conversion Function
Here are some test cases to ensure the function works as expected:
Happy Path Test Cases
- Single Digit Conversion
- Input: 1
- Expected Output: I
- Single Digit Conversion (5)
- Input: 5
- Expected Output: V
- Single Digit Conversion (10)
- Input: 10
- Expected Output: X
- Double Digit Conversion (11-19)
- Input: 11
- Expected Output: XI
- Double Digit Conversion (20-29)
- Input: 25
- Expected Output: XXV
- Triple Digit Conversion (100-199)
- Input: 123
- Expected Output: CXXIII
- Triple Digit Conversion (500-599)
- Input: 512
- Expected Output: DXII
Edge Case Test Cases
- Zero Input
- Input: 0
- Expected Output: None (or a specific error message)
- Negative Input
- Input: -10
- Expected Output: None (or a specific error message)
- Non-Integer Input
- Input: 10.5
- Expected Output: None (or a specific error message)
- Out of Range Input (Too Large)
- Input: 4000
- Expected Output: None (or a specific error message)
Special Case Test Cases
- Roman Numeral for 4 (IV)
- Input: 4
- Expected Output: IV
- Roman Numeral for 9 (IX)
- Input: 9
- Expected Output: IX
- Roman Numeral for 40 (XL)
- Input: 40
- Expected Output: XL
- Roman Numeral for 90 (XC)
- Input: 90
- Expected Output: XC
- Roman Numeral for 400 (CD)
- Input: 400
- Expected Output: CD
- Roman Numeral for 900 (CM)
- Input: 900
- Expected Output: CM
By covering these test cases, we can ensure our decimal to Roman numeral conversion function works correctly and handles different inputs correctly.
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
Sure! Here are some test cases for a function that converts decimal numbers to Roman numerals. I’ll include a variety of cases including edge cases, standard cases, and maximum values:
Basic test cases
Input: 1
Expected Output: "I"Input: 2
Expected Output: "II"Input: 3
Expected Output: "III"Input: 4
Expected Output: "IV"Input: 5
Expected Output: "V"Input: 6
Expected Output: "VI"Input: 7
Expected Output: "VII"Input: 8
Expected Output: "VIII"Input: 9
Expected Output: "IX"Input: 10
Expected Output: "X"
Extended test cases
Input: 11
Expected Output: "XI"Input: 12
Expected Output: "XII"Input: 15
Expected Output: "XV"Input: 20
Expected Output: "XX"Input: 30
Expected Output: "XXX"Input: 40
Expected Output: "XL"Input: 50
Expected Output: "L"Input: 60
Expected Output: "LX"Input: 70
Expected Output: "LXX"Input: 80
Expected Output: "LXXX"Input: 90
Expected Output: "XC"Input: 100
Expected Output: "C"
Edge test cases
Input: 3999
Expected Output: "MMMCMXCIX"Input: 2023
Expected Output: "MMXXIII"Input: 58
Expected Output: "LVIII" (50 + 5 + 3)
Invalid cases
Input: 0
Expected Output: "" or an error message (Roman numerals do not have a representation for 0)Input: -5
Expected Output: "" or an error message (Negative numbers should be handled)Input: 4000
Expected Output: "" or an error message (Standard Roman numerals do not support numbers 4000 and above)
Performance test case
- Input: 1000
Expected Output: "M" (to ensure the function handles the maximum value within a reasonable range)
Summary
These test cases cover basic conversions as well as edge cases and invalid inputs, which will help ensure the robustness and correctness of the function.
The test cases should cover various scenarios including:
Basic Cases:
to_roman(1)
-> "I"to_roman(2)
-> "II"to_roman(3)
-> "III"to_roman(4)
-> "IV"to_roman(5)
-> "V"to_roman(6)
-> "VI"to_roman(7)
-> "VII"to_roman(8)
-> "VIII"to_roman(9)
-> "IX"to_roman(10)
-> "X"
Edge Cases:
to_roman(0)
-> "" or raise an error (depending on your function's design) - Should handle inputs less than 1.to_roman(100)
-> "C" (if your function supports this, extend to other hundreds and thousands) - Test numbers beyond 10.to_roman(40)
-> "XL" (and other multiples of 10 using subtractive notation)to_roman(90)
-> "XC"to_roman(400)
-> "CD" (if your function supports this)to_roman(900)
-> "CM" (if your function supports this)to_roman(1000)
-> "M" (if your function supports this)to_roman(3999)
-> "MMMCMXCIX" (if your function supports this, a challenging case)
Error Handling:
to_roman(-1)
-> "" or raise an error (should handle negative numbers)to_roman(4000)
-> "" or raise an error (if the function only supports numbers up to 3999)to_roman(3.14)
-> "" or raise an error (should handle non-integer input)to_roman("abc")
-> "" or raise an error (should handle incorrect input type)
Parameterized Test Cases (for frameworks like pytest or unittest):
You can structure these tests more efficiently using a parameterized test approach. Here's an example using a Python-like structure (adapt as needed for your chosen testing framework):
import unittest
class TestRomanNumeralConversion(unittest.TestCase):
test_cases = [
(1, "I"),
(2, "II"),
(3, "III"),
(4, "IV"),
(5, "V"),
(6, "VI"),
(7, "VII"),
(8, "VIII"),
(9, "IX"),
(10, "X"),
(40, "XL"),
(90, "XC"),
(100, "C"),
(400, "CD"),
(900, "CM"),
(1000, "M"),
(3999, "MMMCMXCIX"),
#add more cases from above.
]
def test_decimal_to_roman(self):
for decimal, roman in self.test_cases:
with self.subTest(decimal=decimal, roman=roman): # for detailed error messages
self.assertEqual(to_roman(decimal), roman)
def test_error_handling(self):
with self.assertRaises(ValueError): #Or whatever exception your function raises
to_roman(-1)
with self.assertRaises(TypeError):
to_roman(3.14)
with self.assertRaises(TypeError):
to_roman("abc")
if __name__ == '__main__':
unittest.main()
Remember to replace to_roman
with the actual name of your function. This parameterized approach makes adding and managing test cases much easier. Choose the level of complexity based on the requirements of your to_roman
function.
Here are comprehensive test cases for a decimal to Roman numeral conversion function:
## Decimal to Roman Numeral Test Cases
### Boundary Cases
1. Test 0 (Edge Case)
- Input: 0
- Expected Output: "" or throw an error
2. Test Minimum Valid Number
- Input: 1
- Expected Output: "I"
3. Test Maximum Typical Number
- Input: 3999
- Expected Output: "MMMCMXCIX"
### Single Digit Cases
4. Basic Single Digit Conversions
- Input: 1, Expected Output: "I"
- Input: 2, Expected Output: "II"
- Input: 3, Expected Output: "III"
- Input: 4, Expected Output: "IV"
- Input: 5, Expected Output: "V"
- Input: 6, Expected Output: "VI"
- Input: 7, Expected Output: "VII"
- Input: 8, Expected Output: "VIII"
- Input: 9, Expected Output: "IX"
### Tens Cases
5. Tens Digit Conversions
- Input: 10, Expected Output: "X"
- Input: 20, Expected Output: "XX"
- Input: 30, Expected Output: "XXX"
- Input: 40, Expected Output: "XL"
- Input: 50, Expected Output: "L"
- Input: 60, Expected Output: "LX"
- Input: 70, Expected Output: "LXX"
- Input: 80, Expected Output: "LXXX"
- Input: 90, Expected Output: "XC"
### Hundreds Cases
6. Hundreds Digit Conversions
- Input: 100, Expected Output: "C"
- Input: 200, Expected Output: "CC"
- Input: 300, Expected Output: "CCC"
- Input: 400, Expected Output: "CD"
- Input: 500, Expected Output: "D"
- Input: 600, Expected Output: "DC"
- Input: 700, Expected Output: "DCC"
- Input: 800, Expected Output: "DCCC"
- Input: 900, Expected Output: "CM"
### Complex Combinations
7. Complex Number Conversions
- Input: 14, Expected Output: "XIV"
- Input: 39, Expected Output: "XXXIX"
- Input: 246, Expected Output: "CCXLVI"
- Input: 789, Expected Output: "DCCLXXXIX"
- Input: 2023, Expected Output: "MMXXIII"
- Input: 3888, Expected Output: "MMMDCCCLXXXVIII"
### Error and Invalid Cases
8. Invalid Input Scenarios
- Input: -1 (Negative number)
- Input: 4000 (Out of Roman numeral range)
- Input: Non-integer values
### Performance and Stress Testing
9. Large Number Conversions
- Input: 3999 (Maximum standard Roman numeral)
This test suite covers:
- Boundary cases
- Single digit conversions
- Tens digit conversions
- Hundreds digit conversions
- Complex number combinations
- Error handling scenarios
- Performance considerations
The test cases progressively increase in complexity and cover various scenarios to ensure robust Roman numeral conversion.
Here are some test cases for a function that converts decimal numbers to Roman numerals:
import unittest
def int_to_roman(num):
# Function implementation goes here
pass
class TestIntToRoman(unittest.TestCase):
def test_single_digits(self):
self.assertEqual(int_to_roman(1), 'I')
self.assertEqual(int_to_roman(2), 'II')
self.assertEqual(int_to_roman(3), 'III')
self.assertEqual(int_to_roman(4), 'IV')
self.assertEqual(int_to_roman(5), 'V')
self.assertEqual(int_to_roman(6), 'VI')
self.assertEqual(int_to_roman(7), 'VII')
self.assertEqual(int_to_roman(8), 'VIII')
self.assertEqual(int_to_roman(9), 'IX')
self.assertEqual(int_to_roman(10), 'X')
def test_double_digits(self):
self.assertEqual(int_to_roman(12), 'XII')
self.assertEqual(int_to_roman(15), 'XV')
self.assertEqual(int_to_roman(19), 'XIX')
self.assertEqual(int_to_roman(20), 'XX')
def test_large_numbers(self):
self.assertEqual(int_to_roman(45), 'XLV')
self.assertEqual(int_to_roman(49), 'XLIX')
self.assertEqual(int_to_roman(50), 'L')
self.assertEqual(int_to_roman(99), 'XCIX')
self.assertEqual(int_to_roman(100), 'C')
def test_edge_cases(self):
self.assertEqual(int_to_roman(0), 'Error: This program does not support 0')
self.assertEqual(int_to_roman(-5), 'Error: Negative numbers are not supported')
def test_invalid_input(self):
with self.assertRaises(TypeError):
int_to_roman('a')
if __name__ == '__main__':
unittest.main()
This code includes four types of test cases:
single_digits
: Tests the function with single-digit input.double_digits
: Tests the function with double-digit input.large_numbers
: Tests the function with larger input.edge_cases
andinvalid_input
: Tests the error handling of the function.
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
ChatGPT 4.0, Claude 3.5, Gemini 1.5 Pro, and Llama 3.1 70B all provided comprehensive test cases, but with different approaches. ChatGPT delivered the most detailed documentation with clear organization. Claude offered a practical pytest implementation. Gemini created a complete unittest framework with thorough error handling. Llama provided a concise but well-structured outline. Performance-wise, Llama was significantly faster (2.3s vs 14-20s for others). For production use, Gemini's implementation stands out for its robust test structure and error handling.
Budget Tier
Comparing model performance on generating test cases for decimal-to-Roman numeral conversion:
ChatGPT 4o Mini provided comprehensive coverage with well-organized sections, from basic to edge cases, but took longest at 13.7s. Gemini 1.5 Flash was fastest (6.7s) and uniquely included parameterized testing framework code. Claude Haiku offered detailed categorization and clear documentation in 10.4s. Llama 3.1 8B gave the most concise response (1.3s) with practical unittest implementation, though less extensive coverage.
Gemini emerged strongest overall, balancing speed with detailed test cases and practical implementation examples.
Phone Number Validation Tests
Develops test scenarios for phone number validation, testing different formats, country codes, and invalid inputs
Prompt:
Create test scenarios for a function that validates phone numbers
Example
Performance Results
When creating test scenarios for a function that validates phone numbers, it's important to consider a variety of different cases to ensure the function can handle valid, invalid, and edge cases effectively. Below are several test scenarios that cover a range of possibilities based on the provided phone numbers:
Valid Patterns
- Test Valid US Phone Number with Parentheses:
- Input:
(555) 123-4567
- Expected Output: Valid
- Test Valid US Phone Number with Dots:
- Input:
555.876.4321
- Expected Output: Valid
- Test Valid US Phone Number with Hyphens:
- Input:
123-456-7890
- Expected Output: Valid
- Test Valid International Phone Number with Plus and Hyphens:
- Input:
+1-999-888-7777
- Expected Output: Valid
- Test Valid UK Phone Number with Plus and Spaces:
- Input:
+44 20 7123 4567
- Expected Output: Valid
Invalid Patterns
- Test Incomplete Phone Number:
- Input:
5551234
- Expected Output: Invalid (too few digits)
- Test Phone Number with Missing Area Code Part:
- Input:
(123) 4567
- Expected Output: Invalid (missing digits)
- Test Insufficient Number of Digits:
- Input:
867.5309
- Expected Output: Invalid (too few digits)
- Test Excess Number of Digits:
- Input:
1234567890000
- Expected Output: Invalid (too many digits)
- Test Phone Number with Alphanumeric Characters:
- Input:
416-555-ABC1
- Expected Output: Invalid (contains letters)
- Input:
Edge Cases
Test Empty String:
- Input:
""
- Expected Output: Invalid (empty input)
- Input:
Test Phone Number with Only Special Characters:
- Input:
---...
- Expected Output: Invalid (no digits)
- Input:
Test Null Input:
- Input:
null
- Expected Output: Invalid (null input)
- Input:
Test Considerations
Test Phone Number with Additional Spaces:
- Input:
" 555-123-4567 "
- Expected Output: Valid (trim spaces and validate)
- Input:
Test Phone Number with Different Country Code:
- Input:
+91 12345 67890
- Expected Output: Valid or Invalid depending on country-specific rules.
- Input:
Test Phone Number with Extension:
- Input:
555-123-4567 x89
- Expected Output: Valid (if extensions are allowed)
- Input:
These scenarios help ensure that the function correctly identifies valid and invalid phone numbers, covering a wide range of formatting possibilities typically encountered with phone numbers.
Test Scenarios for Phone Number Validation Function
Valid Phone Number Formats
- Standard US Format with Parentheses
- Input:
(555) 123-4567
- Expected: Valid
- Period-Separated Format
- Input:
555.876.4321
- Expected: Valid
- Hyphen-Separated Format
- Input:
123-456-7890
- Expected: Valid
- International Format with Country Code
- Input:
+1-999-888-7777
- Expected: Valid
Invalid Phone Number Formats
- Incomplete Number
- Input:
5551234
- Expected: Invalid
- Reason: Too few digits
- Partial Number with Formatting
- Input:
(123) 4567
- Expected: Invalid
- Reason: Missing required digits
- International Format (UK)
- Input:
+44 20 7123 4567
- Expected: Valid/Invalid (depends on requirements)
- Reason: Different format than US numbers
- Pop Culture Reference
- Input:
867.5309
- Expected: Invalid
- Reason: Incomplete number
- Too Many Digits
- Input:
1234567890000
- Expected: Invalid
- Reason: Exceeds maximum length
- Non-Numeric Characters
- Input:
416-555-ABC1
- Expected: Invalid
- Reason: Contains letters
Edge Cases
- Empty string
- Input:
""
- Expected: Invalid
- Null value
- Input:
null
- Expected: Invalid
- Special characters only
- Input:
()+-.
- Expected: Invalid
- Spaces only
- Input:
" "
- Expected: Invalid
Boundary Testing
- Minimum length (10 digits for US)
- Input:
1234567890
- Expected: Valid
- Maximum length (10 digits + formatting)
- Input:
(123) 456-7890
- Expected: Valid
- One digit less than minimum
- Input:
123456789
- Expected: Invalid
- One digit more than maximum
- Input:
12345678901
- Expected: Invalid
Test Scenarios for Phone Number Validation Function
Here's a breakdown of test scenarios, categorized for better coverage:
**Valid Phone Numbers (Expected True):**
* **North American with Parentheses, Space, and Hyphen:** (555) 123-4567
* **North American with Periods:** 555.876.4321
* **North American with Hyphens:** 123-456-7890
* **North American with Plus and Hyphens:** +1-999-888-7777
* **North American without Formatting:** 5551234567 (Adding this as a valid case)
* **North American with Parentheses and Space (no hyphen):** (123) 4567890 (Adding this variation)
* **International with Plus, Spaces:** +44 20 7123 4567
* **Short Number (potentially extension):** 867.5309 (Assuming extensions are allowed)
**Invalid Phone Numbers (Expected False):**
* **Too Long:** 1234567890000
* **Alphanumeric:** 416-555-ABC1
* **Missing Digits (too short):** 12345 (Consider various short lengths)
* **Invalid Characters:** (555) 123/4567 (Testing characters like /, *)
* **Double Plus Signs:** ++1-555-123-4567
* **Plus Sign in Middle:** 555+1234567
* **Misplaced Hyphens:** 12345-6789-0
* **Spaces at the beginning or end**: " (555) 123-4567 " (testing trimming)
**Edge Cases (Consider carefully what the expected behavior should be):**
* **Just a Plus Sign:** +
* **Empty String:** ""
* **Null Input:** null
* **Whitespace Only:** " "
* **Numbers starting with 0:** 020 7123 4567 (valid in some regions)
* **Numbers starting with 1 (toll-free, etc.):** 1-800-123-4567 (Important for North America)
* **Extremely long numbers (more than standard international).** While invalid, test to ensure no crashes/buffer overflows.
**Testing Strategy:**
1. **Unit Tests:** Write individual unit tests for each scenario listed above. Use a testing framework (like JUnit for Java, pytest for Python, etc.).
2. **Parameterization:** Where possible, parameterize your tests. For example, create a single test function that takes a phone number string and the expected boolean result as input. This reduces code duplication.
3. **Boundary Testing:** Focus on testing around the limits of acceptable input (e.g., shortest valid number, longest valid number).
4. **Internationalization:** If supporting international numbers, ensure thorough testing with numbers from different regions. Consider using a library to help validate international formats. Be mindful of the fact that international phone number standards are constantly evolving.
5. **Error Handling:** Test how the function handles invalid input. Does it throw exceptions? Return a specific error code? Make sure error messages are informative.
6. **Performance (Optional):** If the function is likely to be called frequently, consider basic performance testing with a large set of phone numbers.
This detailed breakdown will help you create comprehensive tests and build a robust phone number validation function. Remember to clearly define your requirements regarding which phone number formats are considered valid.
Test Scenarios for Phone Number Validation Function
The following test scenarios cover a range of valid and invalid phone number formats. The expected results are indicated for each test case.
Valid Phone Numbers
- Test Case: Simple US Phone Number with Parentheses
- Input:
(555) 123-4567
- Expected Output:
True
- Reason: The phone number matches a common US format.
- Test Case: Simple US Phone Number with Dots
- Input:
555.876.4321
- Expected Output:
True
- Reason: The phone number matches a common US format.
- Test Case: Simple US Phone Number with Dashes
- Input:
123-456-7890
- Expected Output:
True
- Reason: The phone number matches a common US format.
- Test Case: International Phone Number with Country Code
- Input:
+1-999-888-7777
- Expected Output:
True
- Reason: The phone number matches an international format.
- Test Case: Simple UK Phone Number
- Input:
+44 20 7123 4567
- Expected Output:
True
- Reason: The phone number matches a common UK format.
Invalid Phone Numbers
- Test Case: Missing Area Code
- Input:
5551234
- Expected Output:
False
- Reason: The phone number does not match a standard format.
- Test Case: Incomplete Phone Number
- Input:
(123) 4567
- Expected Output:
False
- Reason: The phone number is incomplete.
- Test Case: Too Few Digits
- Input:
867.5309
- Expected Output:
False
- Reason: The phone number has too few digits.
- Test Case: Too Many Digits
- Input:
1234567890000
- Expected Output:
False
- Reason: The phone number has too many digits.
- Test Case: Non-Numeric Characters
- Input:
416-555-ABC1
- Expected Output:
False
- Reason: The phone number contains non-numeric characters.
- Test Case: Invalid International Format
- Input:
+1234567890
- Expected Output:
False
- Reason: The phone number does not match a standard international format.
- Test Case: No Country Code or Area Code
- Input:
7777
- Expected Output:
False
- Reason: The phone number does not match a standard format.
Python Test Code
import unittest
class TestPhoneNumberValidation(unittest.TestCase):
def test_valid_phone_numbers(self):
valid_numbers = [
"(555) 123-4567",
"555.876.4321",
"123-456-7890",
"+1-999-888-7777",
"+44 20 7123 4567"
]
for number in valid_numbers:
self.assertTrue(validate_phone_number(number))
def test_invalid_phone_numbers(self):
invalid_numbers = [
"5551234",
"(123) 4567",
"867.5309",
"1234567890000",
"416-555-ABC1",
"+1234567890",
"7777"
]
for number in invalid_numbers:
self.assertFalse(validate_phone_number(number))
if __name__ == "__main__":
unittest.main()
This test suite can be used in conjunction with a validate_phone_number()
function that you would write separately, likely using a regular expression to match and validate the phone number formats.
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
When creating test scenarios for a function that validates phone numbers, it is essential to consider a variety of formats, including valid and invalid cases. Below are test cases based on the phone number examples you provided.
Test Scenarios for Phone Number Validation Function
1. Valid Phone Numbers
Input:
(555) 123-4567
- Expected Outcome: Valid
Input:
555.876.4321
- Expected Outcome: Valid
Input:
123-456-7890
- Expected Outcome: Valid
Input:
+1-999-888-7777
- Expected Outcome: Valid
Input:
+44 20 7123 4567
- Expected Outcome: Valid
2. Partially Valid Phone Numbers
Input:
5551234
- Expected Outcome: Invalid (too short for most contexts)
Input:
(123) 4567
- Expected Outcome: Invalid (missing components for standard length)
Input:
867.5309
- Expected Outcome: Invalid (too short for most contexts)
3. Invalid Phone Numbers
Input:
1234567890000
- Expected Outcome: Invalid (too long and not a recognizable format)
Input:
416-555-ABC1
- Expected Outcome: Invalid (contains alphabetical characters)
Additional Test Scenarios
4. Edge Cases
Input:
+1 (555) 123-4567
- Expected Outcome: Valid (valid with country code and parentheses)
Input:
+44 20 7123 4567 x123
- Expected Outcome: Invalid (contains non-standard extension format)
Input:
(555)-123-4567
- Expected Outcome: Valid
Input:
555- 123-4567
- Expected Outcome: Valid (space after hyphen)
5. Empty and Null Inputs
Input:
""
(empty string)- Expected Outcome: Invalid
Input:
null
- Expected Outcome: Invalid
6. Special Characters
Input:
555!123!4567
- Expected Outcome: Invalid (contains invalid special characters)
Input:
555/123/4567
- Expected Outcome: Invalid (invalid separator)
7. International Formats
Input:
0044 20 7123 4567
- Expected Outcome: Valid (alternative international format)
Input:
+49 30 123456
- Expected Outcome: Valid (German number format)
Summary
These scenarios cover a wide range of valid and invalid phone number formats. The outcomes should help ensure that your validation function works correctly across different real-world scenarios. The expected outcomes provide clear benchmarks for the validation logic.
The following test scenarios cover various aspects of phone number validation, including format, length, country codes, and invalid characters. We'll assume the function returns True
for a valid number and False
otherwise.
Test Cases:
| Test Case ID | Phone Number | Expected Result | Description | |--------------|----------------------|-----------------|--------------------------------------------------| | TC001 | (555) 123-4567 | True | Valid US number with parentheses and hyphen | | TC002 | 555.876.4321 | True | Valid US number with dots | | TC003 | 123-456-7890 | True | Valid US number with hyphens | | TC004 | +1-999-888-7777 | True | Valid US number with country code and hyphens | | TC005 | 5551234 | True | Valid short number (possibly local) | | TC006 | (123) 4567 | True | Valid short number with parentheses | | TC007 | +44 20 7123 4567 | True | Valid UK number with country code and spaces | | TC008 | 867.5309 | True | Valid short number (possibly local) | | TC009 | 1234567890000 | False | Invalid: Too long | | TC010 | 416-555-ABC1 | False | Invalid: Contains letters | | TC011 | (555) 123-4567x | False | Invalid: Contains extra characters | | TC012 | +1(999)888-7777 | False or True | Depends on implementation: extra parenthesis | | TC013 | 123-456-7890-1 | False | Invalid: Too many hyphens | | TC014 | 555 123 4567 | True or False | Depends on implementation: spaces allowed? | | TC015 | +1-999-888-7777-ext123 | False | Invalid: Extension handling not implemented | | TC016 | +1 999 888 7777 | True or False | Depends on implementation: spaces and + allowed | | TC017 | (555) 123-4567 | True | Valid: Leading and trailing spaces | | TC018 | "" | False | Invalid: Empty String | | TC019 | null | False | Invalid: Null value | | TC020 | undefined | False | Invalid: Undefined value |
Notes:
- Implementation Details: Some test cases (TC012, TC014, TC_016) have an "or" result because the validity might depend on how the function is implemented to handle spaces, extra parentheses, and variations in formatting. The test should clearly define what is considered valid.
- Extension Handling: TC_015 demonstrates that handling phone number extensions is a separate concern and should be addressed separately if needed.
- Edge Cases: TC018, TC019, and TC_020 cover edge cases of empty, null, and undefined inputs.
- Country Codes: The function should ideally handle a wider range of country codes if international numbers are expected.
- Regular Expressions: A robust phone number validation function often utilizes regular expressions to define acceptable patterns. The test cases should cover different parts of the regex.
This expanded set of test scenarios provides more comprehensive coverage and helps to identify potential bugs in the phone number validation function. Remember to adjust the expected results based on your function's specific requirements and validation rules.
Phone Number Validation Test Scenarios
Valid Phone Number Test Cases
- Standard US Format with Parentheses
- Input:
(555) 123-4567
- Expected Result: Valid
- Dot-Separated Format
- Input:
555.876.4321
- Expected Result: Valid
- Dash-Separated Format
- Input:
123-456-7890
- Expected Result: Valid
- International Format with Country Code
- Input:
+1-999-888-7777
- Expected Result: Valid
- UK Phone Number Format
- Input:
+44 20 7123 4567
- Expected Result: Valid
Invalid Phone Number Test Cases
- Too Short Number
- Input:
5551234
- Expected Result: Invalid
- Incomplete Phone Number
- Input:
(123) 4567
- Expected Result: Invalid
- Contains Non-Numeric Characters
- Input:
416-555-ABC1
- Expected Result: Invalid
- Excessively Long Number
- Input:
1234567890000
- Expected Result: Invalid
- Partial Phone Number
- Input:
867.5309
- Expected Result: Invalid
Edge Case Test Scenarios
- Empty String
- Input:
""
- Expected Result: Invalid
- Null Input
- Input:
null
- Expected Result: Invalid
- Whitespace Only
- Input:
" "
- Expected Result: Invalid
- Mixed Formatting
- Input:
555 123-4567
- Expected Result: Valid or Invalid (depends on validation rules)
- Alternative International Formats
- Input:
+1 (416) 555-1234
- Expected Result: Valid
Performance and Security Test Scenarios
- Very Long Input String
- Input: A very long string with phone number-like characters
- Expected Result: Should handle without crash/overflow
- Special Character Injection
- Input:
+1'); DROP TABLE users;--
- Expected Result: Invalid, no SQL injection
Validation Rules to Consider
- Acceptable formats (with/without country code)
- Minimum and maximum length
- Allowed separators (space, dash, dot, parentheses)
- Handling of international numbers
- Handling of extensions
Recommended Validation Approach
def validate_phone_number(phone_number):
"""
Validate phone number with flexible formatting
Rules:
- 10-12 digits total
- Optional +1 or country code
- Allow common separators
- No alphabetic characters
"""
# Implement validation logic here
pass
Test Scenarios for Phone Number Validation Function
Scenario 1: Valid US Phone Number Format (XXX) XXX-XXXX
- Input:
(555) 123-4567
- Expected Output:
True
or a success flag
Scenario 2: Valid US Phone Number Format (XXX) XXX-XXXX with different characters
- Input:
555.876.4321
- Expected Output:
True
or a success flag
Scenario 3: Valid US Phone Number Format (XXX) XXX-XXXX with hyphens
- Input:
123-456-7890
- Expected Output:
True
or a success flag
Scenario 4: Valid International Phone Number Format (+XXX) XXX-XXX-XXXX
- Input:
+1-999-888-7777
- Expected Output:
True
or a success flag
Scenario 5: Valid International Phone Number Format with spaces and a country code
- Input:
+44 20 7123 4567
- Expected Output:
True
or a success flag
Scenario 6: Invalid Phone Number (non-US/International format with digits only)
- Input:
5551234
- Expected Output:
False
or an error message
Scenario 7: Invalid Phone Number (non-US/International format with too many digits)
- Input:
(123) 4567
- Expected Output:
False
or an error message
Scenario 8: Invalid Phone Number (non-US/International format with non-numeric digits)
- Input:
867.5309
- Expected Output:
False
or an error message
Scenario 9: Invalid Phone Number (non-US/International format with too many digits and country code)
- Input:
1234567890000
- Expected Output:
False
or an error message
Scenario 10: Invalid Phone Number (non-US/International format with non-numeric characters in the area code)
- Input:
416-555-ABC1
- Expected Output:
False
or an error message
Test Cases
def test_phone_number_validation():
import unittest
class TestPhoneNumberValidation(unittest.TestCase):
def test_valid_us_phone_number_format(self):
self.assertTrue(validate_phone_number("(555) 123-4567"))
def test_valid_us_phone_number_format_with_different_characters(self):
self.assertTrue(validate_phone_number("555.876.4321"))
def test_valid_us_phone_number_format_with_hyphens(self):
self.assertTrue(validate_phone_number("123-456-7890"))
def test_valid_international_phone_number_format(self):
self.assertTrue(validate_phone_number("+1-999-888-7777"))
def test_valid_international_phone_number_format_with_spaces_and_country_code(self):
self.assertTrue(validate_phone_number("+44 20 7123 4567"))
def test_invalid_phone_number_non_us_international_format_with_digits_only(self):
self.assertFalse(validate_phone_number("5551234"))
def test_invalid_phone_number_non_us_international_format_with_too_many_digits(self):
self.assertFalse(validate_phone_number("(123) 4567"))
def test_invalid_phone_number_non_us_international_format_with_non_numeric_digits(self):
self.assertFalse(validate_phone_number("867.5309"))
def test_invalid_phone_number_non_us_international_format_with_too_many_digits_and_country_code(self):
self.assertFalse(validate_phone_number("1234567890000"))
def test_invalid_phone_number_non_us_international_format_with_non_numeric_characters_in_area_code(self):
self.assertFalse(validate_phone_number("416-555-ABC1"))
return unittest.main()
if __name__ == "__main__":
test_phone_number_validation()
Example Implementation of the validate_phone_number
function
import re
def validate_phone_number(phone_number):
# Regular expression pattern for US phone number format
us_format_pattern = re.compile(r"^\(\d{3}\) \d{3}-\d{4}$")
# Regular expression pattern for international phone number format
int_format_pattern = re.compile(r"^(\+?\d{1,2})\s?\(?\d{3}\)?[\s.-]?\d{3}[\s.-]?\d{4}$")
# Remove non-numeric characters from the input phone number
cleaned_phone_number = re.sub(r"\D", "", phone_number)
# Check if the cleaned phone number matches the US or international format patterns
if us_format_pattern.match(phone_number):
return True
elif int_format_pattern.match(phone_number):
return True
else:
return False
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
ChatGPT 4.0, Claude 3.5, Gemini 1.5 Pro and Llama 3.1 all provided comprehensive test scenarios for phone number validation. ChatGPT and Claude offered the most structured approaches, with clear categorization of valid patterns, invalid patterns and edge cases. Gemini provided additional testing strategy insights and internationalization considerations. Llama included sample Python test code which was unique. Response times varied significantly - Llama was fastest at 3.6s while Gemini took 18.2s. Overall, Claude delivered the most concise yet complete response for this use case.
Budget Tier
Comparing model performance on phone number validation test scenarios:
ChatGPT 4o Mini and Gemini 1.5 Flash provided the most comprehensive test scenarios, with detailed tables and categorization. Claude 3.5 Haiku offered a well-structured but less detailed approach. Llama 3.1 8B uniquely included implementation code but had less diverse test cases.
Gemini was fastest (7.5s) and most organized with its tabular format. ChatGPT took longest (16.3s) but provided thorough coverage. Claude (11.5s) excelled at categorization. Llama was quickest (1.7s) but less comprehensive.
Overall, Gemini 1.5 Flash delivered the best balance of speed, organization and test coverage.
GCD Calculator Test Suite
Creates unit tests for GCD calculation, testing various number combinations, including prime numbers and edge cases
Prompt:
Write unit tests for a function that finds the greatest common divisor of two numbers
Performance Results
To write unit tests for a function that finds the greatest common divisor (GCD) of two numbers, we should first define the expected behavior of the function. The function should take two integers as arguments and return their GCD. We will write tests ensuring that the function handles different scenarios correctly, such as positive numbers, negative numbers, zero, and equal numbers.
Here's an example of how this might look in Python using the unittest
framework:
First, let's say we have a function gcd
defined in a module named math_utils
:
# math_utils.py
def gcd(a, b):
"""Returns the greatest common divisor of a and b using the Euclidean algorithm."""
while b:
a, b = b, a % b
return abs(a)
Now, we can write the unit tests:
# test_math_utils.py
import unittest
from math_utils import gcd
class TestGCD(unittest.TestCase):
def test_gcd_of_positive_numbers(self):
self.assertEqual(gcd(48, 18), 6)
self.assertEqual(gcd(100, 10), 10)
self.assertEqual(gcd(54, 24), 6)
def test_gcd_of_negative_numbers(self):
self.assertEqual(gcd(-48, -18), 6)
self.assertEqual(gcd(-100, 10), 10)
self.assertEqual(gcd(54, -24), 6)
def test_gcd_with_zero(self):
self.assertEqual(gcd(0, 5), 5)
self.assertEqual(gcd(0, -7), 7)
self.assertEqual(gcd(0, 0), 0) # Special case: GCD of 0 and 0 is usually defined as 0
def test_gcd_of_equal_numbers(self):
self.assertEqual(gcd(10, 10), 10)
self.assertEqual(gcd(-5, -5), 5)
self.assertEqual(gcd(0, 0), 0)
def test_gcd_of_coprime_numbers(self):
self.assertEqual(gcd(17, 13), 1)
self.assertEqual(gcd(31, 2), 1)
if __name__ == '__main__':
unittest.main()
Explanation:
- Test
gcd()
with positive numbers: Ensures the function correctly computes the GCD of positive integers. - Test
gcd()
with negative numbers: Validates that the function handles negative numbers by returning their GCD and considering absolute values. - Test
gcd()
with zero: Handles cases where one or both numbers are zero. Generally, the GCD of any number and zero is the absolute value of the other number; both zero produces zero. - Test
gcd()
with equal numbers: The GCD of two equal numbers should be the absolute value of the number itself. - Test
gcd()
of coprime numbers: Ensures that the function correctly identifies numbers with a GCD of 1.
To run the tests, you would execute the test file using a command like python -m unittest test_math_utils.py
.
import unittest
def test_gcd():
class TestGCD(unittest.TestCase):
def test_positive_numbers(self):
# Test with positive numbers
self.assertEqual(gcd(48, 18), 6)
self.assertEqual(gcd(54, 24), 6)
self.assertEqual(gcd(7, 13), 1) # Coprime numbers
def test_negative_numbers(self):
# Test with negative numbers
self.assertEqual(gcd(-48, 18), 6)
self.assertEqual(gcd(48, -18), 6)
self.assertEqual(gcd(-48, -18), 6)
def test_zero(self):
# Test with zero
self.assertEqual(gcd(0, 5), 5)
self.assertEqual(gcd(5, 0), 5)
self.assertEqual(gcd(0, 0), 0)
def test_same_number(self):
# Test with same numbers
self.assertEqual(gcd(5, 5), 5)
self.assertEqual(gcd(-5, -5), 5)
def test_one(self):
# Test with 1
self.assertEqual(gcd(1, 5), 1)
self.assertEqual(gcd(5, 1), 1)
def test_large_numbers(self):
# Test with large numbers
self.assertEqual(gcd(1000000, 500000), 500000)
self.assertEqual(gcd(123456, 654321), 3)
def test_non_integers(self):
# Test with non-integer inputs
with self.assertRaises(TypeError):
gcd(5.5, 2)
with self.assertRaises(TypeError):
gcd("5", 2)
if __name__ == '__main__':
unittest.main()
This test suite includes tests for:
- Basic positive number cases
- Negative numbers
- Zero as input
- Same number as both inputs
- One as input
- Large numbers
- Invalid inputs (non-integers)
Test cases verify that:
- The GCD function works correctly for standard positive integer inputs
- Sign doesn't affect the result (GCD is always positive)
- GCD with zero returns the other number
- GCD of a number with itself returns that number
- GCD with 1 returns 1
- Function handles large numbers correctly
- Function properly rejects non-integer inputs
Each test case includes assertions to verify the expected output matches the actual output.
You would need to implement the actual gcd()
function that these tests are checking against.
import unittest
import math
def gcd(a, b):
"""
Calculates the greatest common divisor (GCD) of two integers using the Euclidean algorithm.
Args:
a: The first integer.
b: The second integer.
Returns:
The greatest common divisor of a and b. Returns 0 if both a and b are 0.
Raises:
TypeError: If a or b are not integers.
"""
if not isinstance(a, int) or not isinstance(b, int):
raise TypeError("Inputs must be integers")
if a == 0 and b == 0:
return 0 # GCD(0, 0) is undefined, often treated as 0
a = abs(a)
b = abs(b)
while(b):
a, b = b, a % b
return a
class TestGCD(unittest.TestCase):
def test_positive_numbers(self):
self.assertEqual(gcd(48, 18), 6)
self.assertEqual(gcd(18, 48), 6) # Test commutativity
self.assertEqual(gcd(12, 18), 6)
self.assertEqual(gcd(100, 50), 50)
def test_negative_numbers(self):
self.assertEqual(gcd(-48, 18), 6)
self.assertEqual(gcd(48, -18), 6)
self.assertEqual(gcd(-48, -18), 6)
def test_zero(self):
self.assertEqual(gcd(0, 5), 5)
self.assertEqual(gcd(5, 0), 5)
self.assertEqual(gcd(0, 0), 0) # Special case: GCD(0, 0) is 0 by our definition.
def test_one(self): # Test cases with 1
self.assertEqual(gcd(1, 10), 1)
self.assertEqual(gcd(7, 1), 1)
def test_large_numbers(self): # Test with large numbers for efficiency check (indirectly)
self.assertEqual(gcd(123456789, 987654321), 9)
def test_prime_numbers(self):
self.assertEqual(gcd(17, 23), 1)
self.assertEqual(gcd(5,7),1)
def test_type_error(self):
with self.assertRaises(TypeError):
gcd(4.5, 6) # Test float input
with self.assertRaises(TypeError):
gcd(4, "6") # Test string input
if __name__ == '__main__':
unittest.main()
Key improvements in this version:
- Handles zero and negative inputs correctly: The
gcd
function now correctly handles cases where one or both inputs are zero or negative. The tests are updated accordingly. - More comprehensive tests: Added tests for a wider range of cases, including large prime numbers, numbers with known GCDs, and edge cases involving zero and one.
- Tests for error handling: Includes tests to verify that the function raises a
TypeError
when provided with non-integer inputs. - Clearer and more concise code: Improved comments and variable naming for better readability.
- Efficiency (indirectly tested): The
test_large_numbers
case, while not a direct performance test, helps to check against excessively slow algorithms.
This revised test suite provides much more robust validation of the gcd
function. It covers a broader range of scenarios and edge cases, which makes it more likely to catch potential bugs.
Greatest Common Divisor Unit Tests
Here's an example of how you can write unit tests for a function that calculates the greatest common divisor (GCD) of two numbers using Python and the unittest
framework:
import unittest
from gcd import gcd # Import the gcd function from the gcd module
class TestGCDFunction(unittest.TestCase):
def test_gcd_of_same_numbers(self):
"""
Test that the GCD of a number with itself is the number itself.
"""
self.assertEqual(gcd(5, 5), 5)
self.assertEqual(gcd(10, 10), 10)
def test_gcd_of_coprime_numbers(self):
"""
Test that the GCD of two coprime numbers is 1.
"""
self.assertEqual(gcd(5, 7), 1)
self.assertEqual(gcd(13, 29), 1)
def test_gcd_of_multiples(self):
"""
Test that the GCD of two multiples of a common factor is the common factor.
"""
self.assertEqual(gcd(10, 20), 10)
self.assertEqual(gcd(21, 7), 7)
def test_gcd_of_zero_and_nonzero(self):
"""
Test that the GCD of zero and a nonzero number is the nonzero number.
"""
self.assertEqual(gcd(0, 5), 5)
self.assertEqual(gcd(10, 0), 10)
def test_gcd_of_two_zeros(self):
"""
Test that the GCD of two zeros is undefined, so it should raise a ValueError.
"""
with self.assertRaises(ValueError):
gcd(0, 0)
def test_gcd_of_negative_numbers(self):
"""
Test that the GCD of two negative numbers is the same as the GCD of their absolute values.
"""
self.assertEqual(gcd(-5, -5), 5)
self.assertEqual(gcd(-10, 20), 10)
def test_gcd_of_floating_point_numbers(self):
"""
Test that the GCD of two floating-point numbers should raise a TypeError.
"""
with self.assertRaises(TypeError):
gcd(5.5, 7.2)
if __name__ == '__main__':
unittest.main()
Example Implementation of the gcd Function
Here's a simple implementation of the gcd
function using the Euclidean algorithm:
def gcd(a: int, b: int) -> int:
"""
Calculate the greatest common divisor (GCD) of two numbers.
Args:
a (int): The first number.
b (int): The second number.
Returns:
int: The GCD of a and b.
Raises:
ValueError: If both a and b are zero.
TypeError: If either a or b is a non-integer.
"""
if not isinstance(a, int) or not isinstance(b, int):
raise TypeError("Both a and b must be integers.")
if a == 0 and b == 0:
raise ValueError("GCD of 0 and 0 is undefined.")
return _gcd_recursive(a, b)
def _gcd_recursive(a: int, b: int) -> int:
"""
Recursive helper function to calculate the GCD of a and b.
"""
if b == 0:
return a
else:
return _gcd_recursive(b, a % b)
Note that this implementation assumes that the inputs are integers and raises a TypeError
for floating-point numbers and a ValueError
for two zeros.
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
Sure! Let's start with a simple function that finds the greatest common divisor (GCD) of two numbers using the Euclidean algorithm. Then, we'll write unit tests for that function. We'll use Python's built-in unittest
framework for the testing.
Here’s a sample implementation of the GCD function:
def gcd(a: int, b: int) -> int:
"""Returns the greatest common divisor of two integers."""
while b:
a, b = b, a % b
return abs(a) # Ensure the GCD is non-negative
Now, let's write some unit tests:
import unittest
class TestGCD(unittest.TestCase):
def test_positive_numbers(self):
self.assertEqual(gcd(48, 18), 6)
self.assertEqual(gcd(56, 98), 14)
self.assertEqual(gcd(100, 75), 25)
def test_negative_numbers(self):
self.assertEqual(gcd(-48, -18), 6)
self.assertEqual(gcd(-56, 98), 14)
self.assertEqual(gcd(100, -75), 25)
def test_zero(self):
self.assertEqual(gcd(0, 18), 18)
self.assertEqual(gcd(48, 0), 48)
self.assertEqual(gcd(0, 0), 0) # GCD(0, 0) is mathematically undefined, but returns 0 in our implementation.
def test_coprime_numbers(self):
self.assertEqual(gcd(17, 19), 1)
self.assertEqual(gcd(8, 9), 1)
def test_identical_numbers(self):
self.assertEqual(gcd(42, 42), 42)
self.assertEqual(gcd(-30, -30), 30)
def test_one_is_multiple_of_other(self):
self.assertEqual(gcd(10, 100), 10)
self.assertEqual(gcd(15, 45), 15)
if __name__ == '__main__':
unittest.main()
Explanation of the Test Cases:
- testpositivenumbers: Tests the GCD function with typical positive numbers.
- testnegativenumbers: Tests the function with negative numbers to ensure it returns the correct absolute value.
- test_zero: Tests cases where one or both of the input numbers are zero. The GCD of any number and zero should return the number itself, and the GCD(0,0) is defined as returning zero in our implementation.
- testcoprimenumbers: Verifies the GCD of numbers that are coprime (i.e., their GCD is 1).
- testidenticalnumbers: Checks the case where both numbers are the same.
- testoneismultipleof_other: Ensures the function correctly identifies GCD when one number is a multiple of the other.
To run the tests, you can simply execute the script, and it will run all the defined test cases.
The following unit tests cover various scenarios for a Greatest Common Divisor (GCD) function, using Python's unittest
module. We'll test with different input types and edge cases.
import unittest
def gcd(a, b):
"""
Calculates the greatest common divisor of two integers using Euclid's algorithm.
"""
while(b):
a, b = b, a % b
return a
class TestGCD(unittest.TestCase):
def test_positive_integers(self):
self.assertEqual(gcd(12, 18), 6)
self.assertEqual(gcd(48, 18), 6)
self.assertEqual(gcd(100, 50), 50)
self.assertEqual(gcd(17, 23), 1) #coprime
def test_zero(self):
self.assertEqual(gcd(0, 10), 10)
self.assertEqual(gcd(10, 0), 10)
self.assertEqual(gcd(0,0), 0) # special case for 0,0
def test_negative_integers(self):
self.assertEqual(gcd(-12, 18), 6)
self.assertEqual(gcd(12, -18), 6)
self.assertEqual(gcd(-12, -18), 6)
def test_large_numbers(self):
self.assertEqual(gcd(123456789, 987654321), 3)
def test_type_errors(self):
with self.assertRaises(TypeError):
gcd("12", 18) #String input
with self.assertRaises(TypeError):
gcd(12, "18") #String Input
with self.assertRaises(TypeError):
gcd(12.5, 18) #Float input
with self.assertRaises(TypeError):
gcd(12, 18.5) #Float Input
if __name__ == '__main__':
unittest.main()
To run these tests, save the code as a Python file (e.g., gcd_test.py
) and then run it from your terminal using: python gcd_test.py
The output will show you whether the tests passed or failed. This comprehensive set of tests ensures the gcd
function works correctly under various conditions, including edge cases and potential errors. Remember to adapt the gcd
function definition if you're using a different implementation (e.g., recursive).
Here's a set of comprehensive unit tests for a function that finds the greatest common divisor (GCD) using the Euclidean algorithm:
import unittest
def gcd(a, b):
"""
Calculate the greatest common divisor of two numbers using Euclidean algorithm.
Args:
a (int): First number
b (int): Second number
Returns:
int: Greatest common divisor of a and b
"""
# Ensure positive integers
a, b = abs(a), abs(b)
# Handle special cases
if a == 0:
return b
if b == 0:
return a
# Euclidean algorithm
while b:
a, b = b, a % b
return a
class TestGCD(unittest.TestCase):
def test_positive_numbers(self):
"""Test GCD with positive numbers"""
self.assertEqual(gcd(48, 18), 6)
self.assertEqual(gcd(54, 24), 6)
self.assertEqual(gcd(100, 75), 25)
def test_one_number_zero(self):
"""Test when one number is zero"""
self.assertEqual(gcd(0, 5), 5)
self.assertEqual(gcd(7, 0), 7)
self.assertEqual(gcd(0, 0), 0)
def test_one_number_one(self):
"""Test when one number is one"""
self.assertEqual(gcd(1, 5), 1)
self.assertEqual(gcd(7, 1), 1)
self.assertEqual(gcd(1, 1), 1)
def test_negative_numbers(self):
"""Test with negative numbers"""
self.assertEqual(gcd(-48, 18), 6)
self.assertEqual(gcd(48, -18), 6)
self.assertEqual(gcd(-48, -18), 6)
def test_coprime_numbers(self):
"""Test coprime (relatively prime) numbers"""
self.assertEqual(gcd(17, 23), 1)
self.assertEqual(gcd(13, 17), 1)
self.assertEqual(gcd(7, 11), 1)
def test_same_numbers(self):
"""Test when both numbers are the same"""
self.assertEqual(gcd(5, 5), 5)
self.assertEqual(gcd(7, 7), 7)
self.assertEqual(gcd(100, 100), 100)
def test_large_numbers(self):
"""Test with larger numbers"""
self.assertEqual(gcd(1071, 462), 21)
self.assertEqual(gcd(46337, 12345), 1)
def test_input_order_invariance(self):
"""Test that GCD is independent of input order"""
self.assertEqual(gcd(48, 18), gcd(18, 48))
self.assertEqual(gcd(100, 75), gcd(75, 100))
if __name__ == '__main__':
unittest.main()
These unit tests cover various scenarios:
- Positive Numbers: Checking GCD calculation for standard positive integers
- Zero Handling: Verifying behavior when one or both numbers are zero
- One as Input: Testing cases where one number is 1
- Negative Numbers: Ensuring correct GCD calculation with negative inputs
- Coprime Numbers: Checking calculation for numbers with no common factors
- Same Numbers: Verifying GCD when both inputs are identical
- Large Numbers: Testing with larger integer values
- Input Order Invariance: Confirming GCD is symmetric
The accompanying gcd()
function implements the Euclidean algorithm with additional handling for special cases like zero and negative numbers.
Key features of the implementation:
- Takes absolute value of inputs
- Handles zero inputs
- Uses efficient iterative Euclidean algorithm
- Works with positive and negative integers
To run the tests, simply execute the script. The unittest
framework will run all test cases and report any failures.
Here's an example of how you can write unit tests for a function that finds the greatest common divisor (GCD) of two numbers using Python and the unittest
framework:
# gcd.py
def gcd(a, b):
"""
Computes the greatest common divisor of a and b.
:param a: The first number.
:param b: The second number.
:return: The greatest common divisor of a and b.
"""
while b:
a, b = b, a % b
return a
# test_gcd.py
import unittest
from gcd import gcd
class TestGCD(unittest.TestCase):
def test_gcd_of_perfect_square(self):
"""
Test the GCD of two perfect squares.
"""
self.assertEqual(gcd(36, 24), 12)
def test_gcd_of_two_twos(self):
"""
Test the GCD of two numbers, both are 2.
"""
self.assertEqual(gcd(2, 2), 2)
def test_gcd_of_three_and_five(self):
"""
Test the GCD of 3 and 5.
"""
self.assertEqual(gcd(3, 5), 1)
def test_gcd_of_zero(self):
"""
Test the GCD of zero and any number.
"""
self.assertEqual(gcd(5, 0), 5)
def test_gcd_of_negatives(self):
"""
Test the GCD of negative numbers.
"""
self.assertEqual(gcd(-5, -3), 1)
def testfailure_if_non_integer_input(self):
"""
Error occurs due to incorrect gcd method value being passed
a test case of the behaviour to see pass it through
making sure the possible failure gets captured by checks
"""
with self.assertRaises(TypeError):
gcd('a', 5)
def testfailure_if_non_integer_input_for_b(self):
"""
Error occurs due to incorrect gcd method value being passed
a test case of the behaviour to see pass it through making sure the possible failure gets captured by checks
"""
with self.assertRaises(TypeError):
gcd(5, 'a')
def test_gcd_of_zero_and_zero(self):
"""
Test the GCD of zero and zero.
"""
self.assertEqual(gcd(0, 0), 0)
if __name__ == "__main__":
unittest.main()
These tests cover various scenarios such as: the GCD of perfect squares, numbers that are multiples of each other, numbers that don't share common factors, zero, negative numbers, and non-integer inputs which lead to possible failures in the function. The unittest
framework picks up the function for testing. The test failure for non-integer values has been included to show that normal error capturing operation.
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
The models demonstrated varying approaches to testing GCD functionality. ChatGPT 4 provided a clean, well-structured solution with clear test categories and helpful explanations. Claude Sonnet offered comprehensive test coverage, including large numbers and non-integer inputs. Gemini Pro included both implementation and tests with detailed docstrings and error handling. Llama 70B provided the most concise solution with good documentation and a separate recursive implementation.
ChatGPT 4 and Claude performed best overall, with thorough test cases and clear organization. Gemini's addition of implementation code was helpful but made the response longer. Llama's solution, while good, was more basic in comparison.
Budget Tier
Each model performed well but with distinct characteristics. Claude 3.5 Haiku provided the most comprehensive and well-documented test suite, with detailed docstrings and thorough test cases organized into logical groups. ChatGPT 4o Mini offered a solid implementation with good test coverage, though slightly less organized. Gemini 1.5 Flash delivered a concise solution with strong input validation, particularly for type errors. Llama 3.1 8B was the fastest (1068ms) but had less structured test cases. While all solutions were functional, Claude's stood out for completeness and clarity despite taking longest to generate (14251ms).
Leap Year Validator Tests
Develops test cases for leap year validation, covering century years, regular years, and edge cases
Prompt:
Generate test cases for a function that checks if a year is a leap year
Performance Results
To generate test cases for a function that checks if a year is a leap year, it's important to understand the rules for identifying a leap year:
- A year is a leap year if it is divisible by 4.
- However, if the year is divisible by 100, it is not a leap year, unless:
- The year is also divisible by 400, in which case, it is a leap year.
Based on these rules, here are some test cases:
Valid Test Cases
- Test Case: Year is divisible by 4 but not by 100 (Leap Year)
- Input: 2024
- Expected Output: True
- Test Case: Year is not divisible by 4 (Common Year)
- Input: 2019
- Expected Output: False
- Test Case: Year is divisible by 100 but not by 400 (Common Year)
- Input: 1900
- Expected Output: False
- Test Case: Year is divisible by 400 (Leap Year)
- Input: 2000
- Expected Output: True
- Test Case: Year is 0
- Input: 0 (Edge case)
- Expected Output: True
Edge Cases
- Test Case: Negative Year (Assuming historical calendar years work similarly)
- Input: -4
- Expected Output: True
- Test Case: Year is 1 (First positive year, Common Year)
- Input: 1
- Expected Output: False
- Test Case: Minimum Possible Year (System Limit Case)
- Input: -2147483648 (If considering system's integer limit)
- Expected Output: Variable based on user/system specifications; likely False
- Test Case: Maximum Possible Year (System Limit Case)
- Input: 2147483647 (If considering system's integer limit)
- Expected Output: False
Large Numbers
Test Case: Large Year divisible by 4 but not by 100 or 400
- Input: 4000004
- Expected Output: True
Test Case: Large Year divisible by 100 and 400
- Input: 4000400
- Expected Output: True
Test Case: Large Year divisible by 100 but not 400
- Input: 4000100
- Expected Output: False
In creating these test cases, ensure the function handles both typical and edge scenarios effectively, and that it operates correctly for both recent and distant years.
Test Cases for Leap Year Function
Valid Leap Years
test_valid_leap_years = [
(2000, True), # Divisible by 400
(2004, True), # Divisible by 4
(2400, True), # Future leap year
(1600, True), # Historical leap year
(2096, True) # Last leap year of century
]
Non-Leap Years
test_non_leap_years = [
(2001, False), # Regular non-leap year
(2100, False), # Century year not divisible by 400
(1900, False), # Historical century non-leap year
(2003, False), # Not divisible by 4
(2200, False) # Future century non-leap year
]
Edge Cases
test_edge_cases = [
(0, False), # Year zero
(-4, False), # Negative year
(10000, False), # Very large year
(1, False), # First year
(99, False) # Two-digit year
]
Invalid Input Cases
test_invalid_inputs = [
("2020", TypeError), # String input
(3.14, TypeError), # Float input
(None, TypeError), # None input
(True, TypeError), # Boolean input
({}, TypeError) # Invalid type
]
Test Case Explanations
- Valid leap years must be:
- Divisible by 4
- If century year (ending in 00), must be divisible by 400
- Non-leap years are:
- Not divisible by 4
- Century years not divisible by 400
- Edge cases check for:
- Boundary values
- Extreme values
- Special cases
- Invalid inputs test for:
- Different data types
- Null values
- Inappropriate inputs
import unittest
def is_leap_year(year):
"""
Checks if a given year is a leap year.
"""
if year % 4 != 0:
return False
elif year % 100 == 0:
if year % 400 == 0:
return True
else:
return False
else:
return True
class TestLeapYear(unittest.TestCase):
def test_divisible_by_4_but_not_100(self):
self.assertTrue(is_leap_year(2024))
self.assertTrue(is_leap_year(1996))
def test_divisible_by_4_and_100_but_not_400(self):
self.assertFalse(is_leap_year(1900))
self.assertFalse(is_leap_year(1800))
self.assertFalse(is_leap_year(2100))
def test_divisible_by_4_and_100_and_400(self):
self.assertTrue(is_leap_year(2000))
self.assertTrue(is_leap_year(1600))
def test_not_divisible_by_4(self):
self.assertFalse(is_leap_year(2023))
self.assertFalse(is_leap_year(1999))
def test_zero_year(self): # Edge case
self.assertTrue(is_leap_year(0)) # In the Gregorian calendar, year 0 is considered a leap year
def test_negative_year(self): # Edge case
self.assertTrue(is_leap_year(-4)) # Gregorian rules extend backwards
self.assertFalse(is_leap_year(-100))
self.assertTrue(is_leap_year(-400))
def test_large_year(self): # Edge case
self.assertTrue(is_leap_year(3000000)) # Checking for potential overflow issues (though practically irrelevant)
if __name__ == '__main__':
unittest.main()
Explanation of Test Cases:
- Standard Leap Years: Test years divisible by 4 but not by 100.
- Century Years (Not Leap): Test years divisible by 4 and 100 but not by 400.
- Century Years (Leap): Test years divisible by 4, 100, and 400.
- Non-Leap Years: Test years not divisible by 4.
- Edge Cases:
- Year 0: Test the specific case of year 0 (which is a leap year).
- Negative Years: Check if the leap year rules are applied correctly to negative years. (They are intended to be.)
- Large Year: Tests for potential integer overflow issues with very large year inputs (although this is practically irrelevant).
This comprehensive set of test cases ensures good coverage for the is_leap_year
function, helping to catch potential bugs or logic errors. It's a good practice to have tests that cover both normal scenarios and edge cases. The unittest
framework provides a structured way to write and run these tests.
## Test Cases for Leap Year Function
Test Case 1: Normal Year
- Input:
year = 2001
- Expected Output:
False
Test Case 2: Leap Year (Divisible by 4)
- Input:
year = 2004
- Expected Output:
True
Test Case 3: Leap Year (Divisible by 400)
- Input:
year = 2000
- Expected Output:
True
Test Case 4: Not a Leap Year (Divisible by 100 but not 400)
- Input:
year = 1900
- Expected Output:
False
Test Case 5: Edge Case (Year less than 1)
- Input:
year = 0
- Expected Output:
False
(or raise aValueError
)
Test Case 6: Edge Case (Non-integer year)
- Input:
year = 2001.5
- Expected Output:
False
(or raise aValueError
)
Test Case 7: Boundary Case (Year 1)
- Input:
year = 1
- Expected Output:
False
Example Use Case in Python
def is_leap_year(year):
"""
Checks if a given year is a leap year.
Args:
year (int): The year to check.
Returns:
bool: True if the year is a leap year, False otherwise.
Raises:
ValueError: If the input year is not an integer or is less than 1.
"""
if not isinstance(year, int) or year < 1:
raise ValueError("Invalid year")
return year % 4 == 0 and (year % 100 != 0 or year % 400 == 0)
# Test cases
test_years = [
(2001, False),
(2004, True),
(2000, True),
(1900, False),
(0, False),
(2001.5, None), # Raise ValueError
(1, False)
]
for year, expected_output in test_years:
try:
output = is_leap_year(year)
assert output == expected_output
print(f"Year {year}: {output} (Expected: {expected_output})")
except ValueError as e:
print(f"Error for year {year}: {e}")
This Python code defines a function is_leap_year
that takes an integer year as input and returns a boolean indicating whether the year is a leap year. The function uses the standard rules for leap years: a year is a leap year if it is divisible by 4, but not by 100, unless it is also divisible by 400. The code also includes test cases for various inputs, including edge cases and boundary cases.
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
Sure! Below are various test cases for a function that determines if a year is a leap year. The criteria for determining a leap year are:
- A year is a leap year if it is divisible by 4.
- However, if the year is divisible by 100, it is not a leap year, unless:
- The year is also divisible by 400, then it is a leap year.
Based on those rules, here are the test cases:
Test Cases
- Basic Leap Year
- Input: 2020
- Expected Output:
True
- Reason: 2020 is divisible by 4 and not divisible by 100.
- Basic Non-Leap Year
- Input: 2019
- Expected Output:
False
- Reason: 2019 is not divisible by 4.
- Century Year that is Not a Leap Year
- Input: 1900
- Expected Output:
False
- Reason: 1900 is divisible by 100 but not by 400.
- Century Year that is a Leap Year
- Input: 2000
- Expected Output:
True
- Reason: 2000 is divisible by both 100 and 400.
- Leap Year
- Input: 1600
- Expected Output:
True
- Reason: 1600 is divisible by 400.
- Non-Leap Year
- Input: 1700
- Expected Output:
False
- Reason: 1700 is divisible by 100 but not by 400.
- Random Year
- Input: 2023
- Expected Output:
False
- Reason: 2023 is not divisible by 4.
- Negative Year (Leap Year)
- Input: -40
- Expected Output:
True
- Reason: The year -40 (AD 40) is divisible by 4.
- Negative Year (Non-Leap Year)
- Input: -1
- Expected Output:
False
- Reason: The year -1 is not divisible by 4.
- Year Zero (Leap Year)
- Input: 0
- Expected Output:
True
- Reason: Year 0 is divisible by 4 (sometimes year 0 is considered in certain calendars).
Summary of Test Cases
- Positive cases: 2020, 2000, 1600, -40, 0
- Negative cases: 2019, 1900, 1700, 2023, -1
You can use these test cases to verify the correctness of your leap year function. Each of these cases covers the different rules of leap year determination.
The following test cases cover various scenarios for a leap year function. They're categorized for clarity and include expected outputs. Remember that a leap year is divisible by 4, but not by 100 unless it's also divisible by 400.
Test Cases:
| Test Case ID | Year | Expected Result | Rationale |
|--------------|------|-----------------|-------------------------------------------|
| 1 | 2024 | True | Divisible by 4, not divisible by 100 |
| 2 | 2000 | True | Divisible by 4, 100, and 400 |
| 3 | 1900 | False | Divisible by 4 and 100, but not 400 |
| 4 | 2023 | False | Not divisible by 4 |
| 5 | 2100 | False | Divisible by 4 and 100, but not 400 |
| 6 | 2400 | True | Divisible by 4, 100, and 400 |
| 7 | 1700 | False | Divisible by 4 and 100, but not 400 |
| 8 | 1600 | True | Divisible by 4, 100, and 400 |
| 9 | 0 | False | Year 0 is not a valid year (handle as needed) |
| 10 | -1 | False | Negative years are typically not handled as leap years (handle as needed) |
| 11 | 1 | False | Not divisible by 4 |
| 12 | 1582 | False | Before the Gregorian calendar reform (handle as needed)|
| 13 | 1583 | False | Just after the Gregorian calendar reform (handle this carefully) |
Edge Cases (Consider these depending on your requirements):
- Years before the Gregorian Calendar (1582): How should your function handle years before the Gregorian calendar reform? The Julian calendar had a different leap year rule. You might choose to return an error or simply treat them as non-leap years.
- Year 0: The year 0 doesn't exist in the Gregorian calendar. How should your function handle this input?
- Negative Years: Should your function handle negative years? If so, how?
- Non-integer input: How should your function handle input that's not an integer (e.g., a string, a float)? It should probably raise an error or return
False
.
These test cases provide a comprehensive set for validating your leap year function. Remember to choose an appropriate testing framework (like pytest or unittest in Python) to automate these tests.
Here are comprehensive test cases for a leap year function:
# Leap Year Function Test Cases
## Positive Test Cases
1. **Standard Leap Year**
- Input: 2020
- Expected Output: true
- Reasoning: Divisible by 4 and not a century year
2. **Century Leap Year**
- Input: 2000
- Expected Output: true
- Reasoning: Divisible by 400
3. **Minimum Leap Year**
- Input: 4
- Expected Output: true
- Reasoning: First leap year in the sequence
## Negative Test Cases
1. **Non-Leap Century Year**
- Input: 1900
- Expected Output: false
- Reasoning: Divisible by 100 but not by 400
2. **Standard Non-Leap Year**
- Input: 2021
- Expected Output: false
- Reasoning: Not divisible by 4
3. **Boundary Non-Leap Year**
- Input: 2100
- Expected Output: false
- Reasoning: Century year not divisible by 400
## Edge Cases
1. **Zero Year**
- Input: 0
- Expected Output: false
- Reasoning: Special handling for zero
2. **Negative Year**
- Input: -4
- Expected Output: false
- Reasoning: Negative years should be handled
3. **Very Large Leap Year**
- Input: 8000
- Expected Output: true
- Reasoning: Large leap year divisible by 400
These test cases cover various scenarios to thoroughly validate a leap year function.
Test Cases for Leap Year Function
Overview
The following test cases cover various scenarios for the is_leap_year
function, which takes an integer representing a year as input and returns a boolean value indicating whether the year is a leap year.
Test Cases
import unittest
def is_leap_year(year):
"""
Checks if a year is a leap year.
Args:
year (int): The year to check.
Returns:
bool: True if the year is a leap year, False otherwise.
"""
if year % 4 == 0:
if year % 100 == 0:
if year % 400 == 0:
return True
else:
return False
else:
return True
else:
return False
class TestIsLeapYear(unittest.TestCase):
def test_divisible_by_4(self):
self.assertTrue(is_leap_year(2020))
def test_not_divisible_by_100(self):
self.assertTrue(is_leap_year(2019))
def test_divisible_by_100_but_not_400(self):
self.assertFalse(is_leap_year(1900))
def test_divisible_by_400(self):
self.assertTrue(is_leap_year(2000))
def test_non_leap_year(self):
self.assertFalse(is_leap_year(2018))
def test_zero(self):
self.assertFalse(is_leap_year(0))
def test_negative(self):
self.assertFalse(is_leap_year(-2022))
def test_edge_case(self):
self.assertTrue(is_leap_year(1904))
if __name__ == '__main__':
unittest.main()
Explanation
- We define the
is_leap_year
function, which follows the standard Gregorian calendar leap year rule. - We create a test class
TestIsLeapYear
that extendsunittest.TestCase
. - Each test case covers a different scenario:
test_divisible_by_4
: Checks a year that is divisible by 4.test_not_divisible_by_100
: Checks a year that is not divisible by 100.test_divisible_by_100_but_not_400
: Checks a year that is divisible by 100 but not by 400.test_divisible_by_400
: Checks a year that is divisible by 400.test_non_leap_year
: Checks a non-leap year.test_zero
: Checks that 0 is not a leap year.test_negative
: Checks that a negative year is not a leap year.test_edge_case
: Checks a year that is close to the edge case of a leap year (in this case, 1904).
- The
unittest.main()
call runs all the test cases and displays the results.
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
Each model provided comprehensive test cases for the leap year function, but with distinct approaches. ChatGPT 4 offered detailed explanations and well-organized categories, focusing on valid cases and edge scenarios. Claude presented structured test cases in Python format with clear groupings and robust invalid input handling. Gemini delivered a complete unittest implementation with actual code and extensive comments. Llama provided a more concise solution with markdown formatting and included a practical implementation. Claude was fastest at 9.4s, while Gemini took longest at 17s. Overall, Claude's response was most organized and practical for implementation.
Budget Tier
ChatGPT 4o Mini provided thorough test cases with clear reasoning and covered edge cases like negative years. Gemini 1.5 Flash offered a well-organized table format and included historical considerations regarding the Gregorian calendar. Claude 3.5 Haiku delivered concise, categorized test cases in markdown format. Llama 3.1 8B uniquely provided a complete Python implementation with unittest framework. Despite being fastest (1150ms), Llama's response was more implementation-focused than test case-oriented. ChatGPT was slowest (9443ms) but most comprehensive in test case descriptions.
Duplicate Removal Test Suite
Creates test cases for array duplicate removal, testing various data types, array sizes, and edge cases
Prompt:
Create test cases for a function that removes duplicates from an array
Example
Performance Results
When creating test cases for a function that removes duplicates from an array, it's important to cover various scenarios to ensure that the function works correctly for different types of input. Below are some test cases based on the given arrays:
- Test Case 1: Array with Duplicate Strings
- Input:
["apple", "orange", "apple", "banana", "orange", "kiwi"]
- Expected Output:
["apple", "orange", "banana", "kiwi"]
- Description: This test checks if the function can remove duplicate strings from an array while maintaining the order of first occurrence.
- Test Case 2: Array with Duplicate Integers
- Input:
[1, 4, 2, 4, 1, 7, 2, 9, 9]
- Expected Output:
[1, 4, 2, 7, 9]
- Description: This test examines how the function handles an array of integers with duplicates and ensures the first occurrence is kept.
- Test Case 3: Array with Duplicate Booleans
- Input:
[true, false, true, false, true]
- Expected Output:
[true, false]
- Description: This test verifies that the function correctly processes a boolean array by removing duplicates.
- Test Case 4: Empty Array
- Input:
[]
- Expected Output:
[]
- Description: Tests function behavior with an empty array, expecting it to return an empty array as there are no elements to remove.
- Test Case 5: Array with All Unique Elements
- Input:
["grape", "mango", "pear"]
- Expected Output:
["grape", "mango", "pear"]
- Description: Tests the function's handling of an array where all elements are unique, expecting the same array as output.
- Test Case 6: Array with All Identical Elements
- Input:
[5, 5, 5, 5, 5]
- Expected Output:
[5]
- Description: Evaluates the function's handling of an array with identical elements, confirming it reduces it to a single instance of that element.
- Test Case 7: Large Array
- Input:
[i % 10 for i in range(1000)]
(Python list comprehension creating duplicates) - Expected Output:
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
- Description: Tests performance and correctness of the function on a large array with repeated elements.
- Test Case 8: Array with Mixed Data Types
- Input:
[1, "1", true, "true", 1, "1"]
- Expected Output:
[1, "1", true, "true"]
- Description: Tests how the function handles an array with mixed data types, expecting the removal of duplicates based on exact matches in type and value.
These test cases should cover a broad range of scenarios, ensuring that the function is robust and correctly removes duplicates from arrays of various types and configurations.
Test Cases for Remove Duplicates Function
Test Case 1: String Array
test('should remove duplicate strings from array', () => {
const input = ["apple", "orange", "apple", "banana", "orange", "kiwi"];
const expected = ["apple", "orange", "banana", "kiwi"];
expect(removeDuplicates(input)).toEqual(expected);
});
Test Case 2: Number Array
test('should remove duplicate numbers from array', () => {
const input = [1, 4, 2, 4, 1, 7, 2, 9, 9];
const expected = [1, 4, 2, 7, 9];
expect(removeDuplicates(input)).toEqual(expected);
});
Test Case 3: Boolean Array
test('should remove duplicate booleans from array', () => {
const input = [true, false, true, false, true];
const expected = [true, false];
expect(removeDuplicates(input)).toEqual(expected);
});
Additional Test Cases
test('should handle empty array', () => {
const input = [];
const expected = [];
expect(removeDuplicates(input)).toEqual(expected);
});
test('should handle array with no duplicates', () => {
const input = [1, 2, 3, 4, 5];
const expected = [1, 2, 3, 4, 5];
expect(removeDuplicates(input)).toEqual(expected);
});
test('should handle array with all same elements', () => {
const input = [2, 2, 2, 2, 2];
const expected = [2];
expect(removeDuplicates(input)).toEqual(expected);
});
test('should preserve original array order', () => {
const input = ["cat", "dog", "cat", "bird", "dog"];
const expected = ["cat", "dog", "bird"];
expect(removeDuplicates(input)).toEqual(expected);
});
Edge Cases
test('should handle array with mixed data types', () => {
const input = [1, "1", true, "true", {}, {}, null, null];
const expected = [1, "1", true, "true", {}, null];
expect(removeDuplicates(input)).toEqual(expected);
});
test('should handle array with undefined values', () => {
const input = [undefined, null, undefined, null];
const expected = [undefined, null];
expect(removeDuplicates(input)).toEqual(expected);
});
import unittest
def remove_duplicates(arr):
"""Removes duplicate elements from an array while preserving order."""
seen = set()
result = []
for item in arr:
if item not in seen:
seen.add(item)
result.append(item)
return result
class TestRemoveDuplicates(unittest.TestCase):
def test_string_array(self):
arr = ["apple", "orange", "apple", "banana", "orange", "kiwi"]
expected = ["apple", "orange", "banana", "kiwi"]
self.assertEqual(remove_duplicates(arr), expected)
def test_integer_array(self):
arr = [1, 4, 2, 4, 1, 7, 2, 9, 9]
expected = [1, 4, 2, 7, 9]
self.assertEqual(remove_duplicates(arr), expected)
def test_boolean_array(self):
arr = [True, False, True, False, True]
expected = [True, False]
self.assertEqual(remove_duplicates(arr), expected)
def test_empty_array(self):
arr = []
expected = []
self.assertEqual(remove_duplicates(arr), expected)
def test_array_with_none(self):
arr = [1, None, 2, None, 3, 1]
expected = [1, None, 2, 3]
self.assertEqual(remove_duplicates(arr), expected)
def test_array_with_mixed_types(self): # Important edge case
arr = [1, "1", 1.0, True, "True", 1] # Demonstrates distinctness.
expected = [1, "1", 1.0, True, "True"]
self.assertEqual(remove_duplicates(arr), expected)
if __name__ == '__main__':
unittest.main()
Key improvements in these test cases:
- Uses
unittest
framework: Provides a structured and standard way to write tests. - Tests different data types: Strings, integers, booleans, and mixed types are all covered. This ensures the function works correctly with various inputs.
- Edge cases included: Empty array, array with
None
values, and importantly, an array with mixed types (including subtle differences like1
,"1"
, and1.0
, which should not be treated as duplicates of each other) are tested to catch potential issues. - Clearer assertions: Uses
self.assertEqual()
for easy comparison of expected and actual results. - Good naming conventions: Test method names are descriptive (e.g.,
test_string_array
).
This improved set of tests provides better coverage and helps ensure the remove_duplicates
function is robust and reliable. It addresses the potential ambiguity about whether 1
, "1"
, and 1.0
should be considered the same – in most cases, distinct types should be treated separately, which these tests now confirm.
Test Cases for Duplicate Removal Function
Here are some test cases to ensure the function works as expected:
Test Case 1: Remove String Duplicates
- Input:
["apple", "orange", "apple", "banana", "orange", "kiwi"]
- Expected Output:
["apple", "orange", "banana", "kiwi"]
- Description: This test case checks if the function correctly removes duplicate strings from the input array.
Test Case 2: Remove Number Duplicates
- Input:
[1, 4, 2, 4, 1, 7, 2, 9, 9]
- Expected Output:
[1, 4, 2, 7, 9]
- Description: This test case checks if the function correctly removes duplicate numbers from the input array.
Test Case 3: Remove Boolean Duplicates
- Input:
[true, false, true, false, true]
- Expected Output:
[true, false]
- Description: This test case checks if the function correctly removes duplicate boolean values from the input array.
Test Case 4: Empty Array
- Input:
[]
- Expected Output:
[]
- Description: This test case checks if the function correctly handles an empty input array.
Test Case 5: Array with Single Element
- Input:
["apple"]
- Expected Output:
["apple"]
- Description: This test case checks if the function correctly handles an input array with a single element.
Test Case 6: Array with No Duplicates
- Input:
["apple", "orange", "banana", "kiwi"]
- Expected Output:
["apple", "orange", "banana", "kiwi"]
- Description: This test case checks if the function correctly handles an input array with no duplicates.
Test Case 7: Array with All Duplicates
- Input:
[1, 1, 1, 1, 1]
- Expected Output:
[1]
- Description: This test case checks if the function correctly handles an input array with all duplicates.
These test cases should provide adequate coverage to ensure the function works as expected.
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
Creating test cases for a function that removes duplicates from an array involves considering various scenarios, including typical cases, edge cases, and special conditions. Below are some test cases based on the input arrays you provided:
Test Cases for Removing Duplicates Function
- Basic Test Case - Mixed String Values
- Input:
["apple", "orange", "apple", "banana", "orange", "kiwi"]
- Expected Output:
["apple", "orange", "banana", "kiwi"]
- Description: Tests removal of duplicates in a typical case with string values.
- Basic Test Case - Mixed Integer Values
- Input:
[1, 4, 2, 4, 1, 7, 2, 9, 9]
- Expected Output:
[1, 4, 2, 7, 9]
- Description: Tests removal of duplicates in a typical case with integer values.
- Basic Test Case - Boolean Values
- Input:
[true, false, true, false, true]
- Expected Output:
[true, false]
- Description: Tests removal of duplicates for boolean values.
- Edge Case - Empty Array
- Input:
[]
- Expected Output:
[]
- Description: Ensure the function correctly handles an empty array.
- Edge Case - Single Element Array
- Input:
["apple"]
- Expected Output:
["apple"]
- Description: Case with a single element should return that element.
- Edge Case - All Duplicates
- Input:
[2, 2, 2, 2]
- Expected Output:
[2]
- Description: Should reduce an array of identical elements to a single element.
- Edge Case - Non-Duplicate Values
- Input:
[3, 5, 1, 2]
- Expected Output:
[3, 5, 1, 2]
- Description: Ensure that an array with no duplicates remains unchanged.
- Complex Case - Special Characters and Mixed Types
- Input:
["!@#", "abc", "!@#", "123", "abc"]
- Expected Output: ["!@#", "abc", "123"]
- Description: Tests removal of duplicates when special characters and mixed string types are present.
- Edge Case - Large Input Size
- Input:
["a"] * 10**6 + ["b"] * 10**6 + ["c"]
- Expected Output:
["a", "b", "c"]
- Description: Test function performance and correctness with a large input size.
- Mixed Case Sensitivity
- Input:
["apple", "Apple", "APPLE", "banana"]
- Expected Output:
["apple", "Apple", "APPLE", "banana"]
- Description: Tests if the function treats differently cased strings as distinct.
- Input:
Summary
These test cases cover a variety of scenarios, ensuring that the function for removing duplicates handles typical values, special cases, and retains expected behavior across different data types and conditions.
The test cases should cover various scenarios to ensure the function correctly handles duplicates in different data types and edge cases. Here's a comprehensive set of test cases, focusing on clarity and organization:
import unittest
def remove_duplicates(input_array):
"""Removes duplicate elements from an array while preserving order."""
seen = set()
result = []
for item in input_array:
if item not in seen:
seen.add(item)
result.append(item)
return result
class TestRemoveDuplicates(unittest.TestCase):
def test_string_array(self):
self.assertEqual(remove_duplicates(["apple", "orange", "apple", "banana", "orange", "kiwi"]), ["apple", "orange", "banana", "kiwi"])
def test_integer_array(self):
self.assertEqual(remove_duplicates([1, 4, 2, 4, 1, 7, 2, 9, 9]), [1, 4, 2, 7, 9])
def test_boolean_array(self):
self.assertEqual(remove_duplicates([True, False, True, False, True]), [True, False])
def test_empty_array(self):
self.assertEqual(remove_duplicates([]), [])
def test_all_duplicates(self):
self.assertEqual(remove_duplicates(["apple", "apple", "apple"]), ["apple"])
def test_all_unique(self):
self.assertEqual(remove_duplicates(["apple", "banana", "kiwi"]), ["apple", "banana", "kiwi"])
def test_mixed_types_error(self): # This will only pass if the function handles mixed types appropriately (or throws an error as intended)
with self.assertRaises(TypeError): #expecting error, not a specific output
remove_duplicates([1, "apple", True])
def test_large_array(self): #test performance with a larger array
large_array = list(range(1000)) + list(range(500))
self.assertEqual(len(remove_duplicates(large_array)), 1000)
if __name__ == '__main__':
unittest.main()
Explanation of Test Cases:
test_string_array
,test_integer_array
,test_boolean_array
: These cover the example arrays provided, demonstrating correct functionality with different data types.test_empty_array
: Checks the function's behavior with an empty input array. This is a crucial edge case.test_all_duplicates
: Verifies that the function correctly handles an array containing only duplicate elements.test_all_unique
: Ensures that the function doesn't modify an array with all unique elements.test_mixed_types_error
: This tests how the function handles arrays with mixed data types. The currentremove_duplicates
function will likely throw a TypeError if you try to compare integers and strings usingin
. You might choose to adjust your function to handle this differently, or keep the error check as is.test_large_array
: This is a performance test, although not strictly a correctness test, to make sure the solution doesn't have unexpected performance issues with large input.
This comprehensive suite provides a robust test coverage for your remove_duplicates
function. Remember to run these tests using a testing framework like unittest
(shown above) to automate the process and get immediate feedback.
Here are comprehensive test cases for a function that removes duplicates from an array:
Test Suite: Remove Duplicates Function
Test Case 1: String Array with Duplicates
describe('Remove Duplicates - String Array', () => {
const input = ["apple", "orange", "apple", "banana", "orange", "kiwi"];
const expected = ["apple", "orange", "banana", "kiwi"];
it('should remove duplicate strings', () => {
const result = removeDuplicates(input);
expect(result).toEqual(expected);
});
it('should preserve original order of first occurrence', () => {
const result = removeDuplicates(input);
expect(result[0]).toBe("apple");
expect(result[1]).toBe("orange");
});
it('should return an array with the same length as unique elements', () => {
const result = removeDuplicates(input);
expect(result.length).toBe(4);
});
});
Test Case 2: Numeric Array with Duplicates
describe('Remove Duplicates - Numeric Array', () => {
const input = [1, 4, 2, 4, 1, 7, 2, 9, 9];
const expected = [1, 4, 2, 7, 9];
it('should remove duplicate numbers', () => {
const result = removeDuplicates(input);
expect(result).toEqual(expected);
});
it('should preserve first occurrence of each number', () => {
const result = removeDuplicates(input);
expect(result[0]).toBe(1);
expect(result[1]).toBe(4);
});
it('should handle numeric duplicates correctly', () => {
const result = removeDuplicates(input);
expect(result.length).toBe(5);
});
});
Test Case 3: Boolean Array with Duplicates
describe('Remove Duplicates - Boolean Array', () => {
const input = [true, false, true, false, true];
const expected = [true, false];
it('should remove duplicate boolean values', () => {
const result = removeDuplicates(input);
expect(result).toEqual(expected);
});
it('should preserve first occurrence of each boolean', () => {
const result = removeDuplicates(input);
expect(result[0]).toBe(true);
expect(result[1]).toBe(false);
});
it('should return correct number of unique boolean values', () => {
const result = removeDuplicates(input);
expect(result.length).toBe(2);
});
});
Edge Case Tests
describe('Remove Duplicates - Edge Cases', () => {
it('should return empty array for empty input', () => {
const result = removeDuplicates([]);
expect(result).toEqual([]);
});
it('should handle array with no duplicates', () => {
const input = [1, 2, 3, 4, 5];
const result = removeDuplicates(input);
expect(result).toEqual(input);
});
it('should work with all duplicate elements', () => {
const input = [5, 5, 5, 5, 5];
const result = removeDuplicates(input);
expect(result).toEqual([5]);
});
});
Test Coverage Notes:
- Covers string, numeric, and boolean arrays
- Verifies preservation of original order
- Checks correct number of unique elements
- Includes edge cases like empty array and all duplicates
- Ensures first occurrence of each element is retained
Recommended Implementation Hints:
- Use
Set
orfilter()
withindexOf()
- Maintain original array order
- Handle different data types
These test cases provide comprehensive coverage for a function that removes duplicates while preserving the original order of elements.
Test Cases for Removing Duplicates from Array
describe('removeDuplicates', () => {
it('should remove duplicates from an array of strings', () => {
const input = ["apple", "orange", "apple", "banana", "orange", "kiwi"];
const expected = ["apple", "orange", "banana", "kiwi"];
expect(removeDuplicates(input)).toEqual(expected);
});
it('should remove duplicates from an array of integers', () => {
const input = [1, 4, 2, 4, 1, 7, 2, 9, 9];
const expected = [1, 2, 4, 7, 9];
expect(removeDuplicates(input)).toEqual(expected);
});
it('should remove duplicates from an array of booleans', () => {
const input = [true, false, true, false, true];
const expected = [true, false];
expect(removeDuplicates(input)).toEqual(expected);
});
it('should throw an error if input is not an array', () => {
expect(() => removeDuplicates(1)).toThrowError('Input must be an array');
});
it('should throw an error if input array is empty', () => {
expect(() => removeDuplicates([])).toThrowError('Input array cannot be empty');
});
});
// Implementing the removeDuplicates function
function removeDuplicates(arr) {
if (!Array.isArray(arr)) {
throw new Error('Input must be an array');
}
if (arr.length === 0) {
throw new Error('Input array cannot be empty');
}
const uniqueArr = [...new Set(arr)];
return uniqueArr.reverse();
}
Explanation:
In this example, we create test cases for a function called removeDuplicates
using Jest testing library. We test its behavior on different types of inputs (arrays of strings, integers, and booleans), and also edge cases (empty array and non-array input).
The removeDuplicates
function uses the Set object to remove duplicates, and then reverses the resulting array to maintain the original order.
If the input is not an array or is an empty array, the function throws a custom error with a clear message.
Note that the removeDuplicates
function modifies the original array by creating a new array with unique elements. If you prefer to keep the original array unchanged, you can use a different implementation, such as using for...of
loop or Array.prototype.filter()
method.
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
ChatGPT 4.0 and Claude 3.5 provided the most comprehensive test cases, with ChatGPT offering detailed descriptions and Claude presenting practical code implementations. Gemini 1.5 Pro uniquely included a complete working implementation with unittest framework, though perhaps excessive for the task. Llama 3.1 delivered a concise but adequate set of test cases with clear formatting. Performance-wise, Llama was fastest (2.2s), while ChatGPT was slowest (17.7s). Claude struck the best balance between thoroughness and implementation focus, making it arguably the most useful response for this specific task.
Budget Tier
Comparing AI model performance on generating test cases for array duplicate removal:
ChatGPT 4o Mini provided comprehensive test cases with clear descriptions but took longest (13s). Gemini 1.5 Flash delivered detailed Python unittest implementation in half the time (6.3s). Claude 3.5 Haiku produced well-structured JavaScript tests with thorough edge cases (12.7s). Llama 3.1 8B generated the most concise solution with working code in just 1s, though less comprehensive.
Gemini offered best balance of completeness and speed. Llama excelled at quick basic implementation. ChatGPT and Claude provided more thorough coverage but required more processing time.
Compound Interest Calculator Tests
Develops unit tests for compound interest calculations, testing different principal amounts, rates, and time periods
Prompt:
Write unit tests for a function that calculates compound interest
Performance Results
Certainly! To write unit tests for a function that calculates compound interest, we first need to establish the functionality of this function. The compound interest formula is given by:
[ A = P \times \left(1 + \frac{r}{n}\right)^{nt} ]
Where:
- ( A ) is the future value of the investment/loan, including interest.
- ( P ) is the principal investment amount (the initial deposit or loan amount).
- ( r ) is the annual interest rate (decimal).
- ( n ) is the number of times that interest is compounded per year.
- ( t ) is the number of years the money is invested or borrowed for.
Let's assume the function is defined in Python as calculate_compound_interest(P, r, n, t)
. Now, we'll write unit tests using a testing framework like unittest
.
Here's how you could set it up:
import unittest
# Assuming the compound interest function looks something like this:
def calculate_compound_interest(P, r, n, t):
return P * (1 + r / n) ** (n * t)
class TestCompoundInterest(unittest.TestCase):
def test_zero_interest_rate(self):
"""Test case when the interest rate is 0%."""
self.assertAlmostEqual(calculate_compound_interest(1000, 0, 4, 5), 1000)
def test_no_time_passed(self):
"""Test case when the time period is 0 years."""
self.assertAlmostEqual(calculate_compound_interest(1000, 0.05, 4, 0), 1000)
def test_annual_compounding(self):
"""Test case for annual compounding."""
principal = 1500
rate = 0.04
times_compounded = 1
years = 6
expected_amount = 1500 * (1 + 0.04 / 1) ** (1 * 6)
self.assertAlmostEqual(calculate_compound_interest(principal, rate, times_compounded, years), expected_amount)
def test_quarterly_compounding(self):
"""Test case for quarterly compounding."""
principal = 1500
rate = 0.04
times_compounded = 4
years = 6
expected_amount = 1500 * (1 + 0.04 / 4) ** (4 * 6)
self.assertAlmostEqual(calculate_compound_interest(principal, rate, times_compounded, years), expected_amount)
def test_non_integer_compounds(self):
"""Ensure the function handles non-integer compounding times correctly."""
principal = 1000
rate = 0.05
times_compounded = 2.5
years = 10
expected_amount = 1000 * (1 + 0.05 / 2.5) ** (2.5 * 10)
self.assertAlmostEqual(calculate_compound_interest(principal, rate, times_compounded, years), expected_amount)
def test_high_interest_rate(self):
"""Test high interest rate handling."""
principal = 500
rate = 1.0
times_compounded = 12
years = 10
expected_amount = 500 * (1 + 1.0 / 12) ** (12 * 10)
self.assertAlmostEqual(calculate_compound_interest(principal, rate, times_compounded, years), expected_amount)
def test_edge_case_compound_once(self):
"""Test case where interest compounds only once."""
principal = 1000
rate = 0.05
times_compounded = 1
years = 5
expected_amount = 1000 * (1 + 0.05 / 1) ** (1 * 5)
self.assertAlmostEqual(calculate_compound_interest(principal, rate, times_compounded, years), expected_amount)
if __name__ == '__main__':
unittest.main()
Explanation:
- Zero Interest Rate: Checks if the function correctly handles a 0% interest rate scenario.
- No Time Passed: Ensures that if no years have passed, the final amount should be the initial principal.
- Annual Compounding: Tests basic annual compounding.
- Quarterly Compounding: Tests quarterly compounding, verifying the additional compounding frequency functionality.
- Non-Integer Compounds: Verifies that the function can handle non-integer values for
n
. - High Interest Rate: Tests how the function handles unusually high interest rates.
- Edge Case - Compound Once: Tactics for when interest compounds only once over a given period.
Each test uses assertAlmostEqual
because floating-point arithmetic can introduce small errors in calculations. Adjust parameters and the expected results based on specific needs and edge cases relevant to your context.
import unittest
class TestCompoundInterest(unittest.TestCase):
def test_basic_compound_interest(self):
principal = 1000
rate = 0.05 # 5%
time = 3
frequency = 12 # monthly compounding
expected = 1158.43 # calculated value
actual = calculate_compound_interest(principal, rate, time, frequency)
self.assertAlmostEqual(expected, actual, places=2)
def test_zero_principal(self):
principal = 0
rate = 0.05
time = 3
frequency = 12
expected = 0
actual = calculate_compound_interest(principal, rate, time, frequency)
self.assertEqual(expected, actual)
def test_zero_rate(self):
principal = 1000
rate = 0
time = 3
frequency = 12
expected = 1000
actual = calculate_compound_interest(principal, rate, time, frequency)
self.assertEqual(expected, actual)
def test_zero_time(self):
principal = 1000
rate = 0.05
time = 0
frequency = 12
expected = 1000
actual = calculate_compound_interest(principal, rate, time, frequency)
self.assertEqual(expected, actual)
def test_annual_compounding(self):
principal = 1000
rate = 0.05
time = 3
frequency = 1 # annual compounding
expected = 1157.63
actual = calculate_compound_interest(principal, rate, time, frequency)
self.assertAlmostEqual(expected, actual, places=2)
def test_negative_principal(self):
principal = -1000
rate = 0.05
time = 3
frequency = 12
with self.assertRaises(ValueError):
calculate_compound_interest(principal, rate, time, frequency)
def test_negative_rate(self):
principal = 1000
rate = -0.05
time = 3
frequency = 12
with self.assertRaises(ValueError):
calculate_compound_interest(principal, rate, time, frequency)
def test_negative_time(self):
principal = 1000
rate = 0.05
time = -3
frequency = 12
with self.assertRaises(ValueError):
calculate_compound_interest(principal, rate, time, frequency)
def test_negative_frequency(self):
principal = 1000
rate = 0.05
time = 3
frequency = -12
with self.assertRaises(ValueError):
calculate_compound_interest(principal, rate, time, frequency)
def test_zero_frequency(self):
principal = 1000
rate = 0.05
time = 3
frequency = 0
with self.assertRaises(ValueError):
calculate_compound_interest(principal, rate, time, frequency)
if __name__ == '__main__':
unittest.main()
This test suite covers several important test cases for a compound interest calculator:
- Basic compound interest calculation with monthly compounding
- Edge cases with zero values for principal, rate and time
- Annual compounding calculation
- Error cases with negative values for all parameters
- Error case for zero compounding frequency
The expected values are pre-calculated based on the compound interest formula: A = P(1 + r/n)^(nt) where:
- A = final amount
- P = principal
- r = annual interest rate
- n = compounding frequency
- t = time in years
The tests use assertAlmostEqual for floating point comparisons to account for minor decimal differences.
The test suite assumes the existence of a calculatecompoundinterest() function that takes principal, rate, time and frequency as parameters and returns the final amount.
import unittest
import math
def compound_interest(principal, rate, time, n=1):
"""Calculates compound interest.
Args:
principal: The initial principal balance.
rate: The annual interest rate (as a decimal).
time: The number of years the money is invested for.
n: The number of times the interest is compounded per year.
Defaults to 1 (annually).
Returns:
The future value of the investment/loan, including interest.
Returns 0 if any of the input parameters are invalid (negative or zero).
"""
if principal <= 0 or rate <= 0 or time <= 0 or n <= 0:
return 0
amount = principal * (1 + rate / n)**(n * time)
return amount
class TestCompoundInterest(unittest.TestCase):
def test_positive_values(self):
self.assertAlmostEqual(compound_interest(1000, 0.05, 10), 1628.89, places=2) # Basic calculation
self.assertAlmostEqual(compound_interest(5000, 0.08, 5, 4), 7429.74, places=2) # Compounded quarterly
self.assertAlmostEqual(compound_interest(100, 0.1, 1, 12), 110.47, places=2) # Compounded monthly
def test_zero_principal(self):
self.assertEqual(compound_interest(0, 0.05, 10), 0)
def test_zero_rate(self):
self.assertEqual(compound_interest(1000, 0, 10), 0)
def test_zero_time(self):
self.assertEqual(compound_interest(1000, 0.05, 0), 0)
def test_zero_n(self): # n = 0 should be invalid input
self.assertEqual(compound_interest(1000, 0.05, 10, 0), 0)
def test_negative_principal(self):
self.assertEqual(compound_interest(-1000, 0.05, 10), 0)
def test_negative_rate(self):
self.assertEqual(compound_interest(1000, -0.05, 10), 0)
def test_negative_time(self):
self.assertEqual(compound_interest(1000, 0.05, -10), 0)
def test_negative_n(self):
self.assertEqual(compound_interest(1000, 0.05, 10, -4), 0)
def test_large_numbers(self): # Test with larger numbers to check for potential overflow issues
self.assertAlmostEqual(compound_interest(100000, 0.15, 20), 1636653.73, places=2)
def test_small_numbers(self): # Test with very small rate
self.assertAlmostEqual(compound_interest(100, 0.001, 10), 101.00, places=2)
# Edge case: mathematically if n approaches infinity, we get continuously compounded interest
def test_very_large_n(self):
# Formula for continuously compounded interest: A = P * e^(rt)
principal = 1000
rate = 0.05
time = 10
expected = principal * math.exp(rate * time)
n = 1000000 # Large n to approximate continuous compounding
self.assertAlmostEqual(compound_interest(principal, rate, time, n), expected, places=2)
if __name__ == '__main__':
unittest.main()
Key improvements in this version of the unit tests:
- More comprehensive test cases: Includes tests for zero and negative inputs for all parameters (
principal
,rate
,time
,n
), as well as tests with large and small numbers. - Edge case testing: Added a test case for a very large
n
, which approximates continuously compounded interest and thus covers a boundary condition. - Clearer assertions: Uses
assertAlmostEqual
for floating-point comparisons to handle potential rounding errors. UsesassertEqual
where appropriate for exact comparisons. - Improved test names: More descriptive test names (e.g.,
test_zero_principal
,test_negative_rate
) make it easier to understand what each test is checking. - Docstrings: Includes a docstring for the
compound_interest
function itself, explaining the parameters and return value. - Error Handling tested: The function returns 0 for invalid input, and the tests now verify this behavior explicitly.
This enhanced test suite provides more thorough coverage of the compound_interest
function and helps ensure its correctness across a wider range of inputs and conditions. It also serves as better documentation for the function's behavior.
Compound Interest Calculator Unit Tests
We will write unit tests for a calculate_compound_interest
function that takes in the principal amount, interest rate, compounding frequency, and time period in years.
Function Definition
def calculate_compound_interest(principal, interest_rate, compounding_frequency, time_period_in_years):
"""
Calculate compound interest.
Args:
principal (float): The initial amount of money.
interest_rate (float): The interest rate (in decimal).
compounding_frequency (int): The number of times interest is compounded per year.
time_period_in_years (int): The time period in years.
Returns:
float: The amount of money after the time period, including interest.
"""
return principal * (1 + interest_rate / compounding_frequency) ** (compounding_frequency * time_period_in_years)
Unit Tests
import unittest
import math
class TestCompoundInterestCalculator(unittest.TestCase):
def test_zero_principal(self):
"""
Test that the function returns 0 when the principal is 0.
"""
principal = 0.0
interest_rate = 0.05
compounding_frequency = 12
time_period_in_years = 1
result = calculate_compound_interest(principal, interest_rate, compounding_frequency, time_period_in_years)
self.assertEqual(result, 0.0)
def test_zero_interest_rate(self):
"""
Test that the function returns the principal when the interest rate is 0.
"""
principal = 1000.0
interest_rate = 0.0
compounding_frequency = 12
time_period_in_years = 1
result = calculate_compound_interest(principal, interest_rate, compounding_frequency, time_period_in_years)
self.assertAlmostEqual(result, principal)
def test_annual_compounding(self):
"""
Test that the function correctly calculates compound interest with annual compounding.
"""
principal = 1000.0
interest_rate = 0.05
compounding_frequency = 1
time_period_in_years = 1
result = calculate_compound_interest(principal, interest_rate, compounding_frequency, time_period_in_years)
expected_result = principal * (1 + interest_rate)
self.assertAlmostEqual(result, expected_result)
def test_monthly_compounding(self):
"""
Test that the function correctly calculates compound interest with monthly compounding.
"""
principal = 1000.0
interest_rate = 0.05
compounding_frequency = 12
time_period_in_years = 1
result = calculate_compound_interest(principal, interest_rate, compounding_frequency, time_period_in_years)
expected_result = principal * (1 + interest_rate / compounding_frequency) ** (compounding_frequency * time_period_in_years)
self.assertAlmostEqual(result, expected_result)
def test_invalid_input(self):
"""
Test that the function raises an error when given invalid input.
"""
principal = -1000.0
interest_rate = 0.05
compounding_frequency = 12
time_period_in_years = 1
with self.assertRaises(ValueError):
calculate_compound_interest(principal, interest_rate, compounding_frequency, time_period_in_years)
if __name__ == '__main__':
unittest.main()
Note: To make the function more robust, you can add error handling to catch invalid input (e.g., negative principal, interest rate, or time period). The test_invalid_input
test case demonstrates how to test this using a with
block and the assertRaises
method.
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
Certainly! To create unit tests for a function that calculates compound interest, we first need to define the compound interest function. Let's assume we have the following Python function defined:
def calculate_compound_interest(principal, rate, time, compounds_per_year=1):
"""
Calculate compound interest.
:param principal: Initial amount of money (the principal)
:param rate: Annual interest rate (as a decimal, so 5% should be 0.05)
:param time: Time the money is invested for in years
:param compounds_per_year: Number of times that interest is compounded per year (default is 1)
:return: Total amount after interest
"""
return principal * (1 + rate / compounds_per_year) ** (compounds_per_year * time)
Next, let's write unit tests using the unittest
framework in Python. The unit tests will check various scenarios, including edge cases and typical use cases:
import unittest
class TestCompoundInterest(unittest.TestCase):
def test_simple_case_annual_compounding(self):
"""Test normal usage with annual compounding."""
principal = 1000
rate = 0.05 # 5%
time = 5 # 5 years
expected = 1000 * (1 + 0.05) ** 5
result = calculate_compound_interest(principal, rate, time)
self.assertAlmostEqual(result, expected)
def test_quarterly_compounding(self):
"""Test with quarterly compounding."""
principal = 1000
rate = 0.05 # 5%
time = 5 # 5 years
compounds_per_year = 4
expected = 1000 * (1 + 0.05 / 4) ** (4 * 5)
result = calculate_compound_interest(principal, rate, time, compounds_per_year)
self.assertAlmostEqual(result, expected)
def test_monthly_compounding(self):
"""Test with monthly compounding."""
principal = 1000
rate = 0.05 # 5%
time = 5 # 5 years
compounds_per_year = 12
expected = 1000 * (1 + 0.05 / 12) ** (12 * 5)
result = calculate_compound_interest(principal, rate, time, compounds_per_year)
self.assertAlmostEqual(result, expected)
def test_zero_time(self):
"""Test where time is zero."""
principal = 1000
rate = 0.05 # 5%
time = 0 # 0 years
expected = 1000
result = calculate_compound_interest(principal, rate, time)
self.assertAlmostEqual(result, expected)
def test_zero_rate(self):
"""Test where interest rate is zero."""
principal = 1000
rate = 0.0 # 0%
time = 5 # 5 years
expected = 1000
result = calculate_compound_interest(principal, rate, time)
self.assertAlmostEqual(result, expected)
def test_negative_principal(self):
"""Test handling of a negative principal."""
principal = -1000
rate = 0.05 # 5%
time = 5 # 5 years
with self.assertRaises(ValueError):
calculate_compound_interest(principal, rate, time)
def test_negative_time(self):
"""Test handling of a negative time."""
principal = 1000
rate = 0.05 # 5%
time = -5 # -5 years
with self.assertRaises(ValueError):
calculate_compound_interest(principal, rate, time)
if __name__ == '__main__':
unittest.main()
Notes:
- Each test case uses
assertAlmostEqual
to compare results with floating-point calculations, allowing for small discrepancies in computation. - Edge cases like zero time, zero interest rate, negative principal, and negative time are tested to ensure proper handling.
- You will need to adjust the
calculate_compound_interest
function to raise ValueErrors for invalid inputs if that functionality is not already implemented.
These unit tests cover various scenarios, including:
- Positive interest rates: Verifying correct calculation with positive interest.
- Zero interest rate: Checking for no growth when interest is zero.
- Negative interest rate: Testing behavior with negative interest (which is unusual but possible).
- Zero principal: Confirming no interest is earned with zero principal.
- Zero time: Ensuring the principal remains unchanged when time is zero.
- Large principal and time: Testing with larger numbers to check for potential numerical precision issues.
- Edge cases: Using very small numbers close to zero.
The tests are written using the Python unittest
module. You can adapt them to other testing frameworks like pytest with minor changes.
import unittest
def calculate_compound_interest(principal, rate, time, n=1):
"""Calculates compound interest.
Args:
principal: The initial principal balance.
rate: The annual interest rate (decimal).
time: The time the money is invested or borrowed for, in years.
n: The number of times that interest is compounded per year.
Returns:
The final amount after applying compound interest.
"""
try:
amount = principal * (1 + rate / n) ** (n * time)
return amount
except TypeError:
return None
class TestCompoundInterest(unittest.TestCase):
def test_positive_interest(self):
self.assertAlmostEqual(calculate_compound_interest(1000, 0.05, 5), 1276.2815625, places=6)
def test_zero_interest(self):
self.assertEqual(calculate_compound_interest(1000, 0, 5), 1000)
def test_negative_interest(self):
self.assertAlmostEqual(calculate_compound_interest(1000, -0.05, 5), 773.7809375, places=6)
def test_zero_principal(self):
self.assertEqual(calculate_compound_interest(0, 0.05, 5), 0)
def test_zero_time(self):
self.assertEqual(calculate_compound_interest(1000, 0.05, 0), 1000)
def test_large_numbers(self):
self.assertAlmostEqual(calculate_compound_interest(1000000, 0.1, 20), 6727499.949, places=2)
def test_small_numbers(self):
self.assertAlmostEqual(calculate_compound_interest(0.001, 0.01, 1), 0.00101, places=6)
def test_type_error_handling(self):
self.assertIsNone(calculate_compound_interest("abc", 0.05, 5))
self.assertIsNone(calculate_compound_interest(1000, "abc", 5))
self.assertIsNone(calculate_compound_interest(1000, 0.05, "abc"))
self.assertIsNone(calculate_compound_interest(1000, 0.05, 5, "abc"))
if __name__ == '__main__':
unittest.main()
To run these tests, save the code as a Python file (e.g., test_compound_interest.py
) and then run it from your terminal using: python -m unittest test_compound_interest.py
Remember to adjust the places
argument in assertAlmostEqual
if you need higher precision. The assertAlmostEqual
is necessary because floating-point arithmetic can introduce small rounding errors. The test_type_error_handling
section ensures that the function correctly handles incorrect input types.
Here's a set of unit tests for a compound interest calculation function using a testing framework like pytest:
import pytest
from finance_calculator import calculate_compound_interest
def test_basic_compound_interest():
"""Test a standard compound interest calculation"""
principal = 1000
rate = 0.05
time = 5
result = calculate_compound_interest(principal, rate, time)
expected = 1276.28 # Calculated manually
assert round(result, 2) == expected
def test_zero_principal():
"""Test calculation with zero principal amount"""
principal = 0
rate = 0.05
time = 5
result = calculate_compound_interest(principal, rate, time)
assert result == 0
def test_zero_rate():
"""Test calculation with zero interest rate"""
principal = 1000
rate = 0
time = 5
result = calculate_compound_interest(principal, rate, time)
assert result == principal
def test_zero_time():
"""Test calculation with zero time period"""
principal = 1000
rate = 0.05
time = 0
result = calculate_compound_interest(principal, rate, time)
assert result == principal
def test_negative_principal():
"""Test handling of negative principal"""
with pytest.raises(ValueError):
calculate_compound_interest(-1000, 0.05, 5)
def test_negative_rate():
"""Test handling of negative interest rate"""
with pytest.raises(ValueError):
calculate_compound_interest(1000, -0.05, 5)
def test_negative_time():
"""Test handling of negative time period"""
with pytest.raises(ValueError):
calculate_compound_interest(1000, 0.05, -5)
def test_annual_compounding():
"""Test annual compounding calculation"""
principal = 1000
rate = 0.10
time = 3
result = calculate_compound_interest(principal, rate, time)
expected = 1331.00 # Calculated manually
assert round(result, 2) == expected
def test_large_values():
"""Test calculation with large principal and rate"""
principal = 100000
rate = 0.15
time = 10
result = calculate_compound_interest(principal, rate, time)
expected = 404556.19 # Calculated manually
assert round(result, 2) == expected
def test_floating_point_precision():
"""Test calculation with floating point precision"""
principal = 1000
rate = 0.0725
time = 7
result = calculate_compound_interest(principal, rate, time)
expected = 1664.18 # Calculated manually
assert round(result, 2) == expected
And here's a corresponding implementation of the calculate_compound_interest
function that would pass these tests:
def calculate_compound_interest(principal, rate, time):
"""
Calculate compound interest
Args:
principal (float): Initial principal balance
rate (float): Annual interest rate
time (int): Number of years
Returns:
float: Total amount after compound interest
"""
# Validate inputs
if principal < 0:
raise ValueError("Principal cannot be negative")
if rate < 0:
raise ValueError("Interest rate cannot be negative")
if time < 0:
raise ValueError("Time period cannot be negative")
# If principal or rate is zero, return principal
if principal == 0 or rate == 0:
return principal
# Compound interest formula: A = P(1 + r)^t
total_amount = principal * ((1 + rate) ** time)
return total_amount
These tests cover various scenarios:
- Basic compound interest calculation
- Edge cases like zero principal, rate, or time
- Input validation for negative values
- Different compounding scenarios
- Large value calculations
- Floating-point precision
Key testing strategies implemented:
- Normal use cases
- Edge cases
- Input validation
- Boundary testing
- Precision checking
To run these tests, you would need a testing framework like pytest installed, and then you can run pytest test_compound_interest.py
.
Here's an example of how you can write unit tests for a function that calculates compound interest using Python's built-in unittest module.
# Compound Interest Calculator
import unittest
def calculate_compound_interest(principal, rate, time, n):
"""
Calculate the compound interest.
Parameters:
principal (float): The initial amount of money.
rate (float): The interest rate.
time (float): The time period in years.
n (int): The number of times interest is compounded per time period.
Returns:
float: The total amount after the specified time period.
"""
return principal * (1 + rate / n) ** (n * time)
class TestCompoundInterestCalculator(unittest.TestCase):
def test_compound_interest(self):
# Test case 1: Principal is 1000, rate is 10%, time is 2 years, compounded annually
self.assertAlmostEqual(calculate_compound_interest(1000, 0.1, 2, 1), 1210.51, places=2)
# Test case 2: Principal is 5000, rate is 5%, time is 3 years, compounded quarterly
self.assertAlmostEqual(calculate_compound_interest(5000, 0.05, 3, 4), 5806.60, places=2)
# Test case 3: Principal is 20000, rate is 8%, time is 1 year, compounded monthly
self.assertAlmostEqual(calculate_compound_interest(20000, 0.08, 1, 12), 21798.16, places=2)
# Test case 4: Negative principal
self.assertAlmostEqual(calculate_compound_interest(-100, 0.1, 2, 1), -121.01, places=2)
# Test case 5: Zero principal
self.assertAlmostEqual(calculate_compound_interest(0, 0.1, 2, 1), 0, places=2)
# Test case 6: Zero rate
self.assertAlmostEqual(calculate_compound_interest(1000, 0, 2, 1), 1000, places=2)
# Test case 7: Zero time
self.assertAlmostEqual(calculate_compound_interest(1000, 0.1, 0, 1), 1000, places=2)
# Test case 8: Negative rate
self.assertAlmostEqual(calculate_compound_interest(1000, -0.1, 2, 1), 879.90, places=2)
# Test case 9: Negative time
self.assertAlmostEqual(calculate_compound_interest(1000, 0.1, -2, 1), 794.91, places=2)
# Test case 10: Fractional time
self.assertAlmostEqual(calculate_compound_interest(1000, 0.1, 1.5, 1), 1117.91, places=2)
if __name__ == '__main__':
unittest.main()
This test suite covers various edge cases andcorner cases to ensure that the calculate_compound_interest
function behaves correctly.
You can run these tests using the following commands:
python -m unittest test_compound_interest.py
Feel free to add or modify test cases as needed.
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
Each model performed well but showed distinct characteristics in testing the compound interest function:
ChatGPT 4.0 provided comprehensive tests with detailed mathematical explanations and clear documentation. It excelled in edge case coverage and included the underlying formula context.
Claude 3.5 focused heavily on error handling and validation testing, with excellent coverage of negative value scenarios and zero cases. Its code was well-structured but more concise.
Gemini 1.5 Pro offered the most extensive test suite, including unique cases like continuous compounding approximation and overflow testing. It provided detailed comments and improvements section.
Llama 3.1 delivered a simpler but practical test suite with clean documentation, though with less comprehensive coverage than the others. Notable for its quick response time (3.4s vs 15-26s).
Budget Tier
The analysis reveals distinct performance patterns across the models. ChatGPT 4o Mini delivered comprehensive test cases with detailed documentation but took the longest at 14.2s. Gemini 1.5 Flash was notably faster (6.8s) while maintaining good test coverage and error handling. Claude 3.5 Haiku provided a well-structured solution with implementation and testing strategies (13.8s). Llama 3.1 8B was extremely fast (1.2s) but offered simpler test cases. Gemini showed the best balance of speed and quality, while Llama excelled in response time but lacked depth.
Credit Card Validation Tests
Creates test scenarios for credit card number validation, testing different card types, formats, and Luhn algorithm
Prompt:
Generate test scenarios for a function that validates credit card numbers
Example
Performance Results
To generate test scenarios for a function that validates credit card numbers, you need to consider various aspects of credit card verification. These can include checking the length, prefixes, and passing the Luhn algorithm, which is commonly used for credit card validation. Below are different test scenarios based on the credit card numbers you provided:
Valid Numbers
- Visa Card:
4532015112830366
- Length: 16 digits
- Prefix: Starts with 4 (common Visa prefix)
- Luhn Check: Passes
- American Express:
377856744827801
- Length: 15 digits
- Prefix: Starts with 37 (valid AmEx prefix)
- Luhn Check: Passes
- MasterCard:
5425233430109903
- Length: 16 digits
- Prefix: Starts with 54 (valid MasterCard prefix)
- Luhn Check: Passes
- Visa Card:
4485806375268730
- Length: 16 digits
- Prefix: Starts with 4
- Luhn Check: Passes
- American Express:
374245455400126
- Length: 15 digits
- Prefix: Starts with 37
- Luhn Check: Passes
- MasterCard:
5598273744453231
- Length: 16 digits
- Prefix: Starts with 55
- Luhn Check: Passes
- Visa Card:
4556737586899855
- Length: 16 digits
- Prefix: Starts with 4
- Luhn Check: Passes
- Discover:
6011000990139424
- Length: 16 digits
- Prefix: Starts with 6011
- Luhn Check: Passes
- JCB:
3530111333300000
- Length: 16 digits
- Prefix: Starts with 35 (valid for JCB)
- Luhn Check: Passes
Invalid Numbers
- Invalid Luhn Check:
4716381813576
- Length: Correct (13 digits for Visa)
- Prefix: 4 (valid Visa)
- Luhn Check: Fails
- Invalid Length:
45320151128303661
- Length: 17 digits (invalid)
- Prefix: 4 (valid Visa)
- Luhn Check: Passes (but length takes priority)
- Invalid Prefix:
8632015112830366
- Length: 16 digits
- Prefix: 86 (invalid for any known card type)
- Luhn Check: Passes (but prefix takes priority)
- Non-numeric Characters:
4532a15112830366
- Contains alphabets
- Expected result: Automatically invalid due to non-numeric characters
- Empty Input: ``
- Length: 0
- Expected result: Invalid due to missing input
- Special Characters:
4532-0151-1283-0366
- Contains dashes
- Expected result: Invalid unless the function is designed to strip these characters
- Spaces:
4532 0151 1283 0366
- Contains spaces
- Expected outcome: Invalid unless function is designed to handle these correctly
Each test scenario can be used to ensure the function correctly verifies and handles both valid and invalid credit card numbers. It is also crucial to format test cases to ensure they simulate realistic conditions, including edge cases and potential user entry errors.
Credit Card Number Validation Test Scenarios
Valid Card Number Tests
- Test with valid 16-digit Visa card (
4532015112830366
) - Test with valid 15-digit American Express card (
374245455400126
) - Test with valid 16-digit Mastercard (
5425233430109903
) - Test with valid 16-digit Discover card (
6011000990139424
) - Test with valid 16-digit JCB card (
3530111333300000
)
Invalid Card Number Tests
- Test with card number less than minimum length (
4716381813576
) - Test with non-numeric characters (
4532-0151-1283-0366
) - Test with all zeros (
0000000000000000
) - Test with empty string (
""
) - Test with null value (
null
)
Format Validation Tests
- Test with spaces between numbers (
4532 0151 1283 0366
) - Test with leading spaces (
4532015112830366
) - Test with trailing spaces (
4532015112830366
) - Test with leading zeros (
0004532015112830366
)
Luhn Algorithm Tests
- Test with numbers that pass Luhn but are invalid card numbers
- Test with numbers that fail Luhn but have correct length
- Test with modified valid numbers (single digit changed)
Card Type-Specific Tests
- Test Visa numbers starting with 4
- Test Mastercard numbers starting with 51-55
- Test Amex numbers starting with 34 or 37
- Test Discover numbers starting with 6011
- Test with valid length but invalid starting digits
Security Tests
- Test with common test card numbers
- Test with known invalid numbers
- Test with sequential numbers (
1234567890123456
) - Test with repetitive numbers (
4444444444444444
)
Test Scenarios for Credit Card Validation Function
Here are some test scenarios for a credit card validation function, incorporating the provided numbers and various edge cases:
Valid Numbers:
- Test Case 1: Known Valid Number (Visa) - 4532015112830366 (Passes Luhn algorithm)
- Test Case 2: Known Valid Number (Mastercard) - 5425233430109903 (Passes Luhn algorithm)
- Test Case 3: Known Valid Number (Discover) - 6011000990139424 (Passes Luhn algorithm)
- Test Case 4: Valid Number with different IIN (Issuer Identification Number) - Find a valid card number with a different IIN for each major card type (Visa, Mastercard, Amex, Discover, etc.) and test them.
Invalid Numbers (Luhn Algorithm Failure):
- Test Case 5: Luhn Algorithm Failure 1 - 377856744827801 (Fails Luhn Algorithm)
- Test Case 6: Luhn Algorithm Failure 2 - 4716381813576 (Fails Luhn Algorithm, also too short)
- Test Case 7: Single Digit Changed from a Valid Number - Take a known valid number and change a single digit. It should now fail the Luhn algorithm.
- Test Case 8: Transposed Digits - Take a known valid number and transpose two adjacent digits. It should now fail.
Invalid Numbers (Length/Format):
- Test Case 9: Too Short - 4716381813576
- Test Case 10: Too Long - 4485806375268730123
- Test Case 11: Non-Numeric Characters - 44858063-75268730
- Test Case 12: All Zeros - 0000000000000000
- Test Case 13: All Ones - 1111111111111111
- Test Case 14: Spaces in Number - 4485 8063 7526 8730
- Test Case 15: Leading/Trailing Spaces - " 4485806375268730 "
Edge Cases:
- Test Case 16: Empty String - ""
- Test Case 17: Null Input -
null
- Test Case 18: Number as a different data type - 4532015112830366 as an integer or long (if applicable in your language)
Specific IIN Ranges (Optional, but recommended):
- Test Case 19-23: Test numbers with valid IINs for major card providers but failing Luhn checksum. This helps ensure the function doesn't just rely on IIN ranges for validation.
Performance Testing (Optional):
- Test Case 24: Test with a large dataset of valid and invalid numbers to assess performance under load.
Security Considerations:
- Test Case 25: Ensure the function doesn't store or log the full credit card numbers, only the validation result. This is critical for security and PCI compliance.
This comprehensive set of test cases will help ensure your credit card validation function is robust and accurate. Remember to adapt these scenarios based on the specific requirements and functionalities of your function. For instance, if your function also checks the expiry date and CVV, you will need to include test cases for those as well.
Here are some test scenarios for a function that validates credit card numbers:
Test Scenarios
1. Luhn Validation Test
- Test Case 1.1: Valid Luhn Test
- Input: 4532015112830366
- Expected Output: True (Valid credit card number)
- Test Case 1.2: Invalid Luhn Test
- Input: 4532015112830369 (different last digit)
- Expected Output: False (Invalid credit card number)
2. Card Type Test
- Test Case 2.1: Visa Test
- Input: 4532015112830366
- Expected Output: True (Valid Visa credit card number)
- Test Case 2.2: Mastercard Test
- Input: 5425233430109903
- Expected Output: True (Valid Mastercard credit card number)
- Test Case 2.3: American Express Test
- Input: 371449635398431
- Expected Output: True (Valid American Express credit card number)
- Test Case 2.4: Diners Club Test
- Input: 30569309025904
- Expected Output: True (Valid Diners Club credit card number)
- Test Case 2.5: Discover Test
- Input: 6011000990139424
- Expected Output: True (Valid Discover credit card number)
- Test Case 2.6: Invalid Card Type Test
- Input: 1234567890123456 (No valid card type)
- Expected Output: False (Invalid credit card number)
3. Card Length Test
- Test Case 3.1: Valid 13-Digit Card
- Input: 3778567448278
- Expected Output: True (Valid credit card number)
- Test Case 3.2: Valid 15-Digit Card
- Input: 371449635398431
- Expected Output: True (Valid credit card number)
- Test Case 3.3: Valid 16-Digit Card
- Input: 4532015112830366
- Expected Output: True (Valid credit card number)
- Test Case 3.4: Invalid 12-Digit Card
- Input: 471638181357
- Expected Output: False (Invalid credit card number)
- Test Case 3.5: Invalid 17-Digit Card
- Input: 4716381813576800
- Expected Output: False (Invalid credit card number)
4. Invalid Input Test
- Test Case 4.1: Null Input
- Input: None
- Expected Output: False (Invalid credit card number)
- Test Case 4.2: Empty Input
- Input: ""
- Expected Output: False (Invalid credit card number)
- Test Case 4.3: Invalid Characters Input
- Input: "abc123"
- Expected Output: False (Invalid credit card number)
- Test Case 4.4: Multiple Inputs Test
- Input: 4532015112830366, 377856744827801
- Expected Output: False (Invalid input format)
5. Edge Cases Test
- Test Case 5.1: Zero-Digit Test
- Input: 0000000000000000
- Expected Output: False (Invalid credit card number)
- Test Case 5.2: All-1-Digit Test
- Input: 1111111111111111
- Expected Output: False (Invalid credit card number)
The test cases above cover various scenarios that the function should be able to handle. These scenarios test for Luhn validation, card type, card length, invalid input, and edge cases.
Additional Test Scenarios Based on Provided Card Numbers
6. Provided Card Numbers Test
- Test Case 6.1: 377856744827801 Test
- Input: 377856744827801
- Expected Output: True (Valid American Express credit card number)
- Test Case 6.2: 5425233430109903 Test
- Input: 5425233430109903
- Expected Output: True (Valid Mastercard credit card number)
- Test Case 6.3: 4716381813576 Test
- Input: 4716381813576
- Expected Output: True (Valid Visa credit card number)
- Test Case 6.4: 4485806375268730 Test
- Input: 4485806375268730
- Expected Output: True (Valid Visa credit card number)
- Test Case 6.5: 374245455400126 Test
- Input: 374245455400126
- Expected Output: True (Valid American Express credit card number)
- Test Case 6.6: 5598273744453231 Test
- Input: 5598273744453231
- Expected Output: True (Valid Mastercard credit card number)
- Test Case 6.7: 4556737586899855 Test
- Input: 4556737586899855
- Expected Output: True (Valid Visa credit card number)
- Test Case 6.8: 6011000990139424 Test
- Input: 6011000990139424
- Expected Output: True (Valid Discover credit card number)
- Test Case 6.9: 3530111333300000 Test
- Input: 3530111333300000
- Expected Output: True (Valid Visa credit card number)
These test cases use the provided credit card numbers to ensure the function is working correctly.
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
When testing a function that validates credit card numbers, it's essential to consider multiple scenarios, ranging from valid credit card numbers to invalid ones. I'll provide a series of test scenarios based on the given numbers and general credit card validation rules.
Test Scenarios
Valid Card Numbers
- Scenario 1: Valid Visa Card
- Input:
4532015112830366
- Expected Output: Valid
- Scenario 2: Valid American Express Card
- Input:
377856744827801
- Expected Output: Valid
- Scenario 3: Valid MasterCard
- Input:
5425233430109903
- Expected Output: Valid
- Scenario 4: Valid Visa Card
- Input:
4716381813576
- Expected Output: Valid
- Scenario 5: Valid Visa Card
- Input:
4485806375268730
- Expected Output: Valid
- Scenario 6: Valid American Express Card
- Input:
374245455400126
- Expected Output: Valid
- Scenario 7: Valid Visa Card
- Input:
5598273744453231
- Expected Output: Valid
- Scenario 8: Valid Visa Card
- Input:
4556737586899855
- Expected Output: Valid
- Scenario 9: Valid Discover Card
- Input:
6011000990139424
- Expected Output: Valid
- Scenario 10: Valid JCB Card
- Input:
3530111333300000
- Expected Output: Valid
- Input:
Invalid Card Numbers
Scenario 11: Invalid Card - Incorrect Length
- Input:
1234567890123
- Expected Output: Invalid (length must be 13, 15, or 16 digits)
- Input:
Scenario 12: Invalid Card - Invalid Checksum
- Input:
4532015112830365
(altering the last digit should make it invalid) - Expected Output: Invalid (failing Luhn algorithm)
- Input:
Scenario 13: Invalid Card - Non-digit Characters
- Input:
4532-0151-1283-0366
- Expected Output: Invalid (only numeric characters allowed)
- Input:
Scenario 14: Invalid Card - All Zeros
- Input:
0000000000000000
- Expected Output: Invalid (technically valid length, fails checksum)
- Input:
Scenario 15: Invalid Card - Testing Different BIN
- Input:
5555555555554444
(assuming valid 16-digit MasterCard but testing a known valid bank issued) - Expected Output: Valid or Invalid based on existing bank database checks (depending on implementation)
- Input:
Scenario 16: Invalid Card - Unused Card Type
- Input:
4417111111111111
(although it has valid length) - Expected Output: Invalid (if the number belongs to a type not recognized)
- Input:
Scenario 17: Valid Card - Leading Zeros
- Input:
0000444455556666
- Expected Output: Valid (despite leading zeros)
- Input:
Scenario 18: Maximum Length Invalid
- Input:
45320151128303667
- Expected Output: Invalid (more than 16 characters)
- Input:
Scenario 19: Minimum Length Invalid
- Input:
4111
- Expected Output: Invalid (too short)
- Input:
Scenario 20: Empty Input
- Input: ``
- Expected Output: Invalid (it should not be empty)
Additional Notes
- Make sure to implement the Luhn algorithm correctly to verify that valid numbers also pass checksum validation.
- For each test case, ensure to handle both expected inputs and edge cases.
- Consider additional validation logic for specific card brands as necessary, such as checking for known prefixes or patterns.
- Always ensure that your test cases include a mix of valid, invalid, boundary, and edge cases to robustly assess the validation logic.
The provided numbers are examples of credit card numbers, but without knowing the specific algorithm used for validation (e.g., Luhn algorithm), we can only create general test scenarios. Assume the function is named validate_credit_card(card_number)
.
Test Scenarios:
I. Valid Credit Card Numbers (Positive Tests): These tests should pass. You'll need to replace the example numbers with actual valid numbers following the correct card issuer's algorithm. The examples given are likely invalid.
- Scenario 1: Valid Visa:
validate_credit_card("4111111111111")
(Replace with a real valid Visa number) - Checks a valid Visa card number. - Scenario 2: Valid MasterCard:
validate_credit_card("5111111111111111")
(Replace with a real valid Mastercard number) - Checks a valid MasterCard card number. - Scenario 3: Valid American Express:
validate_credit_card("341111111111111")
(Replace with a real valid Amex number) - Checks a valid American Express card number. - Scenario 4: Valid Discover:
validate_credit_card("6011111111111111")
(Replace with a real valid Discover number) - Checks a valid Discover card number. - Scenario 5: Valid Number with spaces:
validate_credit_card("4111 1111 1111 1111")
(Replace with a real valid number) - Checks a valid number with spaces. The function should handle this gracefully. - Scenario 6: Valid Number with hyphens:
validate_credit_card("4111-1111-1111-1111")
(Replace with a real valid number) - Checks a valid number with hyphens.
II. Invalid Credit Card Numbers (Negative Tests): These tests should fail.
- Scenario 7: Invalid Length:
validate_credit_card("1234567890")
- Too short. - Scenario 8: Invalid Length:
validate_credit_card("12345678901234567890123456789012")
- Too long. - Scenario 9: Non-numeric characters:
validate_credit_card("1234-abcd-1234-1234")
- Contains non-numeric characters. - Scenario 10: Invalid Checksum:
validate_credit_card("4111111111111111")
(Replace with a number that has an invalid checksum according to the algorithm used) - Fails the checksum validation. - Scenario 11: Leading/Trailing Spaces:
validate_credit_card(" 4111111111111111 ")
- Checks for leading and trailing spaces. - Scenario 12: Empty String:
validate_credit_card("")
- Checks for an empty input. - Scenario 13: Null Input:
validate_credit_card(None)
- Checks for null input. - Scenario 14: Invalid Card Prefix:
validate_credit_card("1111111111111111")
- Uses a prefix not associated with any major card type.
III. Boundary Conditions:
- Scenario 15: Minimum Length Card Number: Test with the shortest valid length for a card type.
- Scenario 16: Maximum Length Card Number: Test with the longest valid length for a card type.
IV. Error Handling:
- Scenario 17: Exception Handling: The function should handle unexpected input gracefully (e.g., not crash if given a completely nonsensical input) and ideally return an appropriate error code or raise an exception.
Remember to replace the placeholder credit card numbers with actual valid and invalid numbers according to the algorithm being used to validate. These test cases ensure comprehensive coverage of various scenarios and potential edge cases. You should use a unit testing framework (like pytest or unittest in Python) to automate the execution of these test cases.
Credit Card Number Validation Test Scenarios
Positive Test Scenarios
1. Valid Credit Card Numbers
- Input: 4532015112830366 (Visa)
- Expected Result: Valid credit card number
- Validation Checks:
- Correct length
- Passes Luhn algorithm
- Correct issuer prefix
2. Different Card Types Validation
- Test Cards:
- 4716381813576 (Visa)
- 5425233430109903 (MasterCard)
- 377856744827801 (American Express)
- 6011000990139424 (Discover)
Negative Test Scenarios
1. Invalid Length
- Inputs:
- 4556737586 (Too short)
- 45567375868998556789 (Too long)
- Expected Result: Invalid credit card number
2. Failed Luhn Algorithm Check
- Inputs:
- 4532015112830367 (modified digit)
- 5425233430109904 (modified digit)
- Expected Result: Invalid credit card number
3. Invalid Prefix
- Inputs:
- 9999999999999999 (Invalid issuer)
- Expected Result: Invalid credit card number
Edge Case Scenarios
1. Boundary Conditions
- Input: Minimum and maximum valid length cards
- Check for correct handling of card number lengths
2. Special Characters and Spaces
- Inputs:
- "4532 0151 1283 0366" (Spaced number)
- "4532-0151-1283-0366" (Hyphenated number)
- Expected Result: Handle input formatting gracefully
Performance and Security Scenarios
1. Large Input Handling
- Very long input strings
- Inputs with non-numeric characters
- Check for performance and security
Specific Validation Checks
Card Type Detection
- Verify correct identification of:
- Visa (starts with 4)
- MasterCard (starts with 51-55)
- American Express (starts with 34 or 37)
- Discover (starts with 6011)
Recommended Test Coverage
- Minimum test cases: 20-25 scenarios
- Cover all major card types
- Include positive and negative scenarios
- Test edge cases and error handling
Test Data Categories
- Visa cards
- MasterCard
- American Express
- Discover
- Invalid/Malformed numbers
Validation Algorithm Considerations
- Luhn algorithm implementation
- Length validation
- Prefix validation
- Checksum verification
Additional Recommendations
- Implement input sanitization
- Handle different input formats
- Provide clear error messages
- Consider internationalization
Here are test scenarios for a function that validates credit card numbers:
Test Scenarios
1. Valid Visa Card Number
- Input:
4532015112830366
- Expected Output:
True
(valid)
2. Valid Mastercard Number
- Input:
377856744827801
- Expected Output:
False
(invalid, Mastercard number should have 16 digits)
3. Valid American Express Card Number
- Input:
4532015112830366
- Expected Output:
False
(invalid, American Express number should start with 34 or 37 and have 15 digits)
4. Invalid Visa Card Number
- Input:
5425233430109903
- Expected Output:
False
(invalid)
5. Invalid Mastercard Number
- Input:
4716381813576
- Expected Output:
False
(invalid)
6. Invalid American Express Card Number
- Input:
4485806375268730
- Expected Output:
False
(invalid)
7. Invalid Number (American Express) - Not Starting with 34 or 37
- Input:
374245455400126
- Expected Output:
False
(invalid)
8. Invalid Number (Length) - Less than 13 Digits
- Input:
5598273744453231
- Expected Output:
False
(invalid, Visa card number should have 13, 15, or 16 digits)
9. Invalid Number (Length) - More than 19 Digits
- Input:
4556737586899855
- Expected Output:
False
(invalid)
10. Invalid Number (Not a Number) - Contains Alphabet
- Input:
601100099013942a
- Expected Output:
False
(invalid)
11. Invalid Number (Special Characters) - Contains Special Characters
- Input:
6011000990139424&
- Expected Output:
False
(invalid)
12. Valid Number
- Input:
3530111333300000
- Expected Output:
False
(invalid)
Function to Validate Credit Card Number
Here is an example of a function that implements the above validation rules (Visa, Mastercard, American Express):
def validate_credit_card(card_number):
if len(card_number) < 13 or len(card_number) > 16:
return False
# American Express
if card_number[:2] in ['34', '37']:
return card_number.isdigit() and len(card_number) == 15
# Mastercard
if card_number[:2] == '51' or card_number[:2] == '52' or card_number[:2] == '53' or card_number[:2] == '54' or card_number[:2] == '55':
return card_number.isdigit() and (len(card_number) == 16) and (
(card_number[0] == '5' and card_number[1] == '1' and card_number[6] == '0') or (
card_number[0] == '5' and card_number[1] == '2' and card_number[6] == '0')) or (
(card_number[0] == '5' and card_number[1] == '3' and card_number[6] == '0') or (
card_number[0] == '5' and card_number[1] == '4' and card_number[6] == '0')) or (
(card_number[0] == '5' and card_number[1] == '5' and card_number[6] == '0'))
# Visa
if card_number[0] == '4':
return card_number.isdigit() and (len(card_number) == 13 or len(card_number) == 15 or len(card_number) == 16)
return False
# Test the function
print(validate_credit_card("4532015112830366")) # True
print(validate_credit_card("377856744827801")) # False
print(validate_credit_card("5425233430109903")) # False
print(validate_credit_card("4716381813576")) # False
print(validate_credit_card("4485806375268730")) # False
print(validate_credit_card("374245455400126")) # False
print(validate_credit_card("5598273744453231")) # False
print(validate_credit_card("4556737586899855")) # False
print(validate_credit_card("6011000990139424")) # False
print(validate_credit_card("3530111333300000")) # False
Please note that there are many different types of credit card numbers based on the card schemes, and the above code is a basic implementation to validate the given test cases. A more comprehensive solution would involve checking for the Luhn algorithm and the card scheme-specific checks for each card type.
The code uses simple string checks for now, but you can replace this with a more complex algorithm if you're handling a large number of test cases.
If there are any concerns about accuracy, or if the code needs improvement, please let me know in the comments.
Hope this helps.
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
In testing credit card validation scenarios, each model demonstrated distinct approaches. ChatGPT 4 provided comprehensive coverage with detailed explanations of valid and invalid cases. Claude Sonnet delivered a well-organized, concise framework focusing on key validation categories. Gemini Pro offered thorough testing scenarios with clear categorization and security considerations. Llama 70B gave the most detailed test cases with specific inputs and expected outputs.
Response times varied significantly: Llama (5.2s), Claude (7.2s), Gemini (20.2s), ChatGPT (37.4s). For practical testing implementation, Claude's structured yet concise approach was most effective, while Llama's detailed test cases provided the most granular validation scenarios.
Budget Tier
Analyzing the responses from all four models for credit card validation test scenarios:
ChatGPT 4o Mini provided comprehensive test cases with clearly structured valid/invalid scenarios, including detailed inputs and expected outputs. Response time was slowest at 17.7s.
Gemini 1.5 Flash offered well-organized test categories focusing on positive/negative tests and boundary conditions. Notable for including error handling scenarios. Fast response at 7.8s.
Claude Haiku delivered a thorough technical outline covering validation checks, edge cases and security considerations. Good balance of detail and organization in 12s.
Llama 3.1 was fastest (1.9s) but provided more basic scenarios and included unnecessary implementation code, making it less focused on the core testing requirements.
Best overall: Claude for completeness and structure.
FizzBuzz Implementation Tests
Develops test cases for FizzBuzz implementation, testing multiples of 3, 5, both, and regular numbers
Prompt:
Create test cases for a function that implements FizzBuzz
Performance Results
When creating test cases for a function that implements the FizzBuzz problem, you want to cover a variety of scenarios to ensure that the function behaves correctly. The FizzBuzz problem typically requires printing numbers from 1 to n, but for multiples of 3, it prints "Fizz" instead of the number, for multiples of 5, it prints "Buzz", and for multiples of both 3 and 5, it prints "FizzBuzz".
Below are example test cases for a FizzBuzz function:
Basic Functionality Tests:
Test Case 1: Input is 1
Input:
1
Expected Output:
[1]
Description: Tests the simplest non-zero positive case.
Test Case 2: Input is 3
Input:
3
Expected Output:
[1, 2, "Fizz"]
Description: Tests the scenario where only one multiple of 3 is included.
Test Case 3: Input is 5
Input:
5
Expected Output:
[1, 2, "Fizz", 4, "Buzz"]
Description: Tests the scenario that includes both "Fizz" and "Buzz".
Test Case 4: Input is 15
Input:
15
Expected Output:
[1, 2, "Fizz", 4, "Buzz", "Fizz", 7, 8, "Fizz", "Buzz", 11, "Fizz", 13, 14, "FizzBuzz"]
Description: Tests a scenario that includes "FizzBuzz", "Fizz", and "Buzz" conditions.
Edge Cases:
Test Case 5: Input is 0 or a Negative Number
Input:
0
Expected Output:
[]
Description: Tests the behavior when the input is zero, expecting no output since there's no number to process.
Test Case 6: Input is Negative
Input:
-3
Expected Output:
[]
Description: Expecting the function to handle negative inputs gracefully (no output).
Large Number Test:
- Test Case 7: Large Input
- Input:
100
- Expected Output:
[1, 2, "Fizz", 4, "Buzz", ... , 97, 98, "Fizz", "Buzz"]
- Description: Ensures the function handles larger ranges efficiently and correctly.
Non-Integer Input:
- Test Case 8: Non-Integer Input
- Input:
'a'
orNone
- Expected Output:
TypeError
or a custom error message - Description: Tests how the function handles invalid input types (non-integers).
These test cases ensure that the FizzBuzz function is thoroughly vetted for correctness, handling of edge cases, efficiency, and input validation. Adjust the implementation specifics as per the requirements, such as whether the function should throw errors or return empty lists for non-positive input.
describe('FizzBuzz', () => {
it('should return number as string when not divisible by 3 or 5', () => {
expect(fizzBuzz(1)).toBe('1');
expect(fizzBuzz(2)).toBe('2');
expect(fizzBuzz(4)).toBe('4');
});
it('should return "Fizz" when number is divisible by 3', () => {
expect(fizzBuzz(3)).toBe('Fizz');
expect(fizzBuzz(6)).toBe('Fizz');
expect(fizzBuzz(9)).toBe('Fizz');
});
it('should return "Buzz" when number is divisible by 5', () => {
expect(fizzBuzz(5)).toBe('Buzz');
expect(fizzBuzz(10)).toBe('Buzz');
expect(fizzBuzz(20)).toBe('Buzz');
});
it('should return "FizzBuzz" when number is divisible by both 3 and 5', () => {
expect(fizzBuzz(15)).toBe('FizzBuzz');
expect(fizzBuzz(30)).toBe('FizzBuzz');
expect(fizzBuzz(45)).toBe('FizzBuzz');
});
it('should throw error when input is not a number', () => {
expect(() => fizzBuzz('abc')).toThrow('Input must be a number');
expect(() => fizzBuzz(null)).toThrow('Input must be a number');
expect(() => fizzBuzz(undefined)).toThrow('Input must be a number');
});
it('should throw error when input is negative', () => {
expect(() => fizzBuzz(-1)).toThrow('Input must be a positive number');
expect(() => fizzBuzz(-15)).toThrow('Input must be a positive number');
});
it('should throw error when input is zero', () => {
expect(() => fizzBuzz(0)).toThrow('Input must be a positive number');
});
});
import unittest
def fizzbuzz(n):
"""
Implements the FizzBuzz algorithm.
Args:
n: An integer.
Returns:
A string:
"Fizz" if n is divisible by 3.
"Buzz" if n is divisible by 5.
"FizzBuzz" if n is divisible by both 3 and 5.
str(n) otherwise.
"""
if n % 3 == 0 and n % 5 == 0:
return "FizzBuzz"
elif n % 3 == 0:
return "Fizz"
elif n % 5 == 0:
return "Buzz"
else:
return str(n)
class TestFizzBuzz(unittest.TestCase):
def test_fizz(self):
self.assertEqual(fizzbuzz(3), "Fizz")
self.assertEqual(fizzbuzz(6), "Fizz")
self.assertEqual(fizzbuzz(99), "Fizz")
def test_buzz(self):
self.assertEqual(fizzbuzz(5), "Buzz")
self.assertEqual(fizzbuzz(10), "Buzz")
self.assertEqual(fizzbuzz(100), "Buzz")
def test_fizzbuzz(self):
self.assertEqual(fizzbuzz(15), "FizzBuzz")
self.assertEqual(fizzbuzz(30), "FizzBuzz")
self.assertEqual(fizzbuzz(45), "FizzBuzz")
def test_numbers(self):
self.assertEqual(fizzbuzz(1), "1")
self.assertEqual(fizzbuzz(2), "2")
self.assertEqual(fizzbuzz(4), "4")
self.assertEqual(fizzbuzz(7), "7")
self.assertEqual(fizzbuzz(11), "11")
self.assertEqual(fizzbuzz(101),"101")
def test_zero(self): # Important edge case
self.assertEqual(fizzbuzz(0), "FizzBuzz") # 0 is divisible by both 3 and 5
def test_negative(self): # Handling negative input, depends on desired behavior.
self.assertEqual(fizzbuzz(-3), "Fizz")
self.assertEqual(fizzbuzz(-5), "Buzz")
self.assertEqual(fizzbuzz(-15), "FizzBuzz")
def test_large_number(self): # Test with a large number
self.assertEqual(fizzbuzz(9999), "Fizz")
if __name__ == '__main__':
unittest.main()
Key improvements in these test cases:
- Organization: Tests are grouped logically (Fizz, Buzz, FizzBuzz, numbers).
- Edge Cases: Includes tests for 0 and negative numbers. How negative numbers are handled might depend on the specific requirements of your FizzBuzz implementation (some versions might return the number as a string with a "-" prefix).
- Large Number: Tests with a larger number to ensure the function scales.
- Clarity: Test method names clearly indicate what they're testing.
- Comprehensive Coverage: Covers all the main branches and conditions in the
fizzbuzz
function.
To run these tests, save the code as a Python file (e.g., test_fizzbuzz.py
) and then in your terminal:
python -m unittest test_fizzbuzz.py
Test Cases for FizzBuzz Function
The FizzBuzz function is a simple program that prints the numbers from 1 to n, but for multiples of three, it prints "Fizz" instead of the number, and for the multiples of five, it prints "Buzz". For numbers that are multiples of both three and five, it prints "FizzBuzz".
Test Case Categories
- Valid Input: Tests with valid inputs that cover a range of numbers, including multiples of three and five.
- Edge Cases: Tests with edge case inputs such as 0, negative numbers, and non-integer inputs.
- Error Handling: Tests that verify the function behaves correctly in case of invalid inputs.
Test Cases
1. Valid Input
Test Case 1: Typical Input
- Input:
fizz_buzz(15)
- Expected Output: A string containing numbers from 1 to 15, with "Fizz" for multiples of 3, "Buzz" for multiples of 5, and "FizzBuzz" for multiples of both.
- Example: "1, 2, Fizz, 4, Buzz, Fizz, 7, 8, Fizz, Buzz, 11, Fizz, 13, 14, FizzBuzz"
Test Case 2: Input with Only Multiples of 3
- Input:
fizz_buzz(12)
- Expected Output: A string containing numbers from 1 to 12, with "Fizz" for multiples of 3.
- Example: "1, 2, Fizz, 4, 5, Fizz, 7, 8, Fizz, 10, 11, Fizz"
Test Case 3: Input with Only Multiples of 5
- Input:
fizz_buzz(10)
- Expected Output: A string containing numbers from 1 to 10, with "Buzz" for multiples of 5.
- Example: "1, 2, 3, 4, Buzz, 6, 7, 8, 9, Buzz"
2. Edge Cases
Test Case 4: Zero Input
- Input:
fizz_buzz(0)
- Expected Output: An empty string or a message indicating no numbers to process.
Test Case 5: Negative Input
- Input:
fizz_buzz(-10)
- Expected Output: An error message or an empty string, depending on the implementation.
Test Case 6: Non-Integer Input
- Input:
fizz_buzz(10.5)
- Expected Output: An error message or an empty string, depending on the implementation.
3. Error Handling
Test Case 7: Non-Positive Integer Input with Custom Error Message
- Input:
fizz_buzz(-10)
- Expected Output: A custom error message, such as "Input must be a positive integer."
Test Case 8: Non-Integer Input with Custom Error Message
- Input:
fizz_buzz(10.5)
- Expected Output: A custom error message, such as "Input must be an integer."
Example Use Case
def fizz_buzz(n):
"""
Returns a string containing numbers from 1 to n,
with "Fizz" for multiples of 3 and "Buzz" for multiples of 5.
Args:
n (int): The upper limit of the range.
Returns:
str: A string containing numbers and "Fizz" or "Buzz" where applicable.
"""
if not isinstance(n, int) or n <= 0:
raise ValueError("Input must be a positive integer.")
result = []
for i in range(1, n + 1):
if i % 3 == 0 and i % 5 == 0:
result.append("FizzBuzz")
elif i % 3 == 0:
result.append("Fizz")
elif i % 5 == 0:
result.append("Buzz")
else:
result.append(str(i))
return ", ".join(result)
# Test cases
print(fizz_buzz(15)) # Typical Input
print(fizz_buzz(12)) # Input with Only Multiples of 3
print(fizz_buzz(10)) # Input with Only Multiples of 5
print(fizz_buzz(0)) # Zero Input
try:
print(fizz_buzz(-10)) # Negative Input
except ValueError as e:
print(e)
try:
print(fizz_buzz(10.5)) # Non-Integer Input
except ValueError as e:
print(e)
Note: These test cases are not exhaustive, and you may want to add more test cases based on your specific requirements and implementation.
AnyModel: Explore and compare over 50 AI models side-by-side.
Budget Results
Sure! The FizzBuzz problem is a common coding exercise that requires printing numbers from 1 to a specified limit. For multiples of 3, print "Fizz" instead of the number, for multiples of 5 print "Buzz", and for numbers that are multiples of both 3 and 5, print "FizzBuzz".
When creating test cases for a FizzBuzz function, we want to consider various scenarios to ensure that our function behaves as expected. Here are some test cases you can use:
Test Cases for FizzBuzz Function
Test Case 1: Basic Functionality
- Input:
1
- Expected Output:
["1"]
Test Case 2: Fizz for multiples of 3
- Input:
3
- Expected Output:
["1", "2", "Fizz"]
Test Case 3: Buzz for multiples of 5
- Input:
5
- Expected Output:
["1", "2", "Fizz", "4", "Buzz"]
Test Case 4: FizzBuzz for multiples of both 3 and 5
- Input:
15
- Expected Output:
["1", "2", "Fizz", "4", "Buzz", "Fizz", "7", "8", "Fizz", "Buzz", "11", "Fizz", "13", "14", "FizzBuzz"]
Test Case 5: Larger Input
- Input:
30
- Expected Output:
[
"1", "2", "Fizz", "4", "Buzz", "Fizz", "7", "8", "Fizz", "Buzz",
"11", "Fizz", "13", "14", "FizzBuzz", "16", "17", "Fizz", "19", "Buzz",
"Fizz", "22", "23", "Fizz", "Buzz", "26", "Fizz", "28", "29", "FizzBuzz"
]
Test Case 6: Input of 0
- Input:
0
- Expected Output:
[]
(assuming we don't print anything for non-positive numbers)
Test Case 7: Check for Accuracy and Length of Output
- Input:
16
- Expected Output:
[
"1", "2", "Fizz", "4", "Buzz", "Fizz", "7", "8", "Fizz", "Buzz",
"11", "Fizz", "13", "14", "Fizz", "16"
]
- Output Length:
16
(to ensure the correct length of the output)
Edge Case
- Input:
-5
- Expected Output:
[]
(assuming we return nothing for negative inputs)
Additional Test for Very Large Input
- Input:
100
- Expected Output:
[
"1", "2", "Fizz", "4", "Buzz", "Fizz", "7", "8", "Fizz", "Buzz",
"11", "Fizz", "13", "14", "FizzBuzz", ..., "97", "98", "Fizz", "Buzz"
]
Each of these test cases can be used to validate that the FizzBuzz function handles both normal cases and edge cases correctly. Make sure to handle various scenarios in your implementation to ensure robustness.
The following test cases cover various scenarios for a FizzBuzz function, aiming for comprehensive coverage. We'll assume the function takes an integer n
as input and returns a list or similar structure containing the FizzBuzz sequence up to n
.
Test Cases:
| Test Case ID | Description | Input (n
) | Expected Output | Pass/Fail |
|--------------|---------------------------------|-------------|-----------------------------------------------|-----------|
| 1 | Empty sequence | 0 | [] or equivalent empty sequence | |
| 2 | Single number | 1 | [1] | |
| 3 | Fizz | 3 | [1, 2, "Fizz"] | |
| 4 | Buzz | 5 | [1, 2, "Fizz", 4, "Buzz"] | |
| 5 | FizzBuzz | 15 | [1, 2, "Fizz", 4, "Buzz", "Fizz", 7, 8, "Fizz", "Buzz", 11, "Fizz", 13, 14, "FizzBuzz"] | |
| 6 | Multiple Fizz and Buzz | 20 | … (Extend the sequence above) | |
| 7 | Large number | 100 | … (A long sequence) | |
| 8 | Negative input (handle gracefully)| -5 | [] or handle appropriately (e.g., raise exception) | |
| 9 | Zero input | 0 | [] or equivalent empty sequence | |
| 10 | Boundary condition (large number)| 1000 | … (Test for potential overflow or performance issues) | |
| 11 | Edge case: Divisible by 15 | 30 | … (Ensure correct "FizzBuzz" placement) | |
| 12 | Edge case: Only divisible by 3 & 5 separately| 105 | … (Should be "FizzBuzz") | |
How to use these test cases:
Choose a testing framework: Use a unit testing framework like
unittest
(Python),pytest
(Python),JUnit
(Java),Jest
(JavaScript), etc.Write test functions: For each test case, create a test function that:
- Calls your FizzBuzz function with the input (
n
). - Compares the returned output with the expected output using the framework's assertion methods (e.g.,
assertEqual
inunittest
).
- Run the tests: Execute your test suite to see which tests pass and which fail. The framework will provide detailed reports.
Example using Python's unittest
:
import unittest
def fizzbuzz(n):
"""Implements the FizzBuzz sequence."""
result = []
for i in range(1, n + 1):
output = ""
if i % 3 == 0:
output += "Fizz"
if i % 5 == 0:
output += "Buzz"
result.append(output or i) # Use i if output is empty
return result
class TestFizzBuzz(unittest.TestCase):
def test_empty(self):
self.assertEqual(fizzbuzz(0), [])
def test_single(self):
self.assertEqual(fizzbuzz(1), [1])
def test_fizz(self):
self.assertEqual(fizzbuzz(3), [1, 2, "Fizz"])
# ... Add more test cases based on the table above ...
if __name__ == '__main__':
unittest.main()
Remember to adapt this example to your chosen programming language and testing framework. The key is to have a structured set of tests that cover a wide range of inputs and potential edge cases. Thorough testing ensures your FizzBuzz function behaves correctly in all situations.
Here are comprehensive test cases for a FizzBuzz function using various testing strategies:
# FizzBuzz Function Test Cases
## Basic Functionality Tests
1. Test number divisible by 3
- Input: 3
- Expected Output: "Fizz"
2. Test number divisible by 5
- Input: 5
- Expected Output: "Buzz"
3. Test number divisible by both 3 and 5
- Input: 15
- Expected Output: "FizzBuzz"
4. Test number not divisible by 3 or 5
- Input: 4
- Expected Output: "4"
## Boundary and Edge Cases
5. Test zero
- Input: 0
- Expected Output: "FizzBuzz"
6. Test negative numbers
- Input: -3
- Expected Output: "Fizz"
7. Test large numbers
- Input: 300
- Expected Output: "FizzBuzz"
## Input Validation Tests
8. Test non-integer input
- Input: 3.5
- Expected Behavior: Raise TypeError or return appropriate error
## Performance and Range Tests
9. Verify correct output for range 1-100
- Input: Range from 1 to 100
- Verify correct sequence of outputs follows FizzBuzz rules
## Sample Test Case Structure
python def testfizzbuzz(fizzbuzzfunction): assert fizzbuzzfunction(3) == "Fizz" assert fizzbuzzfunction(5) == "Buzz" assert fizzbuzzfunction(15) == "FizzBuzz" assert fizzbuzzfunction(4) == "4"
## Test Coverage Considerations
- Multiple cases for divisibility
- Edge case handling
- Input validation
- Performance with large inputs
These test cases cover various scenarios to ensure robust FizzBuzz function implementation.
FizzBuzz Function
def fizzbuzz(n):
"""
Returns a list of FizzBuzz values for numbers from 1 to n.
:param n: The upper limit of the range.
:return: A list of FizzBuzz values.
"""
fizzbuzz_values = []
for i in range(1, n + 1):
if i % 3 == 0 and i % 5 == 0:
fizzbuzz_values.append("FizzBuzz")
elif i % 3 == 0:
fizzbuzz_values.append("Fizz")
elif i % 5 == 0:
fizzbuzz_values.append("Buzz")
else:
fizzbuzz_values.append(i)
return fizzbuzz_values
Test Cases
import unittest
class TestFizzbuzzFunction(unittest.TestCase):
def test_fizzbuzz_with_multiple_of_3_and_5(self):
self.assertEqual(fizzbuzz(15), ["FizzBuzz"])
def test_fizz_with_multiple_of_3(self):
self.assertEqual(fizzbuzz(3), ["Fizz"])
def test_buzz_with_multiple_of_5(self):
self.assertEqual(fizzbuzz(5), ["Buzz"])
def test_fizzbuzz_with_multiple_of_3_and_multiple_of_5(self):
self.assertEqual(fizzbuzz(30), [i for i in range(1, 30 + 1) if i % 3 == 0 and i % 5 == 0])
def test_fizzbuzz_with_multiple_of_3_and_not_multiple_of_5(self):
self.assertEqual(fizzbuzz(12), ["Fizz"])
def test_fizzbuzz_with_not_multiple_of_3_and_multiple_of_5(self):
self.assertEqual(fizzbuzz(10), ["Buzz"])
def test_fizzbuzz_with_single_number(self):
self.assertEqual(fizzbuzz(1), [1])
def test_fizzbuzz_with_negative_input(self):
with self.assertRaises(ValueError):
fizzbuzz(-1)
def test_fizzbuzz_with_zero_input(self):
with self.assertRaises(ValueError):
fizzbuzz(0)
if __name__ == "__main__":
unittest.main()
In these test cases, we first import the unittest
module, which provides a testing framework for Python. We define a test case TestFizzbuzzFunction
that contains multiple test methods.
test_fizzbuzz_with_multiple_of_3_and_5
: Tests if the output is "FizzBuzz" for numbers that are multiples of both 3 and 5.test_fizz_with_multiple_of_3
: Tests if the output is "Fizz" for numbers that are multiples of 3.test_buzz_with_multiple_of_5
: Tests if the output is "Buzz" for numbers that are multiples of 5.test_fizzbuzz_with_multiple_of_3_and_multiple_of_5
: Tests if the output is "FizzBuzz" for numbers that are multiples of both 3 and 5.test_fizzbuzz_with_multiple_of_3_and_not_multiple_of_5
: Tests if the output is "Fizz" for numbers that are multiples of 3 but not multiples of 5.test_fizzbuzz_with_not_multiple_of_3_and_multiple_of_5
: Tests if the output is "Buzz" for numbers that are multiples of 5 but not multiples of 3.test_fizzbuzz_with_single_number
: Tests if the output is the single number for numbers not multiples of neither 3 nor 5.test_fizzbuzz_with_negative_input
: Tests if the function raises an exception for negative input values.test_fizzbuzz_with_zero_input
: Tests if the function raises an exception for input values of 0.
You can run these test cases by executing the script, and they will verify that the fizzbuzz
function behaves as expected.
AnyModel: Explore and compare over 50 AI models side-by-side.
Performance Tier
All models provided comprehensive test cases for FizzBuzz, but with distinct approaches. ChatGPT 4 offered well-structured test cases with clear descriptions and categories. Claude delivered concise, practical test code in JavaScript using modern testing frameworks. Gemini provided a complete Python implementation with unittest framework and detailed comments. Llama gave extensive documentation and example code but was somewhat verbose. ChatGPT and Gemini took longer (15s and 17s) while Claude and Llama were faster (9s and 4s). Overall, Claude's response was most efficient, combining clarity with practical implementation.
Budget Tier
Each model provided comprehensive test cases for FizzBuzz, but with different strengths. ChatGPT 4o Mini gave well-structured test cases with clear expected outputs, taking 11.9s. Gemini 1.5 Flash produced a detailed table format with test IDs and implementation examples in 7.4s. Claude Haiku offered concise test categories with example code in 7.4s. Llama 3.1 8B was fastest at 1.4s and uniquely included both function implementation and complete unittest code. Overall, Llama provided the most practical, implementation-ready solution despite being the smallest model.
Performance Verdict
After analyzing the performance of ChatGPT 4.0, Claude 3.5 Sonnet, Gemini 1.5 Pro, and Llama 3.1 70B across 15 test generation scenarios, here's the comprehensive verdict:
ChatGPT 4.0 Strengths:
- Most thorough and professional test coverage
- Excellent documentation and explanations
- Superior organization and structure
- Best at handling complex test scenarios Weaknesses:
- Consistently slower response times
- Sometimes overly detailed for simple tasks
Claude 3.5 Sonnet Strengths:
- Best balance of speed and completeness
- Clean, practical code implementations
- Excellent error handling
- Efficient and well-organized responses Weaknesses:
- Less detailed explanations than ChatGPT
- Sometimes prioritizes brevity over comprehensiveness
Gemini 1.5 Pro Strengths:
- Most complete solutions with implementation code
- Excellent documentation and comments
- Unique testing insights and improvements Weaknesses:
- Longest response times
- Sometimes overly complex for simple tasks
- Inconsistent performance across different scenarios
Llama 3.1 70B Strengths:
- Significantly faster response times
- Good basic test coverage
- Clean documentation structure Weaknesses:
- Less comprehensive than other models
- Basic implementations
- Limited edge case coverage
Winner: Claude 3.5 Sonnet
Claude wins for consistently delivering the optimal balance of speed, practicality, and comprehensiveness. While ChatGPT often provided more detailed responses, Claude's solutions were more immediately implementable and time-efficient. Claude demonstrated superior error handling and consistently provided well-structured test cases that could be directly integrated into development workflows.
Runner-up: ChatGPT 4.0, which excelled in thoroughness and professional documentation but was held back by slower response times.
Budget Verdict
Based on the collected analyses across multiple test generation prompts, here's a comparative verdict of the models:
ChatGPT 4o Mini: Strengths:
- Most comprehensive test coverage and documentation
- Excellent handling of edge cases
- Clear explanations and structured output Weaknesses:
- Consistently slowest response times (11-17 seconds)
- Sometimes overly verbose
Gemini 1.5 Flash: Strengths:
- Best balance of speed and quality
- Well-organized output, often in tabular format
- Strong focus on practical implementation
- Consistent response times (5-7 seconds) Weaknesses:
- Occasionally too implementation-focused rather than test-case oriented
Claude 3.5 Haiku: Strengths:
- Excellent test categorization and structure
- Strong technical documentation
- Thorough edge case coverage Weaknesses:
- Relatively slow response times (10-14 seconds)
- Sometimes too focused on theory over practical implementation
Llama 3.1 8B: Strengths:
- Extremely fast response times (0.8-1.9 seconds)
- Practical, implementation-ready code
- Good basic test coverage Weaknesses:
- Less comprehensive test scenarios
- Sometimes includes unnecessary implementation details
- Less structured output
Overall Winner: Gemini 1.5 Flash
Gemini consistently demonstrated the best balance of speed, comprehensiveness, and practical utility. While it may not have had the absolute best test coverage (ChatGPT) or fastest speed (Llama), it consistently delivered well-structured, implementation-ready test cases with reasonable response times. Its ability to maintain high quality while being significantly faster than ChatGPT and Claude makes it the most practical choice for real-world test generation tasks.
Runner-up: ChatGPT 4o Mini, which showed superior comprehensiveness but was held back by slower response times.
Conclusion
Conclusion
This comprehensive analysis of AI models' performance in test generation tasks reveals distinct patterns and capabilities across both performance and budget tiers. The evaluation demonstrates that while higher-performance models generally deliver more thorough and nuanced results, budget-tier models can still provide practical, efficient solutions for many test generation needs.
In the performance tier, Claude 3.5 Sonnet emerged as the leader, offering the optimal balance of speed, practicality, and comprehensive test coverage. Its consistent delivery of implementable solutions and superior error handling made it stand out, despite ChatGPT 4.0's more detailed documentation.
In the budget tier, Gemini 1.5 Flash proved to be the most effective solution, combining reasonable speed with well-structured test cases and practical implementation guidance. While not matching the depth of its higher-tier counterparts, it demonstrated that cost-effective solutions can still deliver professional-grade results.
The analysis also highlighted an important trade-off between processing speed and comprehensiveness. Faster models like Llama 3.1 8B excelled in response time but typically provided simpler solutions, while slower models like ChatGPT offered more thorough coverage at the cost of longer processing times.
These findings suggest that organizations should carefully consider their specific needs when selecting an AI model for test generation. Those requiring quick, practical solutions might find budget-tier models sufficient, while projects demanding comprehensive test coverage and detailed documentation might benefit from performance-tier models despite their higher cost and longer processing times.